How to render LISTS in React π
Summary
TLDRThis comprehensive tutorial delves into rendering lists in ReactJS, guiding viewers through the complexities of list rendering step by step. It begins by creating a functional component to manage a list, illustrating how to convert an array of strings into list items using JavaScript's map method. The video then explores sorting and filtering arrays, emphasizing the importance of unique keys for list items. It transitions to rendering arrays of objects, showcasing how to display additional properties like calories alongside fruit names. The tutorial also covers making the list component reusable by passing props, and concludes with practical tips on conditional rendering and setting default props and prop types for robust component design.
Takeaways
- π The tutorial focuses on rendering lists in ReactJS, which can be complex but is broken down step by step.
- π A new functional component named 'List' is created to manage the list, demonstrating basic component setup in React.
- π An array of fruits is used to illustrate rendering arrays, highlighting the conversion of array elements into list items.
- π The importance of using the 'map' method to transform arrays into list elements is emphasized for dynamic list rendering.
- π React's requirement for a unique 'key' prop for list items is discussed, ensuring React can efficiently update the DOM.
- π The tutorial covers sorting arrays with the 'sort' method, including custom sorting functions for alphanumeric order.
- π·οΈ The use of 'filter' method is explained to demonstrate how to display items based on certain criteria, like calorie count.
- π The process of making the 'List' component reusable by passing different lists as props is detailed, enhancing component flexibility.
- π¨ Basic CSS styling is applied to the list components to improve the visual presentation of the rendered lists.
- βοΈ Conditional rendering is introduced to handle cases where lists might be empty, ensuring the UI adapts to data changes.
- π οΈ The tutorial concludes with setting up default props and prop types for the 'List' component to ensure robustness and error checking.
Q & A
What is the main topic of the video?
-The main topic of the video is rendering lists in ReactJS, covering various methods and best practices for handling and displaying lists of data.
How does the tutorial start in terms of creating a list component?
-The tutorial starts by creating a new function-based component named 'list' in a JSX file within the source folder.
What is the initial data structure used in the tutorial for the list?
-Initially, an array of fruit names is used as the data structure for the list.
How is the array of fruits transformed into list items in the component?
-The array of fruits is transformed into list items using the built-in JavaScript 'map' method, which returns a new array of list item elements.
Why is it necessary to use a 'key' prop when rendering lists in React?
-It is necessary to use a 'key' prop to give each list item a unique identifier, which helps React efficiently update and re-render the list when items are added, removed, or reordered.
How can you sort the list of fruits in the tutorial?
-The list of fruits can be sorted using the 'sort' method, which can be applied directly to the array. The tutorial demonstrates sorting both lexicographically by name and numerically by calories.
What is the purpose of converting the array of strings into an array of objects?
-Converting the array of strings into an array of objects allows for more complex data structures, enabling the inclusion of additional properties such as 'calories' and makes it easier to manage and display the data in the list.
How does the tutorial handle empty lists or missing data?
-The tutorial uses conditional rendering to check if the list has elements before rendering it. If the list is empty or data is missing, it returns null or a default value to ensure the component doesn't try to render undefined data.
What is the benefit of making the list component reusable?
-Making the list component reusable allows it to accept different types of lists as props, increasing its flexibility and reducing code duplication across the application.
How does the tutorial enhance the list component with CSS styling?
-The tutorial enhances the list component with CSS styling by adding class names and defining styles for list categories and items, including hover effects, to improve the visual presentation of the list.
Why is it recommended to set up prop types in React components?
-Setting up prop types is recommended for validating the data types of props passed to a component. It helps catch errors and provides warnings during development, ensuring that components receive the expected data types and improving code reliability.
