#5 Variables in Java
Summary
TLDRCette vidéo explique en détail le processus de compilation en Java. L'auteur décrit comment un programme Java est écrit, compilé en bytecode avec l'extension .class, et exécuté dans la JVM (Java Virtual Machine) via le JRE (Java Runtime Environment). Il aborde également la gestion des variables, le stockage temporaire et permanent des données, et l'importance de types de données comme int et string. Le script met en avant des exemples concrets de traitement et d'affichage des données, tout en expliquant les concepts fondamentaux comme l'addition de variables et la structure d'un programme Java.
Takeaways
- 😀 En tant que développeur Java, vous écrivez du code avec une extension .java, qui est ensuite compilé en bytecode (.class).
- 💻 Le bytecode est exécuté par la JVM (Java Virtual Machine), qui fait partie de la JRE (Java Runtime Environment).
- 🔧 Le JDK (Java Development Kit) inclut à la fois la JRE et la JVM, avec des mises à jour régulières pour les nouvelles versions.
- 📅 JDK 17 est une version LTS (Long Term Support) qui reçoit des mises à jour mineures, mais les concepts de base restent les mêmes.
- 📊 La programmation sert à résoudre des problèmes du monde réel avec des solutions virtuelles, comme Amazon ou Uber.
- 🗄️ Les données sont cruciales pour les applications. Les données temporaires sont stockées dans des variables, tandis que les données permanentes vont dans une base de données.
- 🧮 Les variables sont des boîtes dans lesquelles vous stockez des données. Elles ont un type (comme int pour les entiers) et un nom.
- 🔢 Java est un langage fortement typé. Vous devez spécifier le type de données (comme int ou string) lors de la déclaration de la variable.
- ➕ Vous pouvez effectuer des opérations comme l'addition entre des variables (par exemple, num1 + num2) et stocker les résultats dans une autre variable.
- 🎯 En Java, chaque instruction se termine par un point-virgule, sauf dans les blocs de code, où ce n'est pas nécessaire.
Q & A
Qu'est-ce qu'un fichier .class en Java?
-Un fichier .class est le résultat de la compilation d'un fichier Java (.java). Ce fichier contient le bytecode, qui peut être exécuté par la JVM (Java Virtual Machine).
Quelle est la différence entre JDK et JRE?
-Le JDK (Java Development Kit) est utilisé par les développeurs pour écrire et compiler du code Java, tandis que le JRE (Java Runtime Environment) inclut la JVM et les bibliothèques nécessaires pour exécuter des applications Java.
Pourquoi utilisons-nous des bases de données dans les applications logicielles?
-Les bases de données sont utilisées pour stocker de manière permanente les données traitées par les applications, permettant de les conserver même après l'arrêt du programme ou de la machine.
Qu'est-ce qu'une variable en programmation?
-Une variable est un conteneur (ou boîte) où l'on peut stocker des données comme des nombres, du texte, des images, etc., qui peuvent être utilisées pendant l'exécution du programme.
Qu'est-ce qu'un langage fortement typé comme Java?
-Un langage fortement typé, comme Java, exige que chaque variable ait un type de données bien défini, comme int pour les entiers ou String pour les chaînes de caractères.
Quelle est la différence entre int et String en Java?
-int est utilisé pour stocker des nombres entiers (positifs, négatifs ou zéro), tandis que String est utilisé pour stocker du texte (une séquence de caractères).
Pourquoi utilise-t-on println au lieu de print en Java?
-println affiche la sortie sur une nouvelle ligne après chaque impression, tandis que print continue d'écrire sur la même ligne, ce qui peut rendre la sortie confuse.
Comment ajouter deux variables en Java?
-Pour ajouter deux variables en Java, vous pouvez simplement utiliser l'opérateur '+' entre elles, par exemple `int result = num1 + num2;` pour stocker la somme dans une autre variable.
