Ep.3 - Singleton | Flutter Design Patterns
Summary
TLDRThis episode of the Flutter Design Pattern Series explores the Singleton design pattern, which ensures a class has only one instance and offers global access to it. The presenter uses analogies like a country's government and a company's CEO to clarify the concept. Through code examples, they demonstrate creating a private instance, a private constructor, and a static method to control instance creation. The video also discusses different implementations of Singleton in Dart, its use cases like managing a single database connection or socket, and its advantages and disadvantages, including its conflict with the single responsibility principle and challenges in unit testing.
Takeaways
- 📘 The Singleton design pattern restricts a class to a single instance while providing a global access point to that instance.
- 🏛 Analogous to a country having only one government, the Singleton pattern ensures there is only one controlling instance in a system, like a CEO for a company or a file system for an OS.
- 🔒 The Singleton pattern uses a private constructor to prevent external creation of multiple instances, enforcing the single instance rule.
- 🔑 A private static variable is used to hold the single instance of the class, ensuring it is created only once and accessed globally.
- 🛠️ The 'getInstance' method is a common approach to access the Singleton instance, providing controlled access and lazy initialization.
- 👷♂️ Demonstrated through code examples, the Singleton pattern is implemented in Dart with a private constructor and a public static method to access the instance.
- 🔄 Singletons can be implemented in different ways in Dart, such as using a getter or a factory constructor, each with its syntactic nuances.
- 🔗 The pattern is useful in scenarios where a single shared resource is needed, such as a database connection or a socket client in a Flutter application.
- 🚫 Singletons have downsides, including violating the single responsibility principle, difficulty in testing, and the lack of lazy initialization which can lead to unnecessary processing.
- 🛑 The use of 'late' variables can help address the issue of eager initialization in Singletons, only running heavy tasks when the instance is actually used.
- 📚 Singletons are more commonly used in package development rather than application code due to their potential disadvantages in application design.
Q & A
What is the Singleton design pattern?
-The Singleton design pattern ensures that a class has only one instance and provides a global point of access to it. It is useful when exactly one instance of a class is needed to coordinate actions across the system.
Why is the Singleton instance made private?
-The Singleton instance is made private to prevent the creation of multiple instances from outside the class. This ensures that only one instance of the class can exist.
What is the purpose of the private constructor in the Singleton pattern?
-The private constructor is used to prevent the instantiation of the class from outside the class itself. It ensures that no other instances of the class can be created.
How does the Singleton pattern provide a global point of access?
-The Singleton pattern provides a global point of access through a static method, often called 'getInstance', which returns the instance of the class.
What is the difference between a Singleton and a global variable?
-While both provide global access, a Singleton is a class that controls the creation of its instance and ensures there is only one instance, whereas a global variable can be overridden and does not control the number of instances.
Why might the Singleton pattern be difficult to test?
-The Singleton pattern can be difficult to test because it creates a global state that might affect other tests. It also violates the single responsibility principle and can lead to tightly coupled code, making it hard to isolate the code being tested.
What is the advantage of using the 'late' keyword with the Singleton instance?
-The 'late' keyword ensures that the Singleton instance is not created until it is actually used. This can prevent unnecessary initialization and is a form of lazy initialization.
Can the Singleton pattern be used with the Abstract Factory pattern?
-Yes, the Singleton pattern can be used within the Abstract Factory pattern to ensure that only one instance of the abstract factory is created, providing a consistent interface for creating objects.
What are some common use cases for the Singleton pattern in application development?
-Common use cases for the Singleton pattern include managing a single instance of a resource like a database connection, a logging service, or a configuration manager throughout an application.
Why are Singletons generally not recommended for application code?
-Singletons are generally not recommended for application code because they can make code harder to test, they violate the single responsibility principle, and they can lead to memory issues since the instance remains alive as long as the application is running.
