Debugging JavaScript - Are you doing it wrong?
Summary
TLDRThis video script introduces a more efficient debugging method for JavaScript, replacing the traditional scattering of console.log statements. It demonstrates using the 'debugger' keyword in code, which creates breakpoints allowing developers to pause execution, inspect variables, and navigate through the code step-by-step. The tutorial uses a React app as an example, but the technique is applicable to any frontend JavaScript. By utilizing breakpoints, developers can quickly identify and fix issues, leading to a more professional and hassle-free debugging process.
Takeaways
- 🔍 The script discusses a common frustration with debugging JavaScript by using scattered console.log statements.
- 🛠️ The video introduces a better debugging method for frontend JavaScript, applicable regardless of the framework used.
- 💻 The example provided is a broken React app from the 'Learn React and Redux' course, but the debugging method is not framework-specific.
- 🔄 The usual approach of adding console.log to inspect variables is acknowledged but criticized for being cumbersome.
- 🚀 The 'debugger' keyword is introduced as a replacement for console.log, which provides a more interactive debugging experience.
- 🔎 When the 'debugger' statement is hit, the browser's JavaScript execution pauses, allowing for an inspection of the current state.
- 📐 The script explains how to use the browser's developer tools to navigate through the code, inspect variables, and understand the call stack.
- 📌 Breakpoints can be set, viewed, and managed directly within the developer tools for more control over the debugging process.
- 👀 The debugger allows developers to see the exact line of code where an issue occurs, streamlining the process of identifying and fixing bugs.
- 🛑 The use of the debugger statement is temporary and should be removed from the code once the issue is resolved.
- 🌟 The video concludes by emphasizing that using breakpoints is a more professional, faster, and hassle-free way to debug JavaScript code.
Q & A
What is the common issue faced when debugging JavaScript using console.log statements?
-The common issue is that it can be messy and frustrating to constantly add and remove console.log statements throughout the code to check for errors.
What is the main project featured in the video script?
-The main project is a React app from the 'Learn React and Redux from Beginner to Paid Professional' course.
What problem does the app face in the script?
-The app has a problem where the thumbnails do not display correctly when performing a search.
What is the alternative method suggested in the script for debugging JavaScript?
-The alternative method suggested is using the 'debugger' statement instead of console.log to pause the JavaScript execution at a specific point for inspection.
Why is using the 'debugger' statement considered a better way to debug JavaScript?
-Using the 'debugger' statement allows for a more interactive debugging experience, where developers can inspect the current state of the code, see variable values, and navigate through the code without the need for constant logging.
What happens when the JavaScript execution hits the 'debugger' statement?
-When the JavaScript execution hits the 'debugger' statement, the browser stops the execution at that point, creating a breakpoint where developers can inspect the code and its current state.
What can developers see in the browser's developer tools when at a breakpoint?
-Developers can see the current state of the code, variable values, the call stack, variable scopes, and the ability to add new breakpoints or step through the code line by line.
How can developers navigate through the code using breakpoints?
-Developers can navigate through the code by stepping into functions using the down arrow or stepping through the code one line at a time using the right-facing arrow.
What is the process for fixing a problem identified during a debugging session with breakpoints?
-After identifying the problem and the exact line of code causing the issue, developers can go back to their code, make the necessary corrections, and then remove the 'debugger' statement.
Why is removing the 'debugger' statement important after fixing the issue?
-Removing the 'debugger' statement is important to ensure that the code runs smoothly without interruption in a production environment, as it is only meant for debugging purposes.
How does using breakpoints compare to the traditional console.log method in terms of efficiency?
-Using breakpoints is more efficient as it allows for a more structured and less intrusive way to debug code, providing immediate insights into the code's state without the need for multiple console logs.
