State Update Batching | Lecture 132 | React.JS 🔥
Summary
TLDRThis video script delves into the intricacies of React's state update batching process. It explains how React optimizes performance by batching multiple setState calls into a single state update, triggering only one re-render instead of multiple. The speaker illustrates this concept through code examples, highlighting the asynchronous nature of state updates and the potential for stale state before the re-render. Additionally, the script covers React 18's improved batching capabilities, extending automatic batching to situations like timeouts and promises, and mentions rare cases where disabling batching might be necessary.
Takeaways
- 🤝 React batches multiple state updates in event handlers into a single re-render for better performance.
- ⌚ Accessing the state variable immediately after calling setState will return the stale (previous) value, as the state update is asynchronous and only reflected after re-render.
- 📤 If you need to update state based on the previous state update in the same event handler, pass a callback function to setState instead of a value.
- 🆕 React 18 introduced automatic batching for state updates triggered outside React event handlers, such as timeouts, promises, and native events.
- ⚠️ In rare cases where automatic batching causes issues, you can wrap the problematic state update in ReactDOM.flushSync() to exclude it from batching.
- 🧩 Batching state updates makes sense conceptually, as it represents a single new view rather than multiple partial updates.
- ⚡ Avoiding unnecessary re-renders by batching state updates is a performance optimization provided by React out-of-the-box.
- 🔄 Before React 18, state updates triggered outside React event handlers were not batched, leading to multiple re-renders.
- 🧐 Understanding batching is important, especially when working with older React versions where it behaved differently.
- 🎥 The video provides a detailed explanation of state update batching in React, a crucial concept for optimizing performance.
Q & A
What is state batching in React?
-State batching in React refers to the mechanism where multiple state updates within the same event handler are combined into a single re-render. Instead of triggering a re-render for each individual state update, React batches these updates together and performs only one re-render.
Why does React batch state updates?
-React batches state updates for performance optimization. If multiple state updates are related and meant to represent a new view, it would be inefficient to trigger multiple re-renders for each update. Batching ensures that only one re-render occurs, which improves the application's performance.
Can you access the updated state immediately after calling the setState function?
-No, you cannot access the updated state immediately after calling the setState function. The state update is asynchronous, and the new state value is only available after the re-render cycle has completed. Attempting to access the state variable right after calling setState will still give you the previous (stale) state value.
How can you update state based on the previous state value in the same event handler?
-To update state based on the previous state value within the same event handler, you can pass a callback function to the setState function instead of a single value. The callback function receives the previous state as an argument and returns the new state based on that value.
Did React batch state updates in all situations before React 18?
-No, before React 18, state updates were only batched in event handlers triggered by browser events (e.g., click, submit). Updates triggered outside of event handlers, such as timeouts, promises, or native event handlers, were not batched automatically.
What is the behavior of state batching in React 18 and later versions?
-In React 18 and later versions, state updates are batched automatically in all situations, including timeouts, promises, and native event handlers. This improvement enhances performance by reducing unnecessary re-renders.
Is there a way to opt-out of automatic batching in React?
-Yes, in rare cases where automatic batching might cause problems, you can opt-out by wrapping the problematic state update within the ReactDOM.flushSync function. This will exclude that specific update from being batched.
Why is it important to understand state batching in React?
-Understanding state batching is crucial because it affects the way you work with state updates and can have surprising results if not accounted for. It also helps in writing more efficient and performant React applications by avoiding unnecessary re-renders.
Can state batching lead to unexpected behavior if not handled properly?
-Yes, state batching can lead to unexpected behavior if not handled properly. For example, if you try to access the state value immediately after calling setState, you might get the previous (stale) state value instead of the updated one, which could cause bugs or unintended behaviors.
How does state batching relate to the overall render lifecycle in React?
-State batching is an important part of the render lifecycle in React. It occurs during the initial triggering phase, where React collects all state updates that need to be applied. The batched updates are then processed during the render phase, and a single re-render is triggered, followed by the commit phase.
Outlines
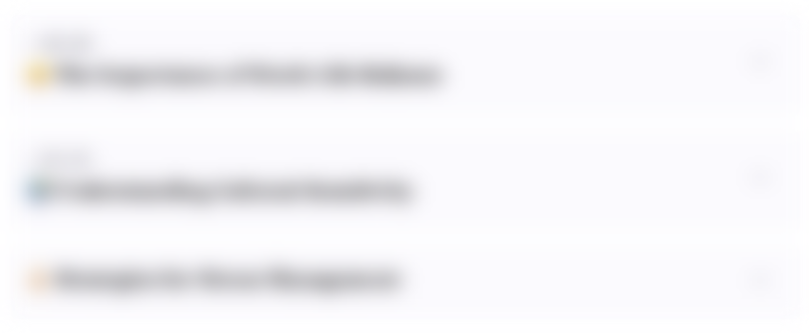
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
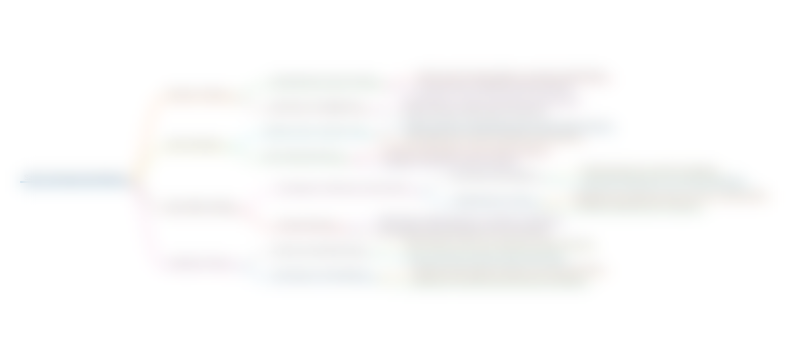
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
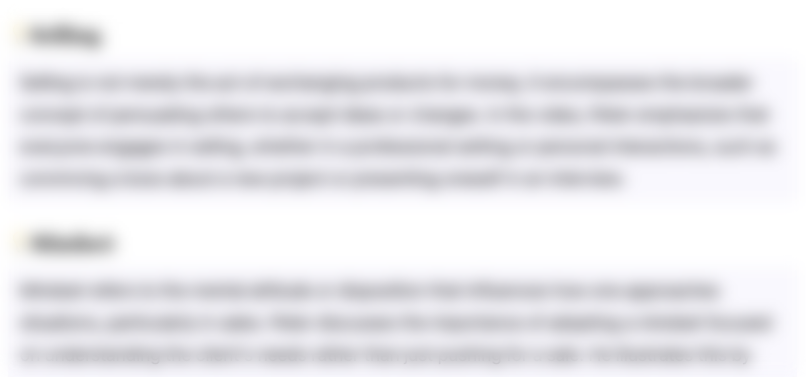
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
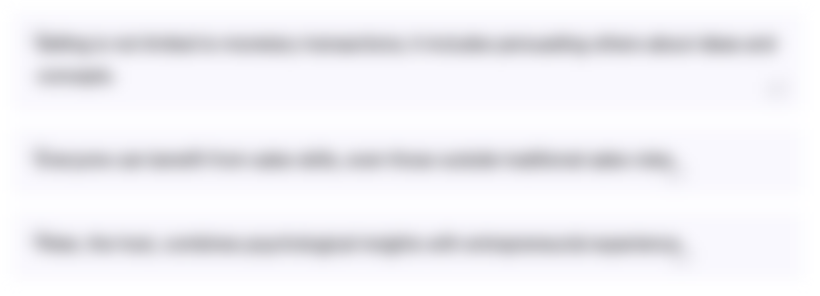
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
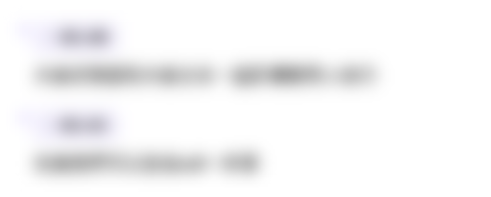
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