Outlines
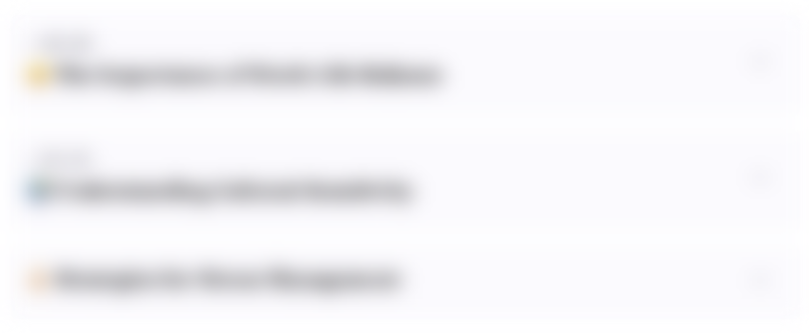
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
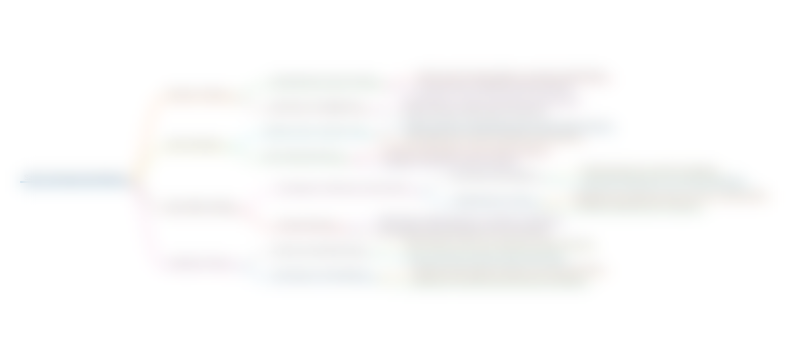
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
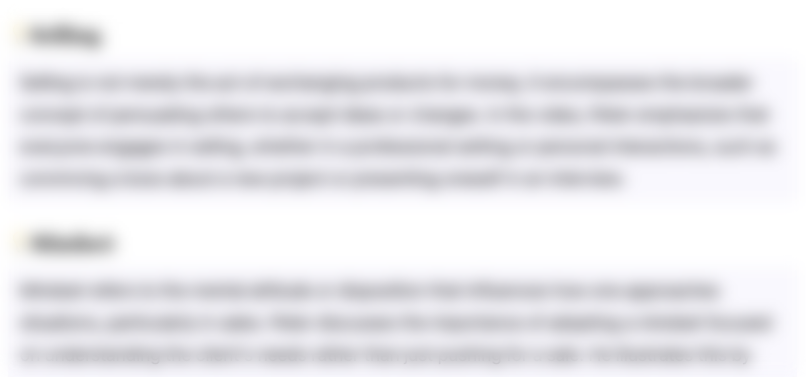
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