Outlines
🛠️ Improving JavaScript Debugging with Breakpoints
The paragraph introduces a common frustration developers face when debugging JavaScript by using console.log statements scattered throughout the code. It presents a web app from a React and Redux course as an example of a broken application, where search results are not displaying correctly. The speaker proposes a more efficient debugging method by using the 'debugger' keyword instead of console.log, which allows the browser to pause execution at a specific point, creating a breakpoint. This method enables developers to inspect the current state of the code, examine variable values, and navigate through the call stack and variable scopes, providing a clearer path to identifying and fixing issues.
Mindmap
Keywords
💡JavaScript
💡Console.log
💡Debugging
💡React
💡Breakpoints
💡Developer Tools
💡Call Stack
💡Variable Scope
💡Stepping
💡Object
💡URL Property
Highlights
JavaScript debugging can be frustrating with scattered console.logs.
A better way to debug JavaScript is introduced in the video.
The example is from a React app, but the method works for any frontend JavaScript.
The app has a search issue where thumbnails are broken.
Traditional debugging involves adding console.log to inspect variables.
The debugger keyword is a cleaner alternative to console.log for debugging.
Using debugger pauses JavaScript execution at a breakpoint for inspection.
Developer tools must be open to utilize the debugger statement.
The debugger allows inspection of the current state of the code.
Variables' current values can be seen by hovering over them in the debugger.
The call stack shows all functions called to reach the current point in code.
Variable scopes and their values are visible in the debugger.
New breakpoints can be added and existing ones removed in the debugger interface.
Stepping through code allows for detailed examination of function calls.
The debugger identifies the exact line of code causing an issue.
Fixing the code involves adjusting the property access to resolve the issue.
After fixing, the app functions correctly, demonstrating the effectiveness of breakpoints.
Breakpoints offer a professional, faster, and hassle-free debugging method.
Transcripts
whenever your javascript doesn't work
you probably resort to scattering
console.logs around your code and
checking the console but this can be a
messy and frustrating way to debug your
