Lecture 7 : DOM (Part 2) | Document Object Model | JavaScript Full Course
Summary
TLDRIn this engaging lecture on JavaScript, the instructor delves into the crucial concept of the Document Object Model (DOM). The session covers DOM manipulation, including how to access and modify attributes of HTML elements like divs and paragraphs. Practical examples illustrate how to create, insert, and delete elements dynamically using JavaScript methods such as append, prepend, and remove. The instructor also emphasizes the importance of styling elements and introduces the concept of class lists for managing multiple classes. This comprehensive overview sets the stage for further exploration of events in upcoming lectures.
Takeaways
- π Chapter 6 focuses on the important concept of the Document Object Model (DOM) and its applications in JavaScript.
- π The DOM allows developers to manipulate HTML elements dynamically using JavaScript.
- π οΈ Attributes can be added to elements, such as IDs and classes, which provide additional information about the elements.
- π» JavaScript methods like `getAttribute` and `setAttribute` are used to access and modify element attributes.
- π¨ Styles can be applied to elements using the `style` property in JavaScript, allowing for dynamic visual changes.
- π New elements can be created using `document.createElement` and inserted into the DOM with methods like `append`, `prepend`, and `insertBefore`.
- β Elements can be removed from the DOM using the `remove` method, allowing for dynamic updates to the page.
- π The `classList` property is useful for managing multiple classes on an element without overwriting existing ones.
- π Inline styles have the highest priority in CSS, but it's generally better to use external stylesheets for maintainability.
- π The next chapter will introduce event handling, allowing for interactive features like button clicks and form submissions.
Q & A
What is the main topic discussed in chapter six of the JavaScript series?
-Chapter six focuses on an important concept called the Document Object Model (DOM), explaining what it is, how to use it, its purpose, and how it is generated.
How can you access an element's attributes using JavaScript?
-You can access an element's attributes using JavaScript by first selecting the element and then using the 'getAttribute' method. For example, if you want to access the 'id' attribute of a div, you would call 'getElementById' followed by 'getAttribute' and specify the attribute you want.
What is the purpose of the 'id' attribute in HTML elements?
-The 'id' attribute in HTML elements serves as a unique identifier for that element. It is used for various purposes such as CSS styling, JavaScript manipulation, and linking purposes.
How do you add a new attribute to an element in JavaScript?
-To add a new attribute to an element in JavaScript, you can use the 'setAttribute' method on the selected element, specifying the attribute name and the value you want to set.
What is the difference between 'get_attribute' and 'setAttribute' in JavaScript?
-The 'getAttribute' method is used to retrieve the value of a specified attribute of an element, whereas 'setAttribute' is used to set a new value for an existing attribute or to add a new attribute to an element.
How can you change the style of an element dynamically using JavaScript?
-You can change the style of an element dynamically by accessing the 'style' property of the element and modifying its CSS properties. For example, to change the background color, you would write 'element.style.backgroundColor = 'color';'.
What is the 'classList' property in JavaScript and how is it used?
-The 'classList' property in JavaScript is a collection of class attributes of an element. It allows you to manipulate classes, such as adding, removing, or toggling them. It has methods like 'add', 'remove', and 'contains' to manage the classes.
How do you insert a new element into the DOM using JavaScript?
-You can insert a new element into the DOM using JavaScript by creating the element with 'document.createElement', setting its attributes and content, and then using methods like 'appendChild' or 'insertBefore' to place it in the desired location.
What is the 'createElement' method used for in JavaScript?
-The 'createElement' method in JavaScript is used to create a new HTML element with the specified tag name. It returns an Element object that can then be added to the document.
How can you append a newly created element to an existing element in the DOM?
-To append a newly created element to an existing element in the DOM, you can use the 'appendChild' method on the parent element, passing the newly created element as an argument.
What is the role of 'querySelector' and 'getElementById' in selecting elements in JavaScript?
-Both 'querySelector' and 'getElementById' are used to select elements in JavaScript. 'querySelector' allows you to select elements using CSS selectors, while 'getElementById' specifically selects an element by its 'id' attribute.
