Functions & Methods | Java Complete Placement Course | Lecture 7
Summary
TLDRThis lecture delves into the fundamental concept of functions in Java programming, essential across all programming languages. It starts by revisiting the basics covered in the initial lectures, emphasizing the universal programming fundamentals. The lecture explains functions as blocks of code designed to perform specific tasks, taking inputs, executing operations, and producing outputs, illustrated with relatable examples like thermometers and remote controls. It proceeds to detail Java function syntax, including defining return types and parameters, and explains function execution using practical examples. The session also touches on memory impact and the difference between functions and methods, enhancing understanding through practice problems, aiming to strengthen command over language-specific functions.
Takeaways
- 📚 The lecture is part of a placement course focused on Java programming fundamentals.
- 📲 Functions in Java are blocks of code designed to perform operations and return results based on inputs, similar to how physical devices like thermometers or remote controls function.
- 🔬 Syntax for writing functions in Java includes specifying the return type, function name, and arguments (if any), demonstrating how functions can be versatile in accepting various data types.
- 🚀 Functions can be 'void', meaning they do not return any value, which is common for functions performing tasks without needing to send data back.
- 👨💻 Real-world analogies such as thermometers for temperature measurement and volume buttons on phones help illustrate the concept of functions and their inputs and outputs.
- 📝 The lecture emphasizes the importance of understanding programming fundamentals, as they apply across different programming languages despite syntax changes.
- ✏️ The 'public static void main' method in Java, which is the entry point of any Java program, is highlighted as a special type of function.
- 💾 Examples and exercises provided help in reinforcing the concept of functions, including operations like summing numbers or calculating factorials.
- ⬇️ Special attention is given to the syntax and structure of functions, including return types, argument passing, and the significance of 'void' for non-returning functions.
- ⚠️ The script touches on the differences between functions and methods in Java, noting that methods are called on objects or classes while functions can be invoked directly.
Q & A
What is the purpose of functions in Java?
-Functions in Java serve as blocks of code designed to perform specific operations, take inputs, and return outputs after executing the operations.
How does the syntax of defining a function in Java look?
-The syntax for defining a function in Java starts with specifying the return type, followed by the function name, and then the parameters (arguments) inside parentheses.
What is the significance of the return type in a Java function?
-The return type in a Java function specifies the type of value that the function returns after execution. It can be int, float, string, or other data types.
Can a Java function return multiple types of values?
-No, a Java function can only return a single type of value, as specified by its return type. However, it can return complex objects that might encapsulate multiple values.
What is the 'void' keyword used for in Java functions?
-The 'void' keyword is used to declare functions that do not return any value after execution.
How are functions called or executed in Java?
-Functions in Java are called or executed by using their name followed by parentheses, which may include arguments if the function requires input.
What role do parameters (arguments) play in Java functions?
-Parameters or arguments in Java functions allow the passing of values to the function, enabling it to perform operations based on those inputs.
What is the difference between methods and functions in the context of Java?
-In Java, methods are functions that are associated with objects and are called using object references, whereas standalone functions are not directly associated with objects.
How does Java handle functions that do not explicitly return a value?
-Functions that do not explicitly return a value must be declared with a 'void' return type, indicating they perform actions without returning any data.
What is an example of a simple Java function and its purpose?
-An example of a simple Java function could be a 'calculateSum' function that takes two integers as input and returns their sum. Its purpose is to perform the addition operation and return the result.
Outlines
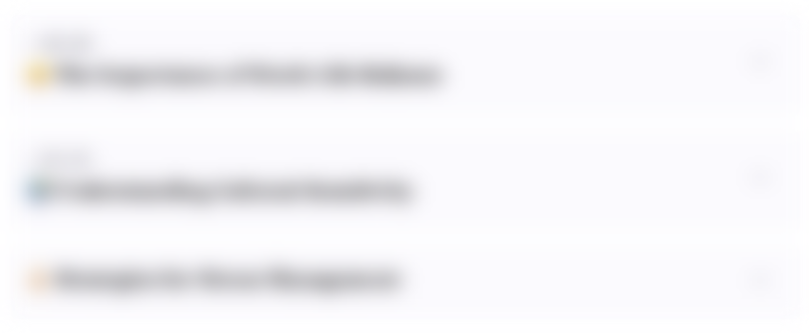
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
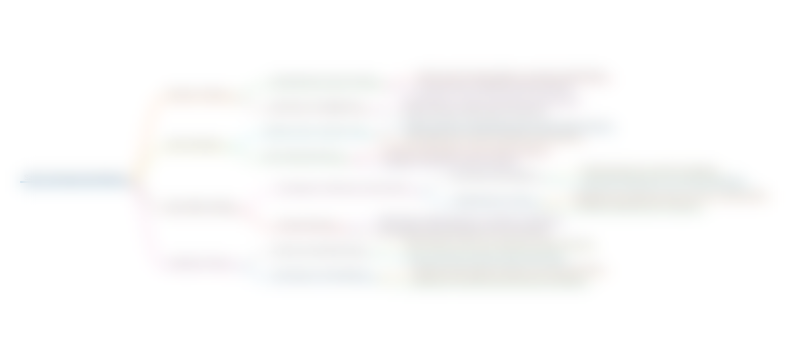
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
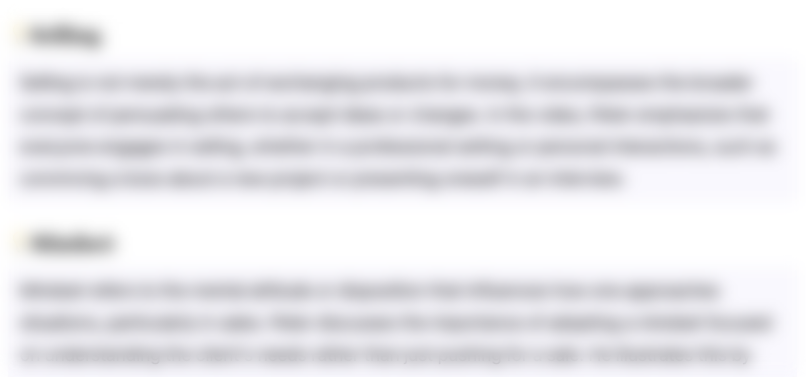
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
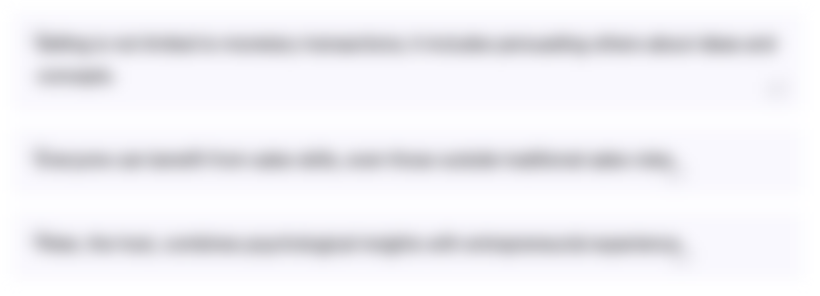
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
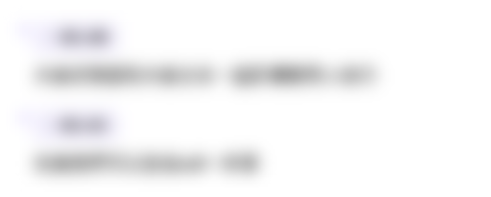
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)