Group Anagrams - Categorize Strings by Count - Leetcode 49
Summary
TLDRIn this coding tutorial, the presenter introduces a method to group anagrams from a list of strings efficiently. By recognizing that anagrams can be identified by sorting or by counting character occurrences, the video suggests using a hash map with character counts as keys for better performance. The presenter explains the process of counting characters, using ASCII values to map characters to indices, and then using these counts to group anagrams. The solution is optimized to O(m*n) time complexity, where m is the number of strings and n is their average length, making it an efficient approach to solving the problem.
Takeaways
- đ The video discusses a coding challenge to group anagrams from a list of strings.
- đ An anagram is a word or phrase formed by rearranging the letters of another, such as 'tan' and 'nat'.
- đ To identify anagrams, the video suggests sorting the strings and comparing them for equality.
- â± The naive approach of sorting each string has a time complexity of O(n log n), where n is the average string length.
- đ A more efficient method is introduced, utilizing a hash map to group anagrams with a time complexity of O(m * n).
- đ The hash map uses a count array to tally the occurrence of each character from 'a' to 'z' in the strings.
- đ The count array is transformed into a tuple to serve as a hash map key, as lists are mutable and not hashable in Python.
- đą The ASCII values of characters are used to map them to indices in the count array, subtracting the ASCII value of 'a'.
- đ For each string, the character counts are incremented in the count array, which then becomes a key in the hash map.
- đŠ The strings with the same character counts are grouped together in the hash map under the same key.
- đ The final output is a list of lists, where each sub-list contains anagrams grouped by their character counts.
Q & A
What is the main topic of the video script?
-The main topic of the video script is about writing code to group anagrams together from a list of strings.
What are anagrams and how can they be identified?
-Anagrams are words or phrases that contain the same characters but in a different order. They can be identified by sorting the characters of each string; if the sorted strings are equal, they are anagrams.
Why is sorting each string not the most efficient way to group anagrams?
-Sorting each string has a time complexity of O(n log n) for each string, where n is the length of the string. This becomes inefficient when you have to sort m strings, resulting in a time complexity of O(m * n log n).
What alternative method is suggested in the script to group anagrams?
-The script suggests using a hash map (or dictionary) to count the frequency of each character in the strings and then group strings with the same character count together.
How does the character counting method work in terms of time complexity?
-The character counting method has a time complexity of O(m * n), where m is the number of strings and n is the average length of the strings, making it more efficient than sorting each string.
What is the maximum number of unique characters considered in the script?
-The script considers a maximum of 26 unique characters, which corresponds to the lowercase letters of the English alphabet from 'a' to 'z'.
How is the 'count' array used in the hash map?
-The 'count' array is used as a key in the hash map, where each index represents the count of a specific character from 'a' to 'z'. The value associated with each key is a list of strings that have the same character count pattern.
Why are lists changed to tuples when used as keys in the hash map?
-Lists are mutable and cannot be used as keys in a hash map. Tuples, on the other hand, are immutable and can be used as keys.
What data structure is used to avoid dealing with edge cases when a count does not exist in the hash map?
-A default dictionary (or default hash map) is used, where the default value for a new key is a list, thus avoiding the need to check if the key exists.
How is the final result of the anagram grouping presented?
-The final result is presented by returning the values of the hash map, which are the lists of anagrams grouped together based on their character counts.
What is the significance of using a hash map for this problem?
-Using a hash map allows for efficient grouping of anagrams by leveraging the constant time complexity for lookups, insertions, and updates, making it an optimal solution for this problem.
