Next.js 14 Tutorial - 50 - Server and Client Components
Summary
TLDRThe video explains the difference between server and client components in Next.js applications. By default, all components in Next.js are server components, which provide benefits like zero bundle size and SEO. However, server components can't use browser APIs or handle interactivity. The video shows how to create a client component using the 'useClient' directive, allowing access to state and browser APIs. It also covers the rendering behavior - client components prerender on the server then execute on client. The video summarizes that server components only run on server, while client components run on both server and client.
Takeaways
- ๐ By default, every component in a Next.js app is a server component, including the default pages
- ๐ Server components provide benefits like zero bundle size, access to server resources, and better SEO
- โฑ Server components cannot interact with browser APIs or handle interactivity like state
- ๐ก Use the `useClient` directive to define client components that can use browser APIs and state
- ๐บ Client components render once on the server for pre-rendering, and then client-side for interactivity
- ๐ต Server components only render on the server, not on the client
- ๐ Client components render on server for pre-rendering, then client for interactivity
- โ๏ธ The `useClient` directive signals Next.js to execute a component client-side
- ๐โโ๏ธ Client components can access browser APIs and handle interactivity
- ๐ก Understanding server vs client components is key to using React Server Components
Q & A
What is the default component type in a Next.js application?
-By default, every component in a Next.js app is considered a server component, including the root layout and root page components.
How do you create a client component in Next.js?
-To create a client component in Next.js, you must include the `useClient` directive at the top of the component file. This signals to Next.js that the component is intended for client-side execution.
Can server components use state and interact with browser APIs?
-No, server components cannot use state or interact directly with browser APIs because they are rendered on the server where there is no browser.
Where do client components execute?
-Client components execute primarily on the client-side to allow access to browser APIs and interactivity. However, they are also pre-rendered once on the server to allow immediate display of HTML without a blank screen.
