Data Types in Java | Master DSA in Java
Summary
TLDRThis video script offers an in-depth exploration of Java's data types, emphasizing the importance of understanding them in a strongly typed language. It covers primitive data types such as byte, short, int, long, float, double, boolean, and char, explaining their memory allocation and range limitations. The script also touches on non-primitive data types like arrays and strings, which are treated as objects in Java. The default data types for integers and real numbers are highlighted, along with the mandatory specification of data types in Java, a practice crucial for clear and error-free programming.
Takeaways
- π Java is a strongly typed programming language, meaning data types must be explicitly specified when working with data.
- π’ The script introduces Java's primitive data types, including byte, short, int, long, float, double, boolean, and char, each with specific memory allocation and use cases.
- π The memory allocation for storing data types in Java varies: byte (1 byte), short (2 bytes), int (4 bytes), and long (8 bytes), affecting how numbers are stored and manipulated.
- π The range of values that can be stored with each data type is defined by its memory size, with byte having the smallest range and long the largest.
- π― When a specific data type is not mentioned for a whole number in Java, the default data type is int.
- π For real numbers with fractional parts, Java offers the float and double data types, with float requiring an 'f' suffix and double being the default without a suffix.
- π The memory allocation for float is 4 bytes and for double is 8 bytes, reflecting their capacity for precision.
- π Boolean data type in Java can only store two values: true or false, and the memory allocation for boolean is JVM-specific with no fixed byte size.
- ποΈ The char data type is used to store a single character, with 2 bytes of memory allocated per character.
- π The script emphasizes the importance of understanding data types for memory optimization and proper data handling in Java.
- π The video also mentions non-primitive data types like arrays and strings, which are considered objects in Java, and will be discussed in more detail in future lessons.
Q & A
Why is understanding data types crucial when working with Java?
-Understanding data types is crucial in Java because it is a strongly typed programming language, which requires specifying the type of data being worked with. This ensures the correct allocation of memory and the appropriate range of values for variables.
What are the primitive data types supported by Java?
-Java supports several primitive data types including byte, short, int, long, float, double, boolean, and char. These types allow direct storage of values in memory.
Why are there different types for storing integer values in Java, such as byte, short, int, and long?
-Different types for integer values allow for memory optimization and accommodate different ranges of numbers. The choice among byte, short, int, and long depends on the size of the number to be stored, with each type occupying a different amount of memory and allowing a specific range of values.
What is the significance of the 'L' suffix when declaring a long variable in Java?
-The 'L' suffix is used to denote a long literal in Java. It is mandatory when declaring a long variable to avoid confusion with an int, especially when the literal exceeds the range of int.
What is the default data type for a whole number in Java if no type is specified?
-In Java, if no data type is specified for a whole number, the default data type is int.
How does Java handle the result of an operation on whole numbers?
-In Java, the result of an operation on whole numbers is always an integer, regardless of the original data types of the operands, unless explicitly cast to a smaller data type like byte or short.
What are the two values that can be stored using the Boolean data type in Java?
-The Boolean data type in Java can store only two values: true and false.
What is the purpose of the char data type in Java?
-The char data type in Java is used to store a single character, including alphabets, numbers, and special characters, within single quotes.
Why is the memory allocation for the Boolean data type in Java considered JVM-specific and not fixed?
-The memory allocation for the Boolean data type in Java is JVM-specific because the Java Virtual Machine may optimize storage for Boolean values differently depending on the implementation, and there is no fixed documentation for the exact number of bytes used.
What is the default data type for a real number in Java if no type is specified?
-In Java, if no data type is specified for a real number, the default data type is double.
What suffix is required when declaring a float literal in Java?
-When declaring a float literal in Java, an 'f' or 'F' suffix is required to differentiate it from a double literal.
How many bytes of memory are typically allocated for storing a char value in Java?
-In Java, storing a char value typically occupies two bytes of memory.
