Lists Part- 1
Summary
TLDRThis lecture introduces the concept of lists as an ordered data structure, allowing elements of different data types. It demonstrates creating lists with employee ID and names, and combining them into an employee list. The script explains positive and negative indexing in Python, showcasing how to access both top-level and sub-level components within a list, providing a clear guide to list manipulation and access.
Takeaways
- 📚 Lists are a data structure consisting of an ordered collection of objects.
- 🔢 Objects in a list, also called elements or components, can be of different data types.
- 🔲 Elements inside a list are enclosed between square brackets.
- 📝 Example lists include employee ID and employee name, with values separated by commas.
- 👥 An employee list can be created using employee ID, employee name, and the number of employees.
- 📋 Lists in Python can be viewed using the print function.
- ➡️ Positive indexing starts from the leftmost element with an index of 0 and goes up to n-1.
- ⬅️ Negative indexing starts from the rightmost element with an index of -1 and continues backwards.
- 🔍 Accessing components in a list can be done using single slicing for top-level components and double slicing for sub-level components.
- 🗂️ Example commands for accessing elements include using indices within square brackets.
Q & A
What is a list in the context of data structures?
-A list is a data structure consisting of an ordered collection of objects, where the objects can be of different data types and are enclosed between square brackets.
How can elements in a list be referred to?
-Elements in a list can be referred to as elements, objects, or components of the list.
What are the different data types that can be included in a list?
-A list can include elements of various data types such as arrays, vectors, numeric matrices, logical values, complex vectors, etc.
How is a list created in Python?
-A list in Python is created by enclosing a set of values within square brackets, separated by commas.
Can you provide an example of creating a list for employee IDs?
-An example of creating a list for employee IDs would be using the list name 'id' with values [1, 2, 3, 4].
What is the purpose of creating a variable for the number of employees?
-Creating a variable for the number of employees helps in keeping track of the total count of employees and can be used for various operations related to the employee list.
What command is used to view the contents of a list in Python?
-The 'print' command is used in Python to view the contents of a list.
What are the two types of indexing in Python?
-The two types of indexing in Python are positive indexing, which starts from the leftmost element with index 0, and negative indexing, which starts from the rightmost element with index -1.
How does positive indexing work in Python?
-Positive indexing in Python starts from the leftmost element and the first index is 0, continuing up to n-1 where n is the length of the list.
How does negative indexing work in Python?
-Negative indexing in Python starts from the rightmost element with an index of -1, and continues with decreasing values towards the leftmost element.
How can you access a specific element in a list using indexing?
-To access a specific element in a list, you use the index of that element within square brackets following the list name. For example, 'list_name[index_value]'.
What is the difference between accessing top-level and sub-level components in a list?
-Accessing top-level components in a list is done using a single set of square brackets with the index of the component. Sub-level components require an additional set of square brackets to specify the index within the nested structure.
Can you provide an example of accessing a sub-level component in a list?
-An example of accessing a sub-level component would be accessing an employee's name from an employee list. If 'employee_list' is the top-level list and 'employee_name' is a sub-level list, you would access it as 'employee_list[1][1]' where 1 is the index of 'employee_name' and the second 1 is the index of the specific name within 'employee_name'.
How does the script summarize the key points covered about lists in Python?
-The script summarizes by explaining how to create a list, the concept of positive and negative indexing in Python, and how to access both top-level and sub-level components within a list.
Outlines
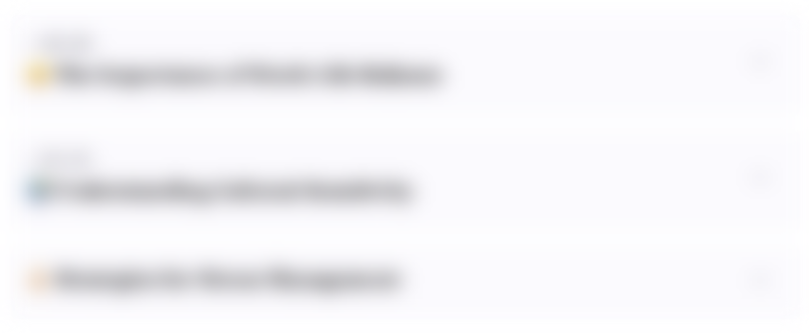
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
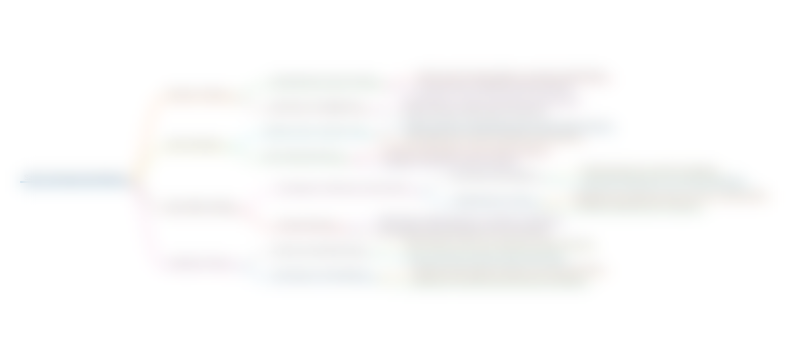
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
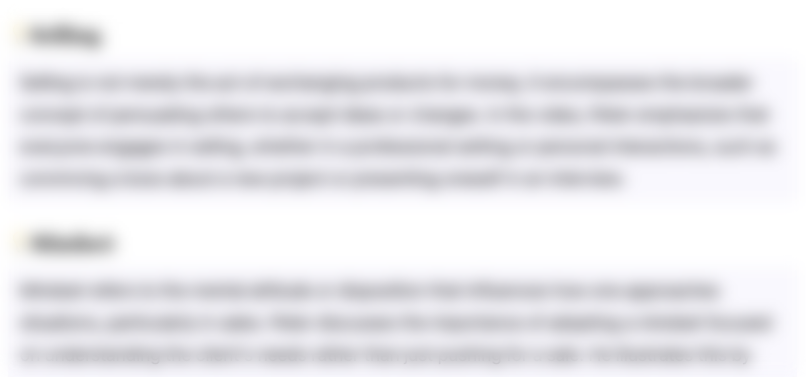
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
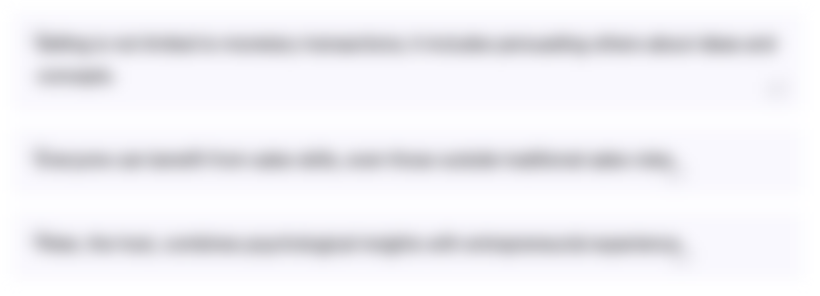
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
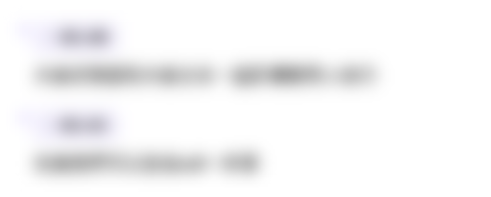
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)