Modular Programming, Widely-used C++ functions
Summary
TLDRThis C++ programming tutorial covers essential concepts such as the main function, function prototypes, and modular programming. The script explains how the main function serves as the entry point of the program and introduces function prototypes for better code organization. It highlights the importance of modular programming for reusability and testing, with an example of creating a library. Additionally, it demonstrates the use of standard C++ functions from various libraries (like math, algorithm, and time). Finally, the script provides insight into random number generation using C++'s pseudo-random number generator, showcasing the flexibility and efficiency of modular programming.
Takeaways
- 😀 Every C++ program must have a main function, which serves as the entry point when the operating system runs the program.
- 😀 Function prototypes are typically declared before the main function, while their definitions or implementations are placed after the main function.
- 😀 Modular programming allows you to group related functions into a library, making them reusable across different programs.
- 😀 C++ supports modular programming, which leads to code reusability, reduced duplication, and better program organization.
- 😀 Libraries in C++ can be structured into header files (with function prototypes) and implementation files (with function definitions).
- 😀 To use functions from another file, you need to include the header file in your main program using an #include directive.
- 😀 When working with multiple C++ files, you compile and link them together to create an executable, using commands like g++ to compile them.
- 😀 The concept of 'don't repeat yourself' (DRY) is key in modular programming, where you write a function once and reuse it across multiple programs.
- 😀 Standard C++ libraries, such as the math, algorithm, and ctime libraries, provide many pre-built functions that are ready to use without having to reimplement them.
- 😀 To generate random numbers in C++, you use the rand() function, but you must first initialize the random number generator with sRand() to ensure variability.
- 😀 C++ provides a wide variety of libraries and functions for different use cases, such as mathematical calculations, sorting, and time manipulation, which are crucial for efficient programming.
Q & A
What is the main function in a C++ program and why is it important?
-The main function is the entry point of any C++ program. When the operating system starts executing the program, it begins at the main function. The main function is crucial because it marks the start of the program's execution.
What is the role of function prototypes in C++ programming?
-Function prototypes are declarations of functions that specify the function's return type, name, and parameters without including the actual body of the function. They allow the compiler to verify that functions are called correctly before they are defined or implemented.
What is modular programming in C++ and why is it important?
-Modular programming involves dividing a program into smaller, self-contained units or modules, each handling a specific task. This approach increases code reusability, simplifies maintenance, and improves program clarity.
Why is it preferred to place function prototypes before the main function in C++?
-Placing function prototypes before the main function allows the compiler to check for correct usage of functions during compilation, even before their full implementation is provided. This helps in detecting errors early.
What does the file structure for a C++ program that uses a library typically look like?
-In a C++ program that uses a library, the program usually consists of three files: a header file (with function prototypes), a source file (with function definitions), and a driver program (main.cpp) that calls the functions from the library.
How do you compile and link multiple C++ files?
-To compile and link multiple C++ files, use the command 'g++ -o output_file source_file1 source_file2'. This compiles the source files and links them into an executable file. For example, 'g++ -o main main.cpp li.cpp' compiles main.cpp and li.cpp into an executable named 'main'.
What is the benefit of using libraries in C++ programming?
-Using libraries in C++ allows programmers to reuse tested and predefined functions, which saves time and ensures reliability. Instead of rewriting common functions like sorting or mathematical operations, you can include libraries that provide these functionalities.
What are some of the common libraries in C++ and what functions do they provide?
-Some common C++ libraries include the 'algorithm' library (for functions like min, max, sorting), 'cmath' (for mathematical functions like sqrt, pow), 'ctime' (for time-related functions), and 'cstdlib' (for functions like rand, abs). These libraries provide a wide range of utilities for programming.
How does the random number generation work in C++ using the 'rand' function?
-To generate random numbers in C++, the 'rand' function is used, but it requires initialization with 'srand'. The seed for the random number generator is typically set using the current time to ensure different sequences of random numbers on each program run.
How can you generate random numbers within a specific range in C++?
-To generate random numbers within a specific range, you use the 'rand' function with the modulo operator. For example, 'rand() % range' gives a random number between 0 and 'range-1'. To shift the range, you can add a base value to the result.
