First-Class and Higher-Order Functions | JavaScript π₯ | Lecture 121
Summary
TLDRThis video script delves into the powerful concept of first-class functions in JavaScript, a fundamental feature that treats functions as values, enabling higher-order functions. It explores how functions can be stored, passed as arguments, and returned from other functions, showcasing practical examples. The script distinguishes between first-class functions, a language feature, and higher-order functions, which receive or return functions as arguments. By mastering these concepts, developers can unlock advanced JavaScript techniques and write more flexible, modular code.
Takeaways
- π» JavaScript has first-class functions, meaning functions are treated as values and can be manipulated similarly to objects.
- π§ First-class functions allow JavaScript to support higher order functions, which can either accept functions as arguments or return functions.
- π Functions in JavaScript can be stored in variables or object properties, showcasing their versatility as first-class citizens.
- βοΈ Passing functions as arguments to other functions is a common practice in JavaScript, utilized in scenarios like adding event listeners.
- βͺ Returning a function from another function is possible in JavaScript, opening up advanced programming patterns and techniques.
- π¨βπ» Functions in JavaScript are objects, and they can have methods called on them, such as the bind method.
- π‘ Higher order functions are crucial for JavaScript's flexibility, allowing for functions that operate on or return other functions.
- π’ The 'add event listener' function is an example of a higher order function because it takes another function as an input.
- β Callback functions are used in higher order functions and are executed later, based on certain events or conditions.
- β First-class functions and higher order functions are distinct concepts; the former refers to the treatment of functions as values, while the latter involves functions that operate on or return other functions.
- π Understanding the difference between first-class functions and higher order functions is essential for mastering JavaScript.
Q & A
What is the fundamental property of JavaScript language discussed in the script?
-The fundamental property discussed is that JavaScript has first-class functions, meaning that functions are treated as values, similar to any other data type.
What are some examples of how functions can be treated as values in JavaScript?
-Functions can be stored in variables, object properties, passed as arguments to other functions, and returned from other functions.
Why is the ability to pass functions as arguments to other functions useful?
-Passing functions as arguments to other functions enables the use of callback functions, which can be called later by the higher-order function when specific conditions are met, such as an event occurring.
What is a higher-order function?
-A higher-order function is either a function that receives another function as an argument (like the addEventListener function), or a function that returns a new function.
What is the difference between first-class functions and higher-order functions?
-First-class functions is a language feature that allows functions to be treated as values, while higher-order functions are specific functions that either take functions as arguments or return new functions, made possible by the first-class functions feature.
What is a callback function?
-A callback function is a function that is passed as an argument to another function (a higher-order function), and it will be called later by the higher-order function when specific conditions are met.
Why are functions treated as objects in JavaScript?
-Functions are treated as objects in JavaScript because they are a type of object, and objects in JavaScript can have properties and methods, including functions themselves.
Can you provide an example of a function method in JavaScript?
-One example of a function method in JavaScript is the bind method, which is mentioned in the script and will be covered in more detail later.
What is the purpose of higher-order functions in JavaScript?
-Higher-order functions enable more flexible and powerful programming patterns, allowing functions to be passed around and manipulated like any other data type, facilitating code reusability and abstraction.
Why is it important to understand the concept of first-class functions and higher-order functions in JavaScript?
-Understanding these concepts is crucial for mastering JavaScript and writing more advanced, modular, and reusable code. It also helps in comprehending various programming patterns and techniques used in modern JavaScript development.