javascript in this video i'm going to
teach you a better way
so i have this web app here it's
actually the main project from my learn
react and redux from beginner to paid
professional course so it's a react app
but that's completely irrelevant
what i'm going to show you will work for
any frontend javascript whether you're
using a framework or not
anyway this app is broken if we do a
search the thumbnails all come back
broken
so what do we do so most people will do
this
they'll go to the part of the code where
we've got the function that processes
the search results and do a console.log
on the results
then go back to the browser
and reload it
then you want to bring up the console
and we want to check the results that
we've outputted
and yeah they all look fine to us so the
next step is usually to add another
console.log somewhere else
now this way of debugging does work but
it is a bit cumbersome constantly adding
and removing console.logs all over the
place
i'm going to show you a slightly nicer
way
in the code we simply remove the
console.log
and in its place we put the word
debugger
now let's go back to the browser and see
what that does
so we reload in the browser and you're
going to need to make sure the developer
tools are open all this won't work at
all
we perform our search again
now when the code hits the debugger
statement the browser stops the
javascript execution is paused at this
point we call this a breakpoint we can
now do some really cool things
we can inspect the current state of the
code
we can see exactly where we are in the
code we can mouse over variables and it
shows their current values
if you go down here we can see a call
stack showing all of the functions that
were called to get to this point
over here we can see all of the variable
scopes we've got available at the
current point and see all of the
variables in each scope and even their
current values
we can even add new breakpoints by
clicking like this and a blue arrow
comes up then we press the blue arrow
down here and this moves on to the next
break point or just to the end of the
code if there isn't one and you can just
remove the breakpoint by clicking it
again
you can press this down arrow here to
skip into functions or this right facing
arrow here to step through the code one
line at a time
so this goes into another function call
and somewhere down here
we've got the valley we want we thought