Outlines
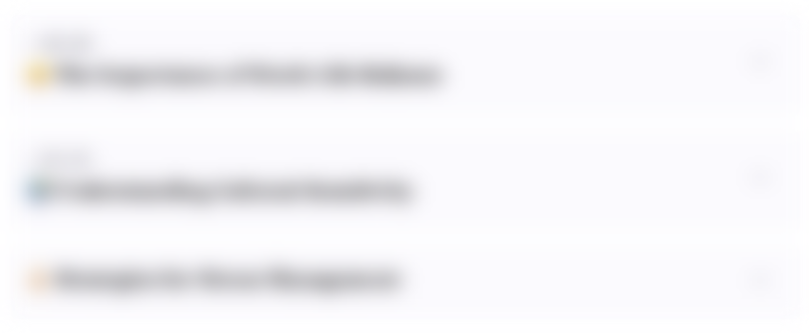
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
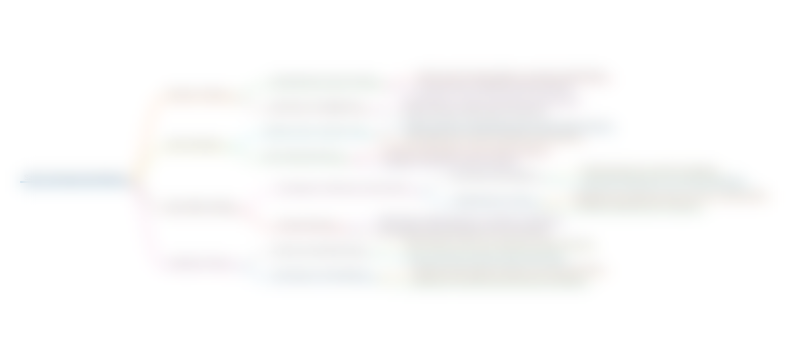
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
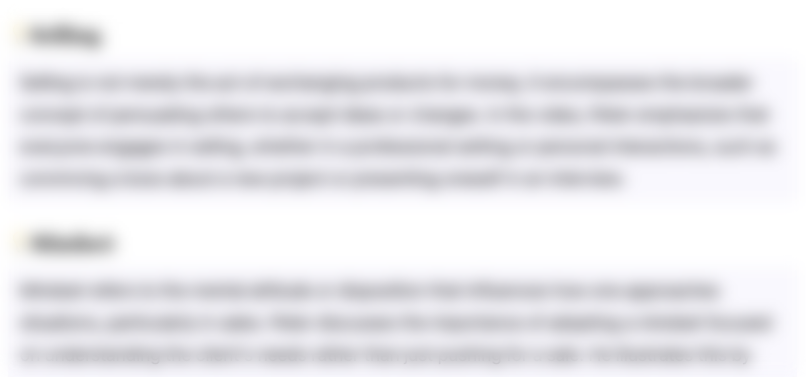
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