Outlines
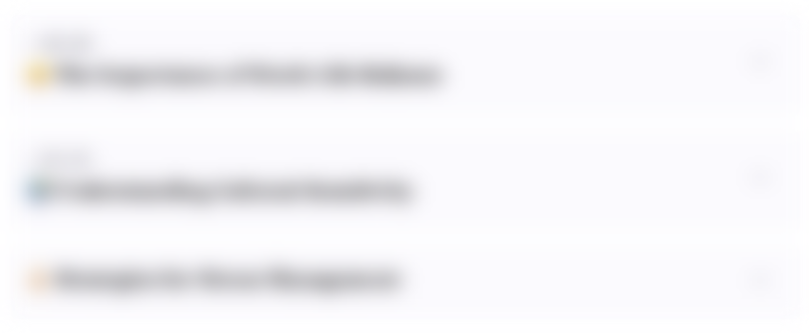
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
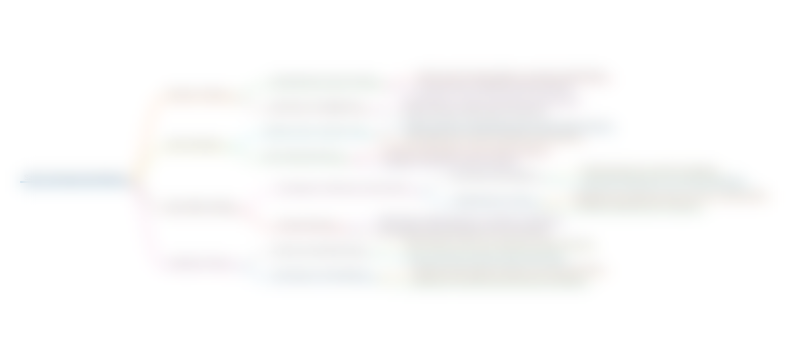
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
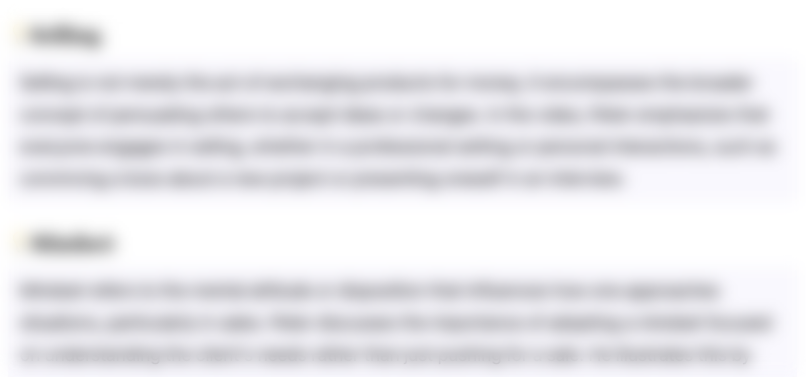
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
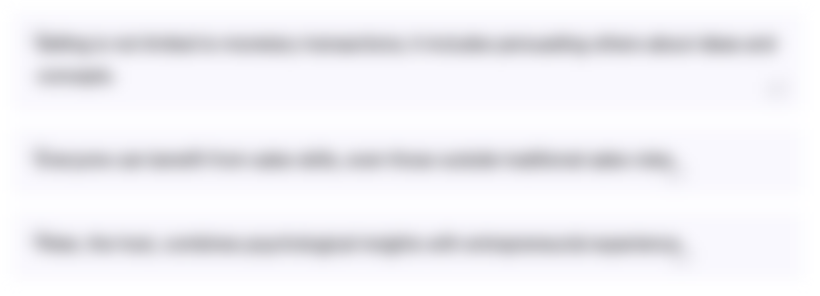