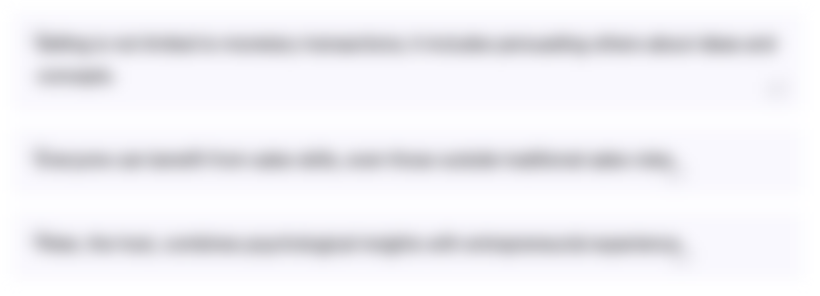
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
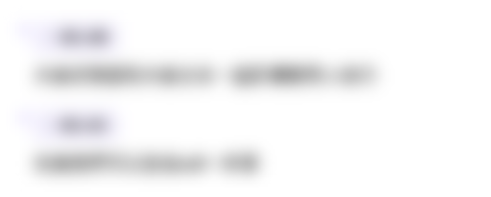
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
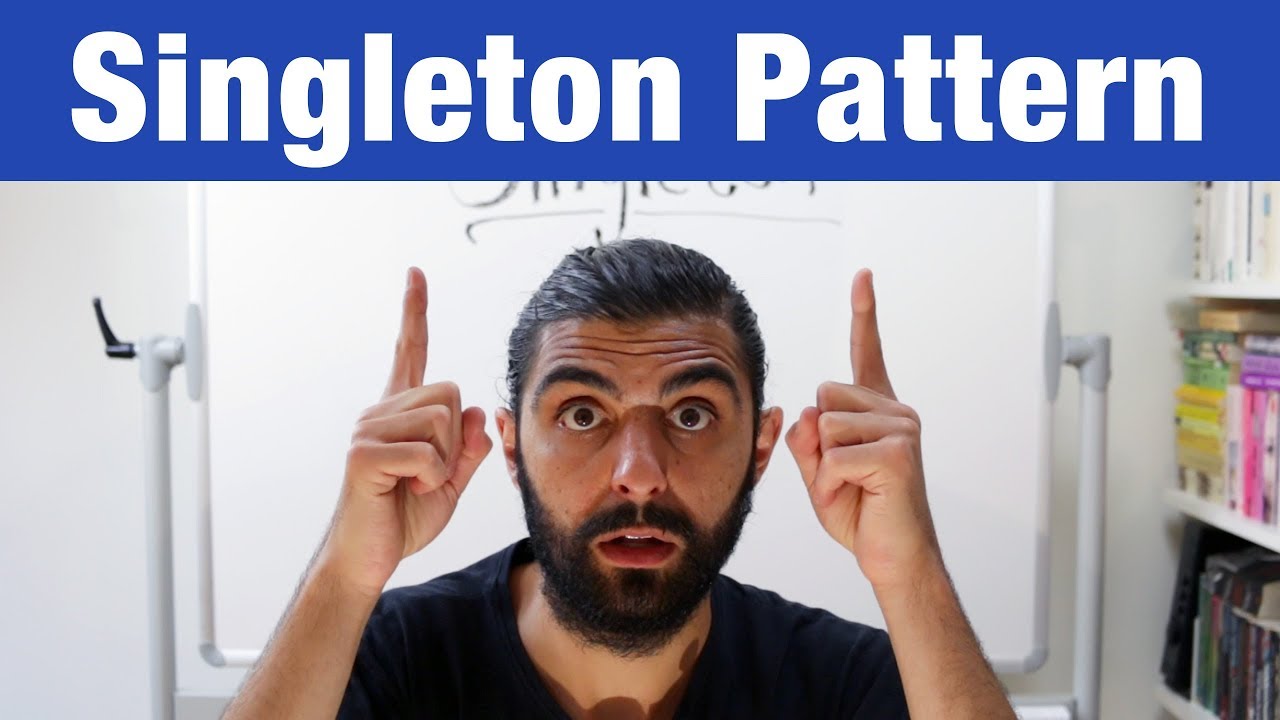
