COS 333: Chapter 11, Part 1
Summary
TLDRThis lecture delves into abstract data types (ADTs) and encapsulation, fundamental concepts in programming languages. It explains abstraction as a simplified representation focusing on significant attributes, integral to high-level languages that hide lower-level details. ADTs are user-defined, grouped in syntactic units with hidden representations, ensuring data integrity and simplifying program organization. The lecture also discusses design issues for ADT support in languages, using C++ as an example to illustrate class-based encapsulation, constructors, destructors, and the importance of separating interface from implementation.
Takeaways
- π The lecture introduces the concepts of Abstract Data Types (ADTs) and encapsulation within the context of programming languages.
- π― Abstraction in programming is about providing a summarized view of an entity that includes only the most significant attributes, hiding the lower-level details.
- ποΈ High-level programming languages have evolved to offer varying degrees of abstraction, simplifying the implementation of complex systems without exposing the intricacies of lower-level operations.
- π ADTs are user-defined constructs that encapsulate data and operations within a single syntactic unit, ensuring data integrity and preventing external code from directly accessing the data.
- π Two key conditions for ADTs are: 1) the representation and operations must be defined within a single unit, and 2) the representation must be hidden from the program units that use these objects.
- π οΈ The advantages of grouping related ADT elements within a single syntactic unit include better organization, increased modifiability, and the ability to compile each ADT separately, improving efficiency.
- π Hiding the ADT representation from user code enhances reliability, as changes to the internal workings do not affect the code that uses the ADT, and reduces the likelihood of naming conflicts.
- π A programming language must provide a syntactic unit for encapsulating ADTs, a method for making essential parts of an ADT visible to clients while hiding its representation, and support for access controls to regulate visibility.
- π Design issues for ADTs in programming languages include the form of the interface container, the parameterization of ADTs, access controls, and whether the ADT specification is separate from its implementation.
- π» The lecture provides examples of how C++ supports ADTs through classes, access control specifiers, constructors, destructors, and friend functions, demonstrating how these features address the design issues discussed.
- π The implementation of ADTs in C++ can be separated into a header file for declarations and an implementation file for definitions, allowing for the hiding of object representation from the client and better organization of code.
Q & A
What are the main topics discussed in the lectures on Chapter 11?
-The main topics discussed are abstract data types (ADTs), encapsulation, and the implementation of ADTs in programming languages, along with design issues associated with ADTs.
What is the general concept of abstraction in programming languages?
-Abstraction in programming is a view or representation of an entity that includes only the most significant attributes, essentially providing a summarized view that contains only the most important details.
How do high-level programming languages provide abstraction?
-High-level programming languages provide abstraction by hiding details of lower-level implementations, allowing programmers to implement complex systems without needing to understand the underlying operations.
What are the two conditions that an abstract data type (ADT) must satisfy?
-An ADT must have its representation and operations defined in a single syntactic unit, and the representation of the objects must be hidden from the program units that use these objects.
Why is it advantageous to group everything related to an ADT within the same syntactic unit?
-Grouping everything related to an ADT within the same syntactic unit allows for better organization, increased modifiability, and the ability to compile each ADT separately, which can be more efficient.
What is the purpose of hiding the representation of an ADT from the user code?
-Hiding the representation of an ADT from the user code increases reliability, as it prevents the user code from depending on the specific representation and allows the internal workings of the ADT to be changed without affecting the user code.
What are the advantages of hiding the representation of an ADT in terms of programming?
-The advantages include increased reliability, as the user code cannot directly access the objects of the ADT; increased simplicity for the programmer, as they only need to know about the interface; and reduced likelihood of name conflicts.
What features must a programming language provide to support ADTs?
-A programming language must provide a syntactic unit to contain the ADT's representation and operations, and a method to make the essential parts of an ADT visible to clients while hiding the actual data representation.
What are some design issues to consider when a high-level programming language provides support for ADTs?
-Design issues include the form of the container for the interface, whether ADTs can be parameterized, the access controls provided, and whether the specification of the ADT is physically separate from its implementation.
How does C++ support ADTs?
-C++ supports ADTs through the use of classes, which encapsulate the data and operations within a single syntactic unit, and provides features like constructors, destructors, access control specifiers, and friend functions to support various aspects of ADT implementation.
What is the significance of constructors and destructors in C++ for ADTs?