Outlines
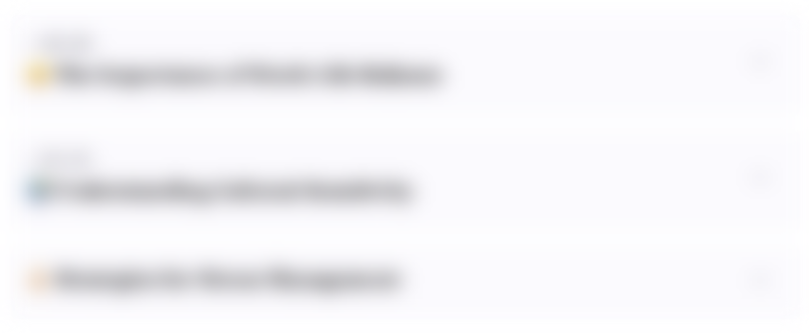
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
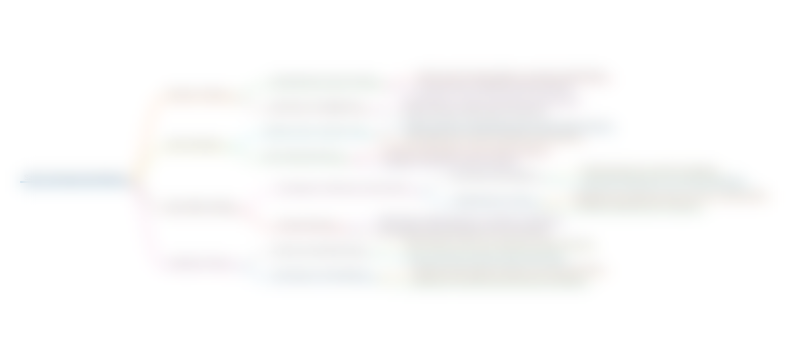
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
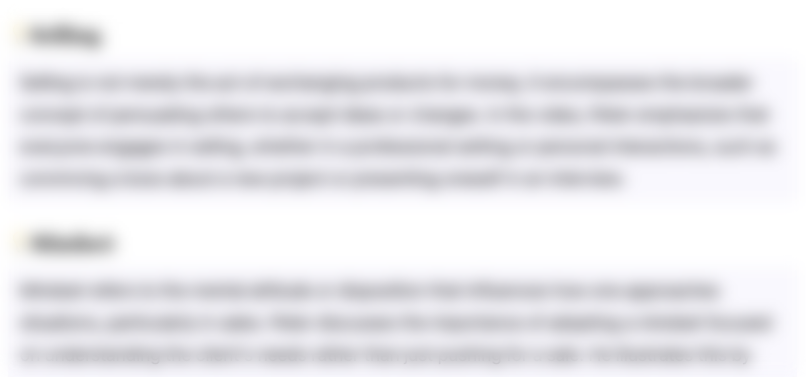
