Strings | Lecture 12 | Java Placement Series
Summary
TLDRThis Java tutorial video focuses on the concept of Strings, explaining that Strings are non-primitive data types used extensively in Java. The instructor covers String declaration, manipulation, and memory usage. They teach how to declare a String, input Strings from a user, and concatenate Strings. The video also discusses String methods like length(), charAt(), and comparison using compareTo(). It emphasizes the immutability of Strings and the importance of using the compareTo() method for accurate comparison, hinting at the String Builder class to be covered in the next video.
Takeaways
- 😀 Strings in Java are non-primitive data types, which means their length and content can be defined by the programmer.
- ✍️ The 'String' class in Java is used to define strings, and it is important to note the capital 'S' to avoid errors.
- 🔑 Strings can store various types of text, such as sentences, words, or characters, and are extensively used in Java.
- 🧠 The concept of strings will be further explored in future videos, specifically with the String Builder class.
- 🔍 To declare a string in Java, you specify the data type 'String', followed by the variable name and the string value to be stored.
- 🔢 Java allows you to input strings from the user and store them using the Scanner class.
- 🔗 The 'concat' function is used to join two strings together in Java.
- 📏 The 'length' method is used to calculate the length of a string in Java.
- 🔎 The 'charAt' method allows you to access and manipulate individual characters within a string.
- 🆚 The 'compareTo' function is used to compare two strings in Java, considering case sensitivity.
- 🔄 Java strings are immutable, meaning they cannot be modified after creation. Any change requires the creation of a new string.
Q & A
What is a string in Java?
-A string in Java is a non-primitive data type used to store sequences of characters. It can be defined by the programmer to store any text, sentence, or characters as a single entity.
Why is it important to use capital 'S' when declaring the String class in Java?
-In Java, the String class is case-sensitive, and it must be declared with a capital 'S'. Many students make the mistake of not capitalizing it, which can lead to errors in the program.
How do you declare a string variable in Java?
-You declare a string variable in Java by first specifying the data type 'String', followed by the variable name, and then assigning it a value, such as 'String name = "Tony";'.
What is the significance of the 'length' method in strings?
-The 'length' method in Java is used to calculate the number of characters in a string. It is a built-in method that returns the length of the string when called.
How can you take input from the user and store it as a string in a Java program?
-To take input from the user and store it as a string, you need to create an object of the Scanner class and use its 'nextLine' method to read the input as a full line of text.
What is the difference between using 'next' and 'nextLine' when taking input in Java?
-The 'next' method is used to take a single word input, while 'nextLine' is used to take a full line of input, including spaces.
How can you concatenate two strings in Java?
-In Java, you can concatenate two strings by using the '+' operator to join them together, or by using the 'concat' method of the String class.
What is the purpose of the 'charAt' method in strings?
-The 'charAt' method in Java is used to access a character at a specific index within a string. It returns the character at the given index position.
How does the 'substring' method work in Java?
-The 'substring' method in Java is used to extract a part of a string. It takes a starting index and an optional ending index to define the portion of the string to return.
Why is it recommended to use the 'compareTo' method instead of 'equals' when comparing strings in Java?
-The 'compareTo' method is recommended over 'equals' for comparing strings in Java because 'equals' can fail in certain cases where 'compareTo' provides a lexicographical comparison that is more reliable.
What is the concept of immutability in strings and why is it important?
-In Java, strings are immutable, which means once a string is created, it cannot be changed. Any operation that appears to modify a string actually creates a new string. This concept is important because it ensures that strings behave in a predictable and safe manner, especially when used as keys in a Hashtable or a similar data structure.
Outlines
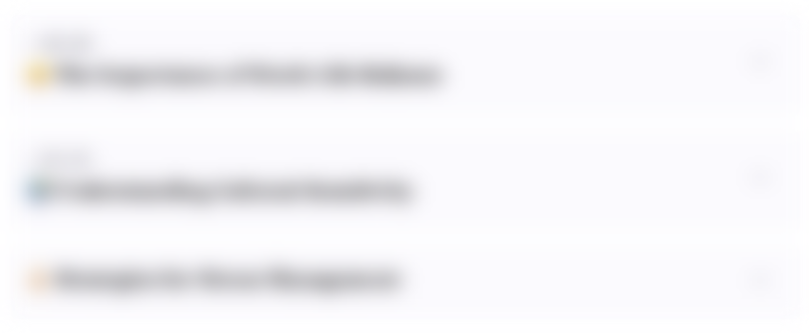
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
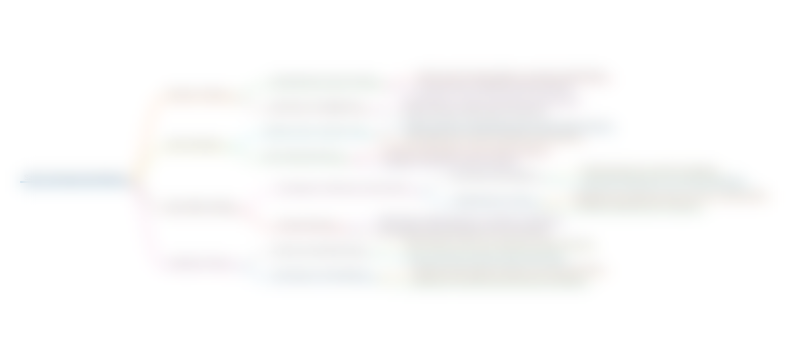
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
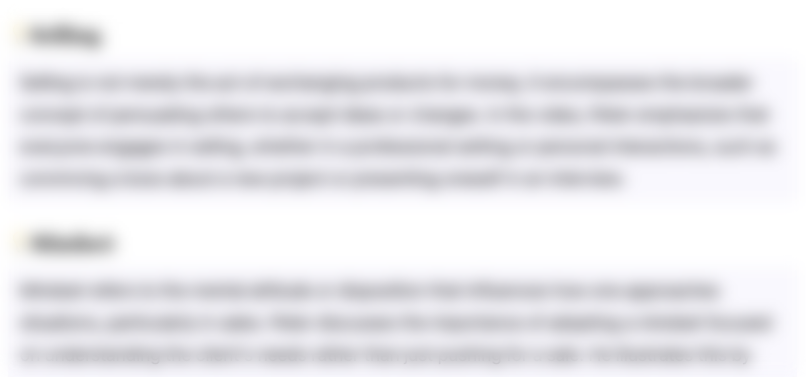
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
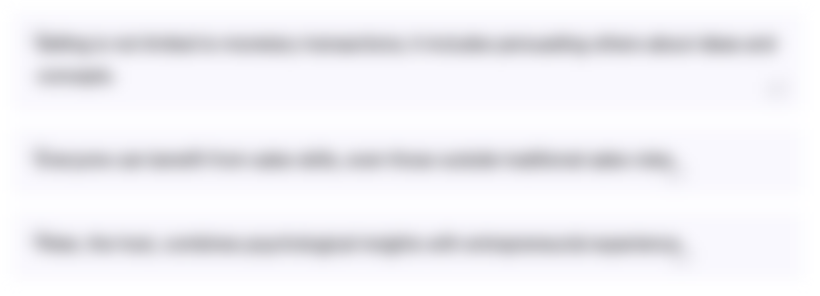
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
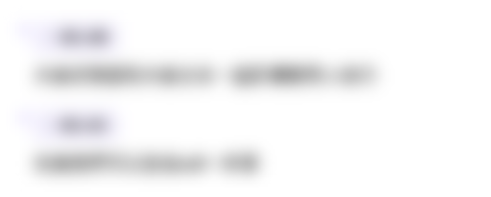
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)