PHP Variable Storage & Object Comparison - Zend Value (zval) - Full PHP 8 Tutorial
Summary
TLDRThis video script explores object comparison in PHP, explaining the use of double and triple equal signs for equality checks. It clarifies that the double equals checks for property value equality within the same class, while triple equals verifies if two variables reference the same object instance. The script also delves into PHP's memory management, explaining how variables and objects are stored, and the implications of variable assignment on object references. It concludes with a discussion on recursive comparisons and the potential for circular references to cause errors, providing a comprehensive guide for PHP developers.
Takeaways
- π PHP uses the double equal sign (==) for a loose comparison of object properties, which can result in unexpected outcomes due to type juggling.
- π The triple equal sign (===) in PHP is used for a strict identity check, ensuring both the object and its properties are identical.
- π PHP's comparison operators check if objects are instances of the same class and have the same property values for the double equal sign, but only if they are the exact same instance for the triple equal sign.
- π When assigning one object to another, PHP copies the reference, not the object itself, which means changes to one object's properties will reflect in the other.
- π‘ PHP stores variable names in a symbols table, separate from their values, which are stored in a zval container.
- π¦ For objects, the zval container holds an object identifier pointing to the actual object stored elsewhere in memory.
- π Assigning an object to another variable creates a new variable pointing to the same zval container, which in turn points to the same object in memory.
- π Changing a property of an object affects all variables pointing to that object, demonstrating PHP's pass-by-value behavior for objects.
- π Extending a class does not affect object identity; an object of a subclass is not considered equal to an object of the parent class for identity checks.
- π Recursive property comparison in PHP can lead to issues like fatal errors when dealing with circular references.
- π PHP allows the use of greater or less than signs to compare objects, which stops at the first property that fails the check.
Q & A
How does PHP determine if two objects are equal using the double equal sign (==)?
-In PHP, the double equal sign (==) checks for equality by comparing if the two objects are instances of the same class and have the same property values. It performs a loose comparison, which can result in unexpected outcomes when types do not match strictly.
What does the triple equal sign (===) operator do when comparing two objects in PHP?
-The triple equal sign (===) is the identity operator in PHP. It checks if two objects are not only equal in value but also refer to the exact same instance in memory.
Why would a comparison using the double equal sign (==) fail even if two objects have the same property values?
-A comparison using the double equal sign (==) can fail if the objects are not of the same class instance, even if their property values are identical.
What is the difference between an object comparison using the double equal sign and the triple equal sign in PHP?
-The double equal sign checks for value equality and type compatibility, while the triple equal sign checks for both value equality and identity, ensuring the two variables point to the exact same object in memory.
How does PHP handle type casting during a loose comparison with the double equal sign?
-PHP performs type juggling during a loose comparison, attempting to match different data types. For example, it might consider the integer 1 and the boolean true to be equal.
What happens when you assign one object to another in PHP, like assigning invoice1 to invoice3?
-When you assign one object to another in PHP, you are not duplicating the object; instead, you are creating a new reference that points to the same object in memory. Changes to the object through either reference will be reflected in all references.
Can you explain the concept of 'copy on write' in the context of PHP objects?
-Copy on write is a technique where a copy of an object is only created when it is modified. In PHP, when you assign an object to another variable, you are not creating a copy of the object; you are copying the reference to the same object. Modifications will affect all references to that object.
What is a 'zval' in PHP, and how does it relate to object storage?
-A 'zval' is a data structure in PHP used to store information about a variable, including its type and value. For objects, the zval contains an object identifier that points to the actual object stored in a separate data structure.
Why might recursive comparison of objects in PHP cause fatal errors?
-Recursive comparison can cause fatal errors if there is a circular reference between objects, leading to an infinite loop during the comparison process.
How can you compare objects using greater or less than signs in PHP?
-In PHP, you can use greater or less than signs to compare objects by their property values. The comparison stops and returns false at the first property that does not match.
What is the role of the 'symbols table' in PHP variable storage?