Qu'est-ce que l'opérateur d'affectation en Java?
-L'opérateur d'affectation en Java est le signe '=', qui prend la valeur à droite et l'assigne à la variable à gauche. Par exemple, `int num = 5;` affecte la valeur 5 à la variable num.
Que signifie la compilation en Java?
-La compilation en Java est le processus par lequel le code source (.java) est transformé en bytecode (.class), que la JVM peut ensuite exécuter sur différentes plateformes.
Outlines
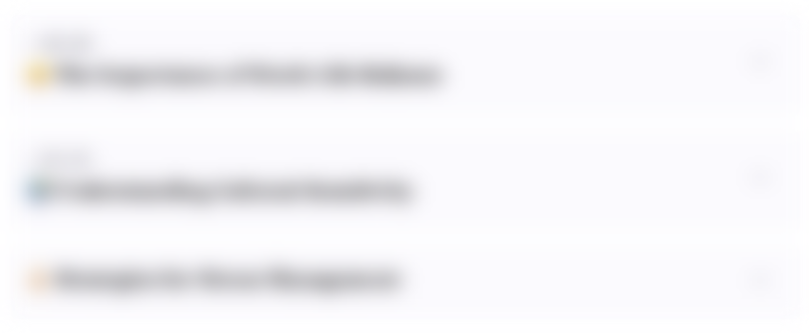
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