Outlines
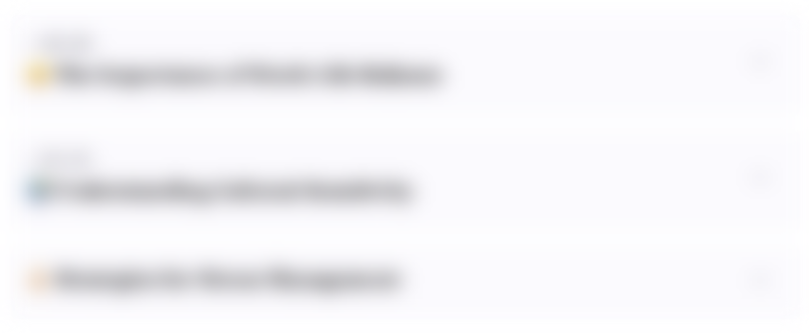
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
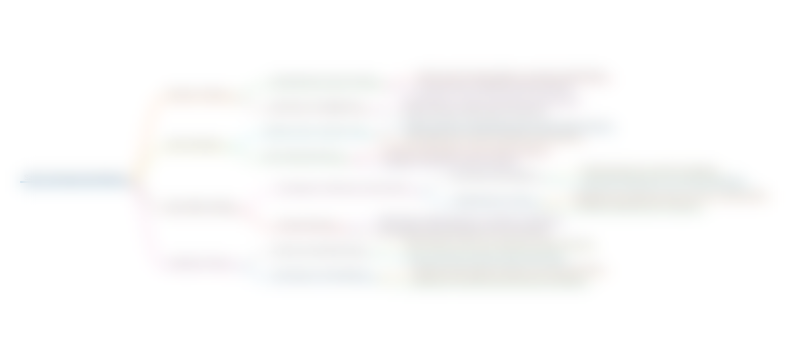
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
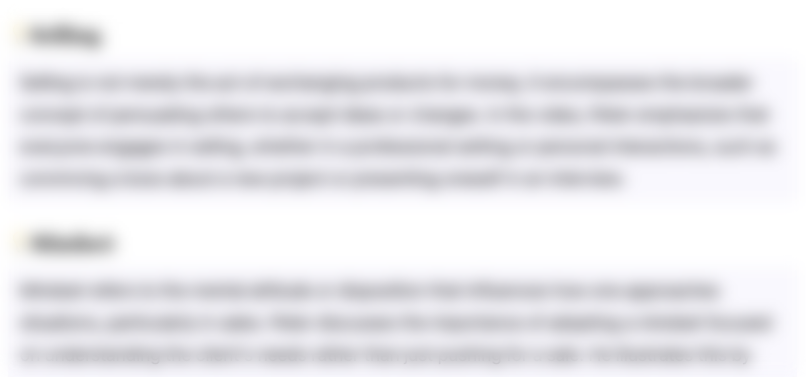
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
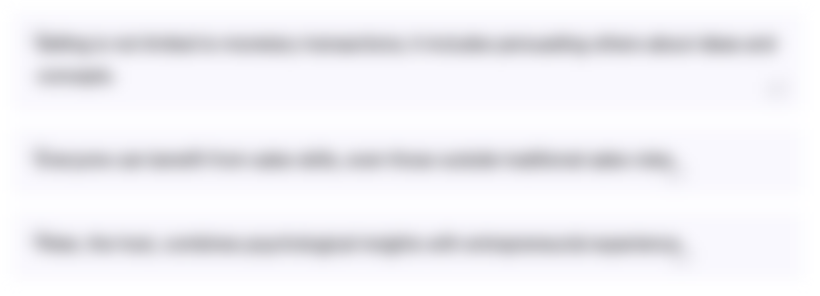
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
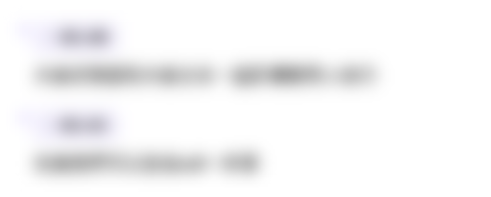
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)