How Passing Arguments Works_ Value vs Reference | JavaScript 🔥 | Lecture 120
Summary
TLDRThis lecture delves into the intricate workings of passing arguments to functions in JavaScript, revisiting the crucial concepts of primitives vs. objects (reference types). Through practical examples, it illustrates how primitives are passed by value, creating copies, while objects are passed by reference, allowing modifications to the original object. The lecturer highlights the potential pitfalls of unintended object mutations across functions, emphasizing the need for caution in large codebases, especially when working with multiple developers. Additionally, the lecture clarifies the distinction between passing by value and passing by reference in JavaScript, addressing common misconceptions among programmers transitioning from languages like C++.
Takeaways
- 🔑 Understanding how primitives and objects are passed into functions is crucial in JavaScript.
- 💻 When a primitive value is passed into a function, a copy of that value is created, leaving the original value unchanged.
- 📘 When an object is passed into a function, a reference to the object in memory is copied, not the object itself.
- ✏️ Modifying an object parameter within a function also modifies the original object outside the function.
- ⚠️ Carelessly modifying objects through functions can lead to unintended consequences in large codebases, especially when working with multiple developers.
- 🔄 JavaScript uses pass-by-value for both primitives and objects, even though objects appear to be passed by reference.
- 🧠 The confusion around pass-by-reference in JavaScript often stems from developers coming from languages like C++ where true pass-by-reference exists.
- 🔍 In JavaScript, even when an object is passed, it's still a value (a memory reference) that's being passed, not the object itself by reference.
- 📝 Writing code examples during lectures helps reinforce learning and understanding for students.
- 🔖 The lecture covers a fundamental concept (primitives vs. objects) in the context of functions, which is essential for JavaScript developers.
Q & A
What is the main topic discussed in this lecture?
-The main topic discussed in this lecture is how arguments are passed into functions in JavaScript, and the difference between passing primitive types and reference types (objects).
What is the difference between passing a primitive type and an object to a function in JavaScript?
-When passing a primitive type (e.g., a string or number) to a function, a copy of the value is created inside the function. Changes made to this copy inside the function do not affect the original value outside the function. However, when passing an object to a function, a reference to the object in memory is passed. Changes made to the object inside the function affect the original object outside the function.
Can you explain the terms 'passing by value' and 'passing by reference' in the context of JavaScript?
-JavaScript does not have true 'passing by reference'. Instead, it uses 'passing by value', even for objects. When an object is passed to a function, the reference (memory address) of the object is passed by value, not the object itself. This reference is a value that contains the memory address of the object.
Why is it important to understand how arguments are passed to functions in JavaScript?
-Understanding how arguments are passed to functions is crucial because it can have unforeseeable consequences in large codebases, especially when working with multiple developers. It helps avoid unexpected behavior and potential issues when multiple functions manipulate the same object.
In the provided example, what happens when the `checkIn` function changes the `flightNum` parameter?
-When the `checkIn` function changes the `flightNum` parameter, it does not affect the original `flight` variable outside the function because `flightNum` is a copy of the primitive string value.
In the provided example, what happens when the `checkIn` function changes the `passenger` object?
-When the `checkIn` function changes the `passenger` object, it affects the original `Jonas` object outside the function because they both point to the same object in memory.
Can you explain the potential issue demonstrated by the `newPassport` function in the example?
-The `newPassport` function demonstrates a potential issue where multiple functions manipulate the same object. In this case, the `newPassport` function changes the passport number of the `Jonas` object, which then causes the `checkIn` function to fail because it expects the original passport number.
What is the purpose of using the `Math.random()` function in the `newPassport` function?
-The `Math.random()` function is used in the `newPassport` function to generate a random new passport number for the person object passed as an argument.
Why does the instructor recommend writing code during the lecture instead of providing boilerplate code?
-The instructor believes that writing code during the lecture forces the viewer to write the code themselves, which reinforces the learning process. Additionally, it makes the examples easier to understand when the code is written step-by-step.
What is the overall message or recommendation given by the instructor regarding passing arguments to functions in JavaScript?
-The overall message is to be aware of how arguments are passed to functions in JavaScript and to be careful when dealing with objects, as multiple functions manipulating the same object can create unexpected issues. Understanding this behavior is crucial, especially when working on large codebases with multiple developers.
