10 common mistakes with the Next.js App Router
Summary
TLDRThe video outlines 10 common mistakes when building Next.js applications using the app router. Topics covered include: incorrectly implementing route handlers, misunderstanding route handler defaults and caching behaviors, unnecessarily using route handlers with client components, improperly utilizing Suspense boundaries with server components, not accessing request data in server components, misplacing context providers in the component tree, misunderstanding client/server component relationships, forgetting to revalidate data after mutations, throwing redirects inside try/catches instead of using routers or actions. The goal is to help developers avoid these pitfalls and build better Next.js apps.
Takeaways
- π― Don't call route handlers from React components, call functions directly instead
- π‘ Route handlers are cached by default like Pages, make them dynamic if needed
- π Server actions work with client components too
- π€ Place Suspense boundaries above data fetching components
- iοΈ Use component props to access request info like headers and params
- π Context providers belong in Root layout, not wrapped around each component
- πͺ Client components don't need explicit `useClient` if parent is already client
- π Common mistake to forget revalidating data after mutations
- βοΈ Don't throw redirects inside try/catch blocks
- π Lots of opportunities to learn from mistakes building with Next.js!
Q & A
What is a common mistake developers make when using route handlers in Next.js applications?
-A common mistake is thinking that route handlers need to be called from React server components, leading to unnecessary network requests by fetching data from an API route that could be directly accessed or function calls within the server component itself.
How does the behavior of route handlers differ between local development and production environments in Next.js?
-In local development, route handlers might return new values on each request due to caching behavior being different. However, in production, after building and starting the server, the data is cached by default and does not change on each request, showcasing the difference between local and production environments.
Why is it considered a mistake to use fetch within React server components for accessing local data in Next.js?
-Using fetch within server components to access local data is unnecessary because both the data fetching and the component rendering occur on the server. Developers can directly call functions to retrieve or manipulate data without making an extra network request.
What is the purpose of route handlers in Next.js applications?
-Route handlers allow developers to handle HTTP verbs like GET, POST, PUT, and DELETE, enabling API routes for fetching or mutating data. They are particularly useful for handling webhooks or external requests.
Can server actions be used with client components in Next.js?
-Yes, server actions can be used with client components to simplify interactions, such as form submissions, without the need for additional code to handle route handlers, thus streamlining the development process.
What is a common misconception about using suspense with server components in Next.js?
-A common misconception is placing suspense boundaries incorrectly, specifically not placing them higher than the data fetching component. For suspense to work effectively, the suspense boundary needs to encompass the component where data fetching occurs.
How can developers handle incoming request data in server components with Next.js?
-Developers can handle incoming request data by utilizing built-in functionalities like headers, cookies, params, and search params. These allow reading information from the request, such as headers or URL parameters, directly within server components.
What is a common mistake when integrating context providers in Next.js applications?
-A common mistake is incorrectly placing context providers within the component tree. The best practice is to place them in the root layout, allowing the application to maintain server components as children while still utilizing client components where necessary.
Why is revalidating data after a mutation important in Next.js applications?
-Revalidating data after a mutation is crucial to ensure that the UI reflects the latest state. Without revalidation, changes made through server actions may not be immediately visible, leading to a mismatch between the server state and the client display.
What is the recommended way to perform redirects in Next.js applications?
-The recommended way to perform redirects is to use them outside of try-catch blocks or in a finally block to avoid conflicts with error handling. For client-side redirects, the useRouter hook should be used for programmatic navigation.
Outlines
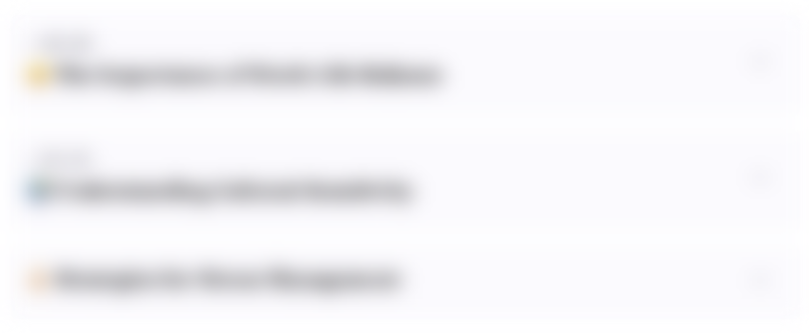
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
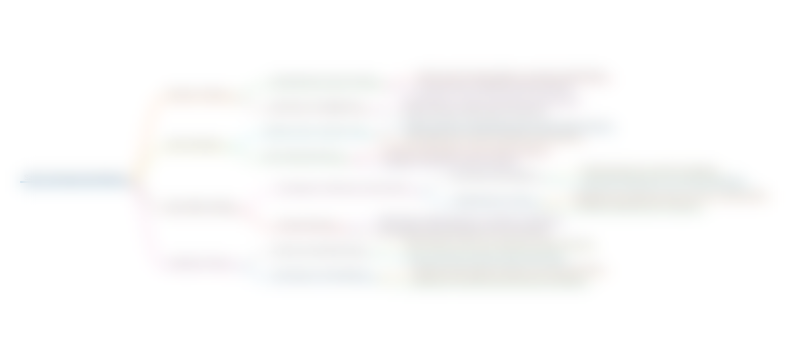
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
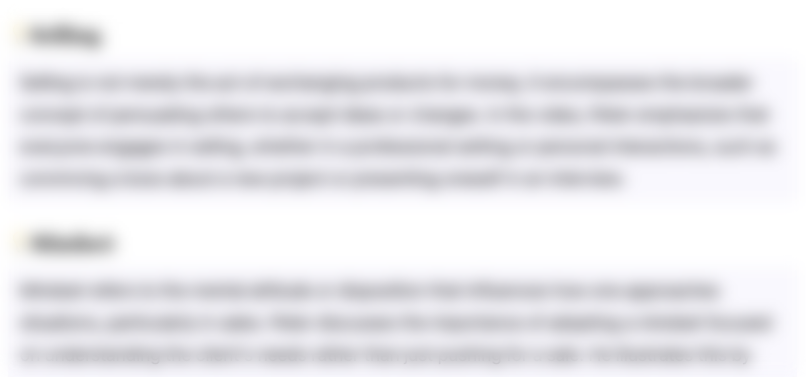
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
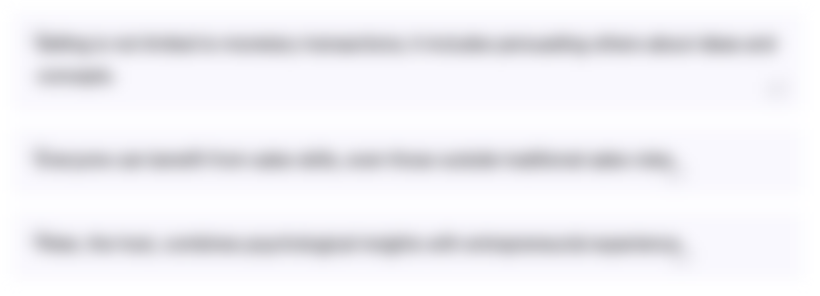
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
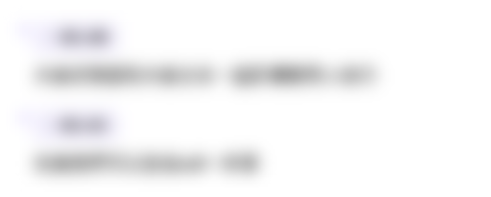
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