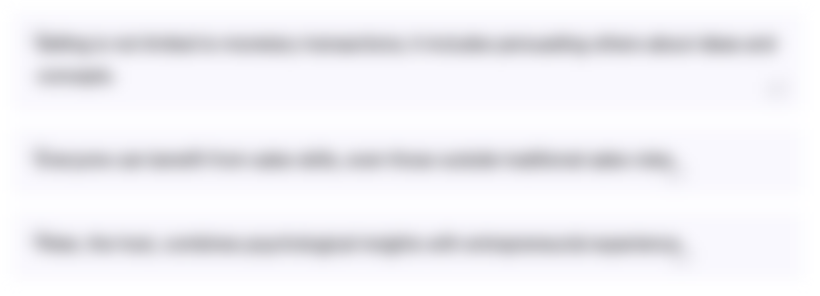
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
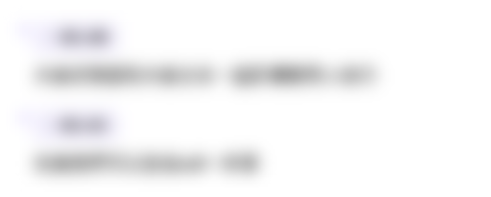
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
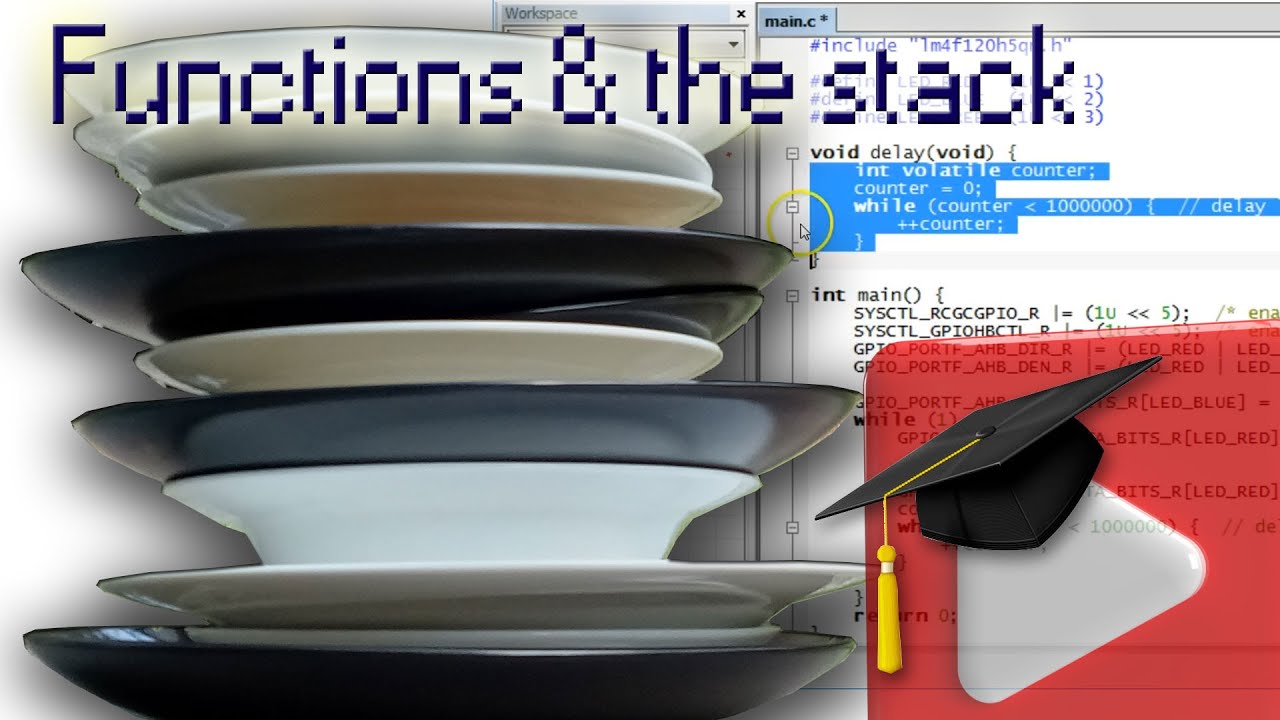
#8 Functions in C and the call stack