Outlines
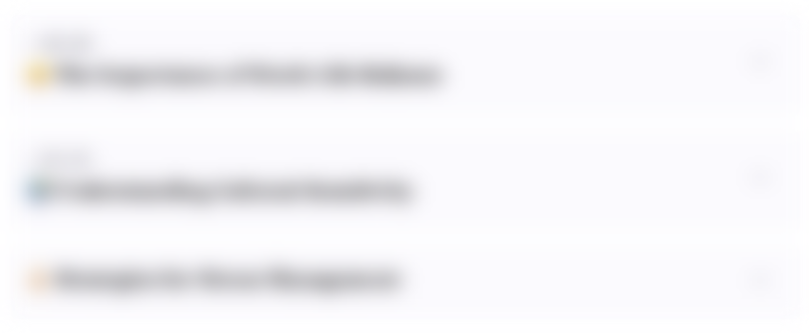
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
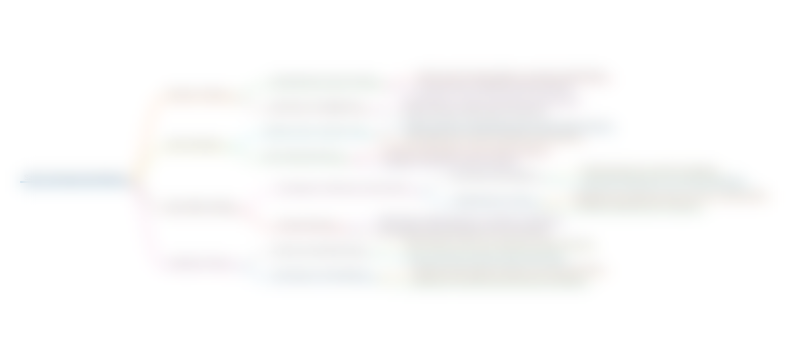
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
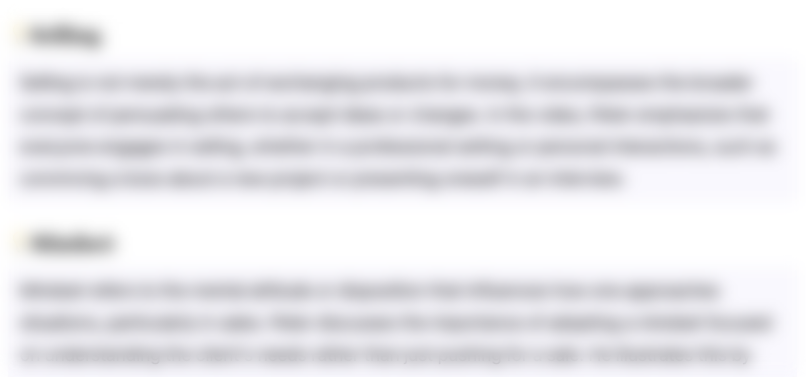