How To Setup Your NextJS Codebase | Routes, Components, Data Access Layer etc

Next.js Fetch Data the Right Way (with a Data Access Layer!) (Security, Auth, Cache, DTO)

A First Look at Effects | Lecture 141 | React.JS π₯
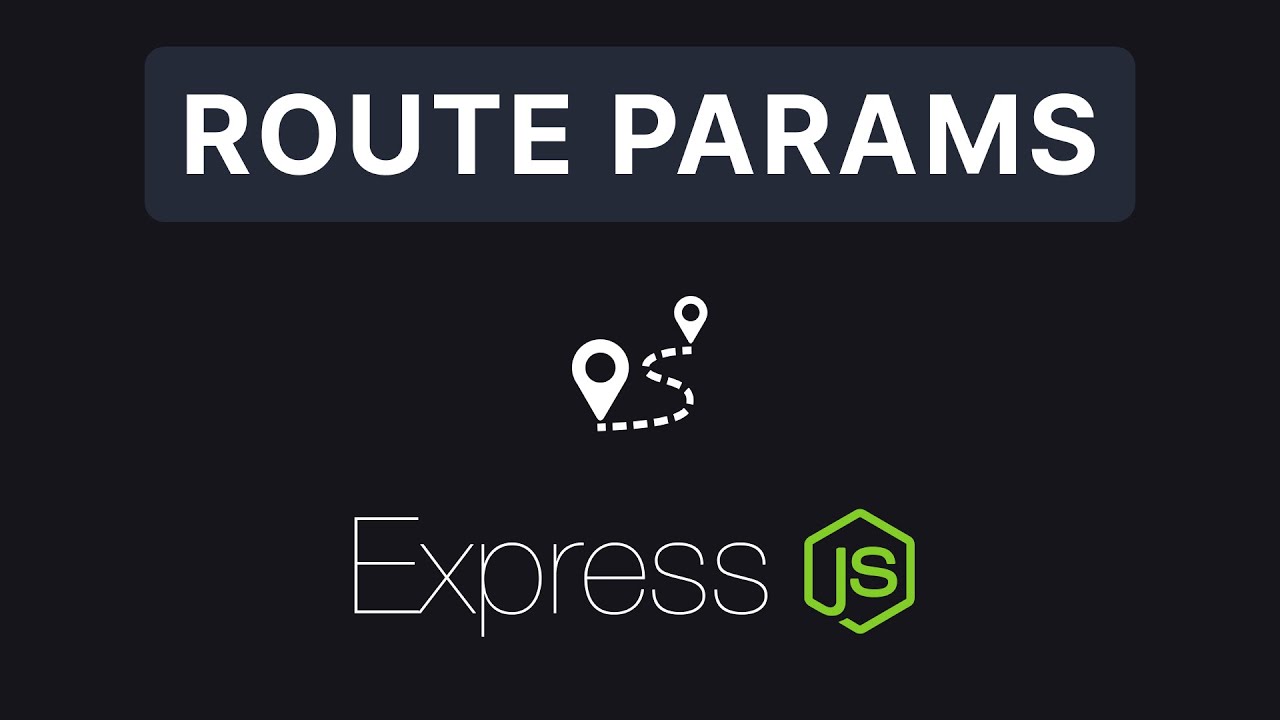
Express JS #3 - Route Parameters

Performance in React and Next.js (Lydia Hallie)

React's most dangerous feature
5.0 / 5 (0 votes)