COS 333: Chapter 12, Part 1
Summary
TLDRThis lecture delves into object-oriented programming (OOP), exploring its support in high-level languages and its foundational concepts. It discusses the necessity of abstract data types, inheritance, and polymorphism in OOP languages. The talk highlights design issues like object exclusivity, subtype considerations, and inheritance models, contrasting single and multiple inheritance. It also examines dynamic binding, type checking, and the initialization of objects. The lecture provides insights into programming languages like Smalltalk, which pioneered OOP, and languages like Java and C++ that primarily support OOP but retain imperative structures.
Takeaways
- ๐ The lecture introduces Chapter 12, focusing on support for Object-Oriented Programming (OOP) in high-level languages, with a second part to follow in the last lecture of the course.
- ๐ OOP is supported in various languages to different extents, with some being purely OOP like Smalltalk, while others like Ada and C++ offer OOP as an additional feature on top of procedural programming.
- ๐ก The core concepts of OOP include abstract data types, inheritance, and polymorphism, which are essential for any language claiming to support OOP.
- ๐ถ Inheritance allows for the creation of new classes based on existing ones, promoting code reuse and establishing a hierarchy among classes.
- ๐ The advantages of inheritance include increased productivity through code reuse and the ability to logically structure classes in a hierarchical order.
- ๐ Design issues in OOP languages involve considerations of object exclusivity, subtypes, type checking, single vs. multiple inheritance, object allocation, binding types, nested classes, and object initialization.
- ๐ Dynamic binding in OOP is facilitated by polymorphic references and occurs at runtime, allowing for method calls to be bound to the correct method dynamically.
- ๐ซ Abstract methods and classes are part of OOP, with abstract methods defining a protocol without an implementation and abstract classes being unable to be instantiated.
- ๐ The script discusses three approaches to object exclusivity: treating everything as an object (as in Smalltalk), adding objects to a complete typing system (as in C++), and using an imperative typing system for primitives while making everything else an object (as in Java).
- ๐ค The disadvantages of OOP include increased complexity due to interdependencies among classes, which can complicate maintenance and upgrades.
- ๐ Smalltalk is highlighted as the first purely OOP language, with all computation handled through message passing and all objects allocated on the heap, but suffers from performance issues due to its uniform object handling and reliance on a garbage collector.
Q & A
What is the main focus of the lecture?
-The lecture primarily focuses on the support for object-oriented programming in high-level programming languages, covering the foundational concepts, design issues, and examples of programming languages that support these principles.
What are the key concepts that must be supported in an object-oriented programming language?
-An object-oriented programming language must support abstract data types, inheritance, and polymorphism.
What is the significance of inheritance in object-oriented programming?
-Inheritance allows new classes to be defined in terms of existing classes, promoting code reuse and enabling a hierarchical organization of classes.
Can you explain the difference between a class and an object in object-oriented programming?
-A class is a blueprint for creating objects, defining the properties and methods that objects of that class will have. An object is an instance of a class, representing a specific entity with its own state and behavior.
What is the meaning of polymorphism in the context of object-oriented programming?
-Polymorphism in object-oriented programming refers to the ability of different objects to respond to the same message (method call) in different ways, typically achieved through method overriding.
How does the Smalltalk programming language exemplify object-oriented programming?
-Smalltalk is considered a pure object-oriented programming language because it treats everything as an object, including primitive types like integers and floats, and it was the first language to be developed with these principles.
What are the different extents to which programming languages can support object-oriented programming?
-Programming languages can range from having very basic support for object-oriented programming as an optional feature, to being primarily object-oriented with some imperative structures, to being pure object-oriented languages that rely entirely on an object model for functionality.
What are some of the design issues that need to be considered when supporting object-oriented programming in a high-level programming language?
-Design issues include the exclusivity of objects, the consideration of subclasses as subtypes, type checking with reference to polymorphism, support for single or multiple inheritance, object allocation and de-allocation, dynamic and static binding, nested classes, and object initialization.
Why might a programming language choose to implement single inheritance over multiple inheritance?
-Single inheritance can be chosen to simplify the language and avoid complexities such as naming collisions and additional runtime overhead associated with multiple inheritance.
What is the role of abstract methods and abstract classes in object-oriented programming languages?
-Abstract methods define a protocol for a method without an implementation, ensuring that derived classes must provide their own implementation. Abstract classes cannot be instantiated and include at least one abstract method, enforcing that certain functionality is implemented by derived classes.
How does Smalltalk handle object allocation and memory management?