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
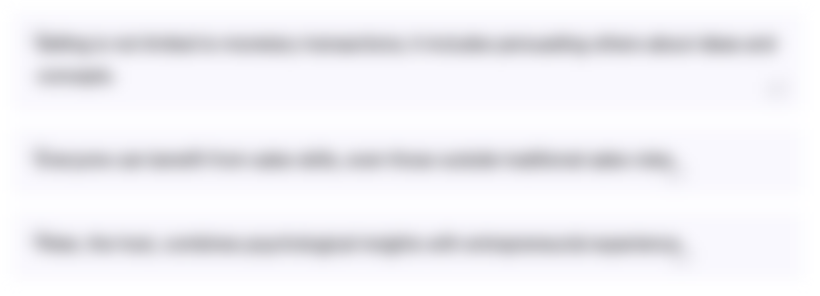
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
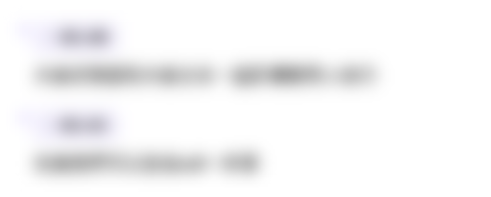
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
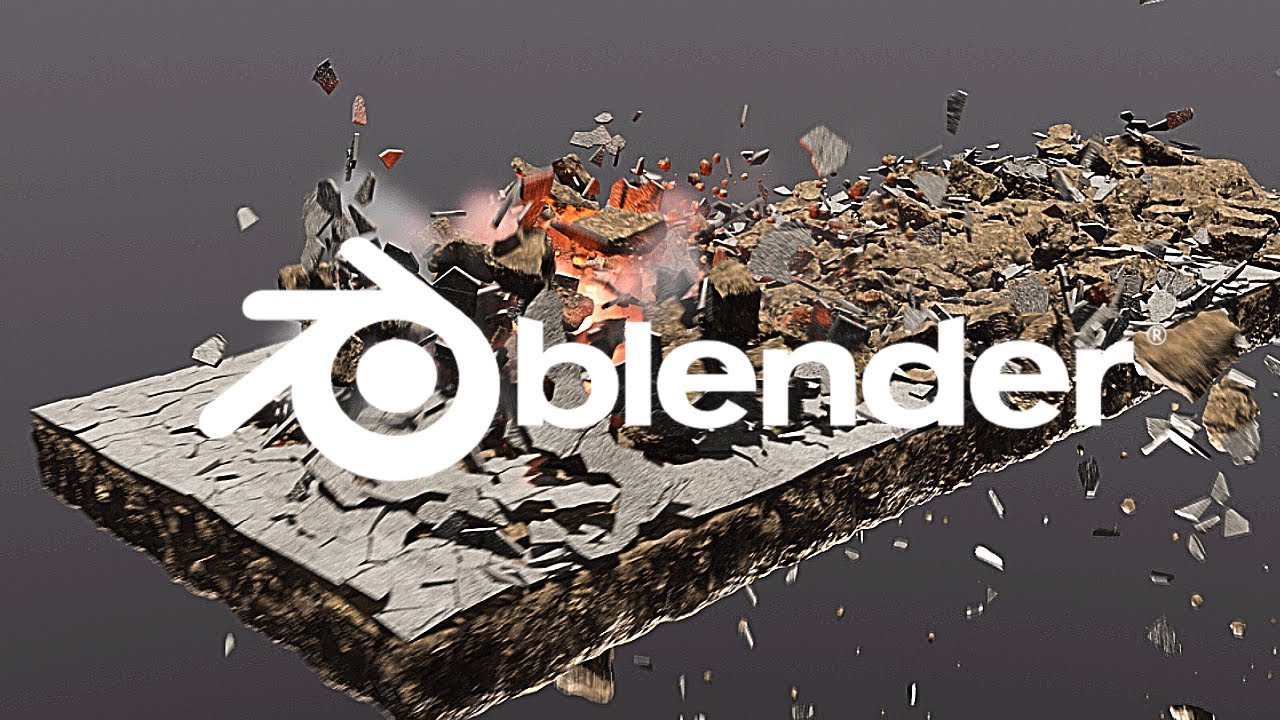
Realistic destruction effects in blender
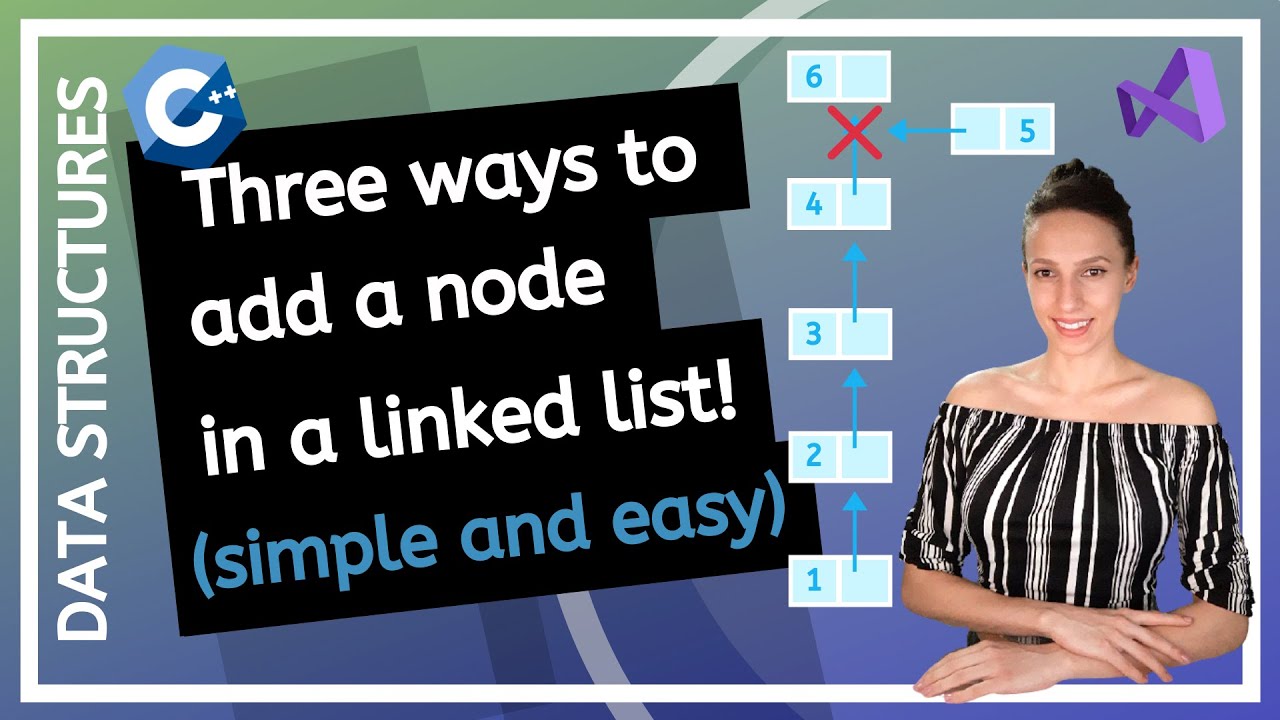
How to insert a new node in a linked list in C++? (at the front, at the end, after a given node)
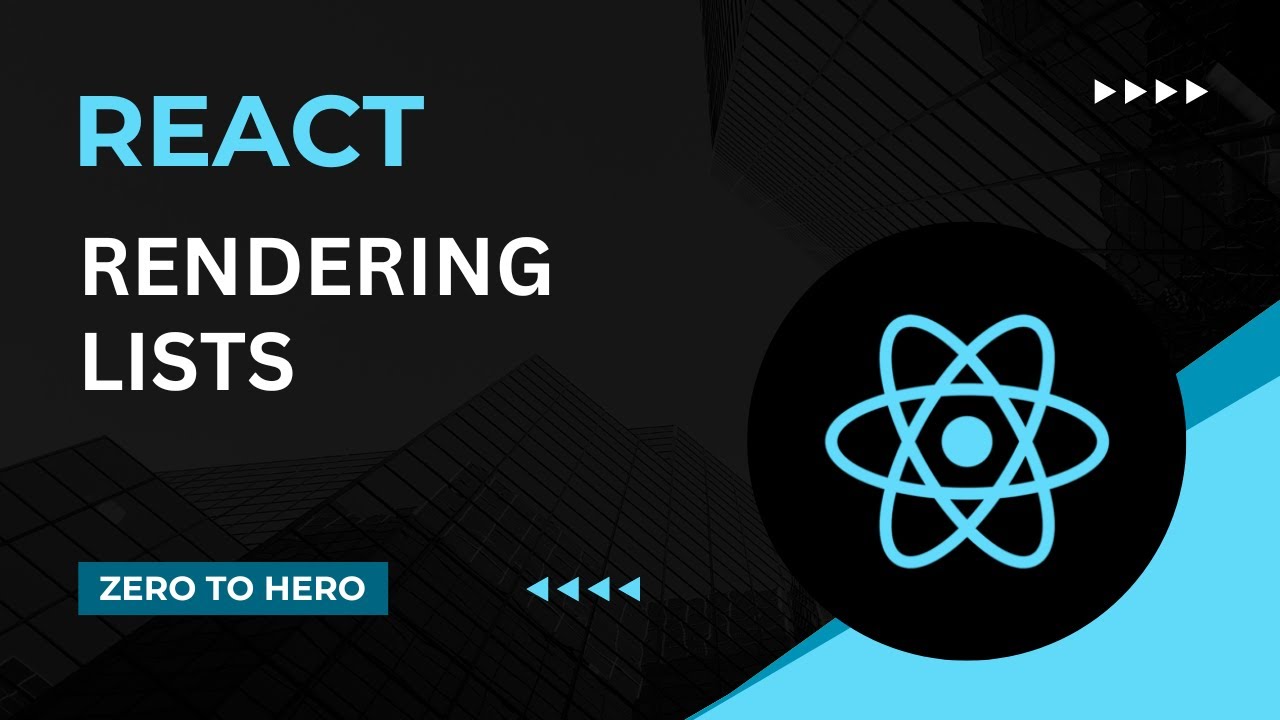
