List Part 2
Summary
TLDRThis lecture delves into list manipulation in Python, teaching how to modify, add, and remove elements. It covers direct index assignment for modification, using the 'append' method to add elements or lists, and the 'insert' function for position-specific additions. The session also explains removal techniques, including 'del' for specified index removal, 'remove' for first-match deletion, and 'pop' for displaying and removing elements at given indices, all illustrated with clear examples.
Takeaways
- 📝 Modifying Lists: The lecture covers how to alter elements in a list by assigning new values using index positions or built-in functions.
- 🔍 Indexing: Lists can be modified at both the top level and sublevel components using specific index values.
- 🔑 Top Level Change: Demonstrated how to change the value of the 'number of employees' in a list by directly accessing its index.
- 🔑 Sublevel Change: Showed the process of altering a specific employee's name within a nested list structure.
- 📚 Built-in Functions: Python provides several built-in functions like 'append', 'insert', 'del', 'remove', and 'pop' for list manipulation.
- 📌 Append Method: Explained how to use 'append' to add elements to the end of a list or concatenate a list with another.
- 📍 Insert Function: Described the 'insert' function to add elements at a specific position within a list.
- 🗑️ Deletion Techniques: Covered three methods to remove elements from a list: 'del' for removing by index, 'remove' for the first occurrence, and 'pop' to remove and return an element at a specific index.
- 🔄 List Concatenation: Illustrated adding a new list to an existing list using the 'append' method to create a multi-level list.
- 📈 Practical Examples: Provided practical examples of modifying a list with employee details, including IDs, names, and ages.
- 👋 Conclusion: Summarized the main points on list manipulation, emphasizing the use of index numbers and built-in functions for adding and removing elements.
Q & A
How can you modify the elements of a list in Python?
-You can modify the elements of a list in Python by directly assigning a new value to a specific index or by using built-in functions like 'append', 'insert', 'remove', and 'pop'.
What is the purpose of assigning a new value to an index in a list?
-Assigning a new value to an index in a list allows you to change or update the value of an element at a specific position within the list.
Can you explain the concept of top-level and sub-level components in a list?
-In a list, top-level components refer to the main elements of the list, while sub-level components refer to elements within those top-level elements, essentially creating a nested list structure.
How do you change the number of employees in a list using indexing?
-To change the number of employees, you would assign a new value to the index that corresponds to the 'number of employees' in the list, for example, `employee_list[2] = 5`.
What does the 'append' method do in Python lists?
-The 'append' method adds an object to the end of the list. If the index is not specified, the object is added at a new level in the existing list.
Can you add a list to another list using the 'append' method?
-Yes, you can add a list to another list using the 'append' method, which is also known as concatenation of lists.
How does the 'insert' function differ from 'append'?
-The 'insert' function adds an object at a specified position in the list, whereas 'append' always adds the object to the end of the list.
What are the different methods to remove elements from a list in Python?
-The methods to remove elements from a list in Python are 'del', 'remove', and 'pop'. 'del' removes an element at a specified index, 'remove' removes the first matching element, and 'pop' removes and returns the element at a specified index.
How does the 'remove' function work in Python lists?
-The 'remove' function searches for the first occurrence of a specified object in the list and removes it.
What does the 'pop' function return when removing an element from a list?
-The 'pop' function not only removes the element at the specified index but also returns that element.
Can you provide an example of how to use the 'del' statement to remove a sublist from a list?
-To remove a sublist, you would use the 'del' statement followed by the list name and the index of the sublist, for example, `del employee_list[3]` to remove the 'age' sublist from the 'employee_list'.
Outlines
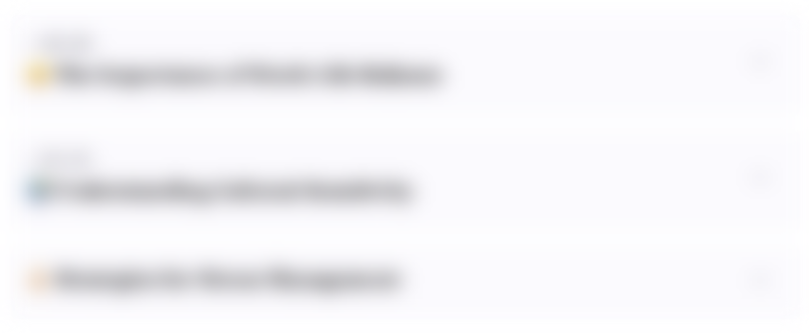
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
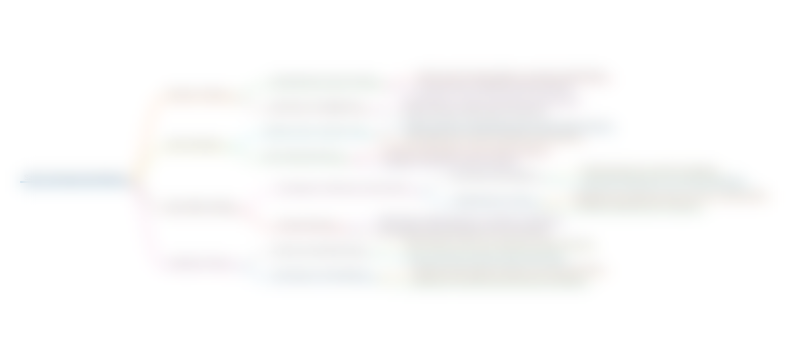
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
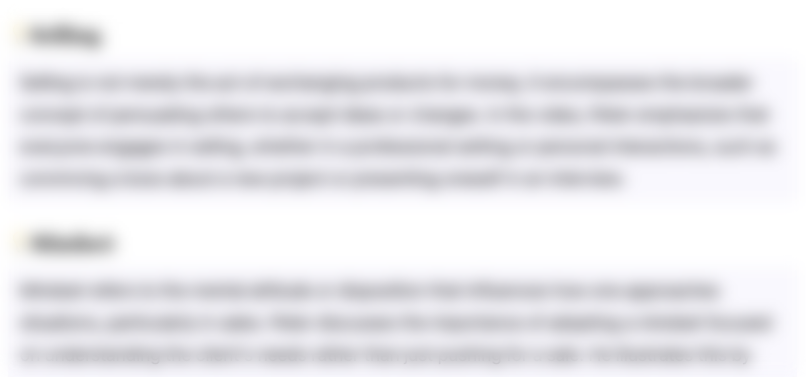
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
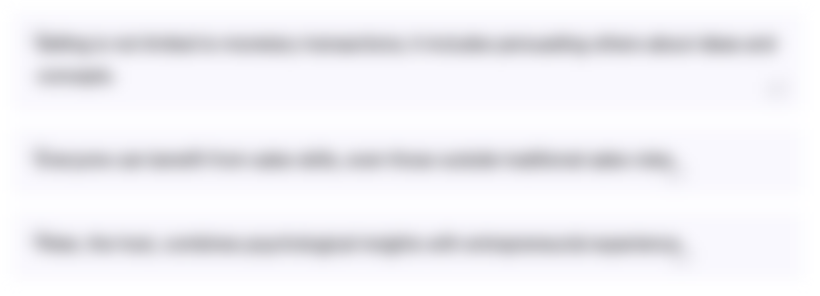
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
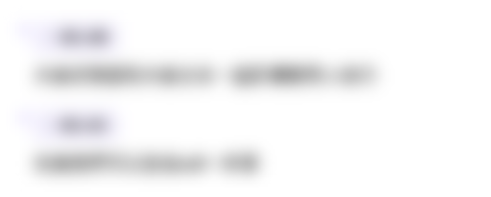
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)