COS 333: Chapter 6, Part 1
Summary
TLDRThis lecture delves into data types, focusing on their importance in programming. It begins with an overview of data types, moving on to discuss primitive data types like integers, floating points, and characters. The lecture then covers user-defined types, including ordinal types with enumerations, and concludes with an exploration of array data types. It touches on the design issues related to data types, such as operations defined for a type and syntactic mechanisms for specification, providing a comprehensive foundation for understanding data structures in programming.
Takeaways
- 📚 The lecture delves into various data types, starting with an introduction and covering primitive data types, character strings, user-defined ordinal data types, and array data types.
- 🔢 Data types define a range of values, memory storage methods, and a set of predefined operations for data objects, emphasizing the importance of type in programming languages.
- 💾 A data type's memory representation can vary, such as using two's complement for integers or IEEE standards for floating points, highlighting the binary encoding of data objects.
- 🔡 Character strings are sequences of characters and can be implemented as primitive types or as arrays of characters, with operations like concatenation and substring referencing.
- 🔑 User-defined ordinal types, such as enumerations, map a set of named constants to integer values, providing readability and type safety in programming.
- 📊 Arrays are aggregate data types that can be static, fixed stack dynamic, stack dynamic, fixed heap dynamic, or heap dynamic, each with different allocation and binding properties.
- 🔄 The dynamic nature of data types, especially arrays, allows for flexibility in programming, but also introduces complexity in memory management and type safety.
- 👍 The advantages of certain data types, like boolean for readability and integer for direct hardware mapping, are discussed, along with their impact on programming language design.
- 👎 The disadvantages, such as memory inefficiency in decimal types or limited range in fixed-length arrays, are also considered in the context of programming language evaluation.
- 🔍 The lecture examines different programming languages' support for data types, such as Java's support for primitive data types and Python's support for complex numbers.
- 🔗 The importance of design issues in data types, like operation definitions and syntactic mechanisms for specifying data types, is emphasized for programming language development.
Q & A
What are the three important aspects defined by a data type in relation to data objects?
-A data type defines a collection of data objects with a range of values, how the data objects are stored in memory, and a set of predefined operations on these data objects.
How does a data type's range of values differ between integers and floating-point values?
-For integers, the range of values is defined by a minimum and maximum whole number that can be represented without a fractional portion. For floating-point values, the range is defined by the minimum and maximum values allowable for the type, along with the precision associated with the type.
What is the significance of the IEEE standard in floating-point representation?
-The IEEE standard floating-point representation is significant as it provides a consistent and widely used method for encoding floating-point values on a binary level, ensuring compatibility and accuracy across different systems and platforms.
Why might a programming language provide support for unsigned integer types?
-Unsigned integer types are provided to allow for a greater range of positive integer values, effectively doubling the range of numbers that can be represented with the same amount of memory, compared to signed integers.
What is the primary advantage of using a decimal type over a floating-point type for representing monetary values?
-The primary advantage of using a decimal type is accuracy, as it stores data using a fixed number of decimal digits, providing an exact representation without the approximations inherent in floating-point types.
What is the main drawback of using a character encoding scheme like ASCII?
-The main drawback of using ASCII is that it only represents a limited set of characters, primarily English letters and some symbols, and does not support the wide range of characters needed for other languages.
Why is the support for character strings considered important in programming languages?
-Support for character strings is important because it enhances readability and writability, allowing programmers to work with sequences of characters in a more intuitive and efficient manner, which is especially useful in applications that involve text manipulation.
What are the two main design issues to consider when implementing support for character strings in a programming language?
-The two main design issues are whether character strings should be a primitive type or a special kind of array, and whether the length of the string should be static (fixed) or dynamic (able to grow and shrink during program execution).
What is an enumeration type and how does it differ from a primitive ordinal type?
-An enumeration type is a user-defined data type with a set of named constants as its possible values. It differs from a primitive ordinal type, which is built into the programming language and has values that can be mapped to a set of positive integers.
Why are heap dynamic arrays considered more flexible than other types of arrays?
-Heap dynamic arrays are considered more flexible because they allow the array to grow and shrink during program execution, with the memory allocated from the heap rather than the stack, which means the array's size can change as needed without being constrained by the scope of the variable.