How to render lists | Mastering React: An In-Depth Zero to Hero Video Series
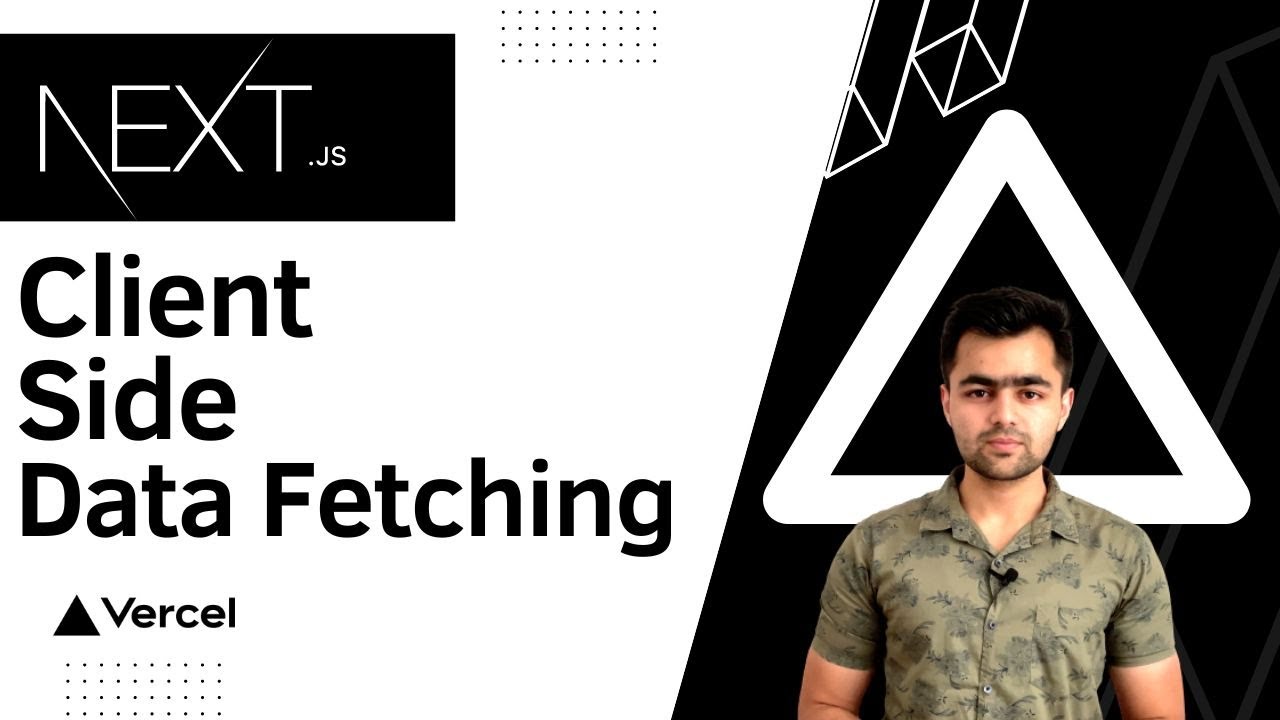
Client Side Data Fetching in NextJS | NextJS in Hindi
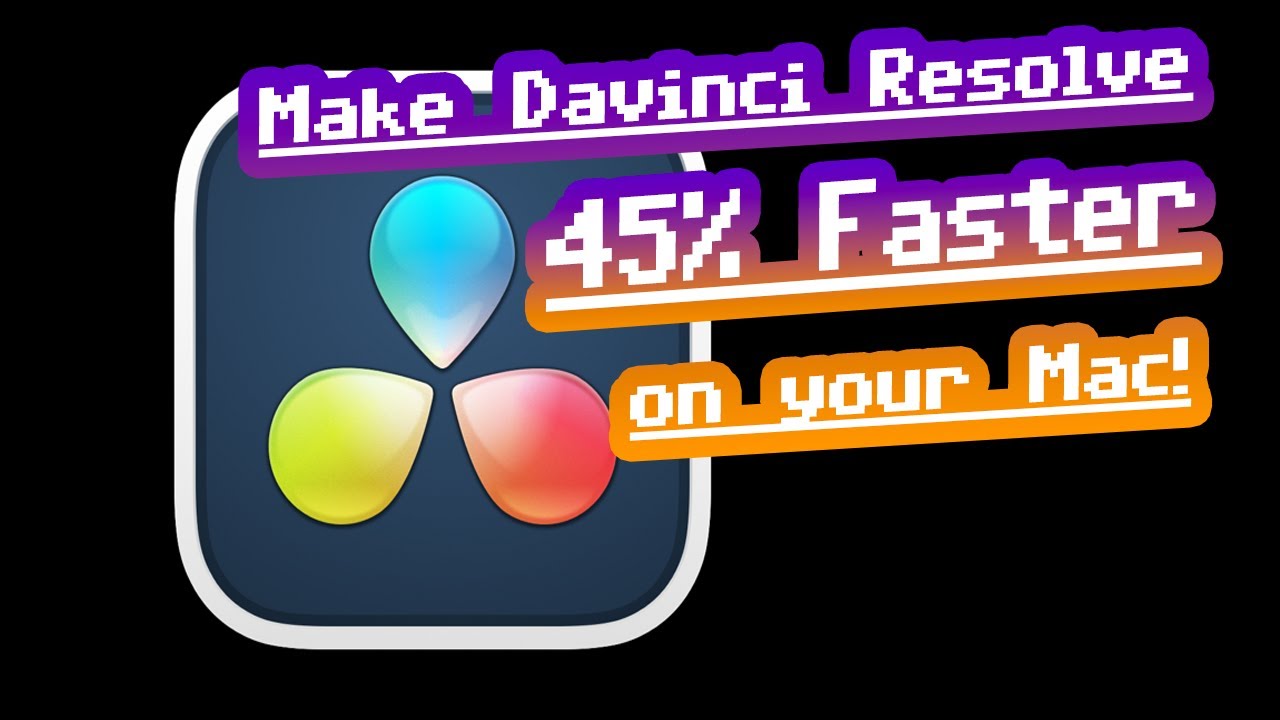
Make DaVinci Resolve 45% Faster on your Mac!
