Remove Elements and Empty an Array in JavaScript - Part - 14
Summary
TLDRIn this video, Lavinia from Automation Labs explains various JavaScript techniques for array manipulation. The video covers how to find specific elements using the `find` method and simplify the code with arrow functions. It also demonstrates how to remove elements from arrays using `pop`, `shift`, and `splice` methods, as well as how to clear an entire array using different approaches. Lavinia provides both practical code examples and insights into when to use each method effectively, helping viewers enhance their understanding of JavaScript array operations.
Takeaways
- 😀 Arrow functions simplify the code for finding elements in arrays by replacing longer traditional function expressions.
- 😀 The `find()` method in JavaScript helps locate a specific element in an array, and using an arrow function makes it more concise.
- 😀 The `pop()` method removes and returns the last element from an array, making it useful for removing elements from the end.
- 😀 The `shift()` method removes and returns the first element of an array, ideal for removing elements from the beginning.
- 😀 To remove elements from the middle of an array, the `splice()` method can be used by specifying the start index and the number of elements to remove.
- 😀 The `splice()` method can also be used without the second parameter to remove all elements from a specific index to the end of the array.
- 😀 To empty an array, you can use multiple methods, such as reassigning it to a new blank array or setting its length property to zero.
- 😀 Setting the `length` property of an array to 0 is the most efficient way to clear an array, as it directly empties it.
- 😀 Using the `splice()` method with the range from 0 to the array's length will also empty the array by removing all its elements.
- 😀 The `pop()` method in a loop can also be used to empty an array, but this method is less efficient for large arrays due to performance concerns.
Q & A
What is the purpose of the 'find' method in JavaScript?
-The 'find' method is used to locate a specific element in an array of objects. It iterates over the array and returns the first element that satisfies the provided condition.
How can you simplify the 'find' method code using an arrow function?
-You can simplify the 'find' method by using an arrow function. Instead of writing a long function expression, you can directly pass a condition to the 'find' method using an arrow function, which makes the code more concise.
What method is used to remove the last element from an array in JavaScript?
-The 'pop' method is used to remove the last element from an array. It also returns the removed element, so it can be stored in a variable if needed.
How do you remove the first element from an array in JavaScript?
-The 'shift' method is used to remove the first element from an array. It also returns the removed element, which can be stored in a variable if desired.
What is the difference between 'pop' and 'shift' methods?
-'Pop' removes the last element from an array, whereas 'shift' removes the first element. Both methods also return the removed element.
How can you remove an element from the middle of an array?
-To remove an element from the middle of an array, you can use the 'splice' method. This method allows you to specify a starting index and the number of elements to remove.
What does the 'splice' method do if no second parameter is provided?
-If no second parameter is provided to the 'splice' method, it will remove all elements from the starting index to the end of the array.
How can you clear all the elements from an array in JavaScript?
-There are multiple ways to clear an array. One way is to set the array's length property to zero (e.g., 'array.length = 0'). Another method is to assign a new empty array to the variable. The 'splice' method and 'pop' in a loop can also be used, but these approaches may not be as efficient.
What is the issue with using 'const' for array assignment when trying to empty an array?
-If an array is declared with 'const', its reference cannot be changed. While the contents of the array can be modified, the array cannot be reassigned to a new array, causing issues when attempting to clear the array by assigning an empty array.
Why is using 'array.length = 0' recommended for clearing an array?
-'array.length = 0' is recommended because it directly modifies the array's length and removes all elements efficiently. It works well even if other references are pointing to the same array.