What are some benefits of server components?
-Benefits of server components include zero bundle size, access to server-side resources, enhanced security, better SEO, etc.
When will a client component log a message?
-A client component will log a message in the browser console when rendered on the client-side. It will also log a message in the terminal when pre-rendered on the server.
Why can't a server component use React hooks like useState?
-Server components run on the server where there is no concept of state that exists in the browser. React hooks like useState require a client-side environment to work properly.
What is the purpose of pre-rendering client components on the server?
-Pre-rendering client components on the server allows the user to immediately see the page's HTML content rather than a blank screen while waiting for client-side JavaScript.
How do you define routes for server and client components?
-Routes for both server and client components are defined the same way in Next.js - by creating files under the pages directory. The component type is determined by whether the `useClient` directive is present.
Can a single Next.js application have both server and client components?
-Yes, a Next.js application can have a mix of server components and client components defined using the `useClient` directive.
Outlines
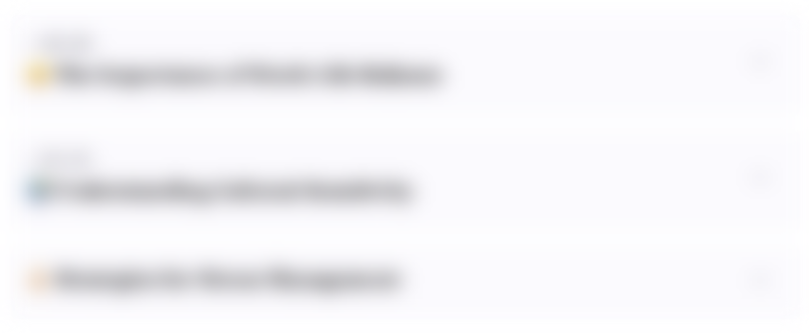
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
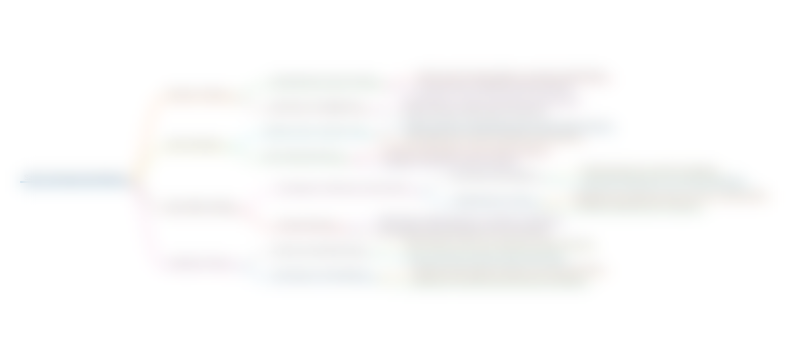
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
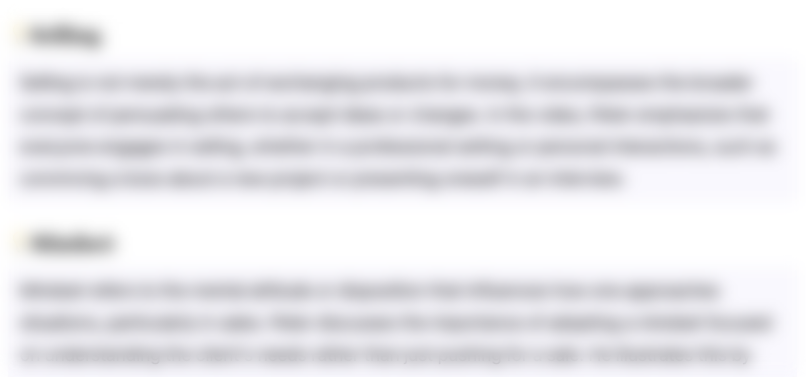
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
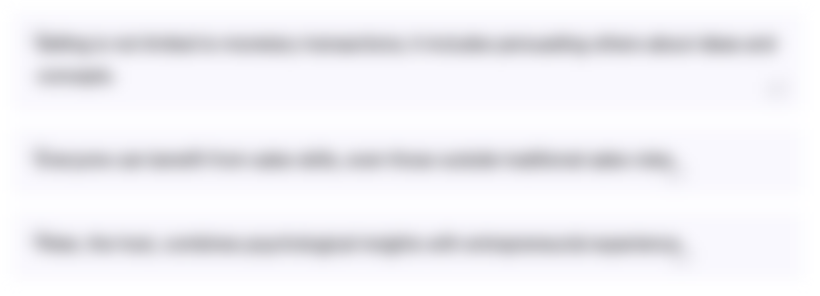
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
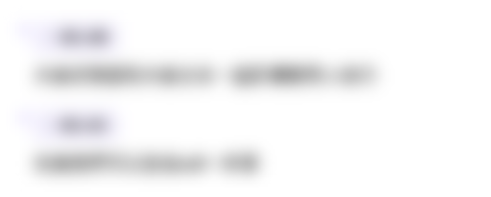
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
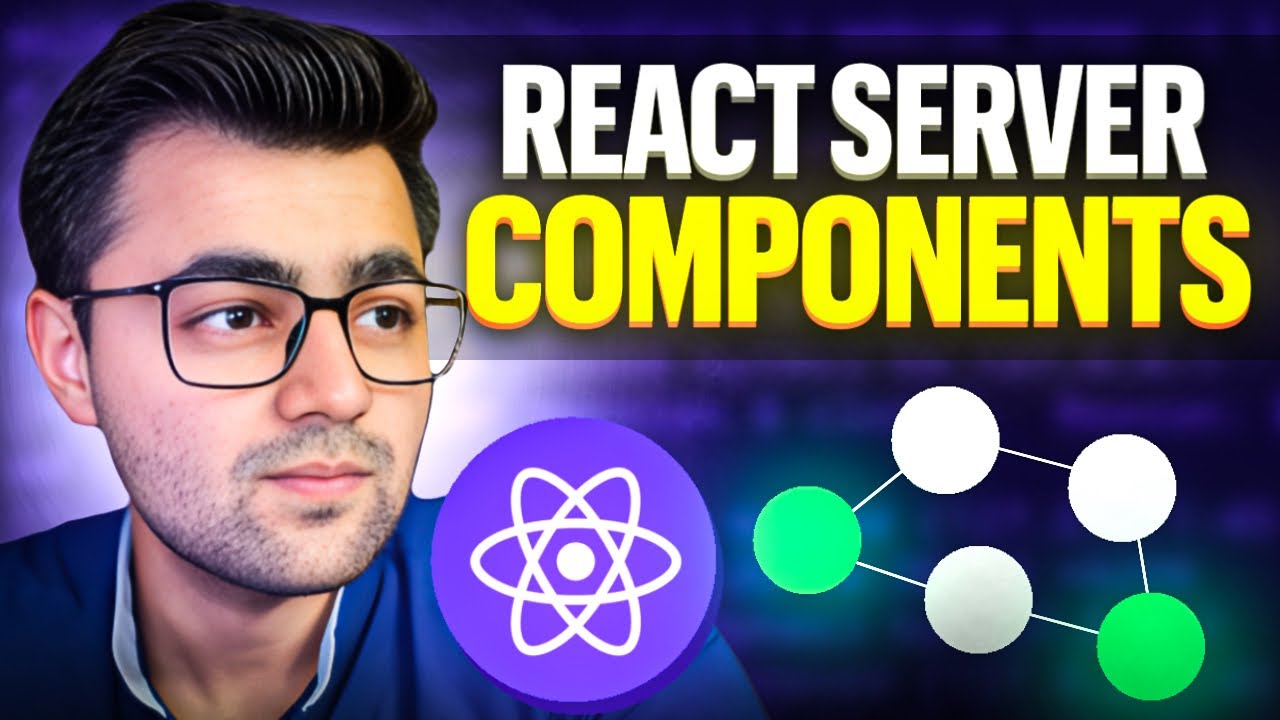
React Server Components vs Client Components
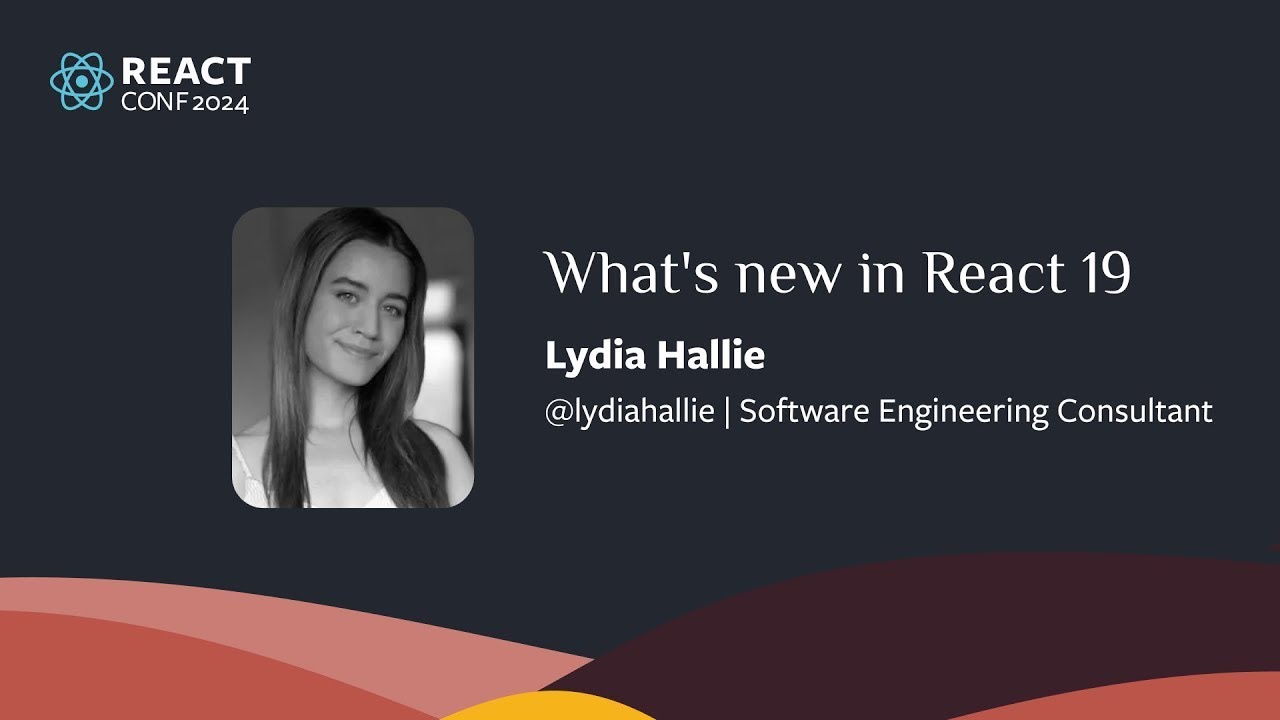
What's new in React 19 | Lydia Hallie

10 common mistakes with the Next.js App Router

Next.js Fetch Data the Right Way (with a Data Access Layer!) (Security, Auth, Cache, DTO)
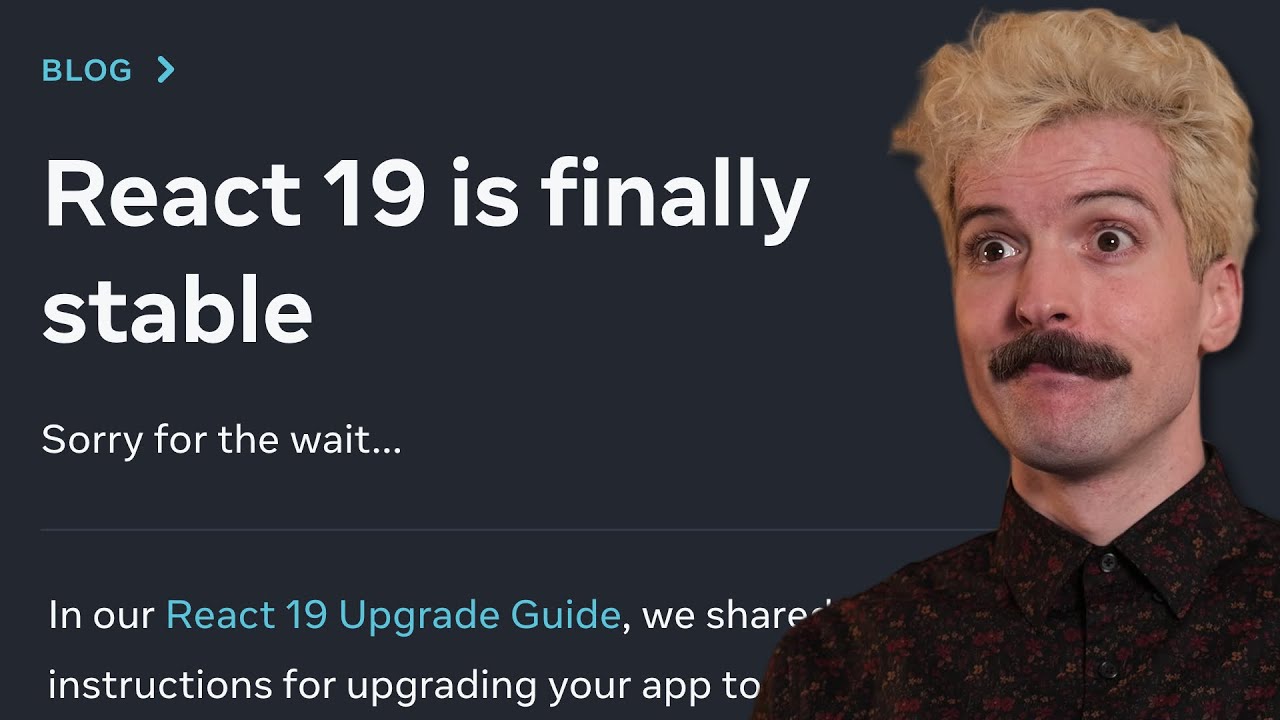
React 19 is finally out!
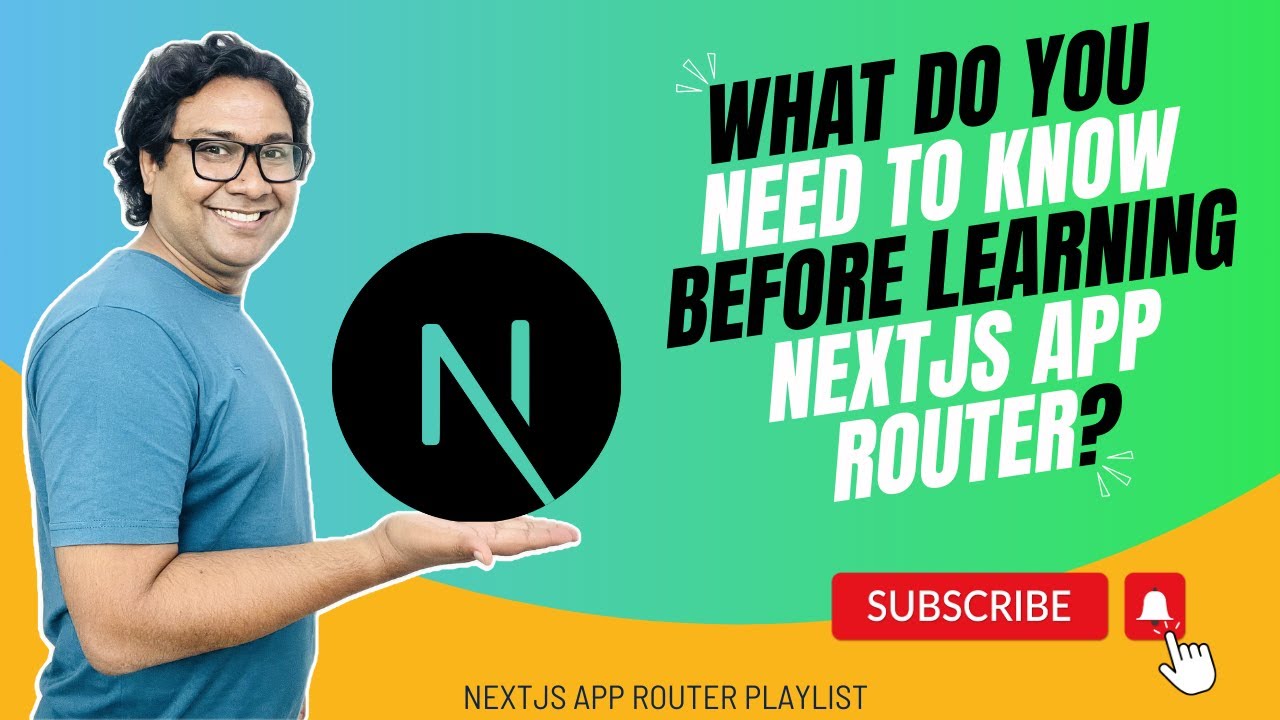
What Do You Need To Know Before Learning NextJS App Router?

Netflix Removed React?
5.0 / 5 (0 votes)