-The 'symbols table' in PHP is a data structure that stores variable names separately from their values. Each entry in the symbols table points to a zval container, which holds the actual value of the variable, including objects.
Outlines
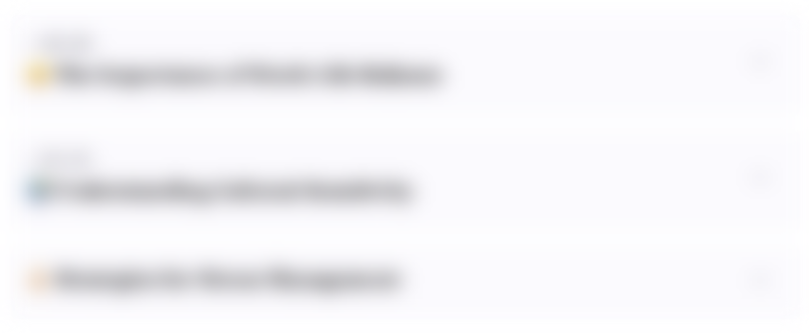
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
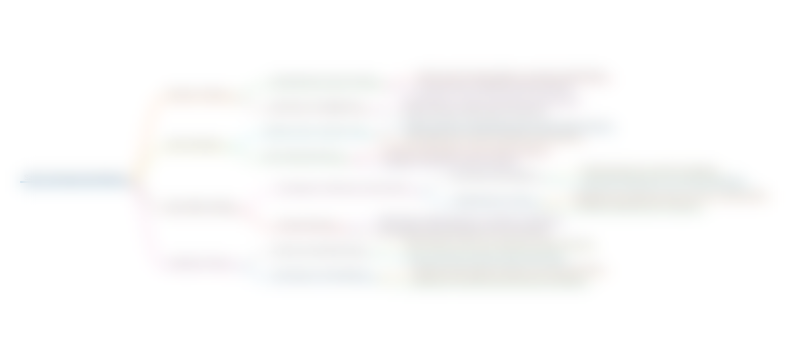
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
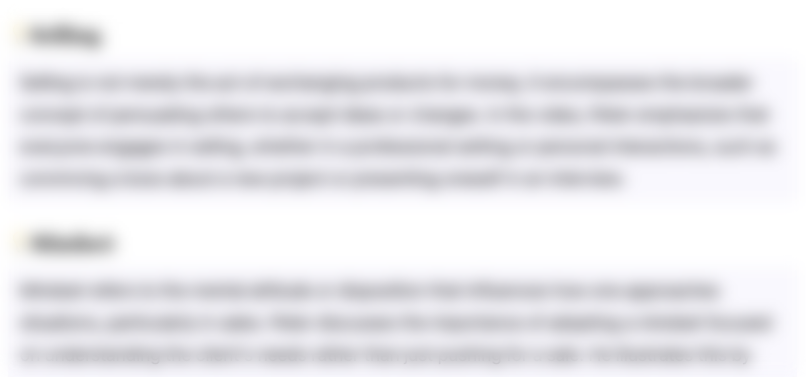
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
