Introduction to C++, The Parts of a C++ Program, Identifier Naming Rules, output statements
Summary
TLDRThis C++ programming language course introduces the fundamental components of a C++ program through a simple example that prints 'Hello, World!' The tutorial covers key concepts such as comments, pre-processor directives, namespaces, and the main function. It explains the use of input/output streams, string literals, variables, data types, and the importance of using meaningful identifiers. Special characters and operators, like the insertion operator and end-of-line manipulator, are discussed in detail. The course emphasizes best practices in naming variables and adhering to identifier rules, making it a comprehensive introduction for beginners in programming.
Takeaways
- 😀 C++ programs begin with comments that are ignored by the compiler, helping to explain the code.
- 📥 The `#include <iostream>` directive is essential for using input and output streams in C++.
- 🔧 The `using namespace std;` line specifies that the standard library should be used, making the code simpler.
- 🚀 Every C++ program requires a `main()` function where execution starts.
- 🖥️ The `cout` object is used for outputting text to the console, with strings enclosed in double quotes.
- 🔄 A semicolon is required at the end of each statement in C++, marking the end of that statement.
- 💾 Variables must be declared with a data type (e.g., `int`, `string`), indicating the type of data they will store.
- 📝 Identifiers (variable names) should be descriptive of their purpose and must follow specific naming rules.
- ⚠️ Keywords in C++ are reserved words and cannot be used as identifiers.
- 🔤 Special characters like underscore (_) are allowed in identifiers, but they cannot start with a digit or use other symbols.
Q & A
What is the purpose of comments in a C++ program?
-Comments are used to explain code and are ignored by the compiler.
What does the pre-processor directive '#include <iostream>' do?
-'#include <iostream>' allows the program to use input and output streams, such as 'cin' for input and 'cout' for output.
Why is the 'main()' function important in C++?
-The 'main()' function is the entry point of a C++ program where the execution begins.
How do you display output in a C++ program?
-Output is displayed using 'cout', which sends output to the standard output stream.
What is the significance of the semicolon in C++?
-In C++, every statement must end with a semicolon to indicate the end of that statement.
What is the 'endl' manipulator used for?
-'endl' is used to end the current line of output and move the cursor to the next line.
What is an escape sequence, and give an example?
-An escape sequence is a character sequence that performs a specific function, such as ' ' for a new line.
What are identifiers in C++?
-Identifiers are names given to variables, functions, classes, etc., that must follow specific naming rules.
What are the rules for naming identifiers in C++?
-Identifiers must start with a letter or underscore, can include letters, digits, or underscores, and cannot use special characters other than the underscore.
What are keywords in C++?
-Keywords are reserved words in C++ that have special meanings and cannot be used as identifiers.
Why is it important to choose descriptive variable names?
-Descriptive variable names make the code more readable and understandable, reflecting the variable's purpose or content.
What happens if you try to print a variable with double quotes?
-If a variable is printed with double quotes, it will print the variable name as a string instead of its value.
Outlines
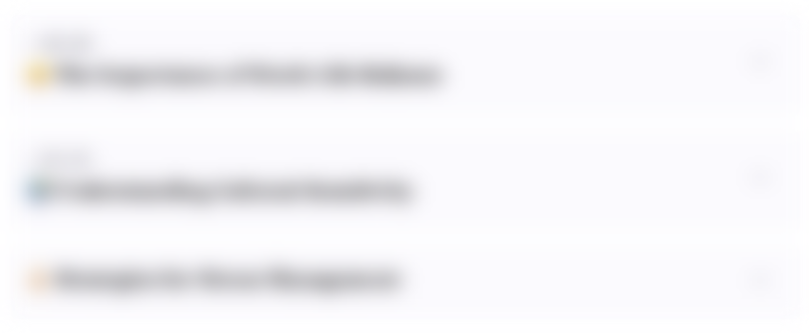
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
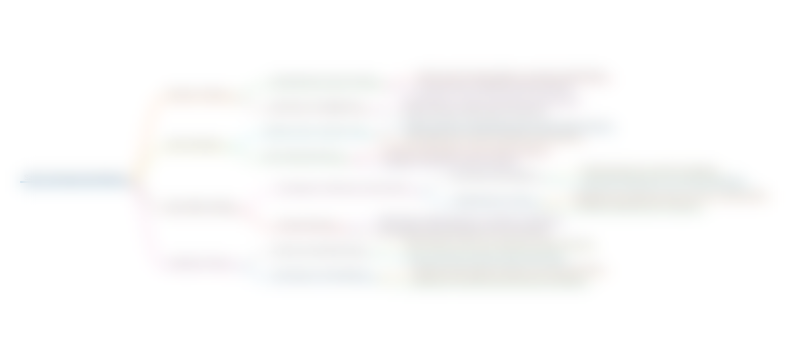
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
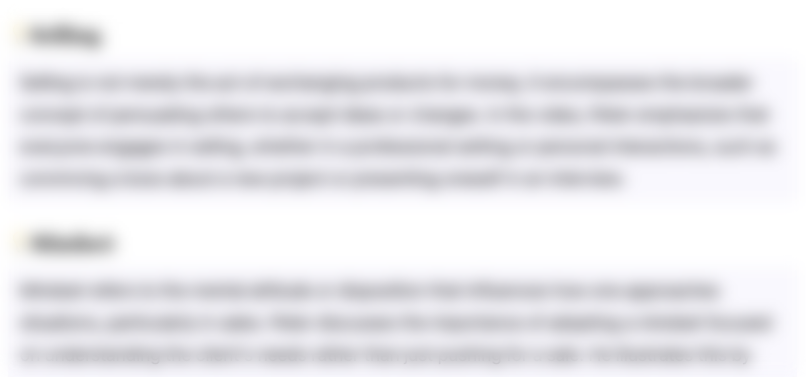
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
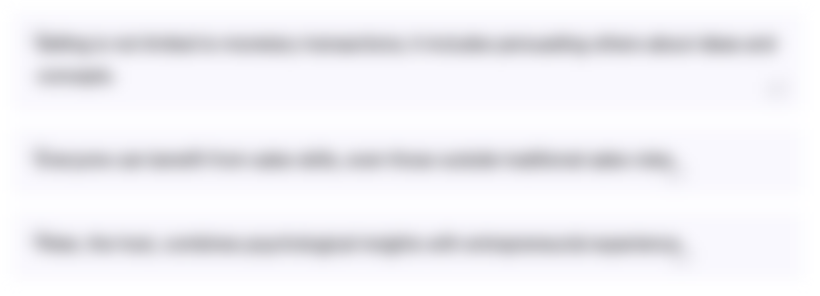
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
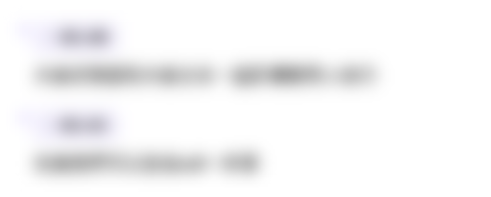
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频
5.0 / 5 (0 votes)