Using an async Function | Lecture 142 | React.JS 🔥
Summary
TLDRIn this video, the presenter demonstrates how to convert a synchronous promise handling to an asynchronous function within a React effect. They explain the importance of avoiding race conditions and the limitations of effect callbacks. The tutorial shows how to create a new async function, 'fetchMovies', to handle API calls and update state, while also addressing the issue of stale state by using the latest data. The video also touches on React's strict mode and its impact on effect calls during development, ensuring that potential issues are caught before production.
Takeaways
- 🔄 Converting a function to async allows for cleaner handling of Promises using async/await syntax.
- 🚨 ESLint warns against using async functions directly in effect callbacks due to React's requirement for them to be synchronous.
- 🎯 To work with async functions in React effects, encapsulate the async logic in a separate function that is called within the effect.
- ✅ The await keyword is used to wait for the Promise to resolve within the async function.
- 📦 Data fetched from an API is typically converted to JSON, which is an asynchronous operation.
- 🔧 State updates in React are asynchronous, meaning the state may not be immediately updated after a setState call.
- 🔍 Logging state to the console right after a state update might show stale data due to the asynchronous nature of state changes.
- 🔁 React's strict mode in development doubles the number of effect calls to help identify potential issues with effects.
- 🛠 Removing strict mode can demonstrate the actual number of HTTP requests made, but it's recommended to keep it for safety in development.
- 📈 Adding a loading state can improve the user experience by indicating when data fetching is in progress.
Q & A
What is the purpose of converting a promise handling to an async function?
-Converting promise handling to an async function makes the code easier and nicer to manage, especially when dealing with complex asynchronous operations.
Why can't we directly use an async function in the effect callback of `useEffect`?
-Effect callbacks in `useEffect` must be synchronous to prevent race conditions. Async functions return promises, which are not allowed in this context.
How does the speaker suggest handling the limitation of not using async functions directly in `useEffect`?
-The speaker suggests creating a new function outside of the `useEffect` and then placing the async function within that new function to avoid the ESLint warning.
What is the role of the `fetchMovies` function in the script?
-The `fetchMovies` function is an async function that is used to fetch data from an API and is called within the effect to handle the data fetching logic.
Why does the speaker mention that setting state is asynchronous?
-Setting state in React is asynchronous to ensure that the state updates do not cause race conditions. This means that the state is not updated immediately after the instruction to set it.
What is the issue with logging the `movies` state before it is updated?
-Logging the `movies` state before it is updated results in an empty array because the state update is asynchronous and has not yet been applied when the log statement is executed.
How does the speaker address the issue of logging the updated `movies` state?
-The speaker suggests using the variable `data.Search` instead of `movies` in the log statement to correctly display the fetched data.
What is the purpose of React's strict mode in the context of effects?
-React's strict mode, when activated, calls effects twice in development to help identify potential issues with the effects. This does not occur in production to ensure performance.
Why does the speaker recommend keeping strict mode enabled in the code?
-Keeping strict mode enabled is recommended because it provides additional safety checks during development, helping to identify and fix potential problems with the effects before the application goes into production.
What is the next step the speaker plans to cover in the video series?
-The next step the speaker plans to cover is adding a loading state to the data fetching process to improve the user experience and provide feedback while data is being fetched.