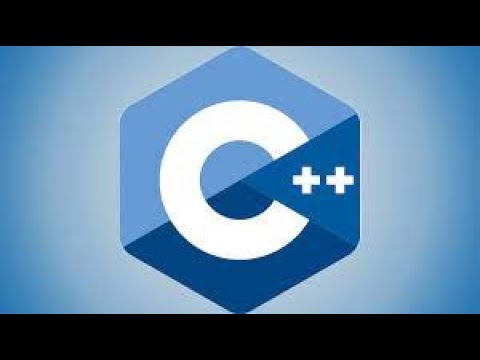
C++ programming, Void function, get input by reference, call function by value and by reference
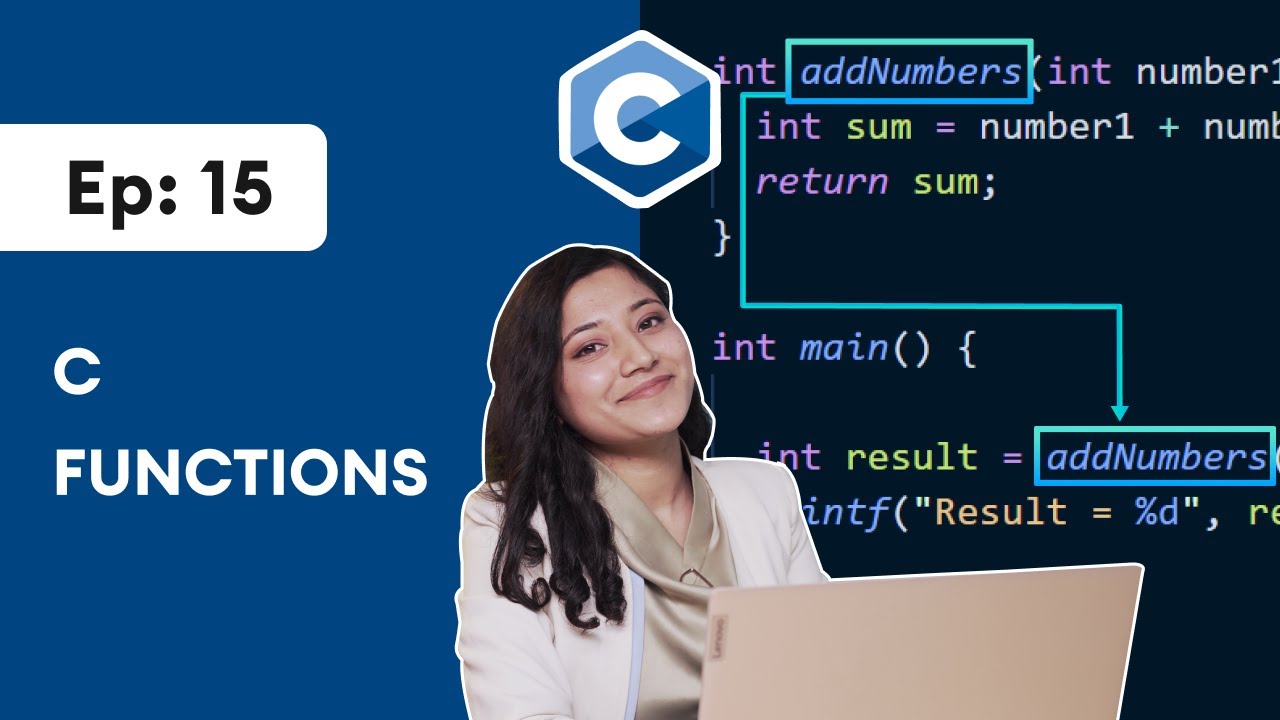
#15 C Functions | C Programming for Beginners
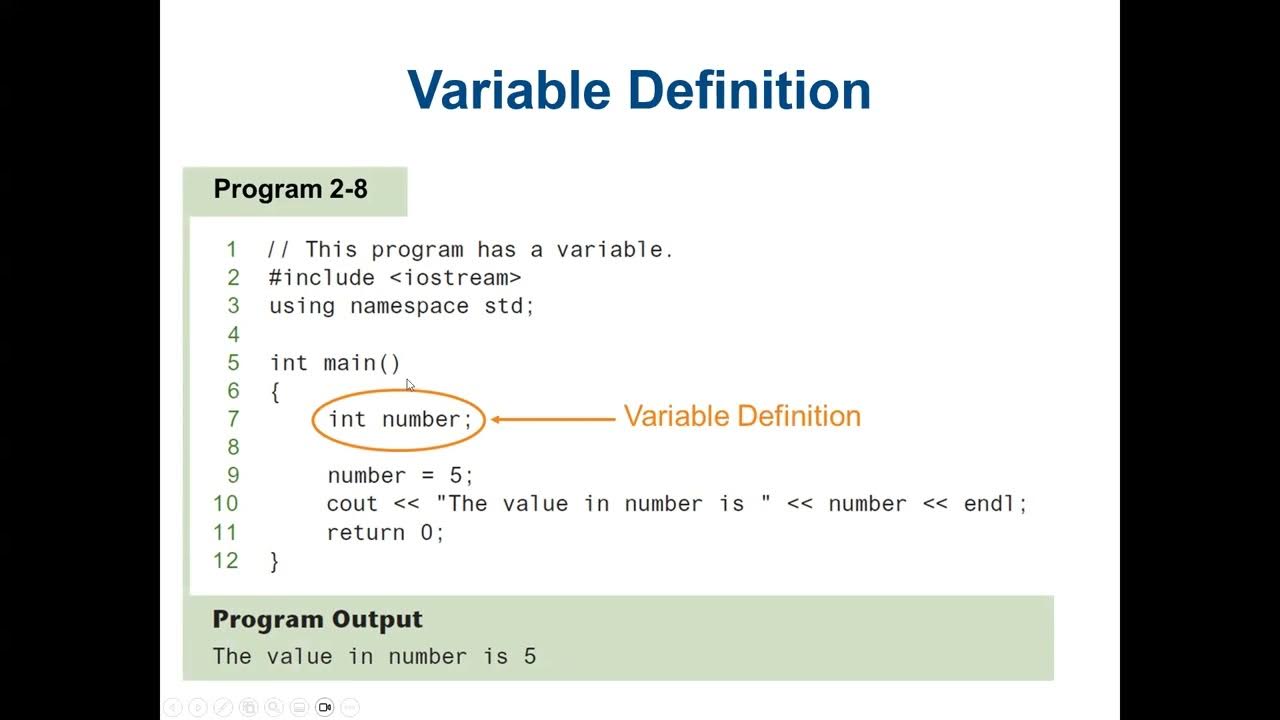