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
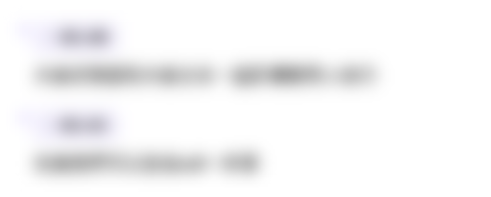
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
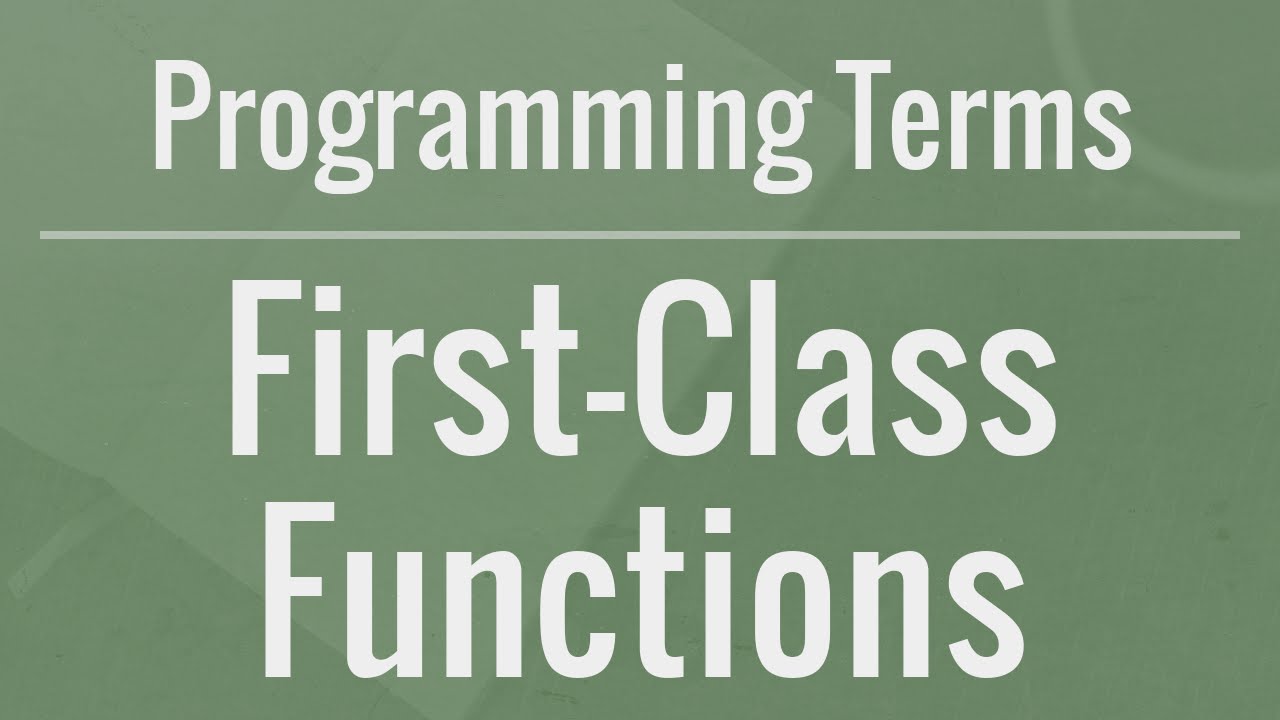
Programming Terms: First-Class Functions

Higher Order Function | javascript interview series

Higher-Order Functions ft. Functional Programming | Namaste JavaScript Ep. 18