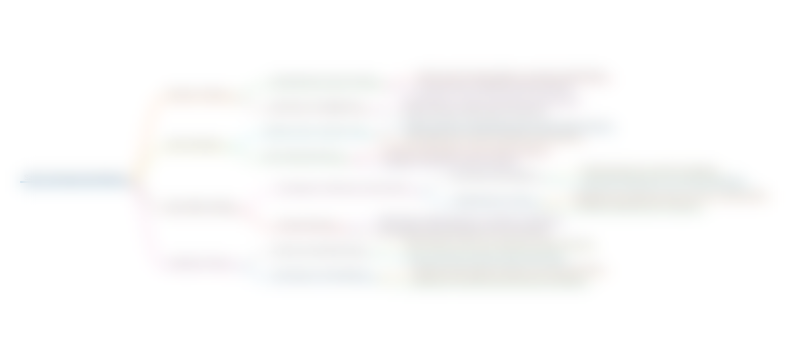
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
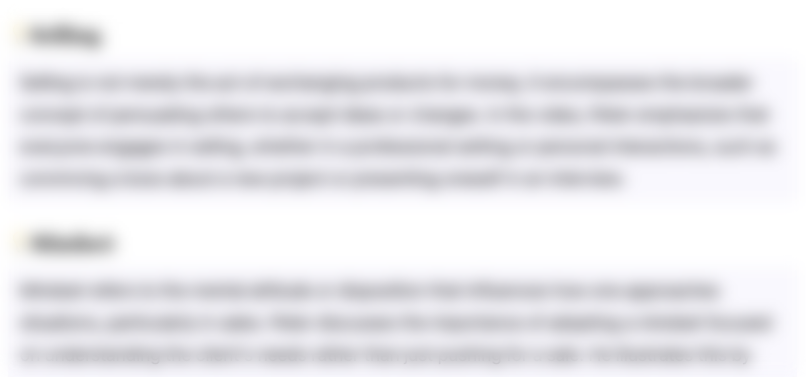
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
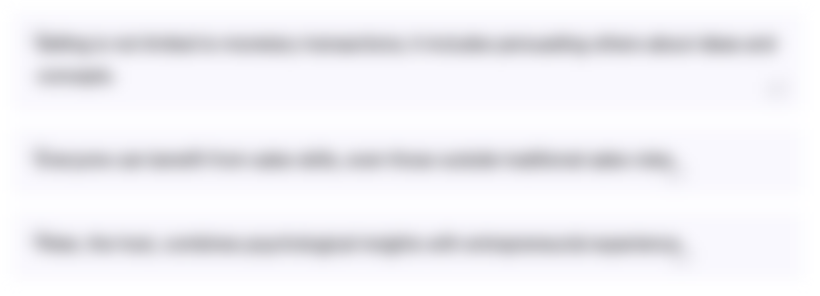
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
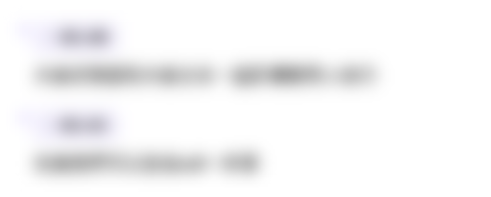
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
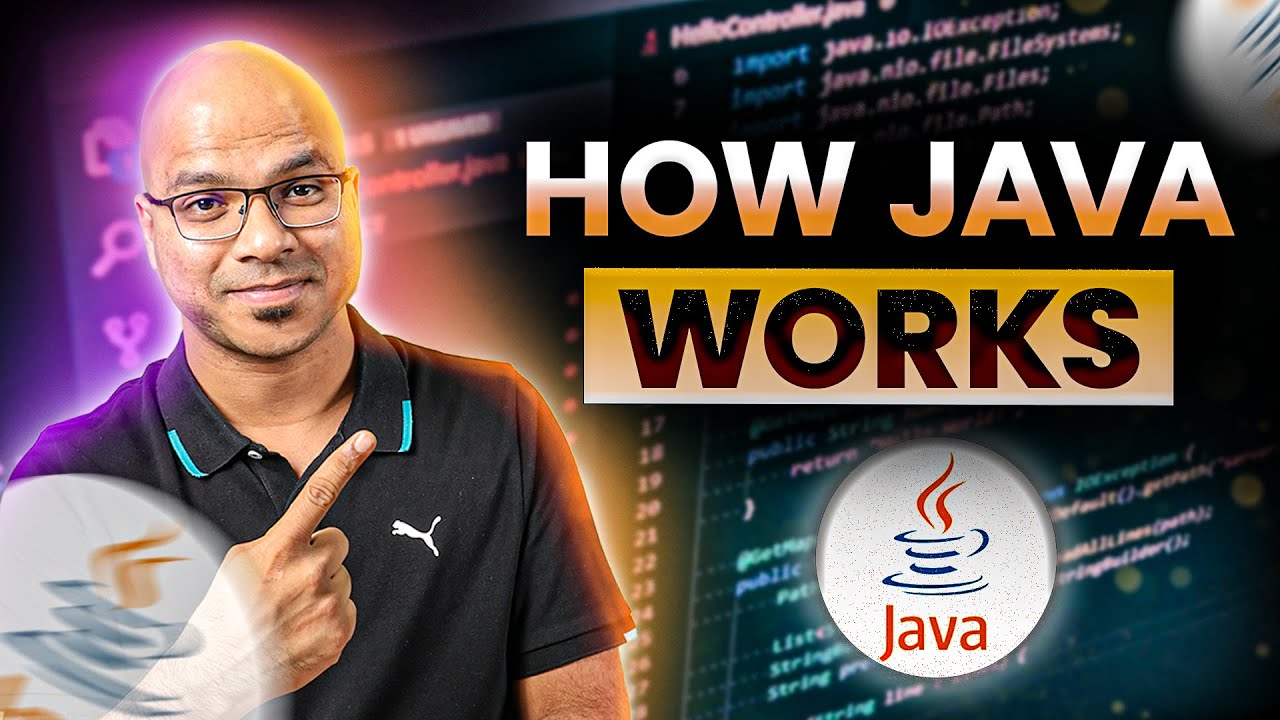
#4 How Java Works

Introduction à l'informatique avec Python : qu'est ce que programmer ?
