COS 333: Chapter 16, Part 3
Summary
TLDRThis lecture delves into logic programming languages, focusing on Prolog's basic elements, including arithmetic operations and list structures. It explores examples of list manipulation and discusses Prolog's limitations, such as resolution order control and the closed world assumption. The session also touches on practical applications of logic programming in databases, expert systems, and natural language processing, providing insights into the real-world uses of these languages.
Takeaways
- 📚 The lecture discusses Prolog, a logic programming language, and its basic elements, list structures, and real-world applications.
- 🔢 Prolog supports integer arithmetic operations using the 'is' operator, but avoids floating-point arithmetic due to potential issues in the resolution process.
- 🚗 The script provides an example of implementing a 'speed' and 'time' relation in Prolog and how to use these to calculate 'distance' with a query.
- 📝 Lists in Prolog are similar to those in Lisp and Scheme, allowing for nested structures and terminated by an empty list.
- 🔑 Prolog uses a recursive approach for list processing, requiring careful ordering of base and recursive cases for efficient execution.
- 🔄 The 'append' and 'reverse' propositions in Prolog are implemented recursively, demonstrating the language's capability for list manipulation.
- 🚫 Prolog has certain deficiencies, including issues with resolution order control, the closed world assumption, the negation problem, and intrinsic limitations in computations.
- ✂️ 'Cuts' in Prolog are used to control backtracking and can improve efficiency by preventing unnecessary search steps.
- 🔍 The 'not' operator in Prolog is used to handle logical negations, but it does not function as a true logical negation due to the closed world assumption.
- 🔑 Practical applications of logic programming include use in relational database management systems, expert systems, and natural language processing.
- 📈 The lecture concludes with a discussion on the practical uses of logic programming, highlighting its role in intelligent query systems and AI.
Q & A
What is the primary focus of the third lecture on logic programming languages?
-The primary focus of the third lecture is to conclude the discussion on Prolog, covering topics such as basic elements of Prolog, simple arithmetic operations, list structures, general deficiencies of Prolog, and application areas of logic programming in the real world.
Why are floating point values and arithmetic not emphasized in Prolog?
-Floating point values and arithmetic are not emphasized in Prolog because floating point values often do not represent exact fractional values, which can result in unexpected failures during the matching process performed by resolution.
How does Prolog support integer arithmetic?
-Prolog supports integer arithmetic through the use of the 'is' operator, which has two operands and uses regular infix notation for arithmetic expressions, similar to languages like C++ or Java.
What is the significance of the 'is' operator in Prolog?
-The 'is' operator in Prolog is used to instantiate a variable with the value of an arithmetic expression. It is important to note that the 'is' operator is not an assignment statement and has specific rules about variable instantiation on both its left and right operands.
Can you explain the concept of facts and rules in Prolog using the example of speed and time relations?
-In Prolog, facts are used to define relations with parameters, such as the speed and time of vehicles. For example, facts like 'speed(ford, 100)' and 'time(ford, 20)' define the speed and time relations for a vehicle named 'ford'. Rules, on the other hand, can define more complex relations, like the distance traveled, using variables and other relations.
How does Prolog handle list structures?
-Prolog handles list structures similarly to Lisp and Scheme, allowing for the creation of nested lists containing atoms, atomic propositions, and other terms. Lists are implicitly terminated by an empty list and can be manipulated using head and tail notation.
What is the general approach for implementing list processing in Prolog?
-List processing in Prolog generally requires a recursive approach, working through lists one element at a time. This involves defining base cases and recursive cases as separate Prolog statements, with the base cases often being atomic propositions and the recursive cases being rules.
What is the purpose of the underscore character in Prolog?
-The underscore character in Prolog represents an anonymous variable, indicating that the programmer does not care about the instantiation of that variable during the unification process. It is used to simplify Prolog implementations, particularly in list processing where certain values may not be relevant.
Can you provide an example of a Prolog proposition that performs list manipulation?
-An example of a Prolog proposition that performs list manipulation is the 'member' proposition, which defines a relation between a term and a list. It checks if the term is contained within the list using a recursive strategy with a base case (when the term is the head of the list) and a recursive case (when the term is a member of the tail of the list).
What are some of the deficiencies associated with the Prolog programming language?
-Some of the deficiencies associated with Prolog include resolution order control, the closed world assumption, the negation problem, and intrinsic limitations with certain kinds of computations that Prolog cannot perform efficiently.
How does Prolog handle the sorting of lists?
