Javascript Design Patterns #2 - Singleton Pattern
Summary
TLDRThis video by Dev Sage explains the Singleton design pattern in JavaScript, which is a creational pattern used to limit the number of instances of a class to at most one. Using a scenario with a process manager handling multiple processes, the video demonstrates how to implement the Singleton pattern. It walks through creating a function to manage instances, ensuring only one process manager is created and reused. The tutorial is practical, with clear code examples and explanations, making it easy for viewers to understand and apply the Singleton pattern in their own projects.
Takeaways
- 💡 The singleton design pattern is a creational pattern used to limit the number of instances of an object to at most one.
- 🛠️ The scenario presented involves managing processes with only one process manager overseeing multiple processes.
- 🧑💻 A function called 'process' is created to represent a process, where the state (running, blocked, or stopped) is passed as an argument.
- 📝 To implement the singleton pattern, a variable called 'singleton' is created and set to an immediately invoked function expression (IIFE).
- 🏗️ Inside the IIFE, a 'process manager' object constructor is defined, initializing the 'num processes' property to 0.
- 📥 A 'createProcessManager' function is created to generate a new instance of the process manager, but it is kept private within the singleton scope.
- 🔍 The singleton exposes a method called 'getProcessManager' that checks if the process manager instance exists. If not, it creates and returns a new one.
- ✅ Multiple calls to 'getProcessManager' return the same instance of the process manager, ensuring that no additional instances are created.
- 🧪 To prove the pattern works, two process manager variables are created, and a console.log shows that both point to the same instance, returning 'true'.
- 📚 The singleton pattern is useful when limiting the number of object instances is necessary, such as when managing resources in an application.
Q & A
What is the Singleton design pattern in JavaScript?
-The Singleton design pattern is a creational pattern that ensures only one instance of an object is created and provides a global point of access to that instance.
Why would you use the Singleton pattern?
-The Singleton pattern is useful when you want to limit the number of instances of a class to one, such as in scenarios where only one instance of an object should manage processes or resources.
What scenario does the video use to explain the Singleton pattern?
-The video explains a scenario where a program manages multiple processes but only one Process Manager should control them. The Singleton pattern ensures there is only one Process Manager instance.
How is the Process Manager created using the Singleton pattern?
-The Process Manager is created using an immediately invoked function expression (IIFE) to encapsulate the constructor and ensure that only one instance of the Process Manager is created and returned when needed.
What is the purpose of the 'singleton' variable in the example?
-The 'singleton' variable stores the result of an immediately invoked function expression (IIFE), which contains the logic to limit the creation of the Process Manager instance to one.
How does the code check if an instance of the Process Manager already exists?
-The code checks if the variable 'P_manager' is null. If it is, it creates a new Process Manager instance. If not, it returns the existing instance.
What does the 'createProcessManager' function do?
-The 'createProcessManager' function creates and returns a new instance of the Process Manager, but it's only called if there isn't already an instance.
How can you confirm that only one instance of Process Manager is created?
-By creating two variables, 'processManager' and 'processManager2', and checking if they point to the same instance using 'console.log(processManager === processManager2)', which should return true.
What happens when the 'getProcessManager' method is called multiple times?
-When 'getProcessManager' is called multiple times, the first call creates a new Process Manager instance. Subsequent calls return the already created instance without creating a new one.
Why would you use the Singleton pattern in process management?
-In process management, you would use the Singleton pattern to ensure that only one Process Manager exists, preventing potential conflicts or mismanagement of resources caused by multiple managers.