Outlines
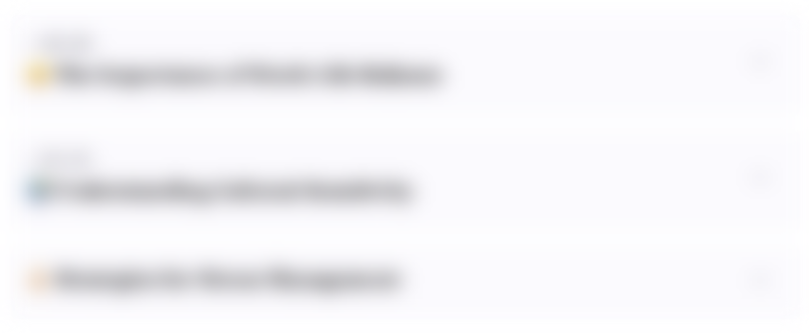
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
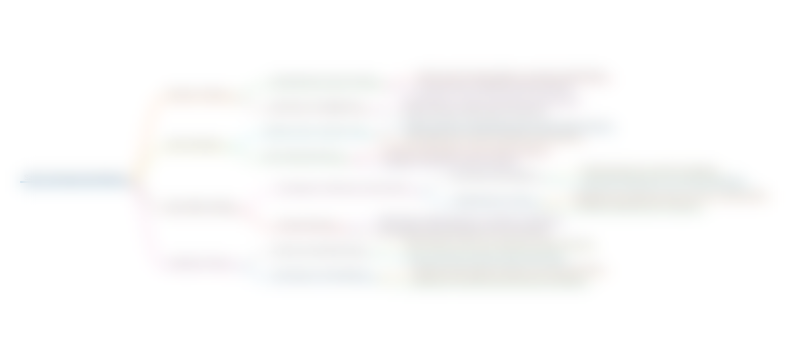
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
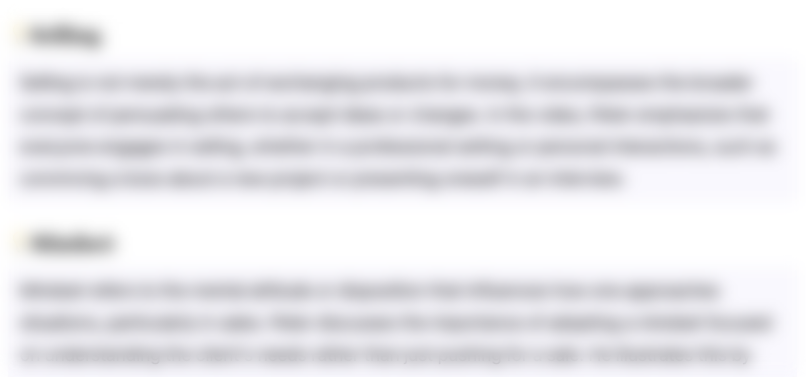
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
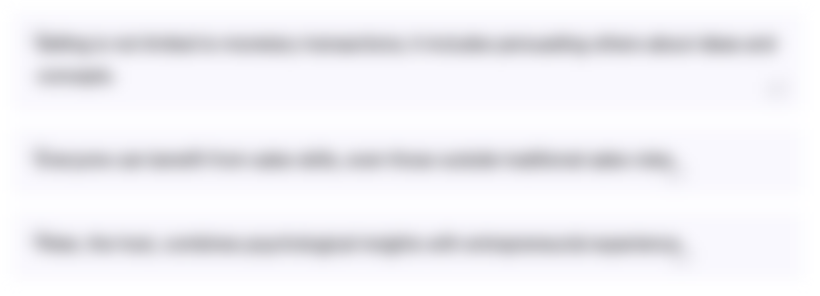
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
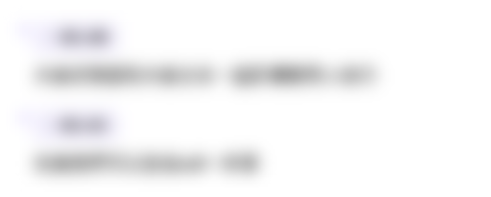
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)