Outlines
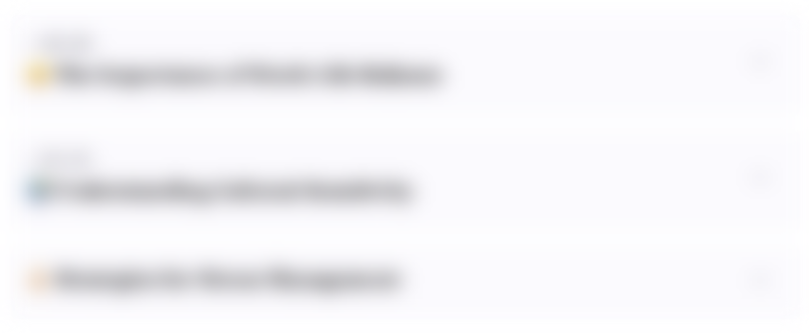
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
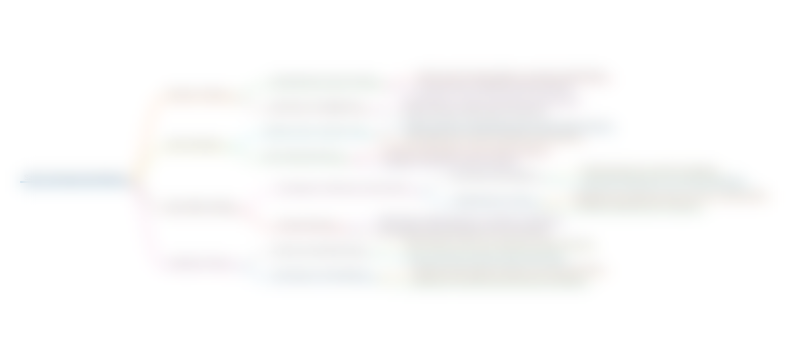
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
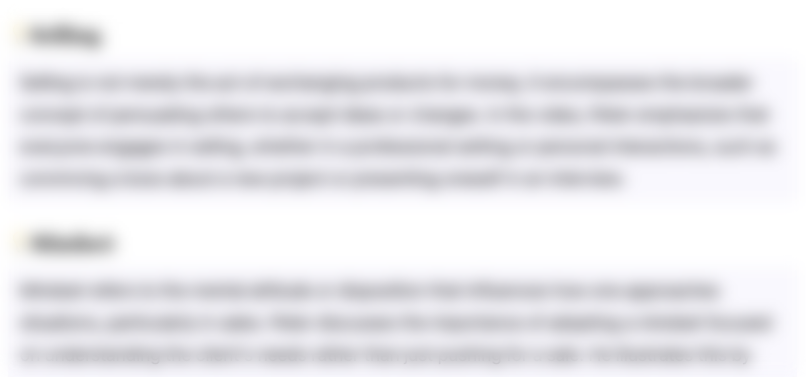
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
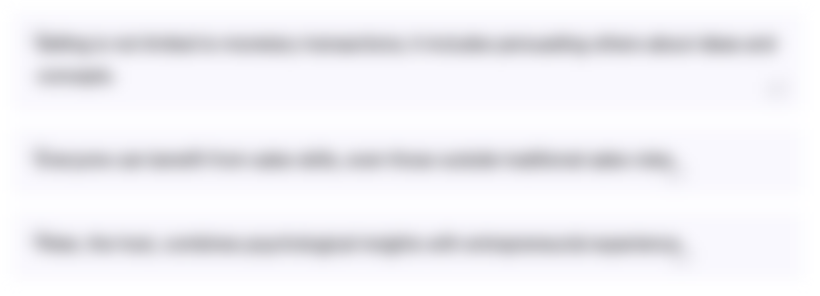