Singleton Pattern – Design Patterns (ep 6)

The Singleton Pattern Explained and Implemented in Java | Creational Design Patterns | Geekific
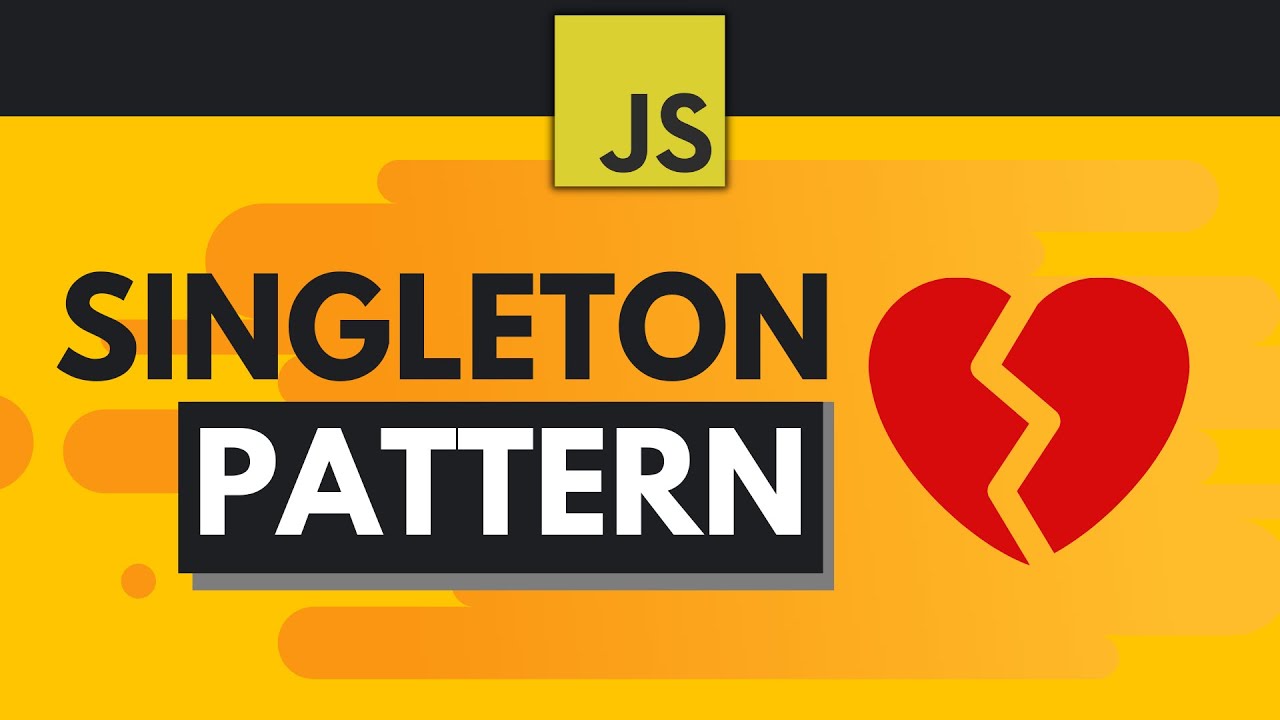
Javascript Design Patterns #2 - Singleton Pattern
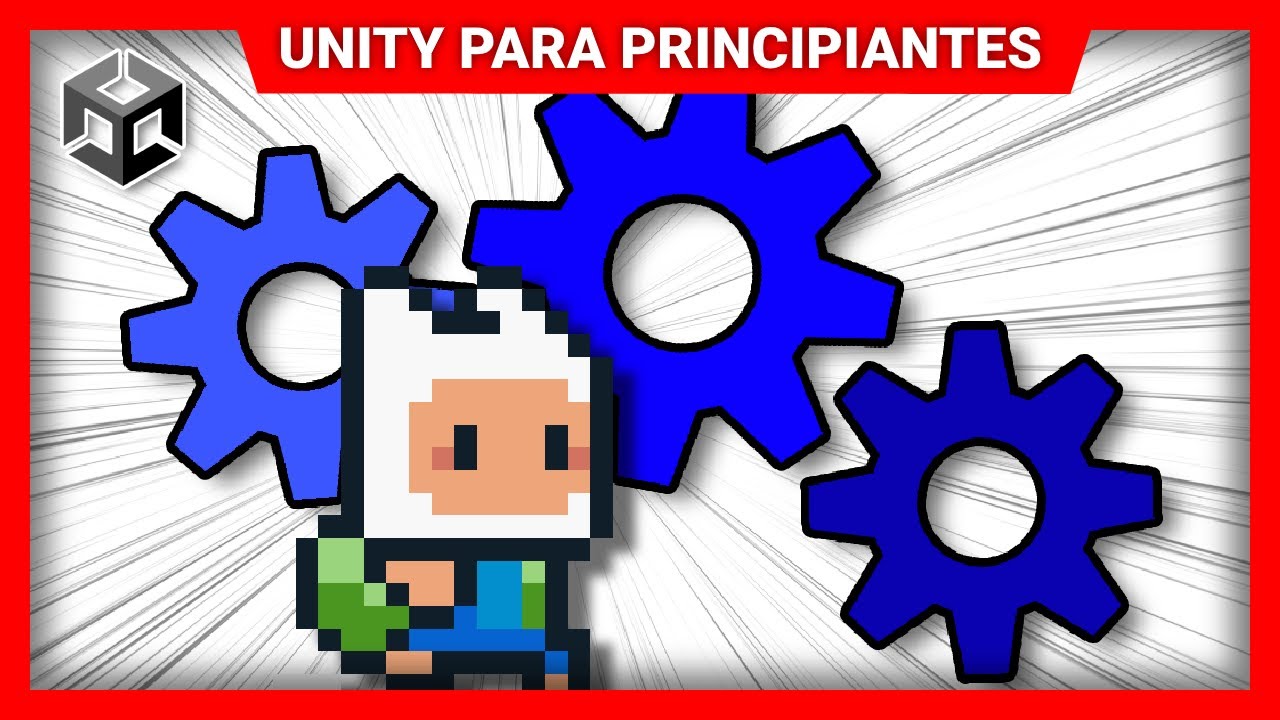