Introduction to C++, The Parts of a C++ Program, Identifier Naming Rules, output statements
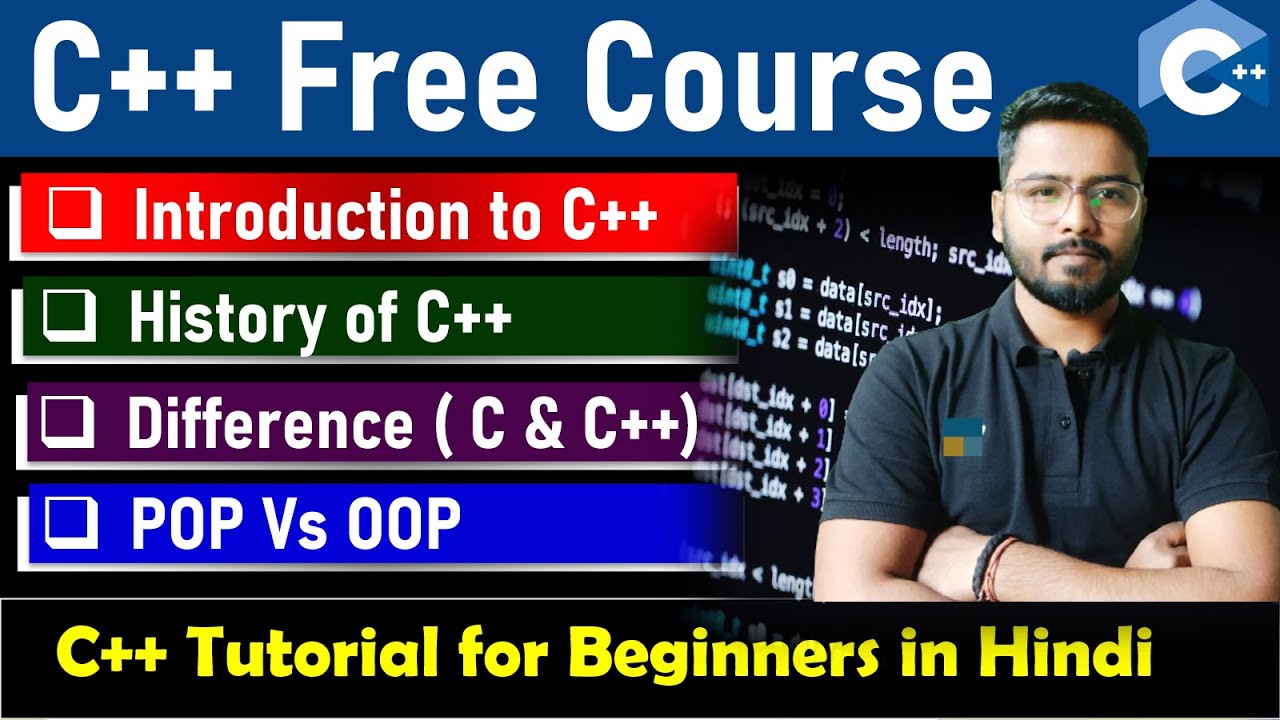
C++ Tutorial - Introduction to C++ | C++ Tutorial For Beginners #c++

C Programming – Features & The First C Program
5.0 / 5 (0 votes)