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
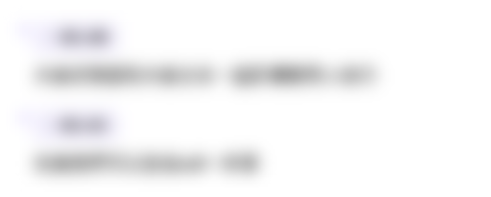
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
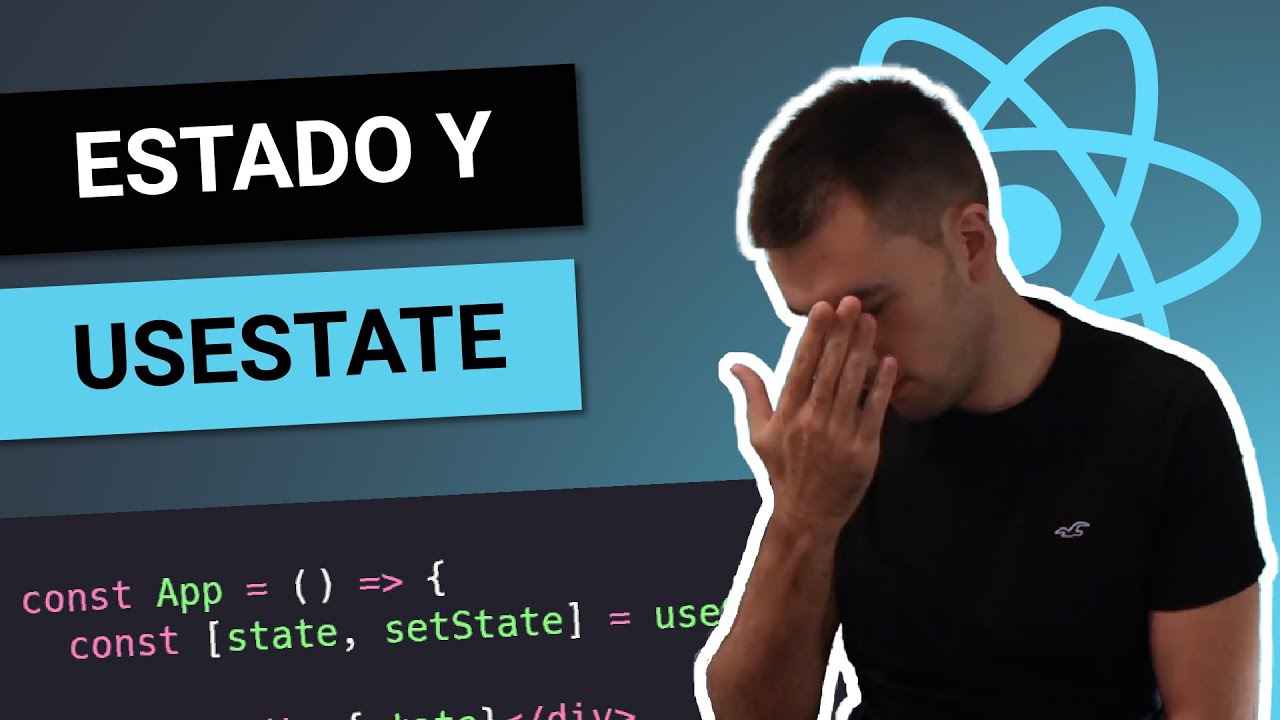
QUÉ es un ESTADO en REACT 🤔 CÓMO usar USESTATE 😎 Curso de React desde cero #9

The useEffect Cleanup Function | Lecture 151 | React.JS 🔥