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
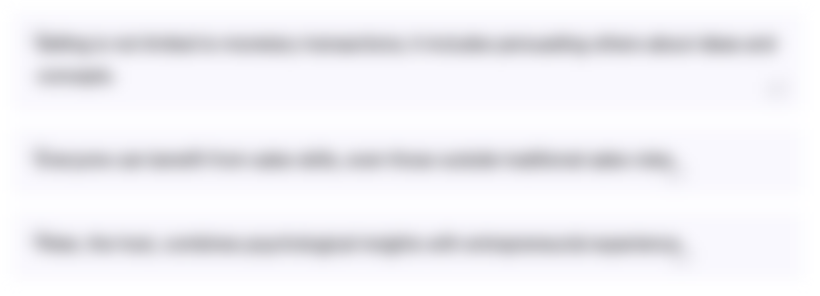
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
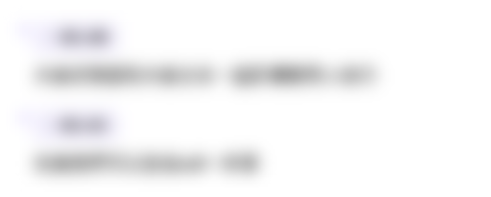
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantVoir Plus de Vidéos Connexes

Two Sum - Leetcode 1 - HashMap - Python
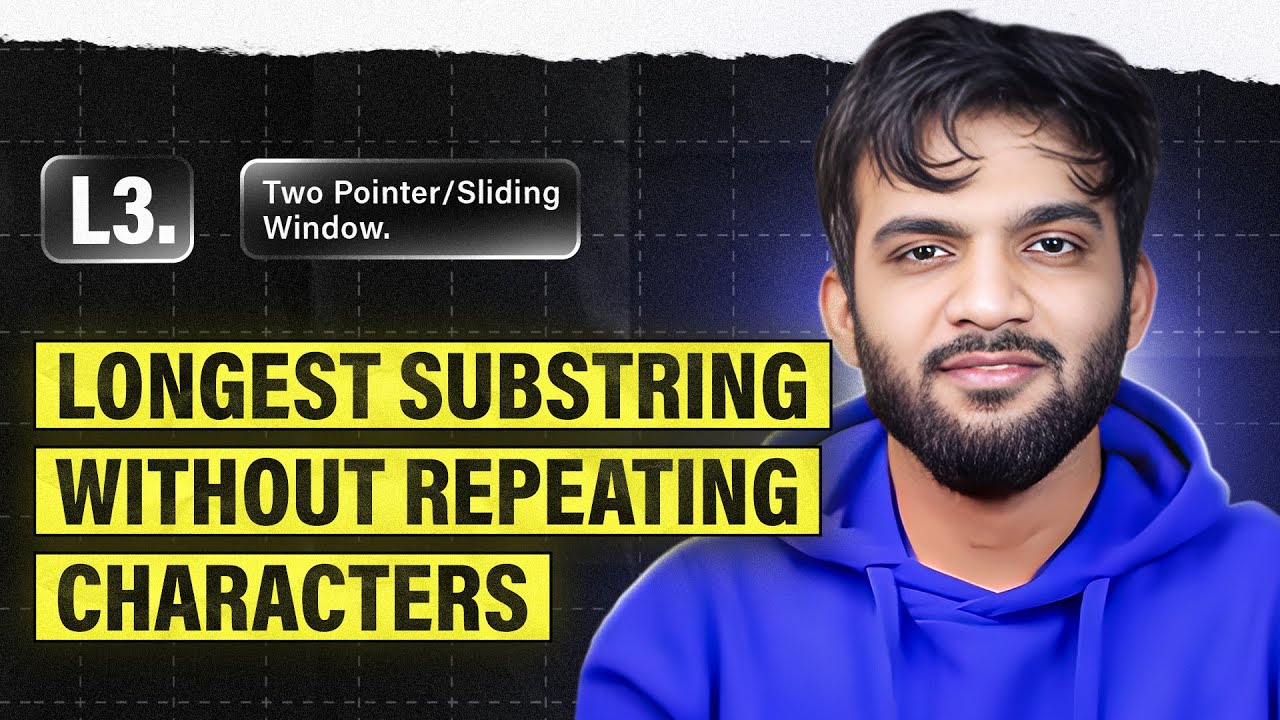
L3. Longest Substring Without Repeating Characters | 2 Pointers and Sliding Window Playlist