Cómo Hacer un Game Manager en Unity (Importante!) ► Unity para principiantes #010
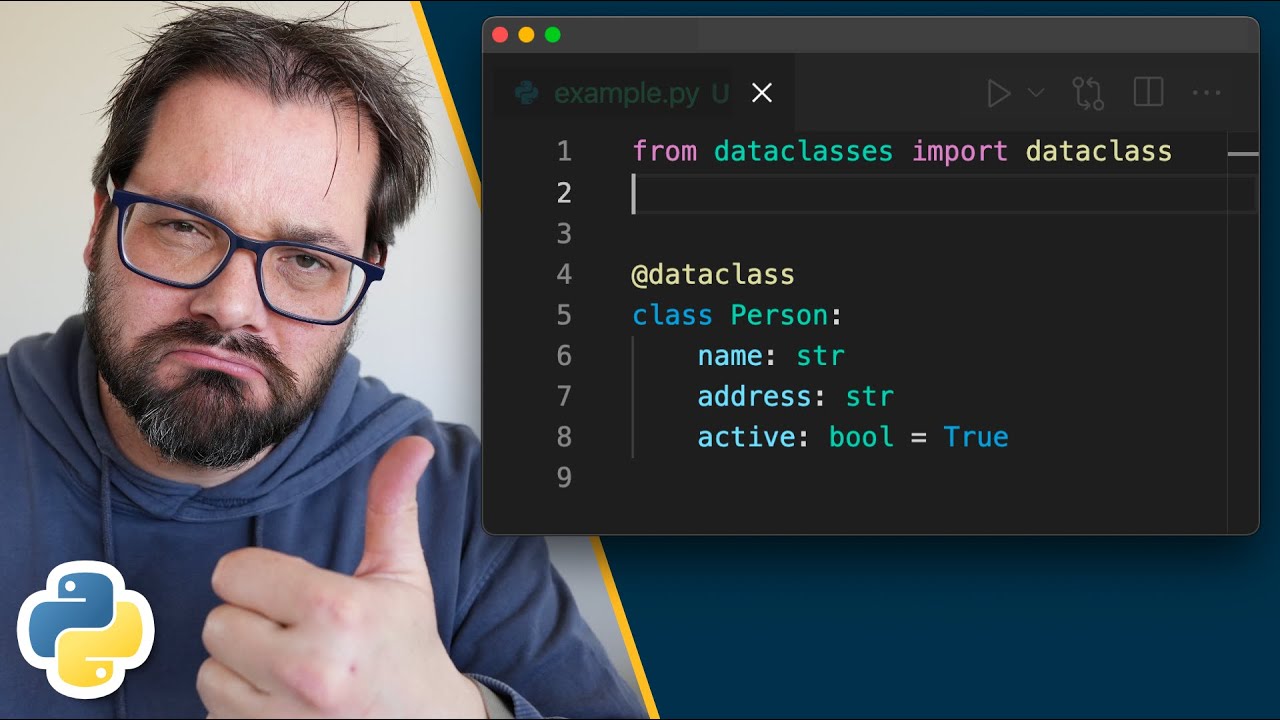
This Is Why Python Data Classes Are Awesome

Strategy Design Pattern Tutorial with Java Coding Example | Strategy Pattern Explained
5.0 / 5 (0 votes)