Outlines
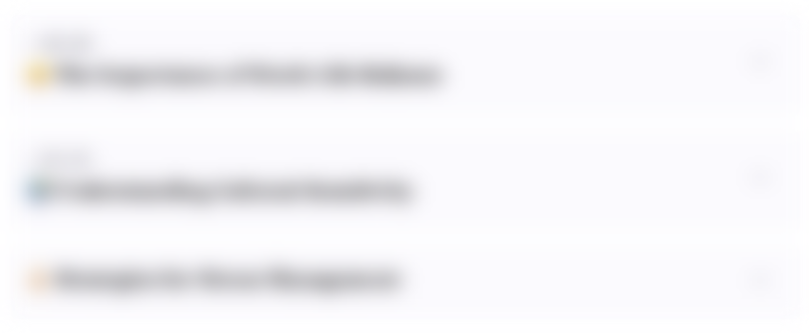
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
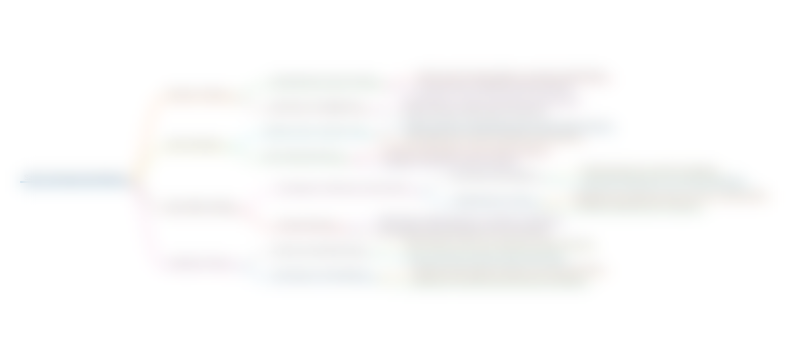
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
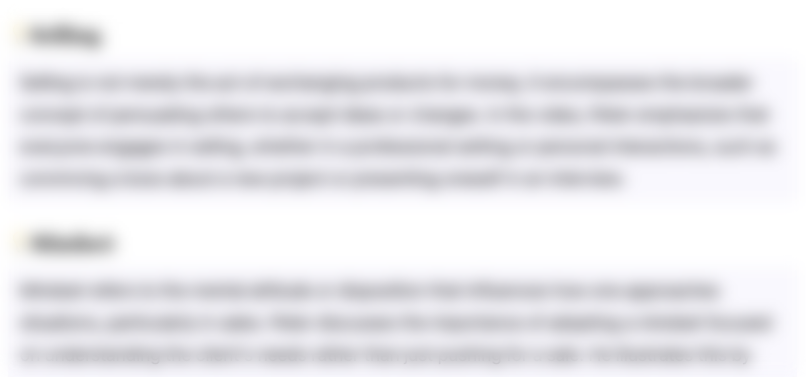
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
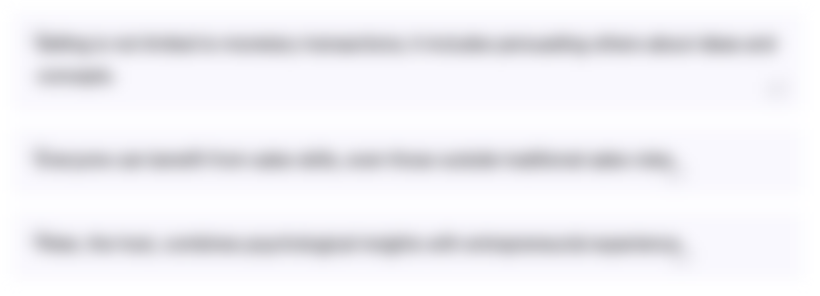
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
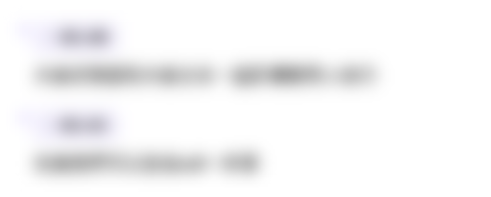
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)