Outlines
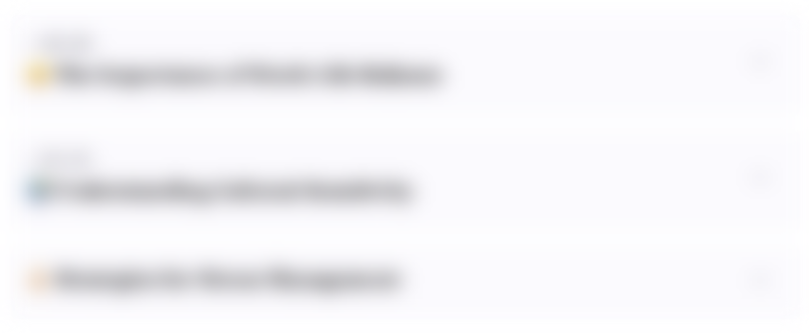
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
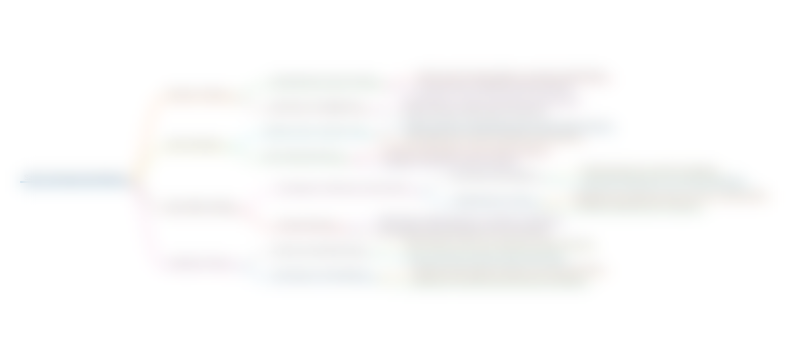
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
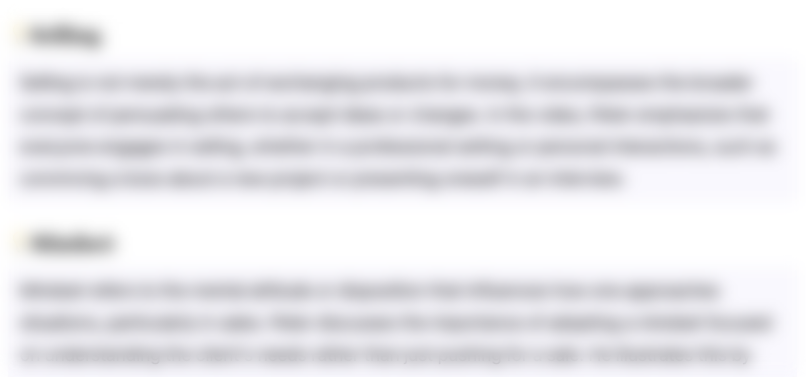
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
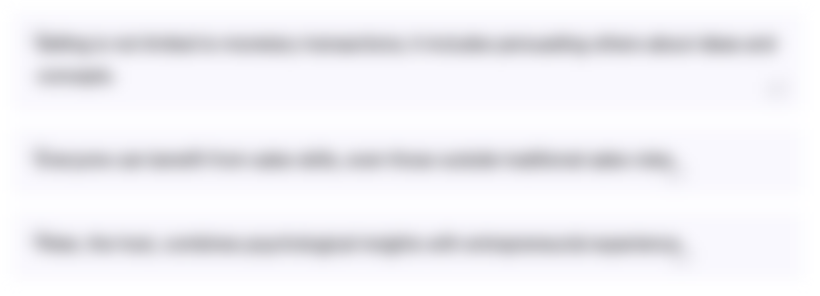
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
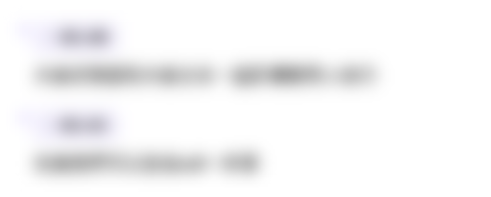
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