State Update Batching in Practice | Lecture 133 | React.JS 🔥

How Rendering Works: The Render Phase | Lecture 124 | React.JS 🔥

How Rendering Works: Overview | Lecture 123 | React.JS 🔥

The useEffect Dependency Array | Lecture 145 | React.JS 🔥

Instances and Elements in Practice | Lecture 122 | React.JS 🔥
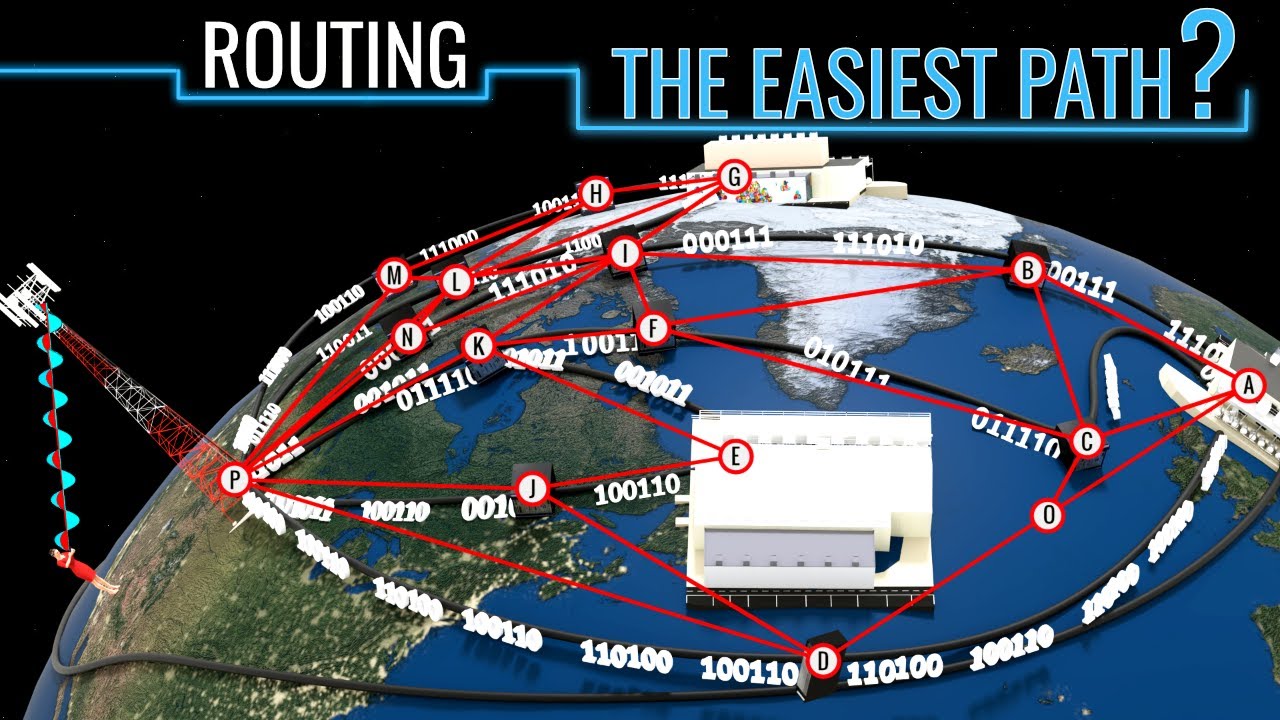
Understanding Routing! | ICT#8
5.0 / 5 (0 votes)