Outlines
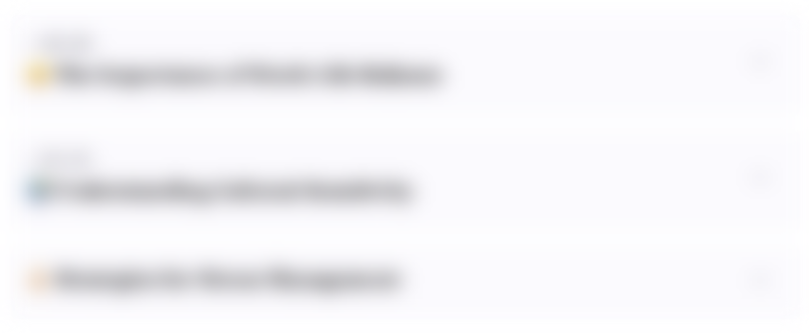
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
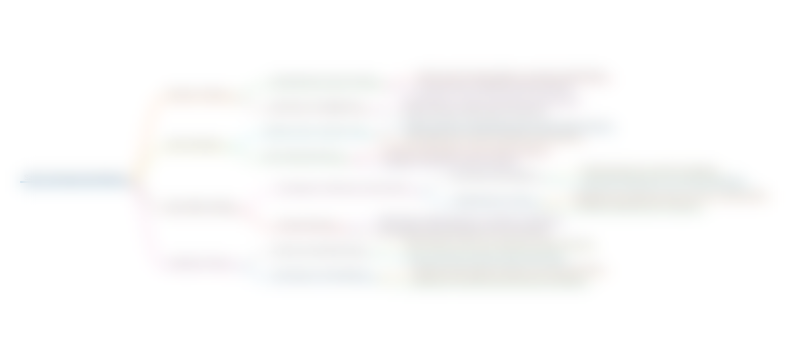
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
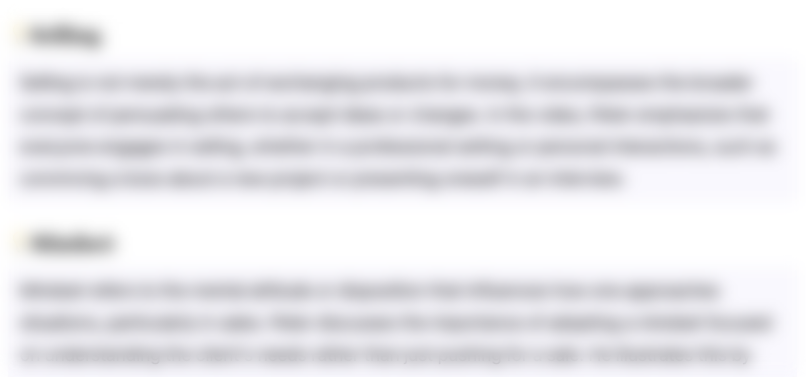
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
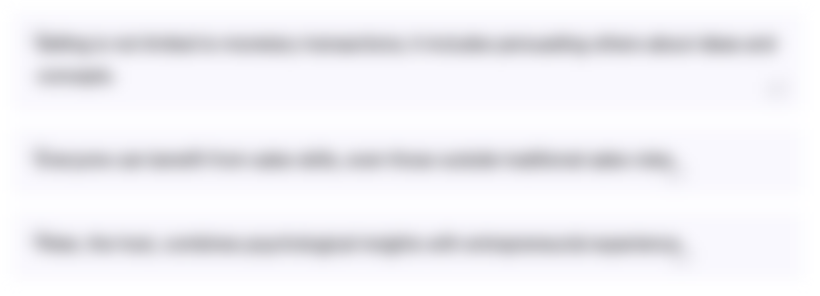