-Prolog handles the sorting of lists by generating permutations of the original list and checking if each permutation is sorted. This process can be very inefficient as it may require generating every possible permutation of the list to find a sorted version.
What are some practical application areas for logic programming languages?
-Logic programming languages have practical applications in relational database management systems for implementing intelligent query systems, in expert systems for decision making based on a set of rules, and in natural language processing within the domain of artificial intelligence.
Outlines
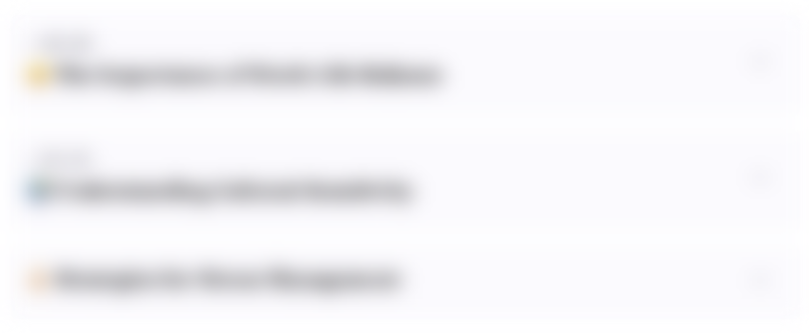
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
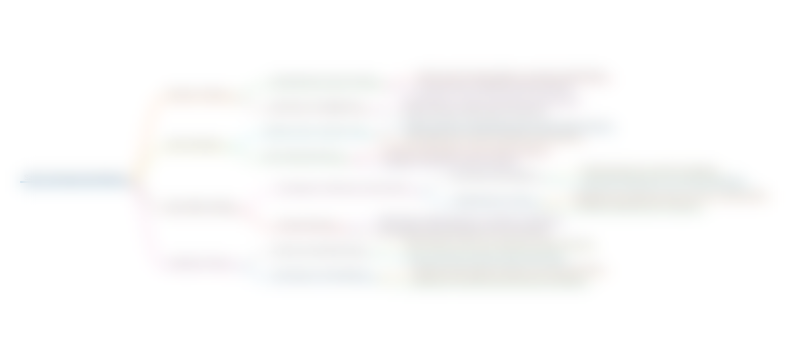
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
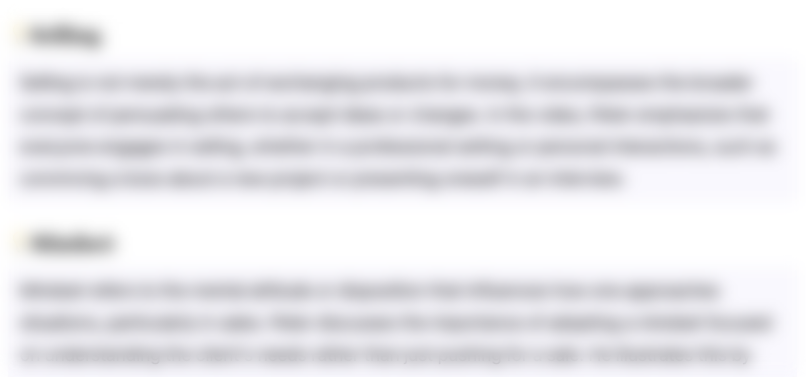
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
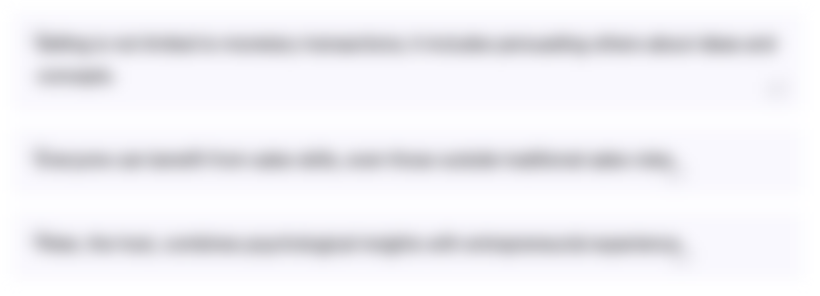
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
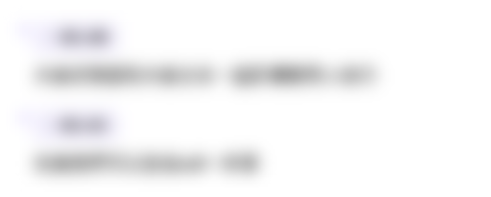
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)