Outlines
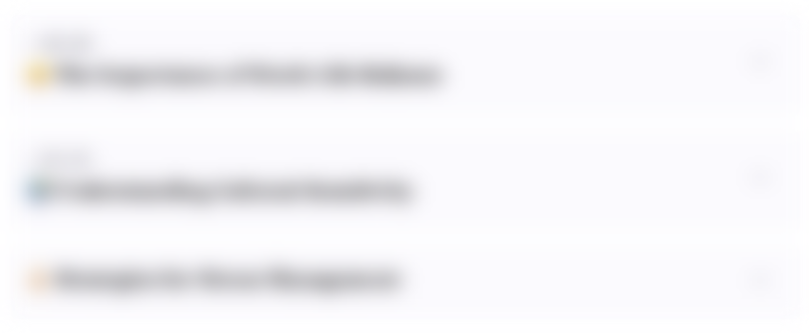
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantMindmap
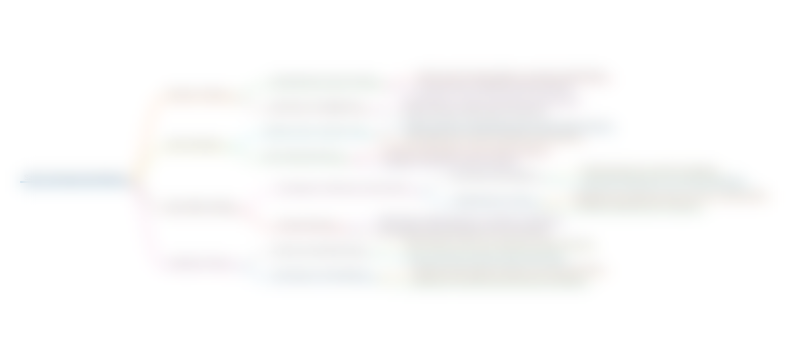
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantKeywords
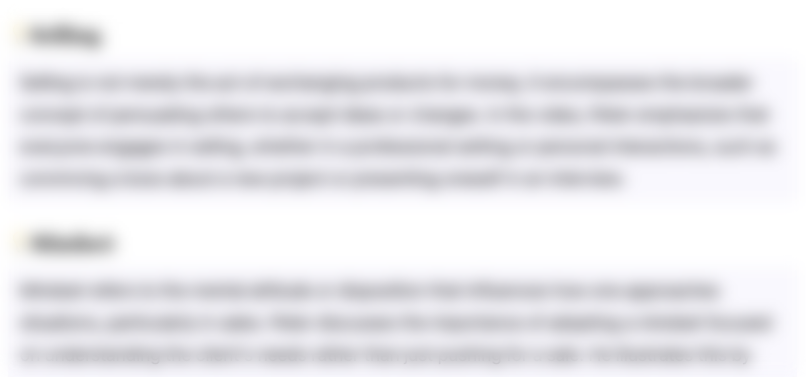
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantHighlights
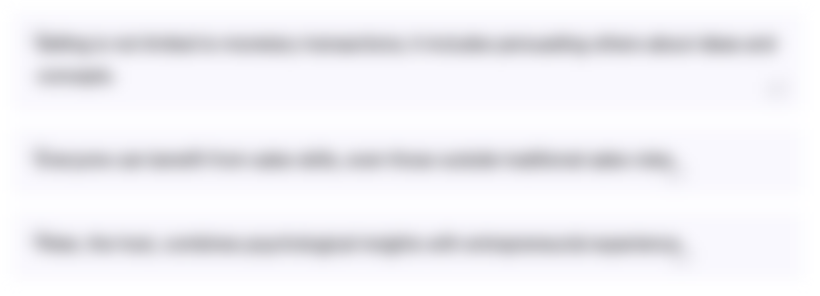
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenantTranscripts
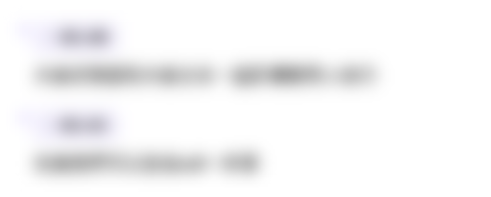
Cette section est réservée aux utilisateurs payants. Améliorez votre compte pour accéder à cette section.
Améliorer maintenant5.0 / 5 (0 votes)