How JavaScript Code is executed? β€οΈ& Call Stack | Namaste JavaScript Ep. 2
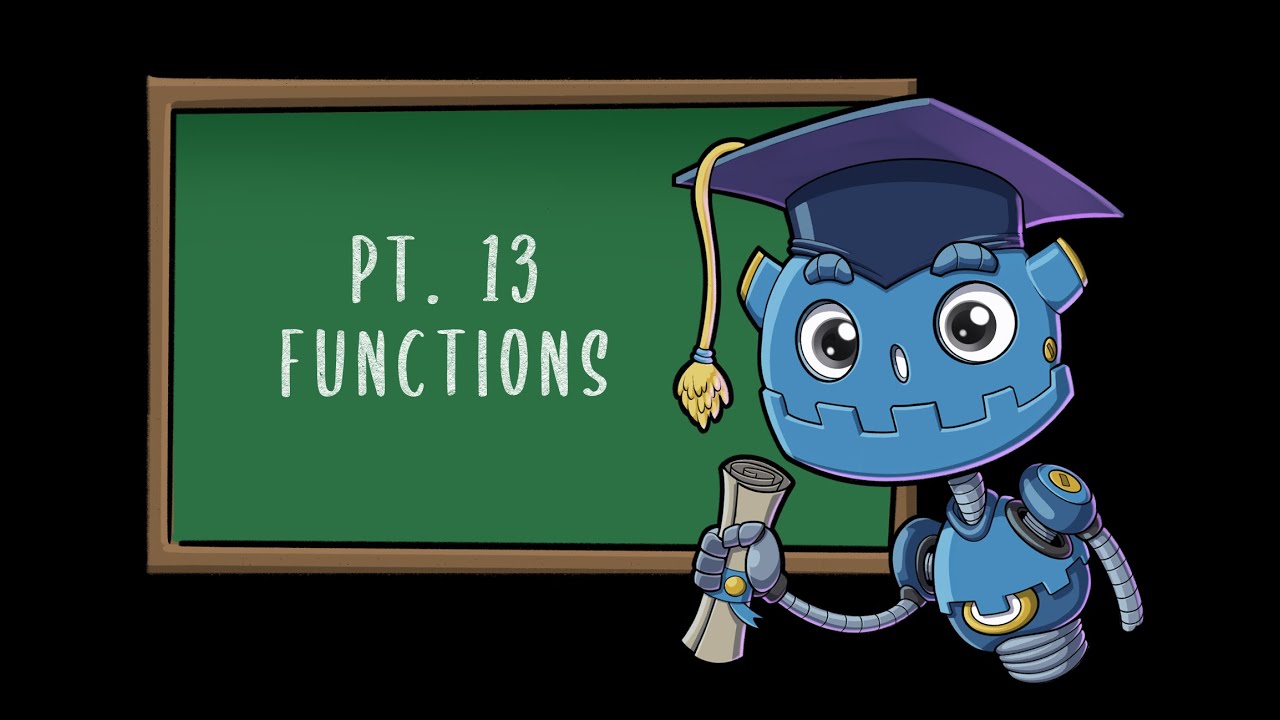
Functions | Godot GDScript Tutorial | Ep 13
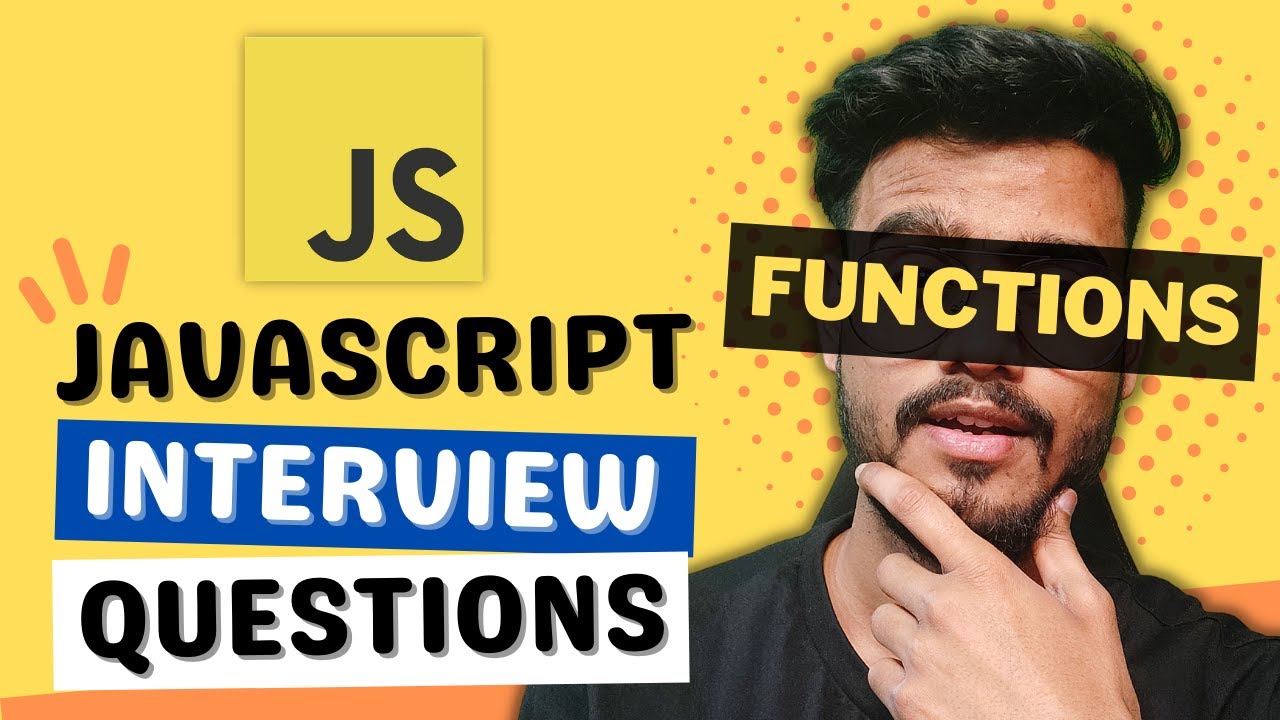
Javascript Interview Questions ( Functions ) - Hoisting, Scope, Callback, Arrow Functions etc
5.0 / 5 (0 votes)