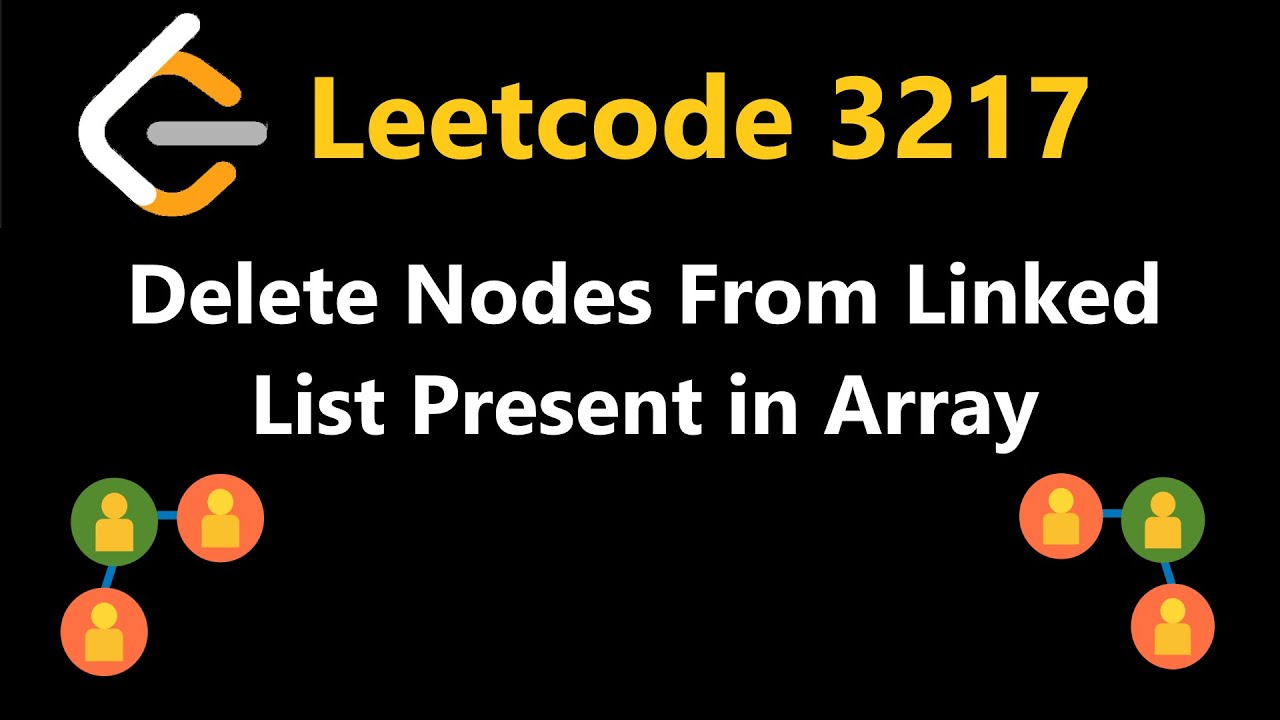
Delete Nodes From Linked List Present in Array - Leetcode 3217 - Python
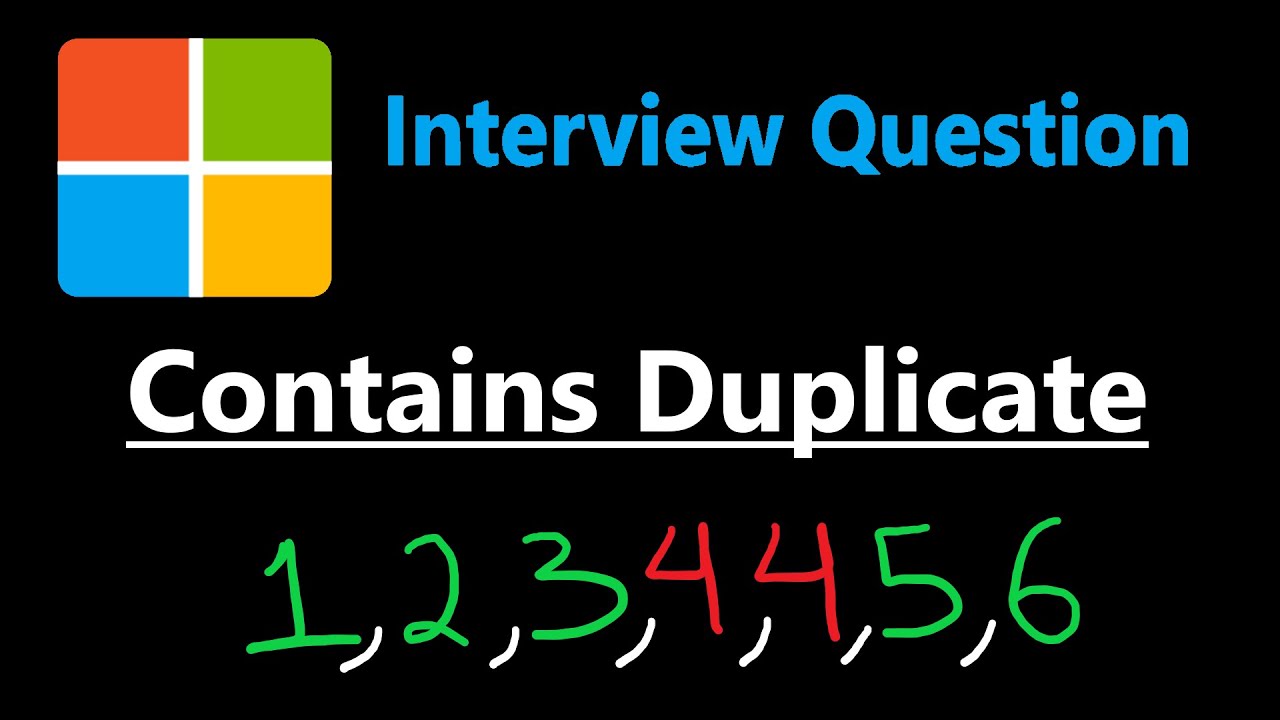
Contains Duplicate - Leetcode 217 - Python
