How NOT to Fetch Data in React | Lecture 139 | React.JS π₯
Summary
TLDRThe script discusses the incorrect way of loading data into a React application, which serves as a valuable learning experience. It demonstrates the pitfalls of updating state within the render logic, leading to an infinite loop of state setting and component re-rendering. By breaking this rule intentionally, the instructor aims to reinforce React's best practices and the importance of separating side effects from the render logic. The script walks through fetching movie data from an API and showcases the consequences of setting state directly, resulting in excessive API requests and potential errors. Ultimately, it sets the stage for introducing the useEffect hook as the correct solution in the subsequent lecture.
Takeaways
- π« Never update state in React's render logic (code at the top level of the component function), as it can cause an infinite re-rendering loop.
- π₯ Breaking rules intentionally can be an effective way to learn React and its principles better.
- π The script demonstrates fetching data from an external API (OMDb) and handling the asynchronous response.
- π When using external APIs, it is best practice to store the API key in a separate variable outside the component function.
- β»οΈ Setting state in the render logic causes the component to re-render, which in turn re-executes the render logic, leading to an infinite loop.
- π°οΈ Updating state with an empty array or directly setting watched triggered the 'too many re-renders' error due to the infinite loop.
- π£ The useEffect hook is introduced as the solution to handle side effects like data fetching correctly in React components.
- π For those unfamiliar with asynchronous JavaScript and the fetch API, the script recommends reviewing the relevant sections from earlier in the course.
- π Each time a component re-renders, the entire function (render logic) is executed again, including any variable definitions within it.
- π§ Defining variables that don't depend on component state or props outside the component function is a good practice to avoid unnecessary re-computations.
Q & A
What is the purpose of this lecture?
-The purpose of this lecture is to demonstrate the improper way of fetching data in a React application and then introduce the correct approach using the useEffect hook, which will be covered in the next lecture.
Why is it considered wrong to update state in render logic?
-Updating state in render logic introduces a side effect, which is an interaction with the outside world. React's render logic should be free of side effects to prevent infinite re-rendering loops and maintain the application's stability.
What happens when state is set within the top-level code of the component function?
-Setting state within the top-level code of the component function triggers an infinite loop of state updates and re-renders. This is because updating the state causes the component to re-render, which in turn re-executes the top-level code, leading to an endless cycle.
How is the OMDb API used in this example?
-The OMDb API is used to fetch movie data based on a search query. The instructor demonstrates how to construct the API request URL, obtain an API key, and make the fetch request using the Fetch API in JavaScript.
Why is it recommended to define variables outside the component function?
-Variables that do not depend on anything inside the component should be defined outside the component function. This is because the entire function is re-executed during each render, and defining variables inside would lead to unnecessary re-creation of those variables.
What is the purpose of the `.then()` method used with the `fetch` function?
-The `.then()` method is used to handle the promise returned by the `fetch` function. It allows you to access the response data and perform additional operations, such as converting the response to JSON format.
Why does the instructor suggest breaking rules in this lecture?
-The instructor suggests breaking the rule of not updating state in render logic as a learning experience. By intentionally violating this rule, students can better understand why the rule exists and the consequences of breaking it.
What is the potential issue with making multiple fetch requests in quick succession?
-Making multiple fetch requests in quick succession can lead to excessive network traffic, performance degradation, and potential API rate limiting issues. It's generally advisable to implement appropriate throttling or caching mechanisms to avoid overloading the API with requests.
What is the significance of the 'too many re-renders' error mentioned in the lecture?
-The 'too many re-renders' error is a warning from React indicating that the component is entering an infinite loop of re-rendering, which can lead to performance issues and potential crashes. This error is triggered when state updates are occurring in an uncontrolled manner within the render logic.
What is the solution proposed to address the issue of updating state in render logic?
-The solution proposed is to use the useEffect hook, which will be covered in the next lecture. The useEffect hook allows developers to perform side effects, such as data fetching, in a controlled and predictable manner, preventing the infinite re-rendering loop.
Outlines
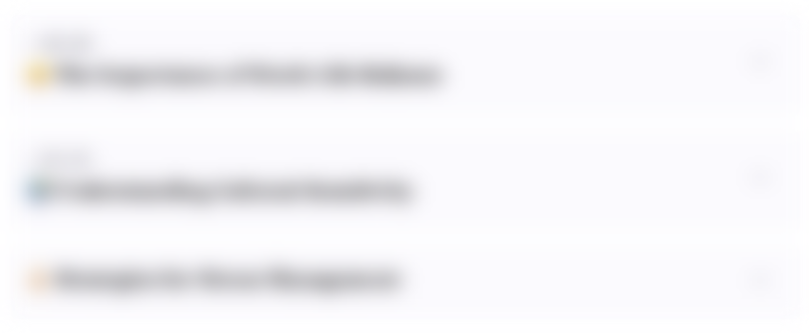
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
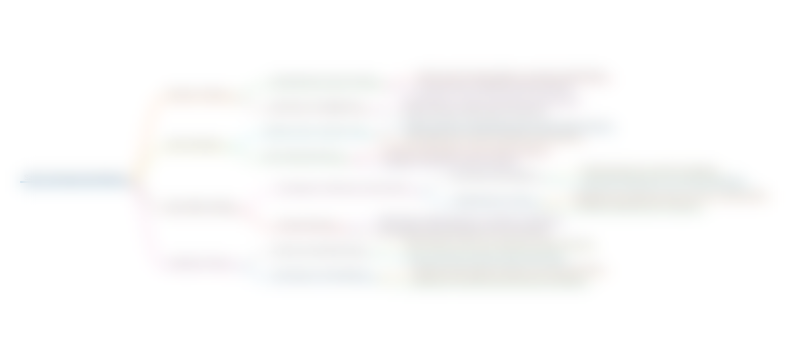
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
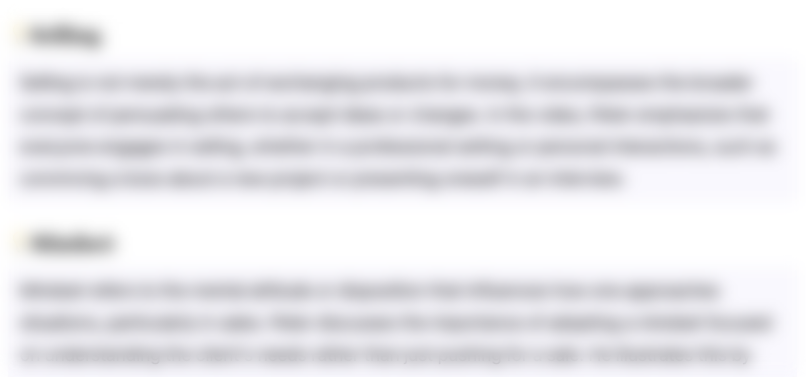
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
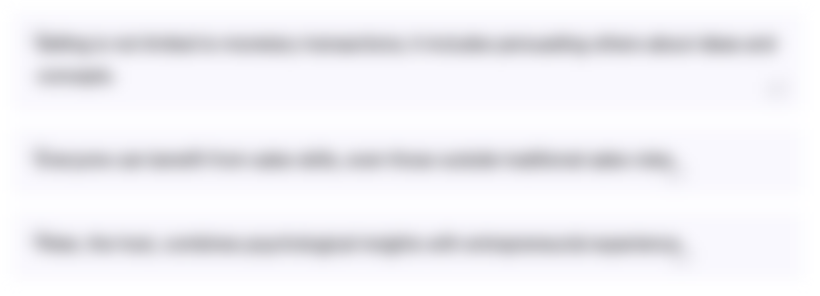
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
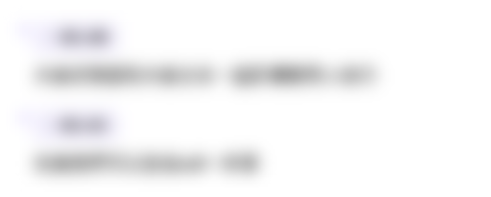
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

