The Singleton Pattern Explained and Implemented in Java | Creational Design Patterns | Geekific
Summary
TLDRIn this video, the presenter introduces the Singleton Design Pattern, a crucial concept in software development. The Singleton ensures only one instance of a class exists throughout an application, offering a global point of access. The video explains the implementation steps, including how to manage multi-threading issues using synchronization and the double-checked locking idiom. It also touches on optimizing performance with the volatile keyword. This comprehensive guide walks viewers through the Singleton’s purpose, benefits, and real-world applications, providing practical insights for developers working with shared resources.
Takeaways
- 😀 The Singleton Pattern is a **creational design pattern** that ensures only one instance of a class exists throughout an application.
- 😀 A real-world analogy for the Singleton is the **government or president** of a country, where only one instance (e.g., one president) can exist at a time.
- 😀 The Singleton Pattern ensures a **single point of access** to its instance, allowing different parts of the application to interact with the same object.
- 😀 The pattern is useful when you want to control **resource usage**, such as with database connections, by ensuring only one instance is created.
- 😀 To implement a Singleton, you create a **private static field** to hold the instance and a **private constructor** to prevent direct instantiation.
- 😀 A public static method like `getInstance()` is used to **return the Singleton instance**, ensuring only one instance exists throughout the application.
- 😀 In multi-threaded environments, you must address the issue of **multiple threads accessing the Singleton** at the same time and creating multiple instances.
- 😀 The solution to this multi-threading issue is to use a **synchronized block** within the `getInstance()` method, ensuring only one thread can initialize the Singleton at a time.
- 😀 The **double-checked locking** technique optimizes synchronization by checking if the instance is already created before acquiring the lock, improving performance.
- 😀 To handle **memory visibility issues** in multi-threaded environments, mark the Singleton instance as **volatile**, preventing threads from using partially constructed instances.
- 😀 For further performance improvements, use a **local variable** to store the Singleton instance after the first memory read, avoiding multiple reads from memory and improving efficiency.
Q & A
What is the Singleton Pattern in design?
-The Singleton Pattern is a creational design pattern that ensures only one instance of a class exists and provides a single point of access to it from anywhere in the application.
Why would someone want to control the number of instances of a class?
-Controlling the number of instances ensures resource efficiency. For example, a database connection should only have one instance shared across the application to prevent unnecessary overhead and errors caused by multiple connections.
What real-world analogy helps explain the Singleton Pattern?
-A real-world analogy is the government or president of a country. There can only be one official government or one official president at any time, similar to how a Singleton class guarantees only one instance.
How does the Singleton class ensure only one instance is created?
-The Singleton class ensures only one instance by using a private static field that stores the instance. A public static method, typically named getInstance(), checks if the instance is null and creates it if necessary, returning the same instance every time.
What is the role of the 'private' constructor in a Singleton class?
-The 'private' constructor ensures that the class cannot be instantiated directly outside of the class. It forces the use of the public static method to access the instance, ensuring controlled access to the class.
Why is synchronization necessary in a multi-threaded environment for Singleton pattern?
-In a multi-threaded environment, synchronization prevents multiple threads from creating different instances of the Singleton at the same time, which could result in inconsistent or incorrect behavior.
What is double-checked locking and why is it used in Singleton implementation?
-Double-checked locking is a technique used to optimize performance by ensuring that synchronization is only applied when the Singleton instance is being created. It first checks if the instance is null without locking, and only acquires the lock if necessary.
What issue can arise when using double-checked locking without the 'volatile' keyword?
-Without the 'volatile' keyword, there's a risk that a thread could see a partially constructed instance due to the way the compiler handles memory, causing other threads to use an invalid instance and potentially crashing the application.
How does marking the Singleton instance as 'volatile' solve the problem in multi-threaded environments?
-The 'volatile' keyword ensures that changes to the Singleton instance are immediately visible to all threads, preventing threads from using a partially constructed instance and ensuring the Singleton instance is correctly shared across threads.
What is the performance optimization mentioned for the Singleton pattern?
-The performance optimization involves using a local variable to store the Singleton instance after it is first retrieved, which reduces the need for multiple direct memory reads, improving the overall performance by up to 40%.