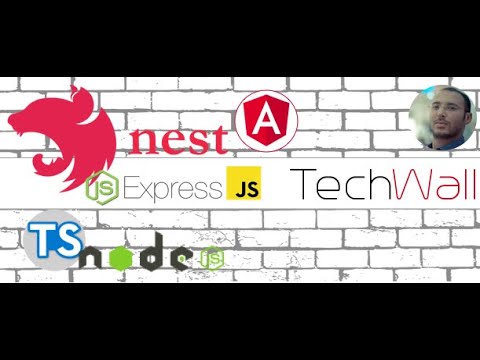
NestJs 7 Récupérer le Body de la request #9

Data Structures Explained for Beginners - How I Wish I was Taught

SQL vs NoSQL expliqué en 5 minutes
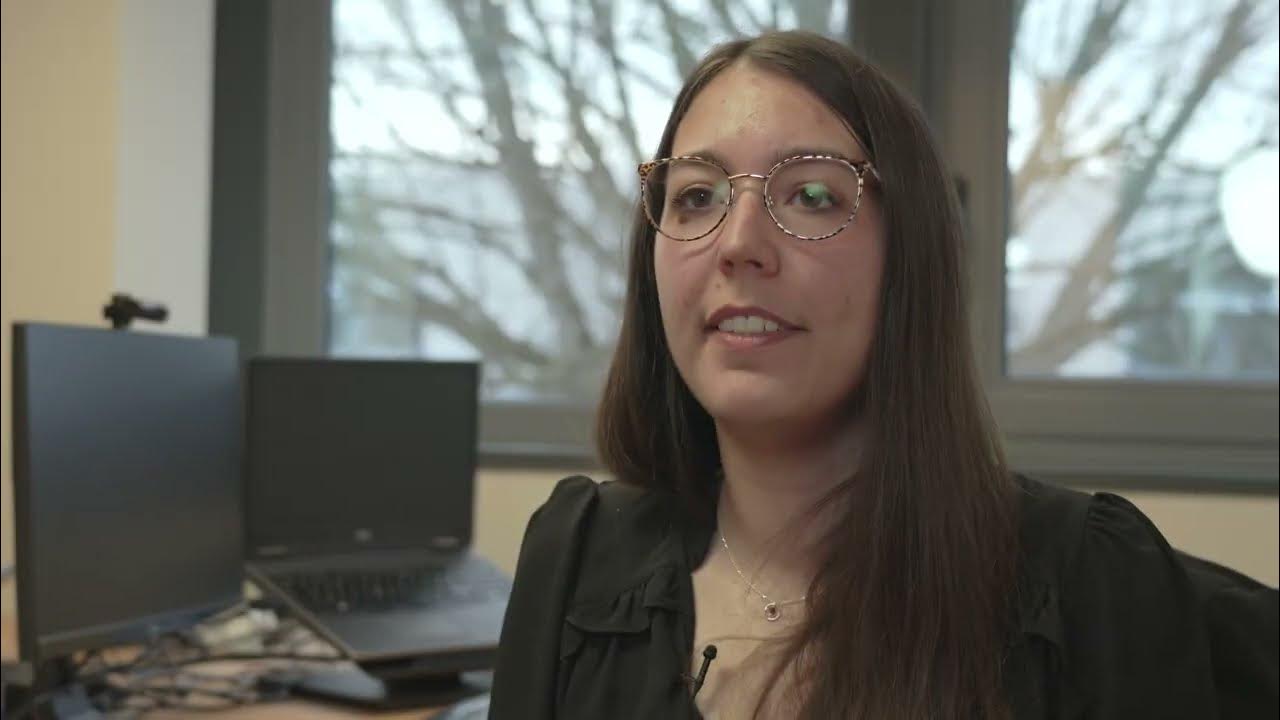
Tout comprendre sur les modèles ARVALIS, au cœur des Outils d’Aide à la Décision - ARVALIS.fr
5.0 / 5 (0 votes)