it was a string and this is why we've
got a problem but it's actually an
object and we want the url property
which is a string
and the debugger gives us the exact line
of code we need to go and fix it so we
go back to our code we know the exact
line number where we've got the problem
because we saw it in the debugger and
now we just fix it by adding dot url
and of course we go down and we remove
the debugger statement
so in the browser again we reload
perform another search
and now we get all our thumbnails
everything works as expected so there
you have it
breakpoints are a more professional
faster and hassle-free way to debug your
javascript code
Ver Más Videos Relacionados

Debugging Node.js with ndb | Lecture 109 | Node.JS 🔥
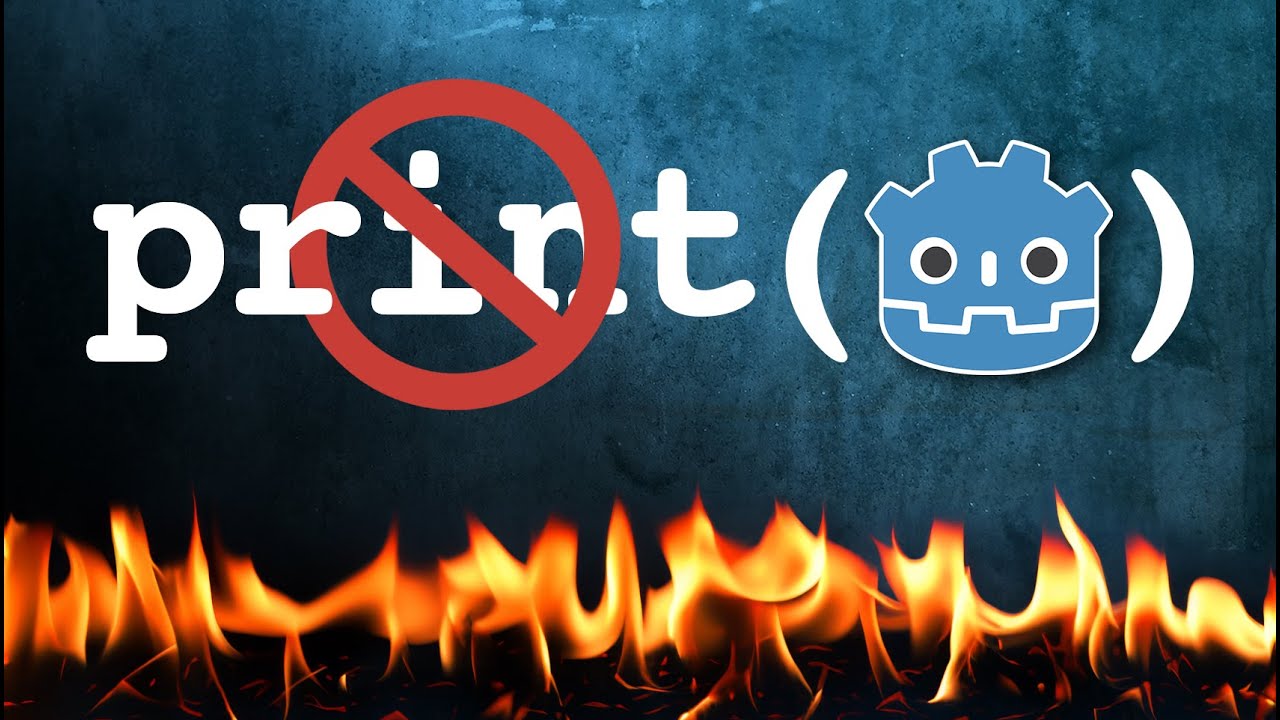
Godot Debugging Techniques EVERY Dev Should Know
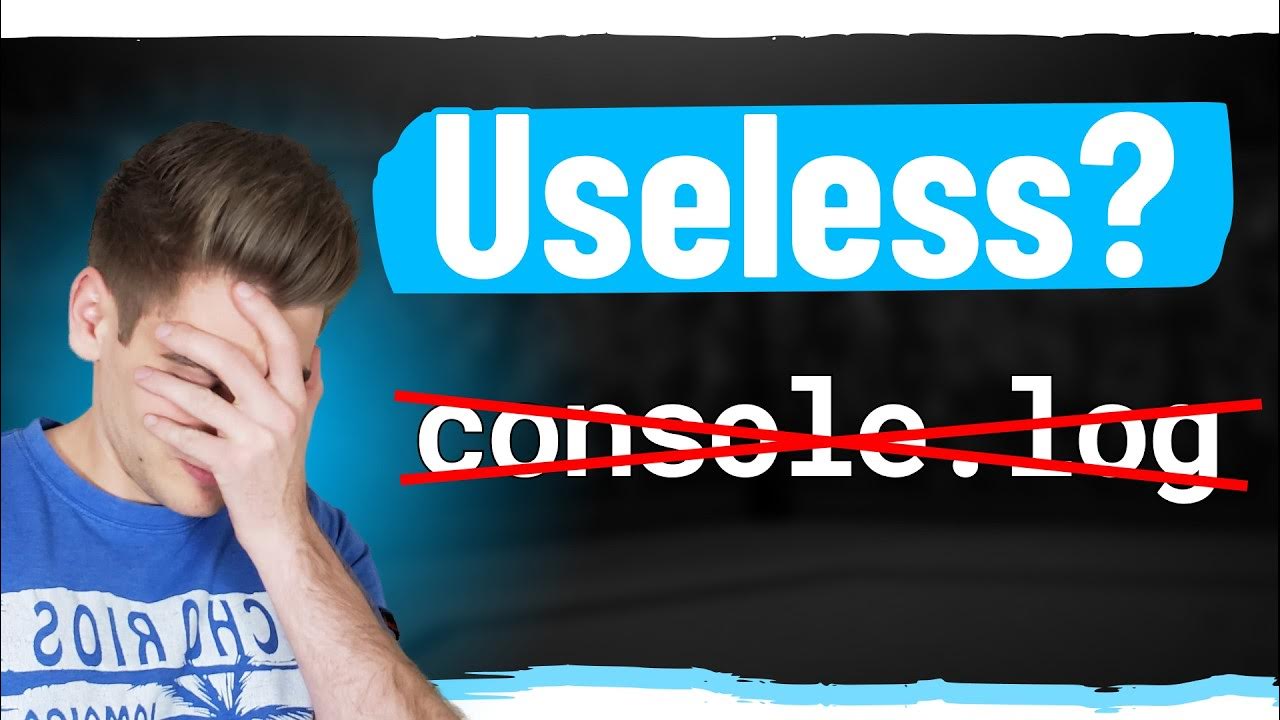
The Most Important Skill You Never Learned
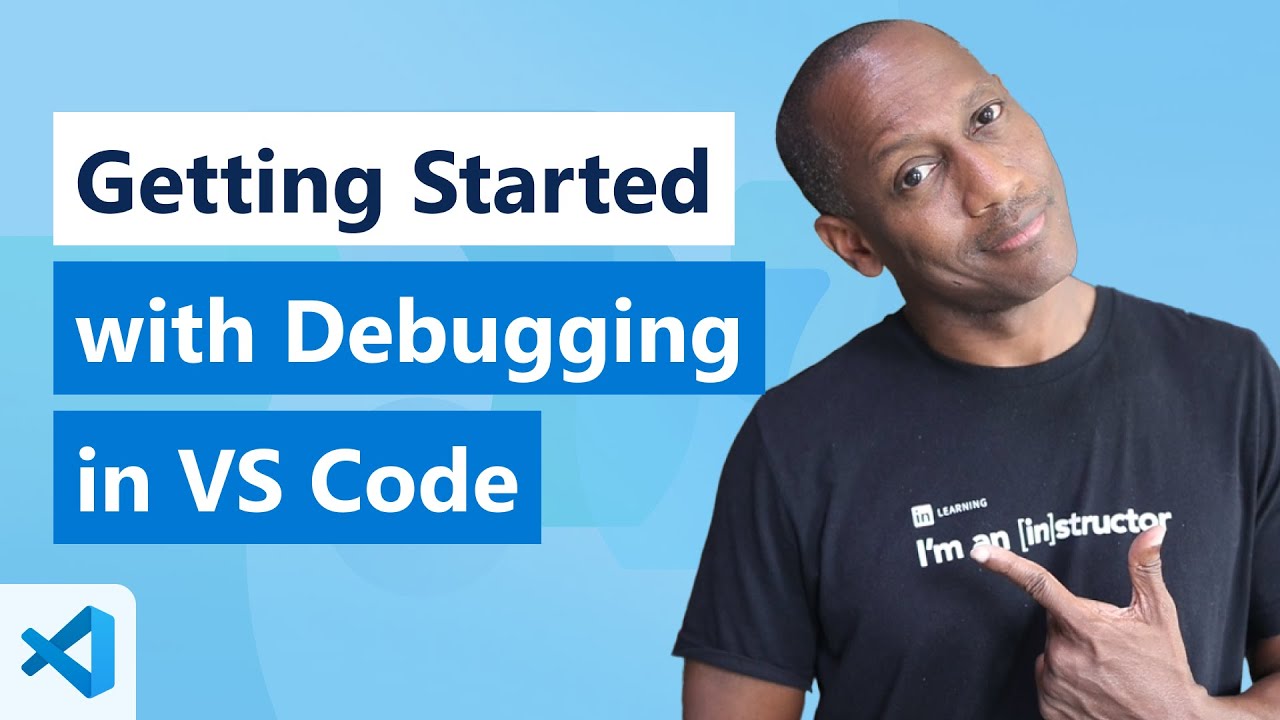
Getting Started with Debugging in VS Code (Official Beginner Guide)
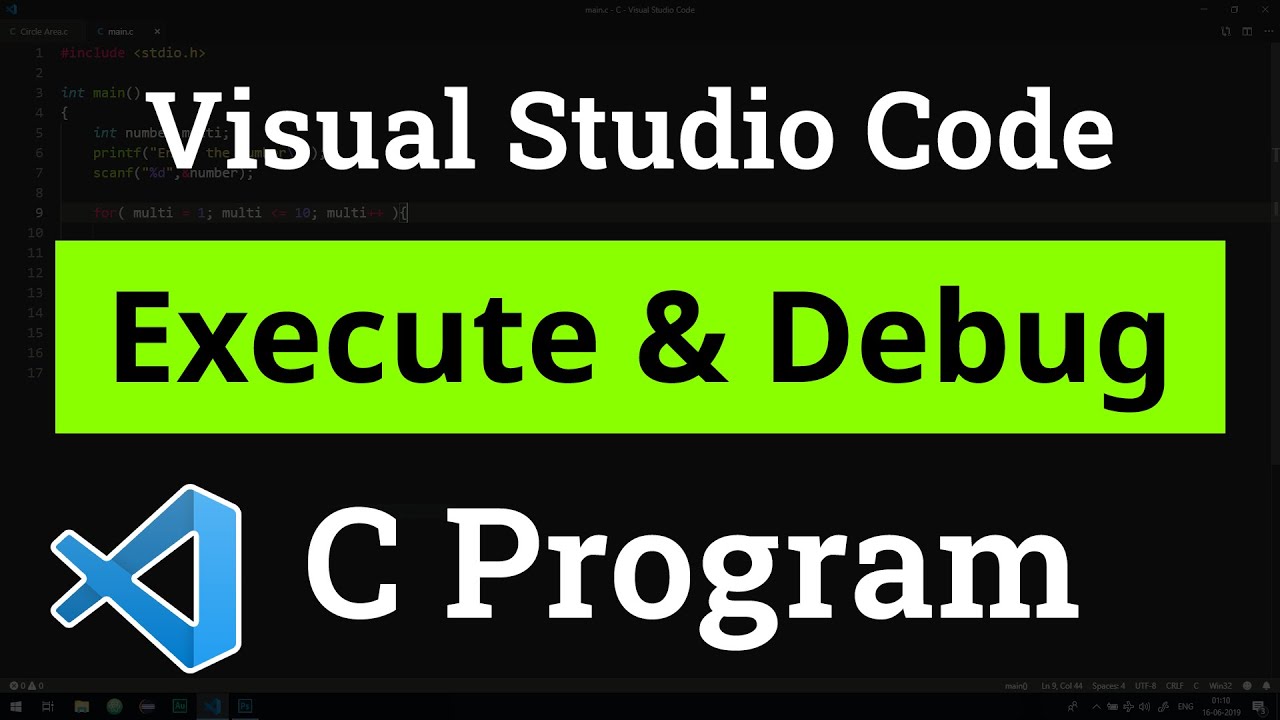
How to set up Visual Studio Code for Executing and Debugging C Programs | Tutorial
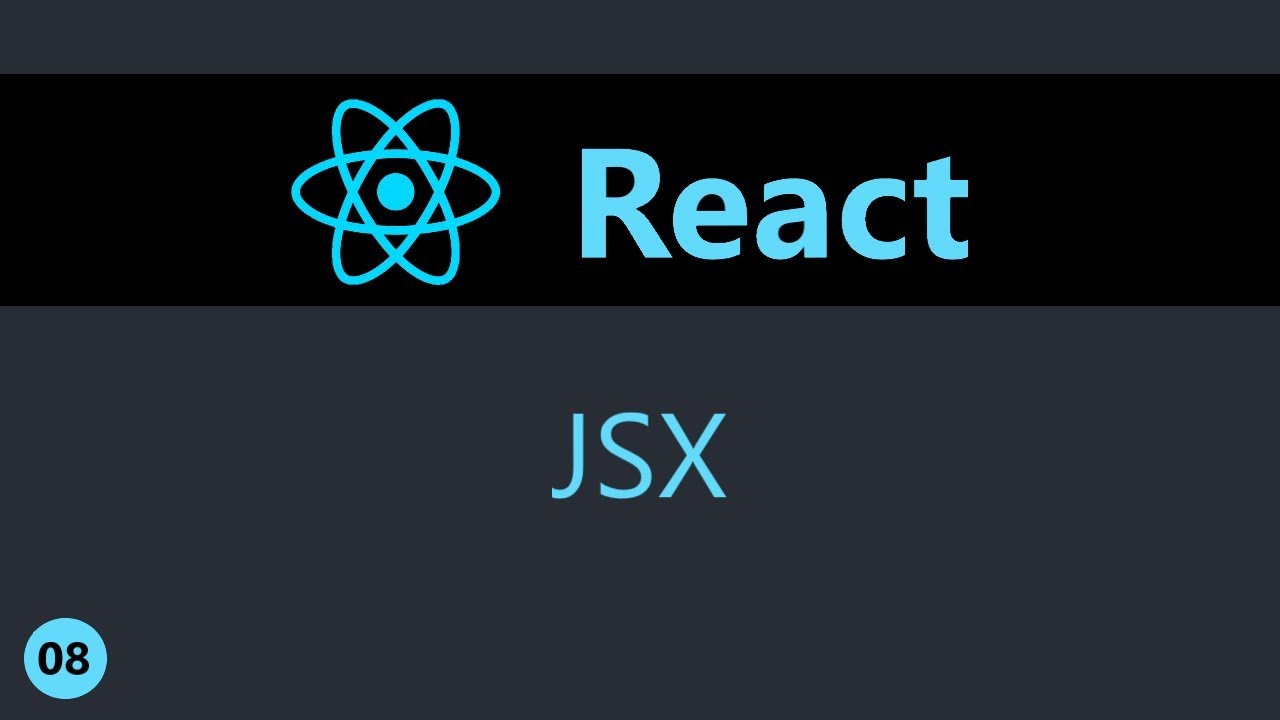
ReactJS Tutorial - 8 - JSX
5.0 / 5 (0 votes)