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
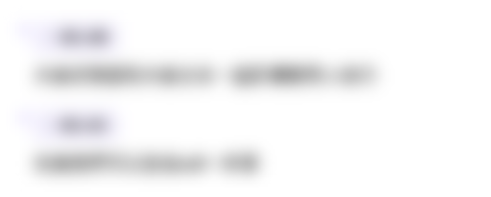
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

The Singleton Pattern Explained and Implemented in Java | Creational Design Patterns | Geekific
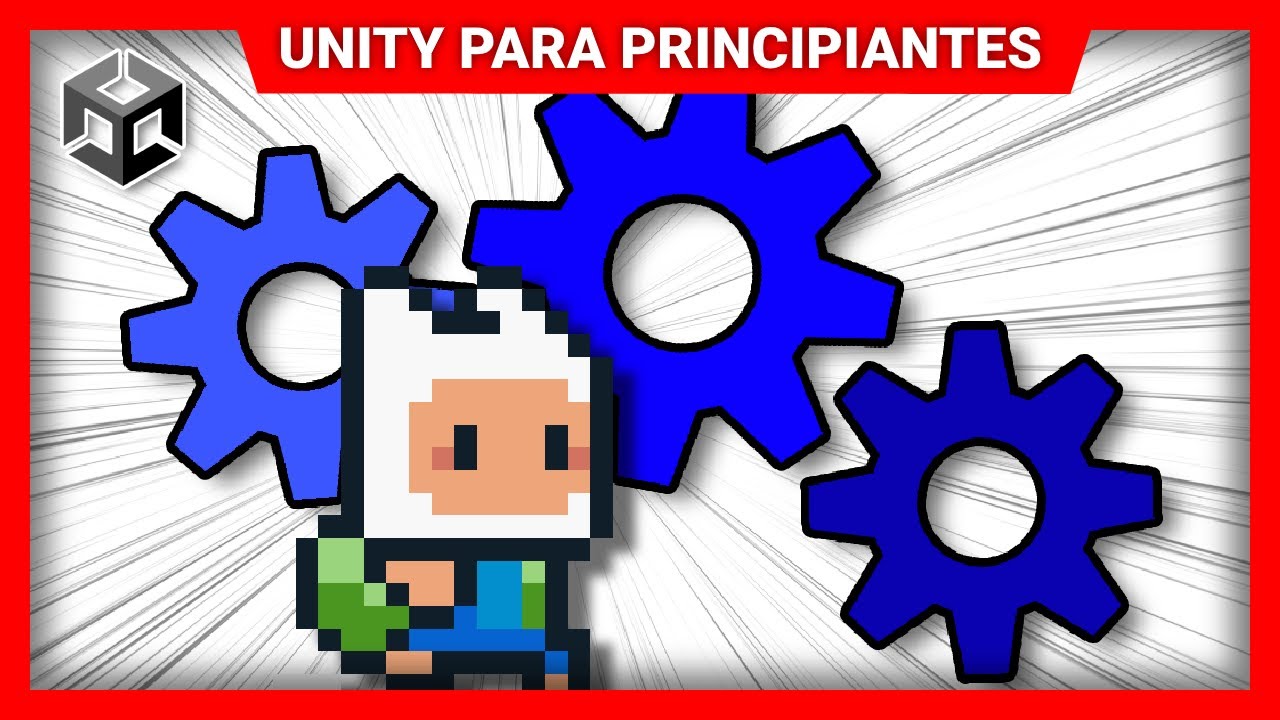
Cómo Hacer un Game Manager en Unity (Importante!) ► Unity para principiantes #010