useEffect to the Rescue | Lecture 140 | React.JS π₯

Rules for Render Logic: Pure Components | Lecture 131 | React.JS π₯

A First Look at Effects | Lecture 141 | React.JS π₯

The useEffect Cleanup Function | Lecture 151 | React.JS π₯
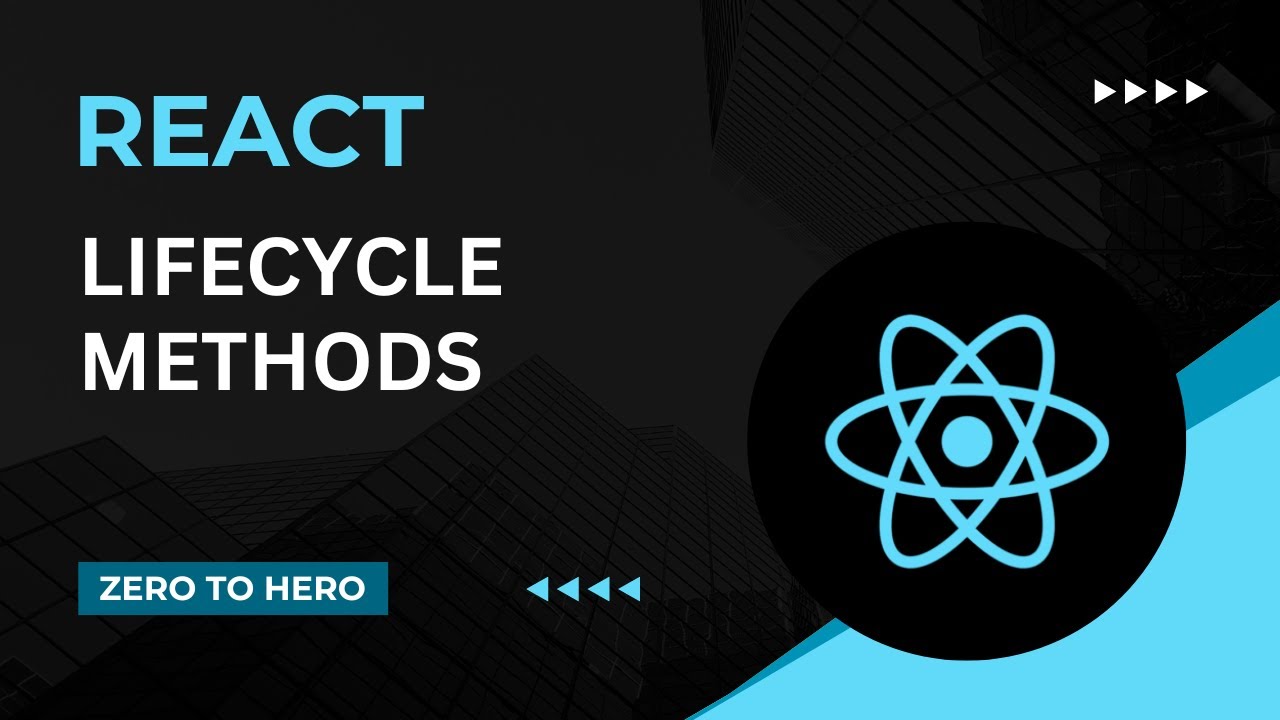
Life Cycle Methods | Mastering React: An In-Depth Zero to Hero Video Series

Introduction to Redux | Lecture 257 | React.JS π₯
5.0 / 5 (0 votes)