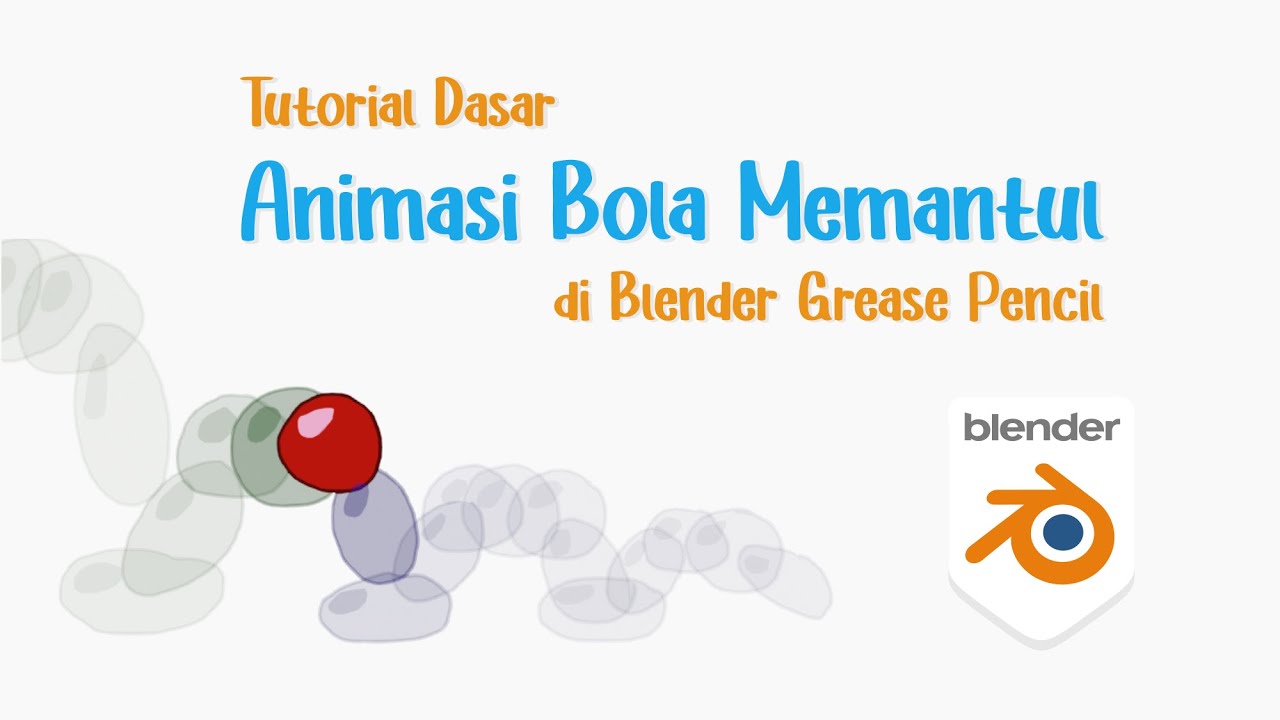
Tutorial Dasar Membuat Animasi Bola Memantul Frame by Frame di Blender Grease Pencil
5.0 / 5 (0 votes)