Singleton Pattern – Design Patterns (ep 6)
Summary
TLDRIn this video, the concept of the Singleton design pattern is explored, covering its definition, implementation, and the potential drawbacks of using it. The Singleton pattern ensures that a class has only one instance, which is globally accessible. While it can be useful in some cases, the pattern has been criticized for promoting global variables, complicating testing, and making assumptions about future requirements. The video emphasizes that while the pattern can be intriguing from a technical standpoint, it's advisable to avoid overusing it and instead consider alternatives for more flexible design. The video concludes by encouraging further discussion on the topic.
Takeaways
- 😀 The Singleton pattern ensures a class has only one instance and provides a global point of access to it.
- 😀 Singleton pattern restricts the instantiation of a class to a single instance, which can be accessed globally.
- 😀 Many developers argue against using the Singleton pattern because it can lead to issues with global state management and control.
- 😀 Singleton pattern's global access can make it harder to manage and reason about code, as any part of the program can modify the global instance.
- 😀 It’s difficult to predict if you will only ever need a single instance of a class, which makes Singleton a risky design choice for growing applications.
- 😀 A major drawback of Singleton is that it can complicate unit testing, especially when mocking instances is required.
- 😀 The Singleton pattern makes testing difficult due to its static nature, as you may need to mock or create multiple instances for testing scenarios.
- 😀 The key feature of Singleton is making the constructor private, which prevents direct instantiation and forces the use of a global access method.
- 😀 The Singleton class uses a static method (e.g., `getInstance()`) to return the single instance of the class, ensuring the same object is used every time.
- 😀 The first call to `getInstance()` creates the instance, which is then stored in a static variable. Subsequent calls return the stored instance without recreating it.
- 😀 While Singleton may be technically interesting, it’s advised to avoid it in most cases due to its potential drawbacks in flexibility and testability.
Q & A
What is the main topic discussed in this video?
-The video discusses the Singleton pattern, which is one of the design patterns covered in the 'Head First Design Patterns' book.
What is the Singleton pattern used for?
-The Singleton pattern ensures that a class has only one instance and provides global access to it, meaning that only one instance of the class is ever created.
Why does the presenter mention that many people argue against using the Singleton pattern?
-The presenter highlights that the Singleton pattern is often considered a 'code smell' because it can lead to difficulties in maintaining control over the global state and complicates testing due to its reliance on a global instance.
What is one of the main critiques of the Singleton pattern mentioned in the video?
-One of the main critiques is that it introduces a global point of access to a class, which can cause problems when trying to maintain control over the scope and state of variables in the program.
What is a potential issue when trying to use Singleton in a growing application, according to the presenter?
-As an application grows, the assumption that only one instance of a class is needed may no longer hold true. For example, in a chat application, you might eventually need multiple chat rooms, which conflicts with the idea of a single global instance.
How does the Singleton pattern impact testing?
-The Singleton pattern makes testing difficult because it involves a global instance that is difficult to mock. In unit testing, it's often necessary to create multiple instances or mock the Singleton, which becomes problematic.
What does the presenter suggest as an alternative approach to the Singleton pattern?
-The presenter suggests that while it's fine to have a single instance of a class in your application, it's not a good idea to make it impossible to create multiple instances in the future, as you may need more than one instance over time.
What is the key feature of the Singleton class as explained in the video?
-The key feature is that the Singleton class has a private constructor, preventing external code from instantiating the class directly. Instead, a static method (getInstance) is used to return the single instance of the class.
How does the static method getInstance in the Singleton class work?
-The getInstance method checks if an instance of the Singleton class already exists. If it doesn't, it creates one and stores it in a static variable. The method then returns the single instance, ensuring only one instance is used throughout the application.
Why is making the constructor of the Singleton class private important?
-Making the constructor private ensures that no other class can directly instantiate the Singleton. This enforces the idea that only one instance of the class can exist, as it can only be created within the Singleton class itself.