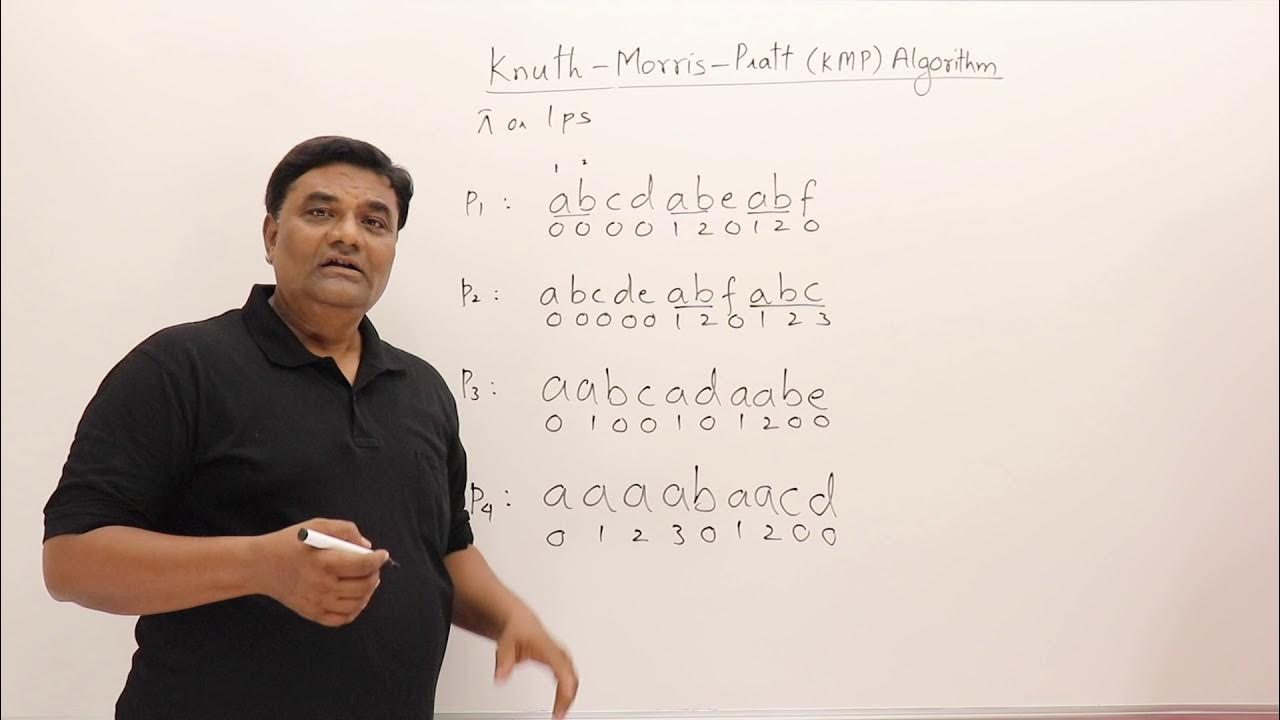
9.1 Knuth-Morris-Pratt KMP String Matching Algorithm
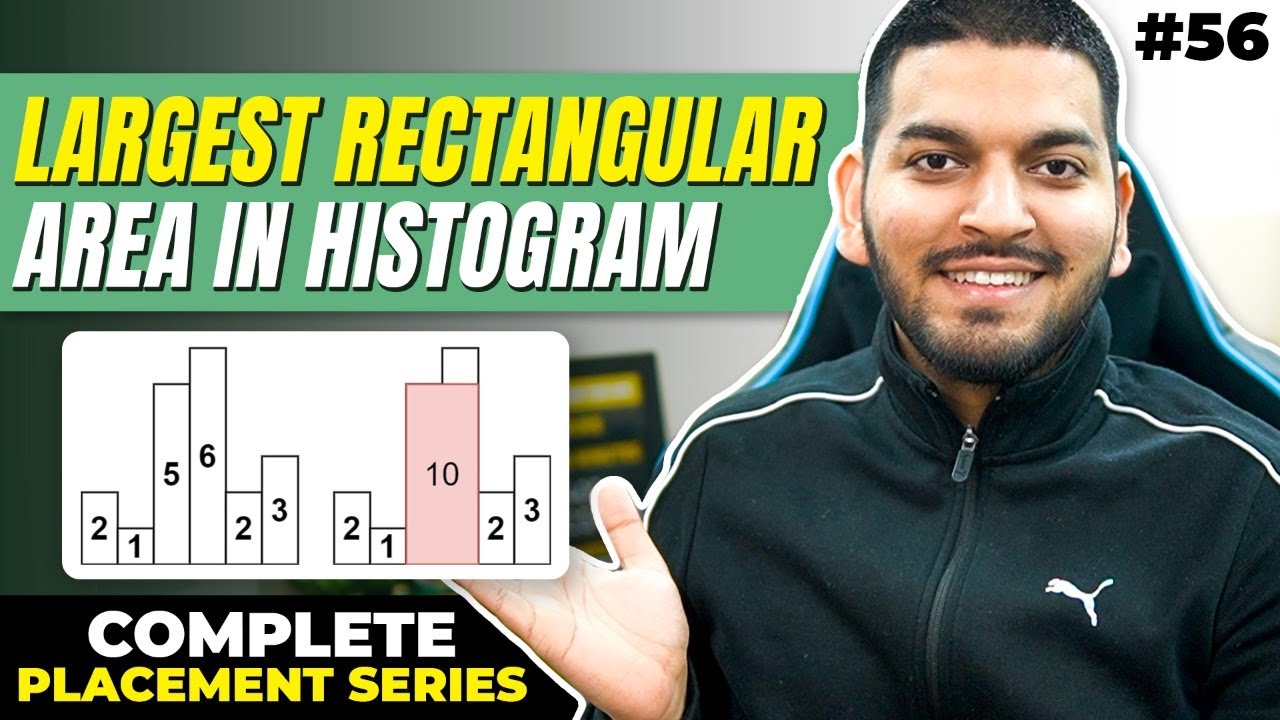
Lecture 56: Largest Rectangular Area in Histogram [Optimised Approach]
5.0 / 5 (0 votes)