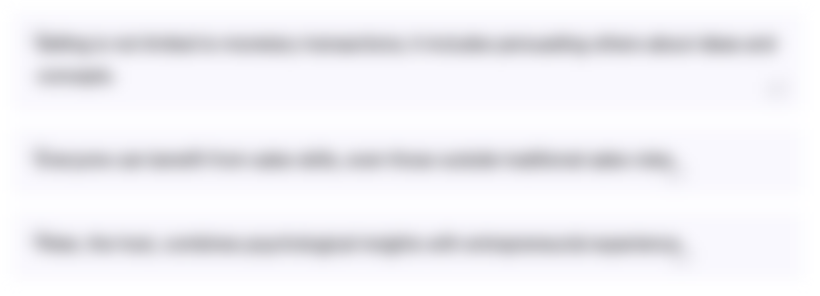
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
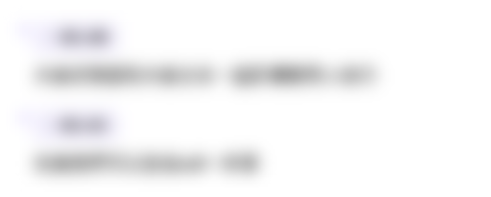
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
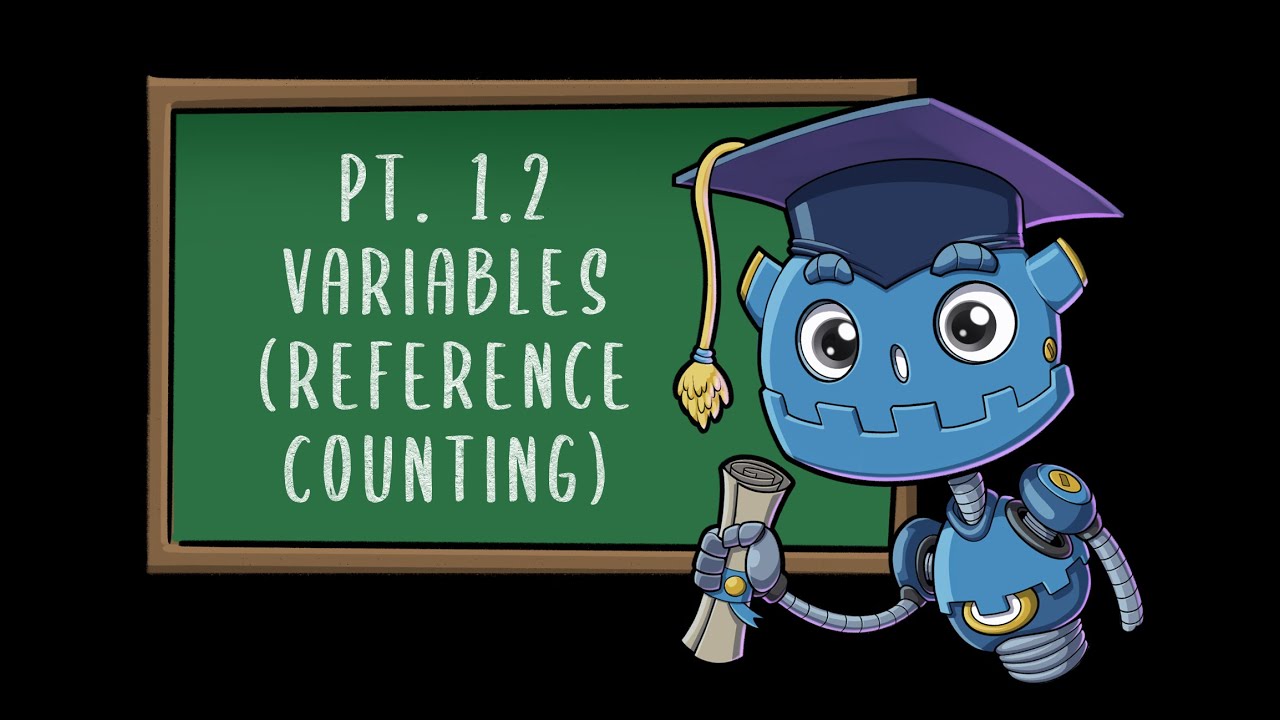
Life Cycle & Reference Counting | Godot GDScript Tutorial | Ep 1.2