Outlines
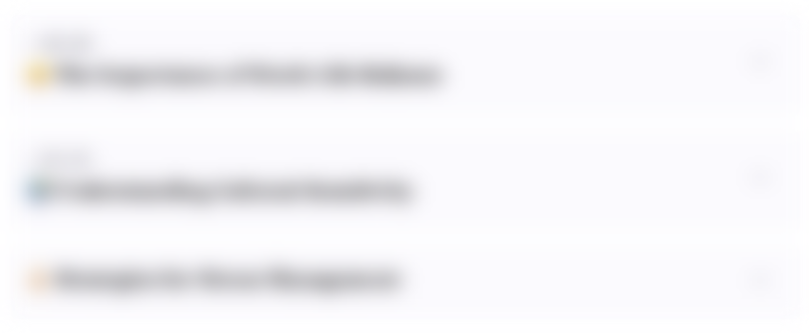
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
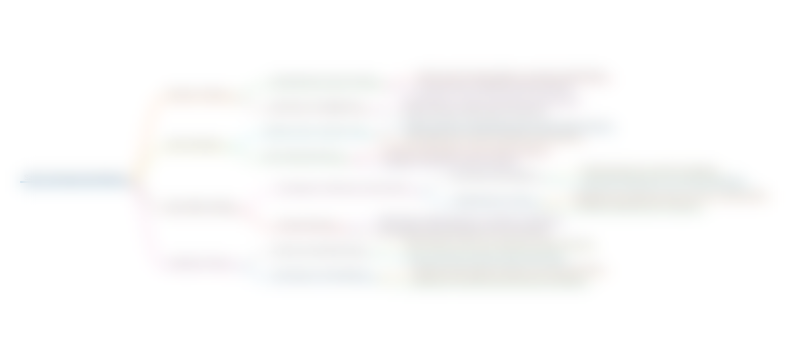
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
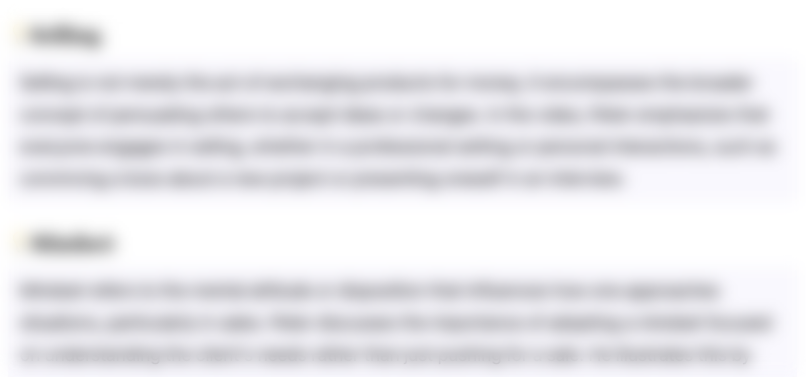
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
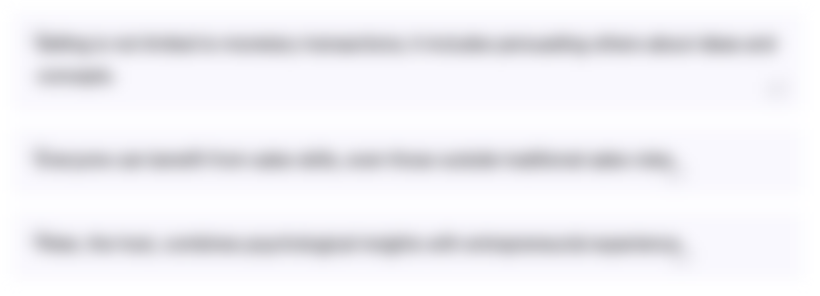
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
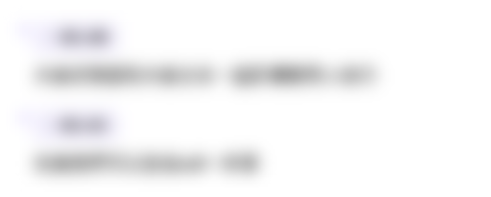
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