-Constructors in C++ are special functions used to initialize the data members of an instance, while destructors are used to clean up after an instance is destroyed, typically by deallocating resources and performing other cleanup operations.
Outlines
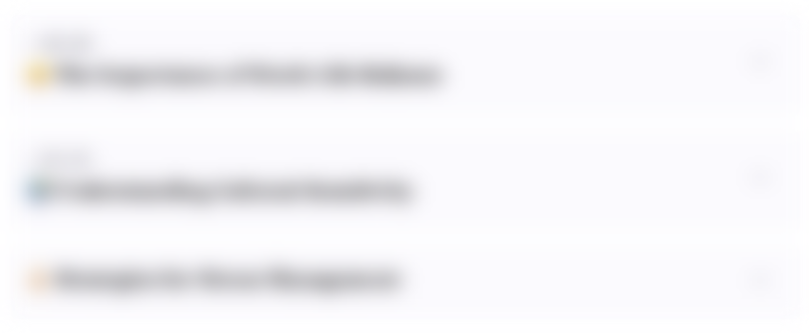
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
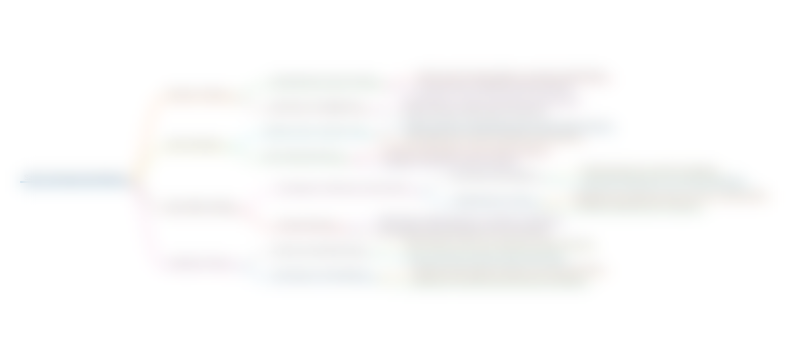
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
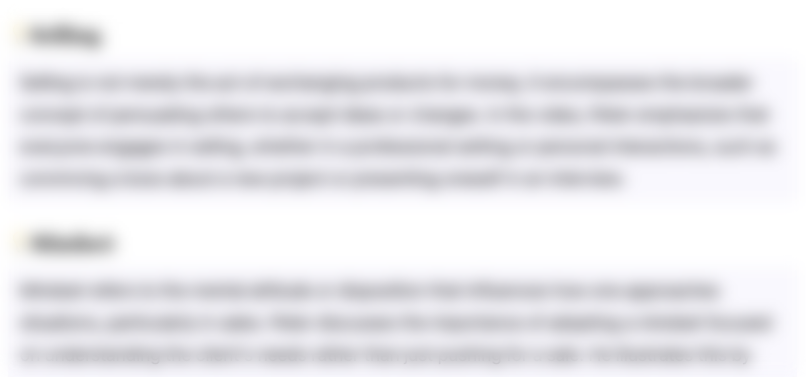
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
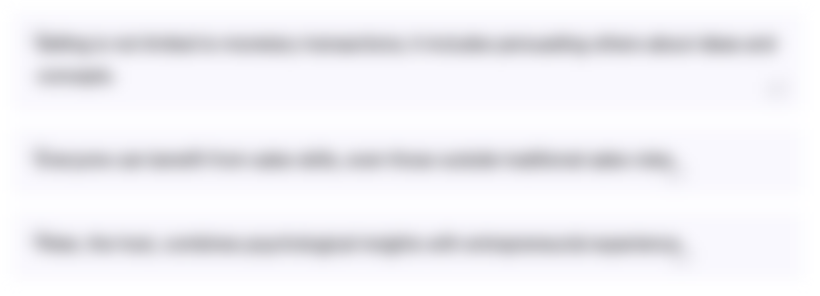
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
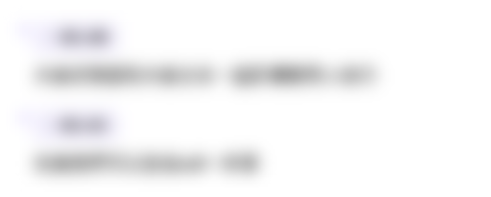
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)