ARRAYLIST VS LINKEDLIST
Summary
TLDRThis video script explores the intricacies of arrays in programming, contrasting their behavior across different languages. It explains that systems programming languages require explicit type and size specification for arrays, whereas scripting languages like JavaScript and Python offer more flexibility, with JavaScript arrays being essentially hashmaps. The script delves into memory allocation, indexing, and the trade-offs between dynamic sizing and cache efficiency, highlighting data structures like linked lists and array lists to overcome array limitations.
Takeaways
- ๐ Arrays are fundamental in programming, but their functionality varies by language, with systems programming languages requiring explicit type and size specification.
- ๐ Scripting languages offer more flexibility with arrays, allowing dynamic resizing and the inclusion of diverse data types.
- ๐ ๏ธ In systems programming, the inability to expand arrays and store different data types is due to the need for contiguous memory allocation and the risk of overwriting other values.
- ๐ Arrays use indexing, starting with zero, to access elements, which is a concept inherited from C and widely used due to its pointer arithmetic simplicity.
- ๐ง The base address of an array is crucial for indexing, as it helps calculate the memory address of any element by adding the product of the element's size and its index to this base address.
- ๐ซ Accessing elements outside the array's bounds can lead to segmentation faults or undefined behavior if the accessed memory is within the process's boundaries.
- ๐ To overcome array limitations, data structures like linked lists and array lists are used, providing dynamic sizing but with trade-offs in caching efficiency and performance.
- ๐ Linked lists allow dynamic sizing but suffer from poor cache performance due to scattered elements in memory.
- ๐ Array lists combine the benefits of arrays with dynamic sizing, using a capacity that can grow as needed, though this can incur performance costs during resizing.
- ๐ JavaScript arrays are implemented as hashmaps rather than traditional arrays, allowing for flexible indexing and efficient memory use.
- ๐ The choice of data structure depends on the specific requirements of the application, including performance, memory usage, and the need for dynamic resizing.
Q & A
What is the primary difference between arrays in systems programming languages and scripting languages?
-In systems programming languages, arrays require the specification of both the type and size of elements they will store, while scripting languages offer more flexibility, allowing for the addition of an unlimited number of elements and the inclusion of diverse data types within the same array.
Why can't arrays in systems programming languages inherently expand to accommodate more elements?
-Arrays in systems programming languages can't inherently expand because adding elements without considering the memory could potentially overwrite other necessary values. This is why such languages often lack methods like append, insert, or push, which are common in scripting languages.
How does the memory address play a role in accessing elements in an array?
-Memory addresses are crucial for accessing array elements because the computer accesses values in terms of memory addresses rather than variables. The base address of the array is used to calculate the memory address of a specific element by adding the product of the element's size and its index to the base address.
What is the main advantage of using linked lists over arrays in terms of size flexibility?
-Linked lists provide a dynamically sized list of elements by storing them wherever there is available memory space. They use nodes that contain an item and a pointer to the next node, which allows for the addition of new elements without the need to know the total number of elements in advance.
Why are arrays preferred over linked lists in terms of cache performance?
-Arrays are preferred for cache performance because they keep elements compacted in memory, which increases the chances of cache hits. Linked lists, with their elements scattered throughout memory, are less likely to be cached efficiently due to their non-contiguous nature.
What is the main idea behind an ArrayList in contrast to a simple array?
-An ArrayList is a data type that includes a reference to an array along with additional information about the array, such as capacity and length. It handles dynamic resizing, allowing the array to grow as elements are added, while also providing constant time access to elements by index.
How does an ArrayList manage to grow its size dynamically while maintaining performance?
-When an ArrayList's array is full, it allocates a new array with a larger capacity, typically twice the size of the full array. It then copies all the values from the old array to the new one, updates the reference and capacity, and finally adds the new element, ensuring dynamic growth without significant performance degradation.
What is the trade-off when considering the use of linked lists for removing elements?
-While removing an element from a linked list is faster because it only requires updating pointers and does not involve shifting subsequent elements, finding the element to remove in the first place can be slow due to the need to traverse the list from the head to the desired position.
How do scripting languages like Python handle arrays differently from systems programming languages?
-Scripting languages like Python use a specialized data structure, such as a PyListObject, which is an array of object pointers. This allows for the addition of elements of different types and simulates the behavior of an array without the strict type and size limitations of systems programming languages.