-In Smalltalk, all objects are allocated on the heap, and memory management is handled implicitly by a garbage collector, which frees up memory occupied by objects that are no longer in use.
What is the impact of dynamic binding on the performance of an object-oriented program?
-Dynamic binding can impact performance because the determination of which method to call is made at runtime, consuming runtime resources. However, it allows for greater flexibility and extensibility in software development.
Outlines
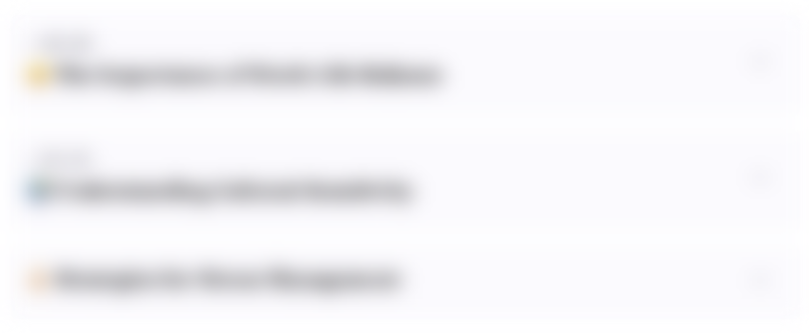
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
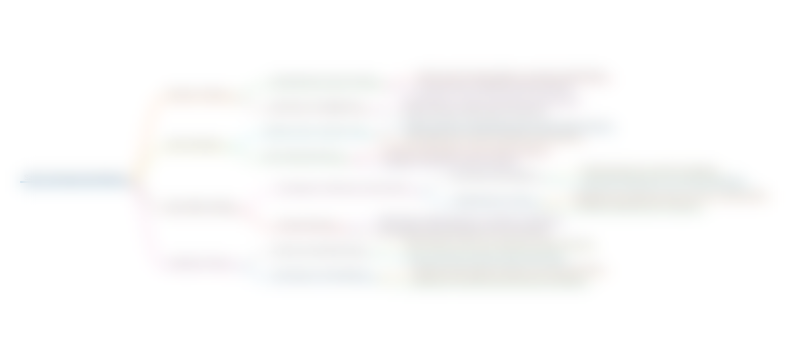
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
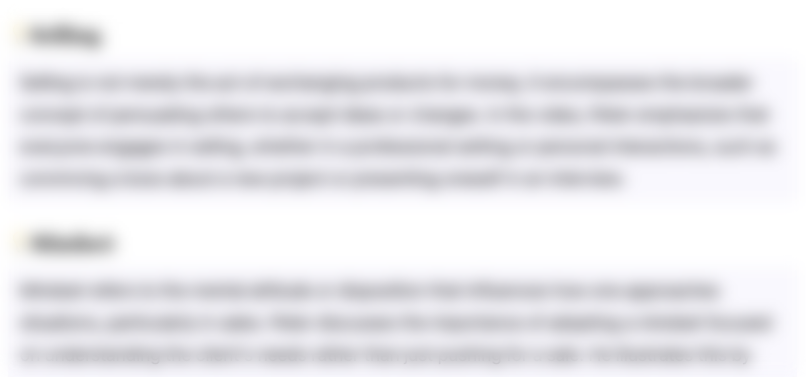
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
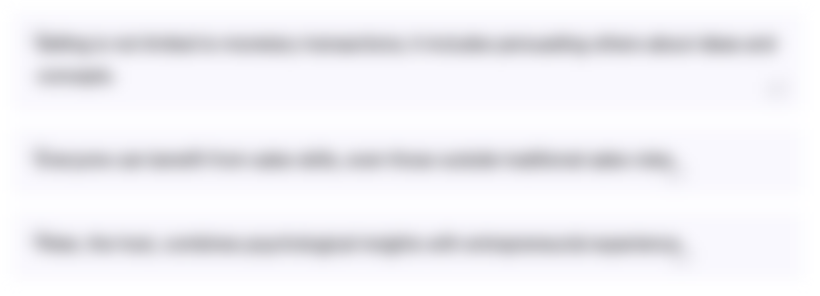
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
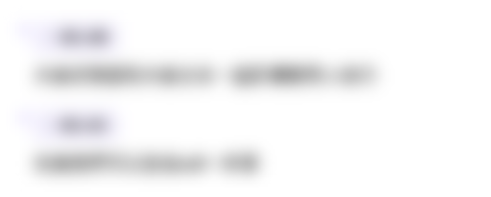
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)