First-Class and Higher-Order Functions | JavaScript 🔥 | Lecture 121
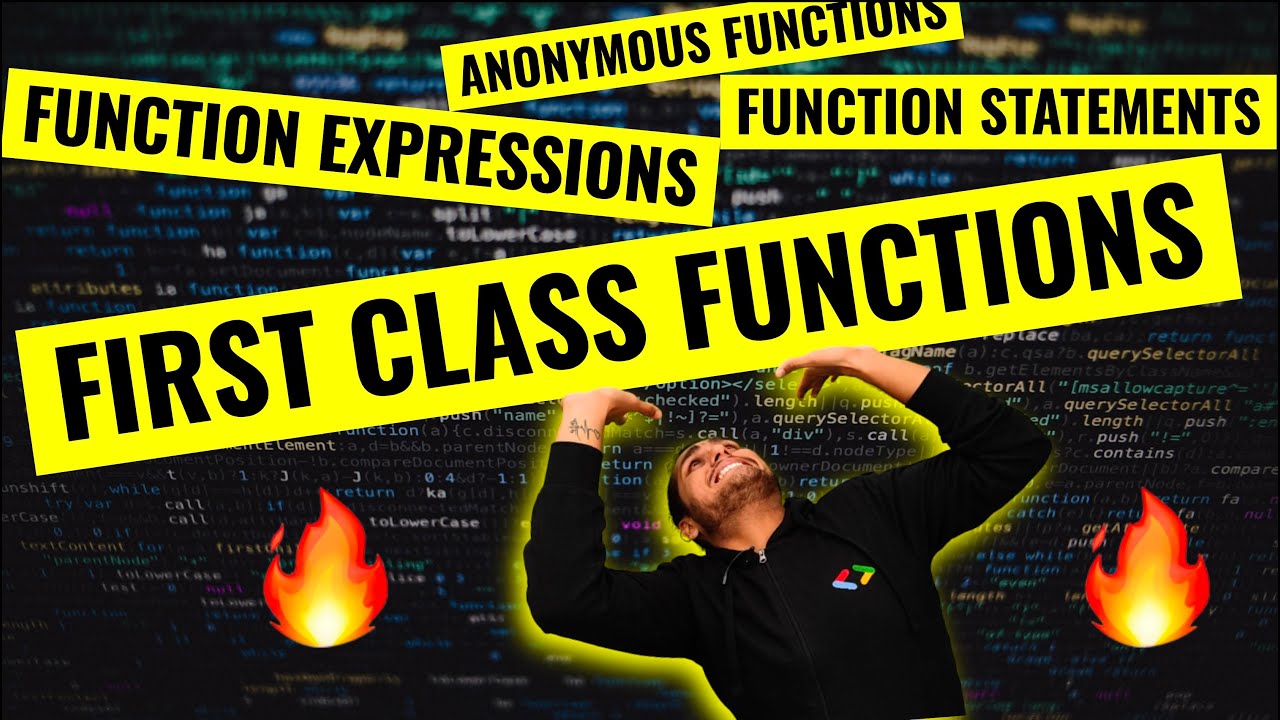
FIRST CLASS FUNCTIONS 🔥ft. Anonymous Functions | Namaste JavaScript Ep. 13

Closures | JavaScript 🔥 | Lecture 128

Functions in Python | Python for Beginners
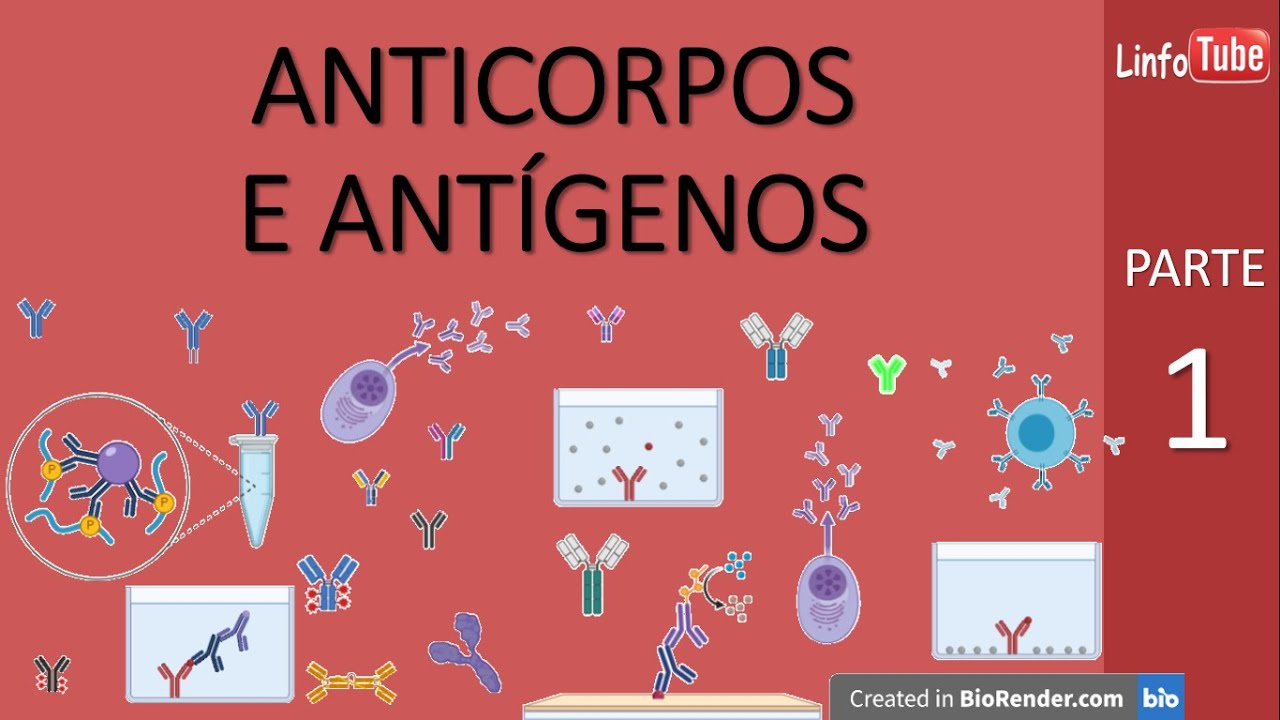
Anticorpos e Antígenos PARTE 1

How JavaScript Code is executed? ❤️& Call Stack | Namaste JavaScript Ep. 2
5.0 / 5 (0 votes)