What is the underlying structure of a JavaScript array, and how does it differ from traditional arrays?
-JavaScript arrays are actually implemented as hashmaps, where keys correspond to indexes. This allows for the addition of elements at any index without the need to allocate memory for all the positions in between, providing a flexible and efficient way to handle arrays.
Outlines
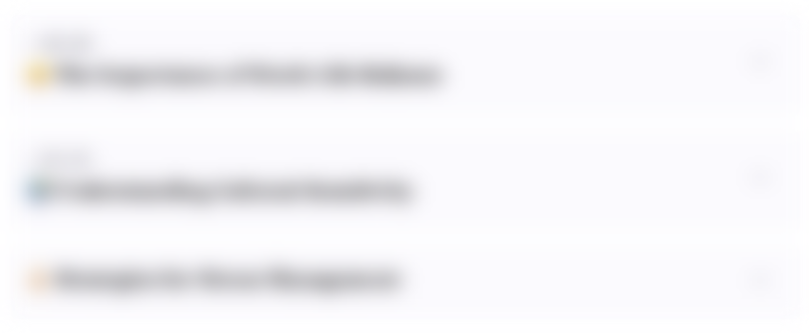
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
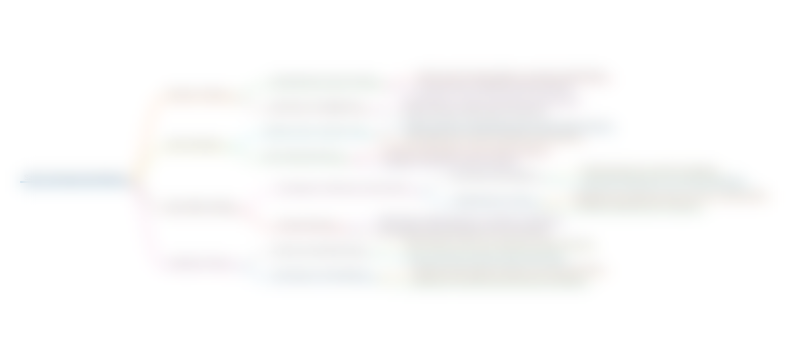
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
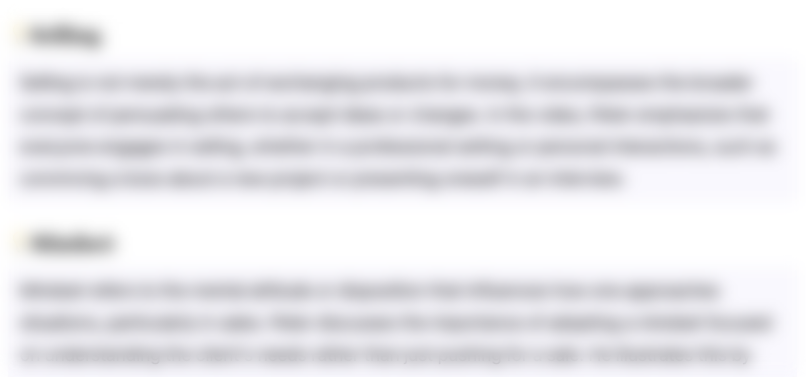
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
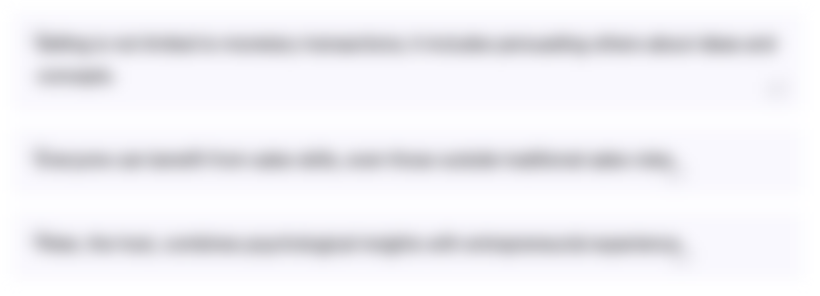
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
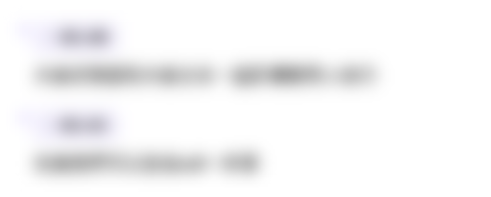
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)