State Update Batching | Lecture 132 | React.JS 🔥
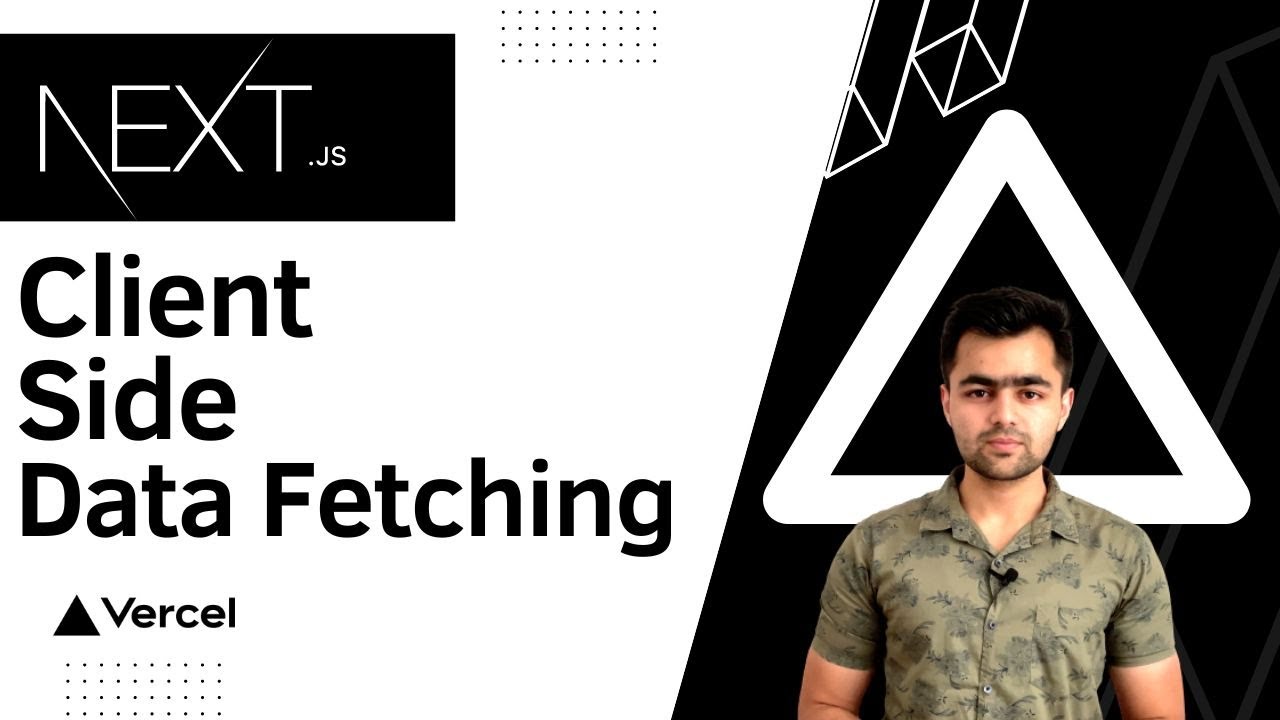
Client Side Data Fetching in NextJS | NextJS in Hindi

Next.js Fetch Data the Right Way (with a Data Access Layer!) (Security, Auth, Cache, DTO)
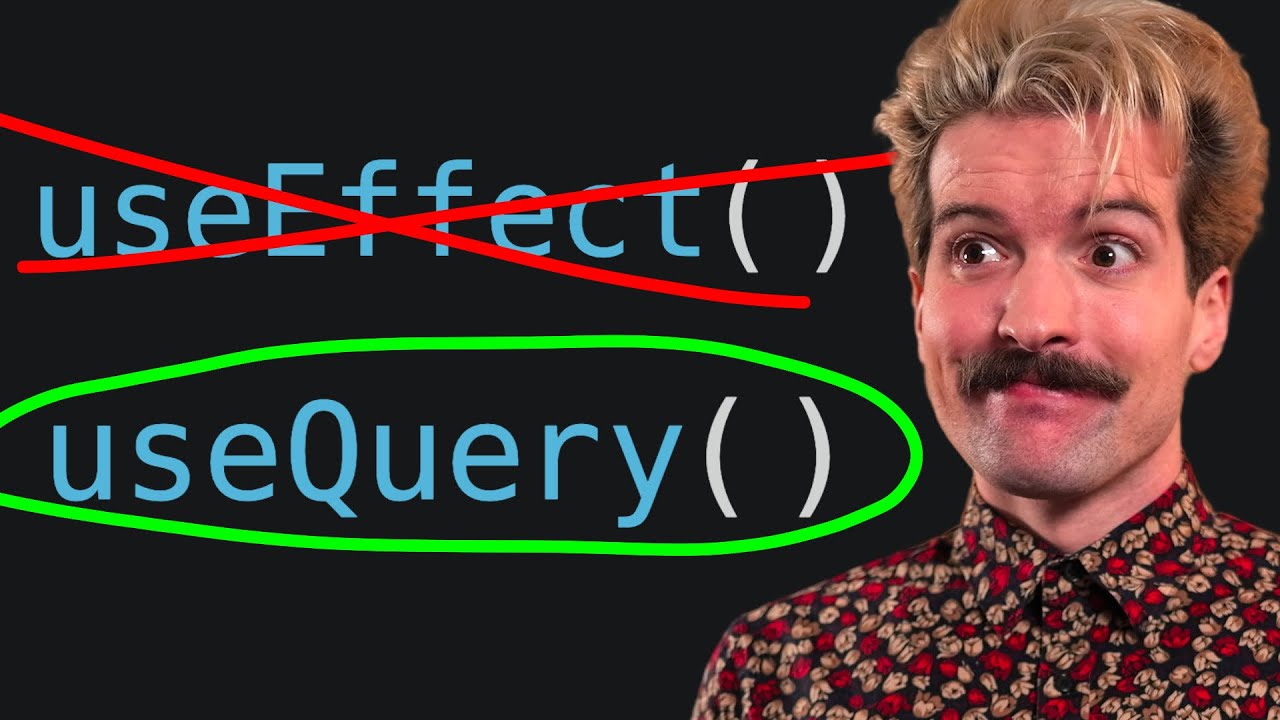
React Query Is (Still) Essential - My Favorite React Library
5.0 / 5 (0 votes)