The Volatile Keyword in Java Explained with Example | Interview Question | Multithreading |
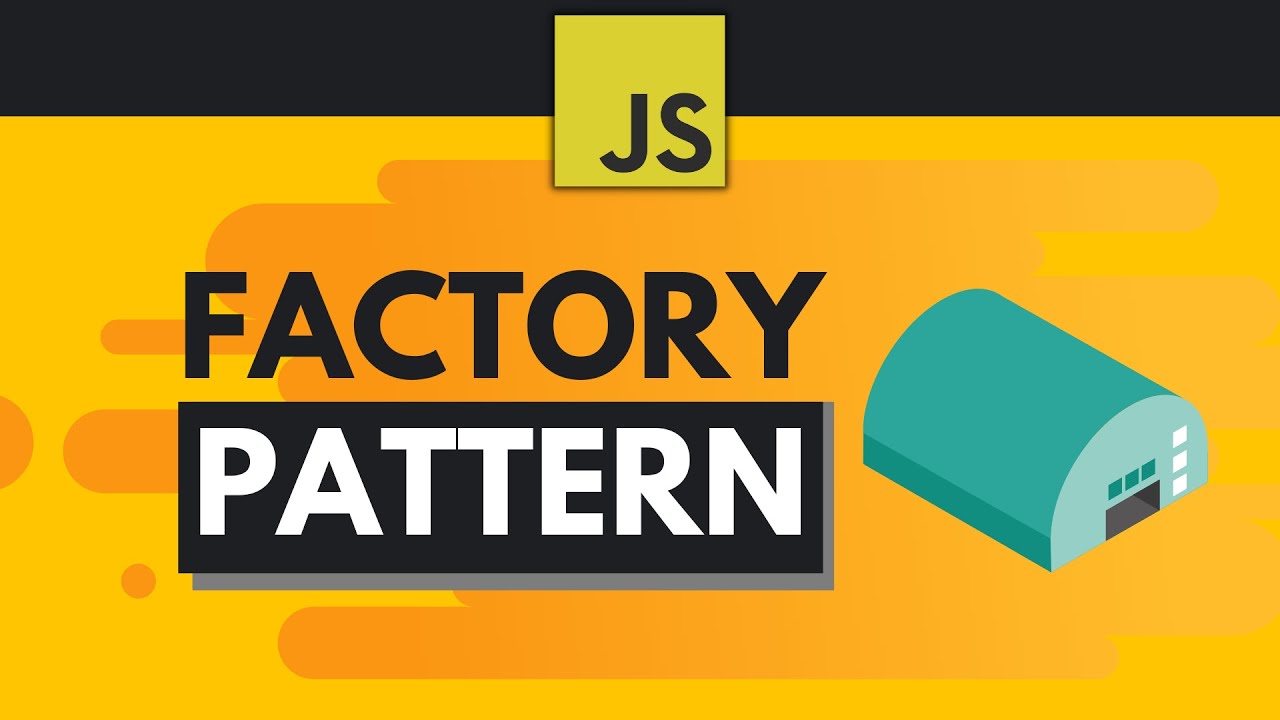
Javascript Design Patterns #1 - Factory Pattern

Lesson Three: The Basic 2 and 3 Patterns, Leonard Slatkin's Conducting School
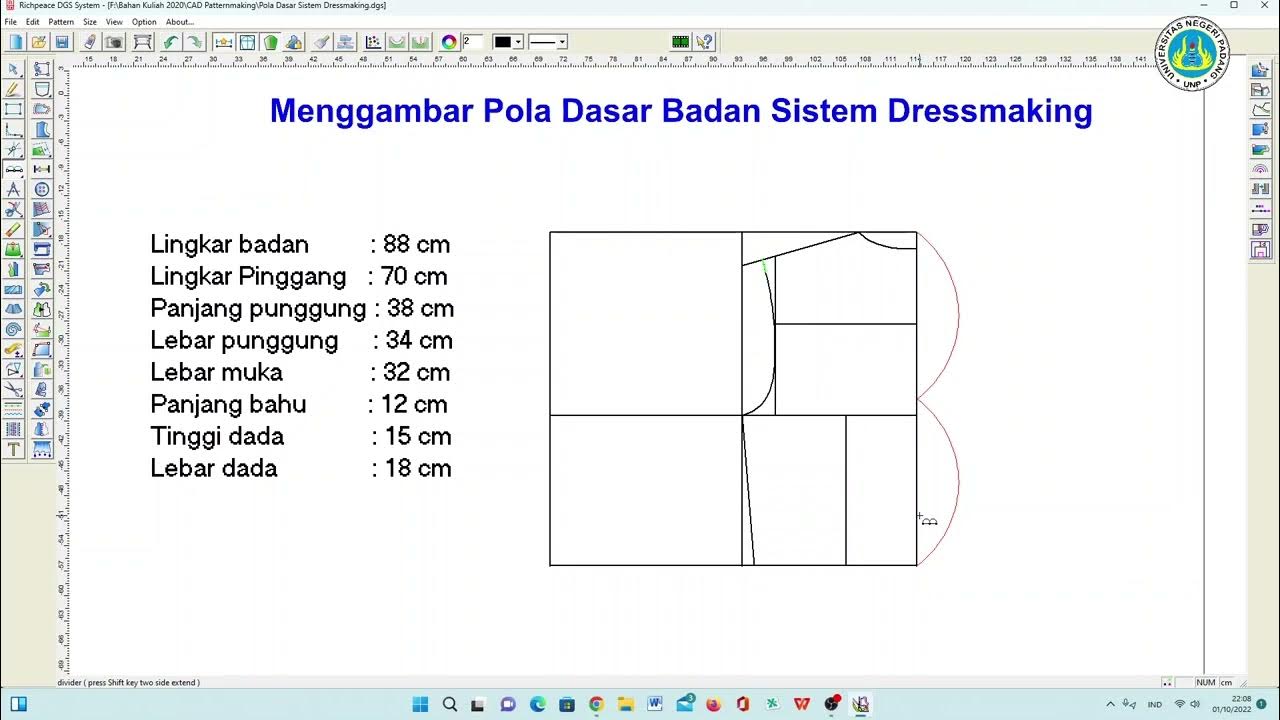
Cara Membuat Pola Dasar Badan Dressmaking dengan Richpeace
5.0 / 5 (0 votes)