Looping Arrays, Breaking and Continuing | JavaScript 🔥 | Lecture 044
Summary
TLDRThe script provides a step-by-step walkthrough of using for loops in JavaScript to iterate through arrays. It starts with a simple example of logging array elements to the console, explaining the logic and syntax behind constructing a basic for loop. It then covers more advanced topics like dynamically setting loop conditions based on array length, constructing new arrays from existing ones, and using continue and break statements to control loop execution flow. The goal is to provide a comprehensive overview of leveraging for loops to efficiently process array data in JavaScript.
Takeaways
- 😀 For loops are commonly used to iterate over arrays
- 👉 We can log each element of an array to the console using a for loop
- 📝 The condition of the for loop should check that the counter stays below the length of the array
- 🧮 We can perform operations on each element of an array using values from the array in the for loop
- 🔢 We started the counter at 0 because arrays are zero-indexed when accessing elements
- 🆕 We can create a new array and fill it based on values from another array using a for loop
- ➕ We can use the .push() method to add elements to an array within a loop
- ❗️ The continue statement skips the current iteration and moves to the next one
- 🛑 The break statement stops the entire loop execution
- 🎉 Understanding how to loop arrays with for loops is very important
Q & A
What is a common use case for for loops?
-A very common use of for loops is to iterate through the elements in an array.
How do you loop through an array with a for loop?
-You need to start a counter at 0, set the loop condition to be that the counter is less than the array length, and increment the counter each iteration.
Why is using the array length better than hardcoding a number for the loop condition?
-Using the array length automatically adjusts if new items are added, instead of having to manually update a hardcoded length.
How can you fill a new array based on values from another array?
-You can use a for loop on the first array, and in each iteration, calculate values to push into the new array.
What does the continue statement do?
-Continue exits the current iteration of the loop and jumps to the next iteration.
What does the break statement do?
-Break exits the whole loop completely, terminating it early.
How could you use continue or break in a loop?
-You could use continue to skip some iterations based on a condition, or use break to stop the loop when you have reached some target condition.
What are the key components of a for loop?
-The key components are the counter initialization, the loop condition, the code executed in the loop body, and updating the counter.
How do you access the current element when looping an array?
-You use the array name and the current counter variable, like array[i], to access the current element.
Why is looping arrays important in programming?
-It allows you to perform the same operations or calculations on every element without repetitive code.
Outlines
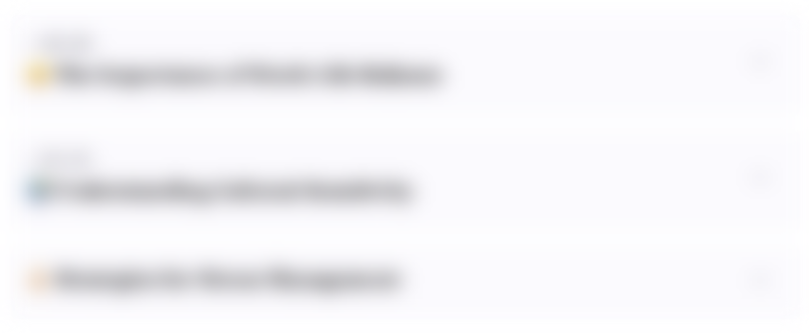
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
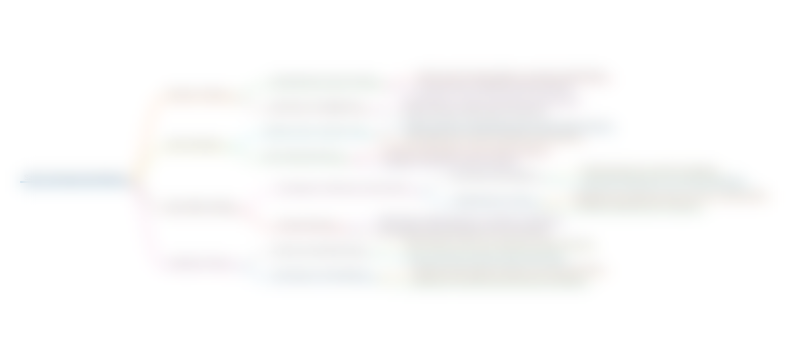
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
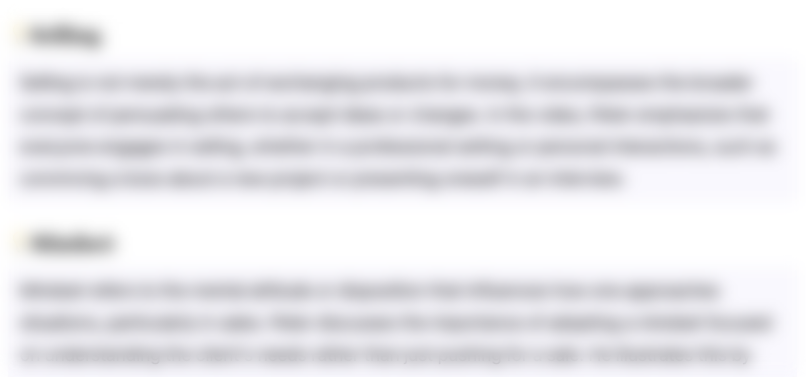
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
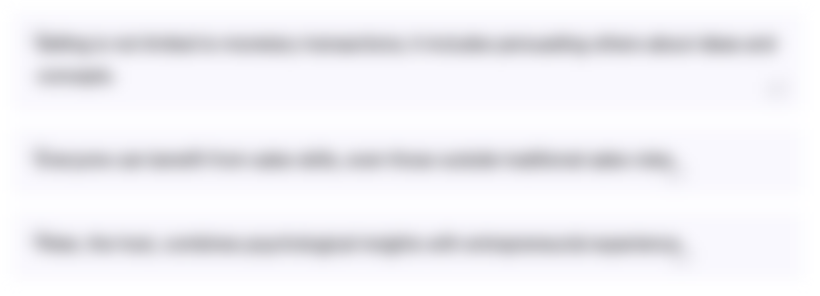
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
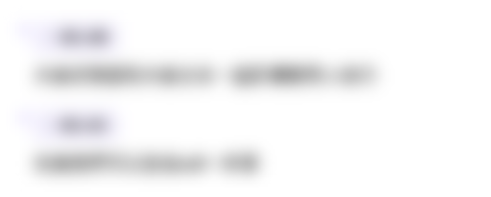
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)