Variables in Java ✘【12 minutes】
Summary
TLDRThis video tutorial introduces viewers to the concept of variables in Java programming. It explains that variables act as placeholders for values, similar to algebraic variables, but with the ability to store not only numbers but also text, sentences, and boolean values. The video covers Java's eight primitive data types and the reference data type 'String', detailing the purpose and memory usage of each. It also demonstrates how to declare, assign, and initialize variables, and discusses the differences between primitive and reference data types. The tutorial concludes with practical examples of creating and using variables in Java, including handling large numbers with 'long', fractional numbers with 'float' and 'double', single characters with 'char', and strings.
Takeaways
- 📌 Variables in Java are placeholders for values and behave as the values they contain.
- 📚 Variables can store different data types, including numbers, words, sentences, and boolean values (true or false).
- 🔢 Java has eight primitive data types and a special reference data type called String.
- 🏷 The boolean data type uses one bit of memory and can only hold two values: true or false.
- 🔢 The byte data type can hold integer numbers from -128 to 127, while the short type can hold numbers up to around 32,000.
- 🔑 The int data type uses 4 bytes and can store numbers up to just over 2 billion.
- 🔑 The long data type uses 8 bytes and can store extremely large numbers, from nearly negative nine quintillion to just over nine quintillion.
- 📉 The float data type stores fractional numbers with up to six to seven digits of precision, while the double type has more precision with up to 15 digits.
- 💬 The char data type uses two bytes and stores a single character, letter, or ASCII value.
- 📝 The String data type is a reference type that stores a sequence of characters, such as words or sentences.
- 🔑 Primitive data types store data directly, use less memory, and are faster, while reference data types store an address and use more memory.
Q & A
What is the primary function of a variable in Java?
-A variable in Java acts as a placeholder for a value, and it behaves as the value that it contains, similar to how variables are used in algebra.
What are the eight primitive data types in Java?
-The eight primitive data types in Java are boolean, byte, short, int, long, float, double, and char.
What is the significance of the boolean data type in Java?
-The boolean data type in Java can only hold two values, true or false, and is typically used for binary conditions.
What is the range of values that can be stored in a byte data type in Java?
-A byte data type in Java can hold integer values between -128 and 127.
What is the difference between the int and long data types in Java?
-The int data type uses 4 bytes of memory and can store values up to just over 2 billion, while the long data type uses 8 bytes and can store much larger values, up to just over positive nine quintillion.
Why is the float data type used in Java?
-The float data type is used in Java to store fractional numbers with a precision of up to six to seven digits.
What is the main difference between float and double data types in Java when assigning values?
-When assigning values to a float variable in Java, the value must be followed by the letter 'f', whereas for a double variable, no such suffix is needed.
What is the purpose of the char data type in Java?
-The char data type in Java is used to store a single character, letter, or ASCII value, and requires single quotes around the value when assigning.
How does a string data type differ from primitive data types in Java?
-A string is a reference data type in Java, which means it stores a sequence of characters and uses more memory compared to primitive data types that store a single value with less memory.
What is the process of creating a variable in Java?
-To create a variable in Java, you first declare the data type and variable name, then you can either assign a value to it separately or combine the declaration and assignment in a single step called initialization.
How can you print the value of a variable in Java?
-In Java, you can print the value of a variable using a print or println statement, making sure not to enclose the variable in quotes unless you intend to print a string literal.
Outlines
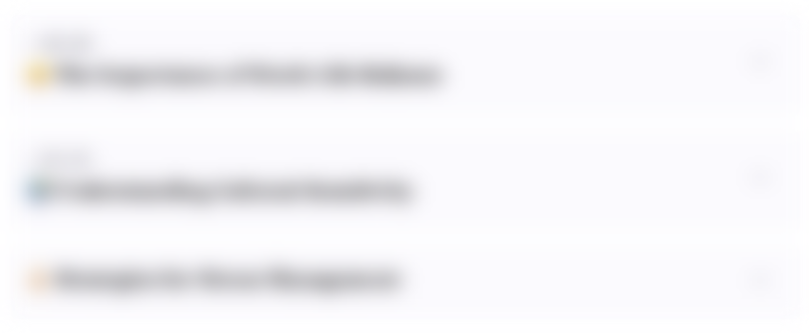
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
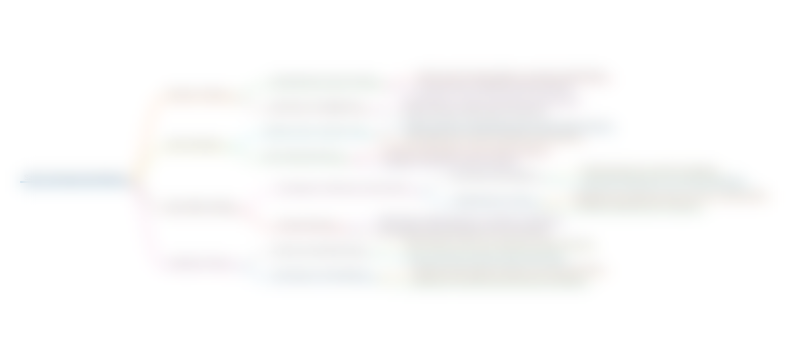
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
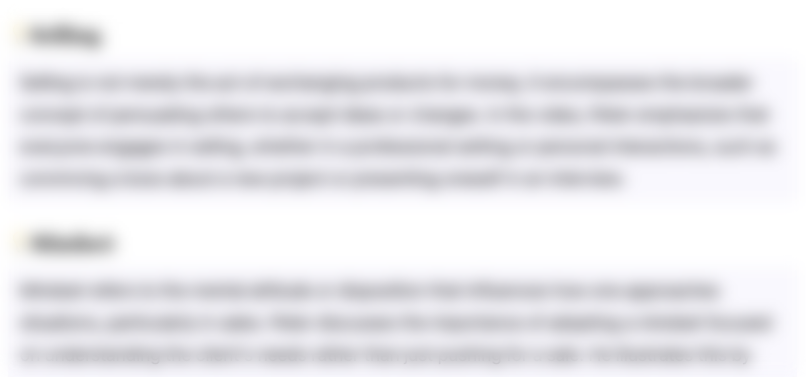
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
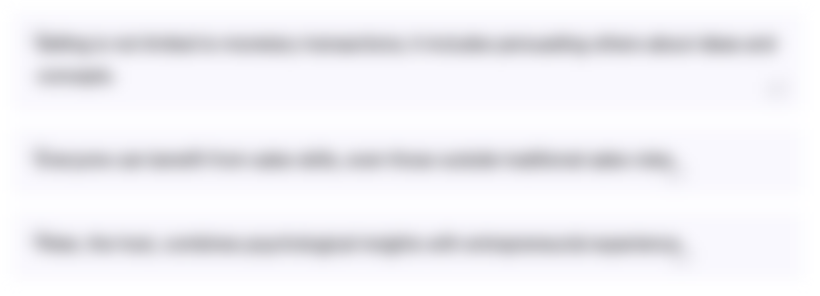
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
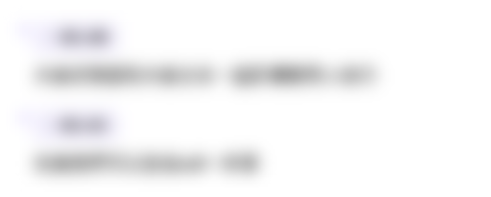
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)