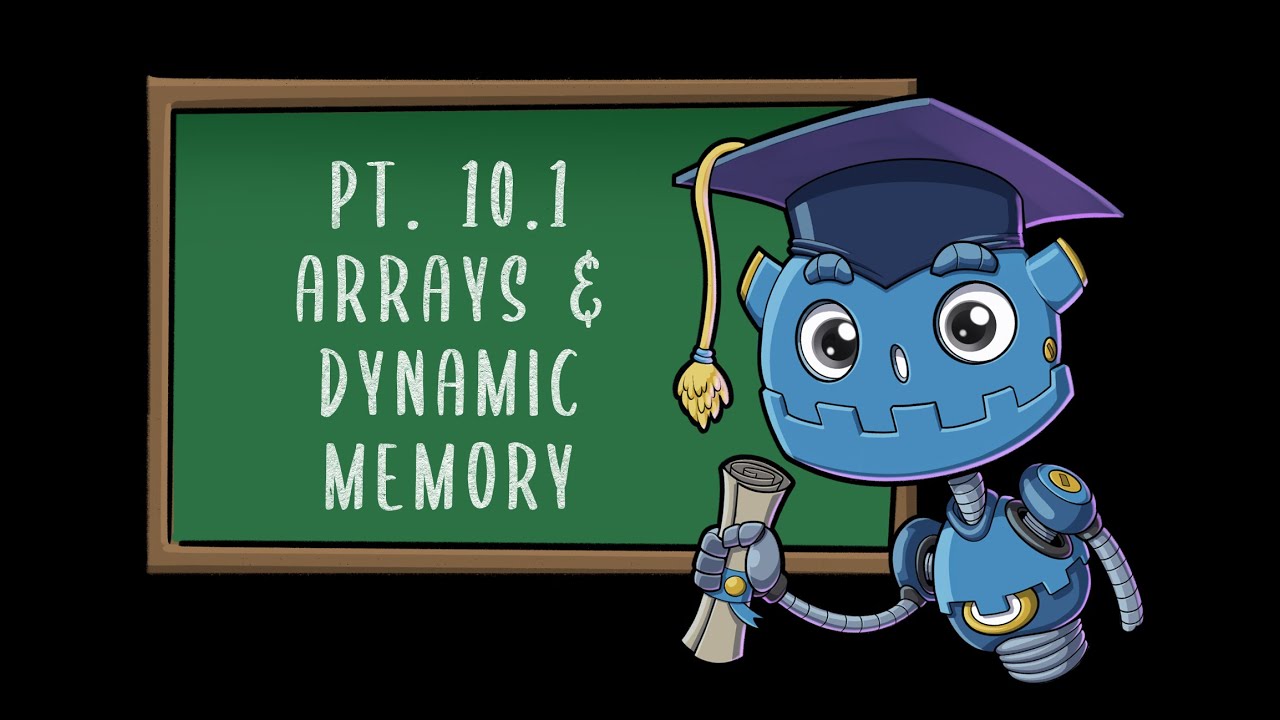
Arrays & Dynamic Memory Address | Godot GDScript Tutorial | Ep 10.1

Class Objects & Constructors | Godot GDScript Tutorial | Ep 16
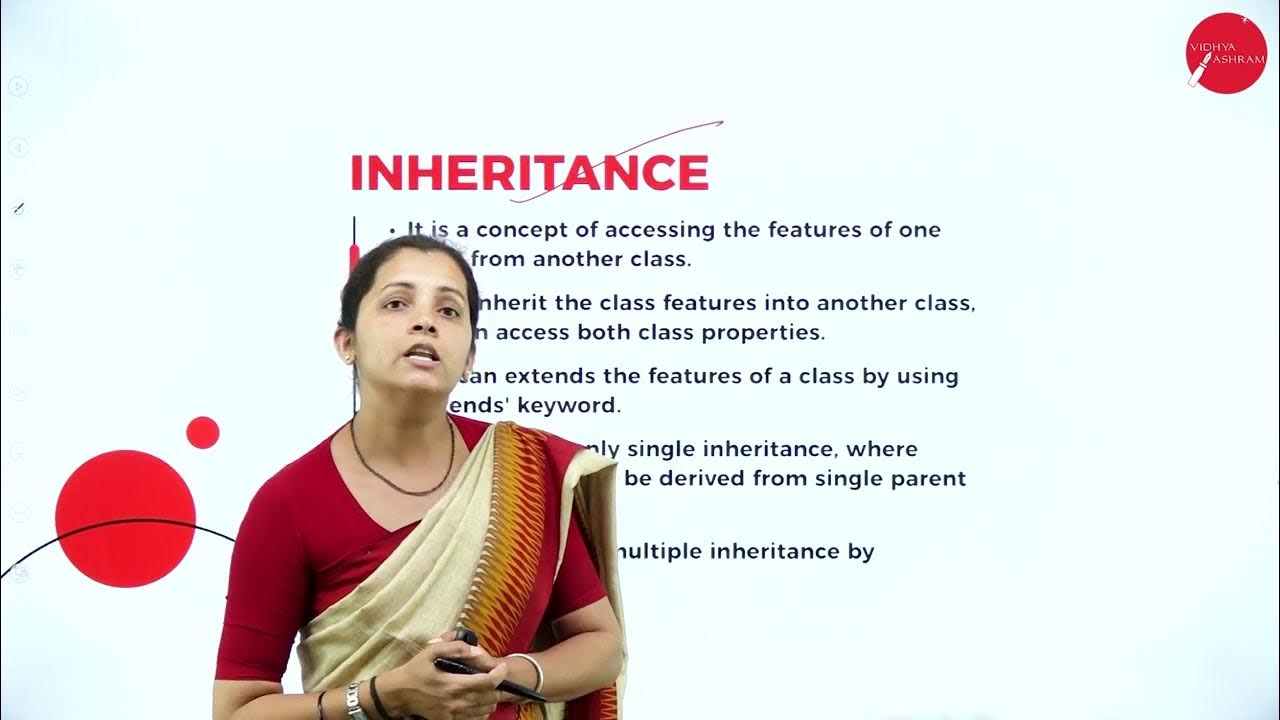
DAY 07 | PHP AND MYSQL | VI SEM | B.CA | CLASS AND OBJECTS IN PHP | L1