The Singleton Pattern Explained and Implemented in Java | Creational Design Patterns | Geekific
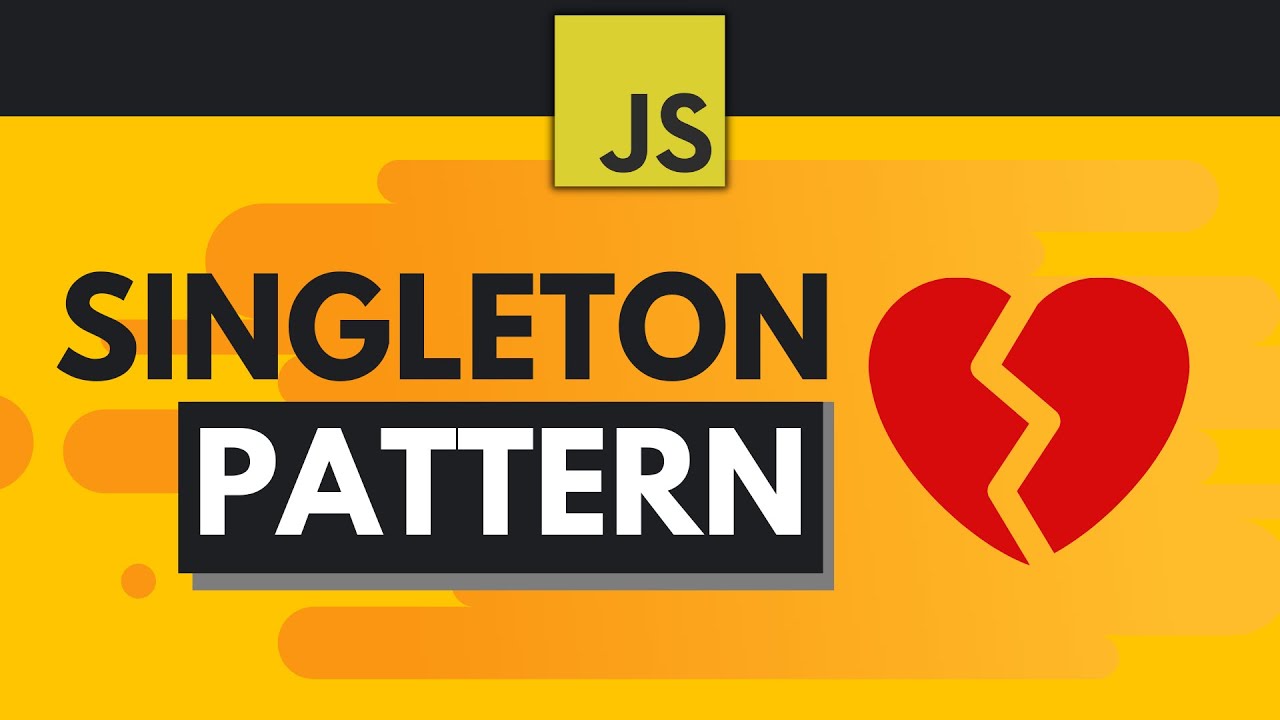
Javascript Design Patterns #2 - Singleton Pattern

Ep.3 - Singleton | Flutter Design Patterns
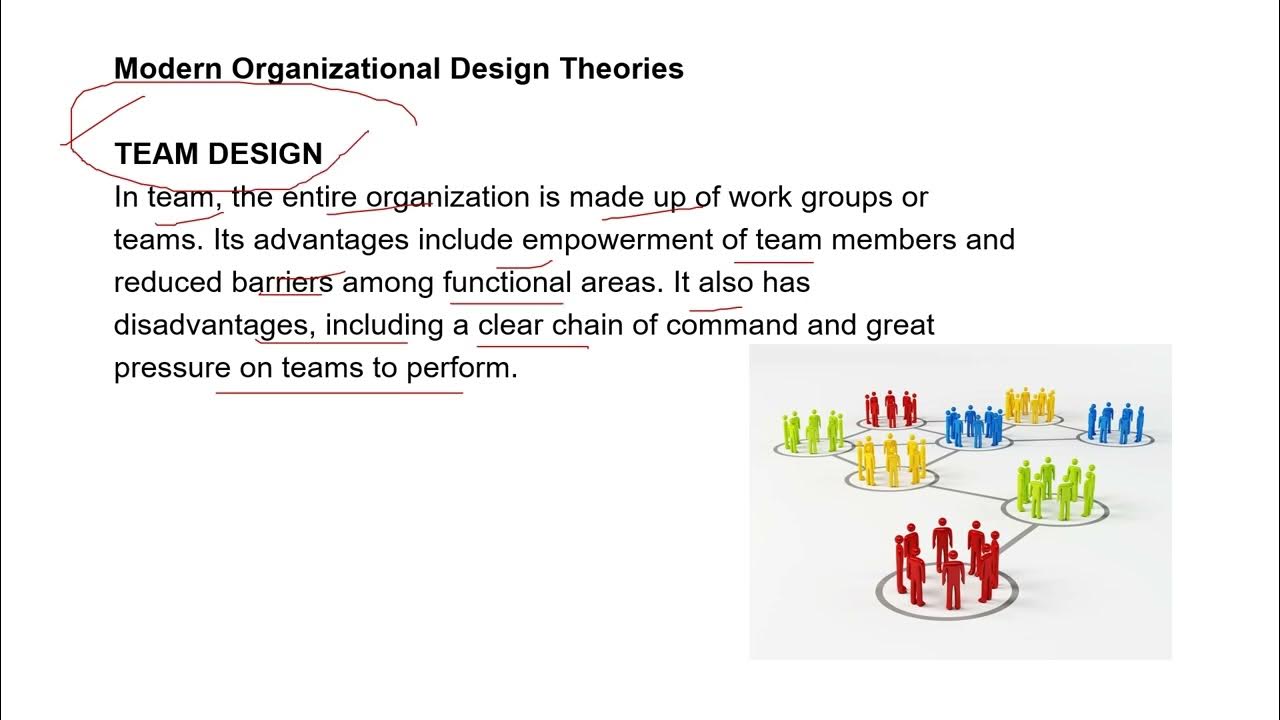
Organization Theories and Applications

Strategy Design Pattern Tutorial with Java Coding Example | Strategy Pattern Explained

Apa itu Desentralisasi?
5.0 / 5 (0 votes)