Outlines
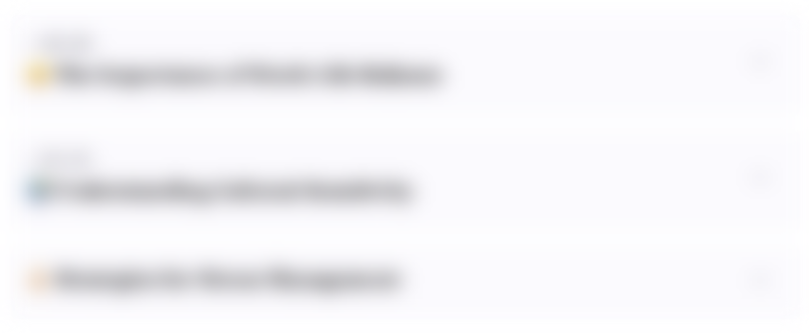
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
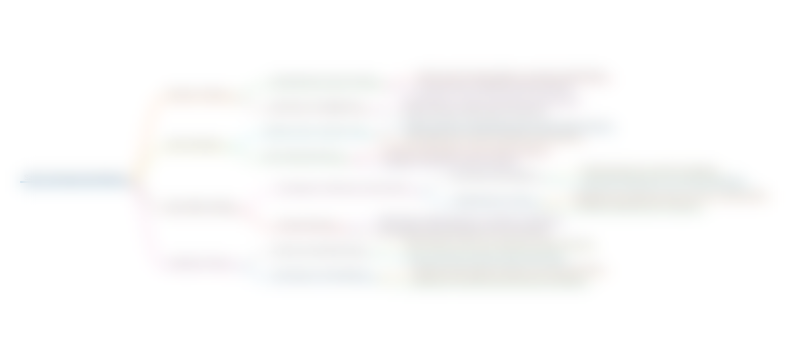
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
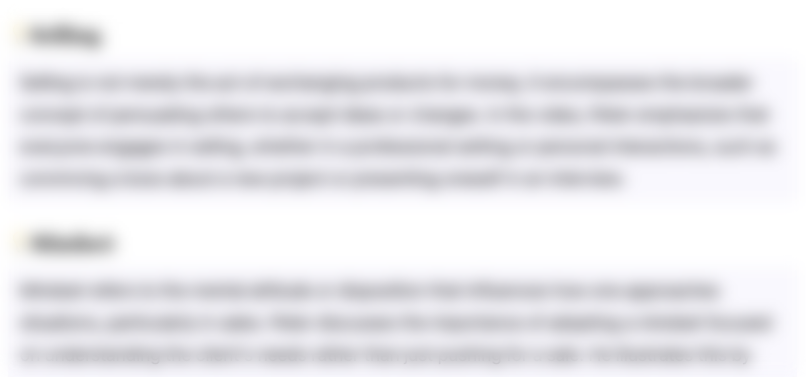
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
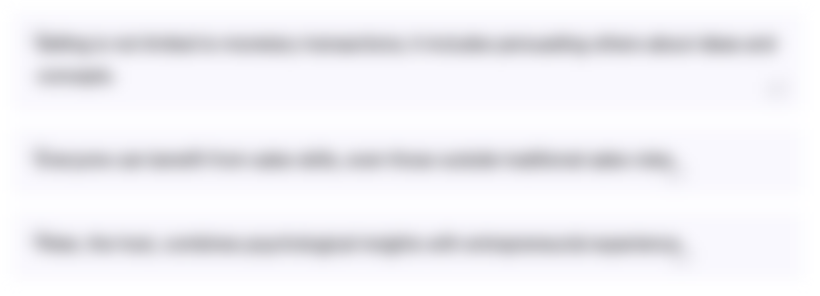
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
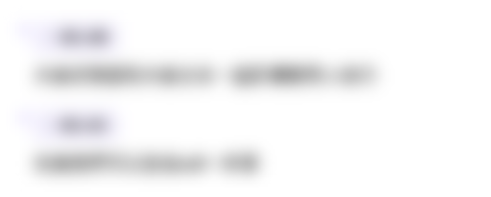
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)