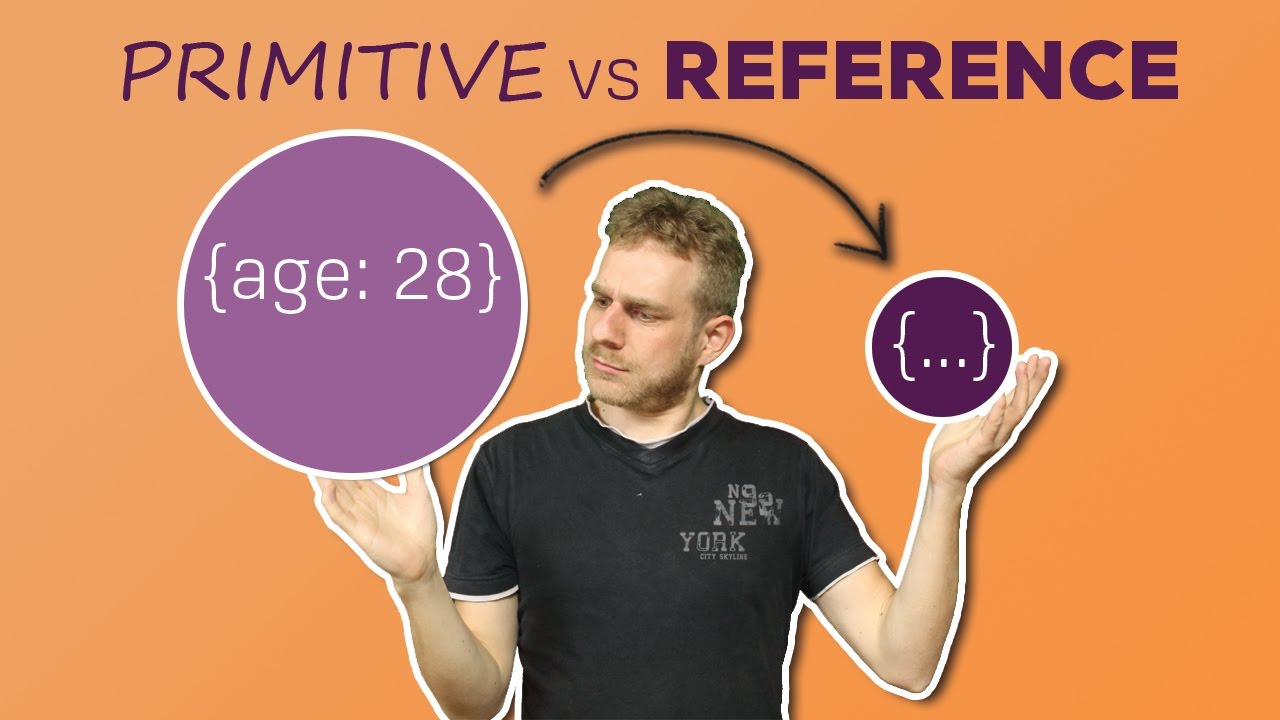
JavaScript - Reference vs Primitive Values/ Types
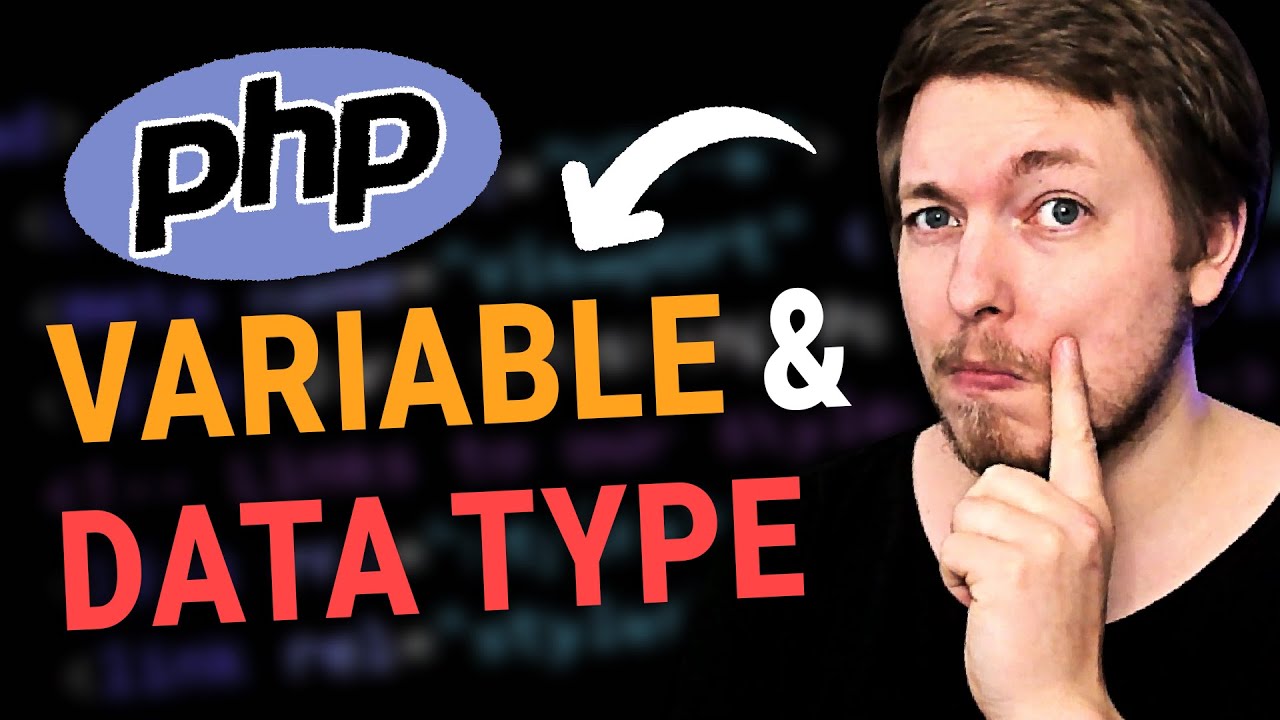
4 | PHP Variable and Data Type Tutorial | 2023 | Learn PHP Full Course for Beginners
5.0 / 5 (0 votes)