C_80 Void Pointer in C | Detailed explanation with program
Summary
TLDRThis video script delves into the concept of void pointers in C programming. It explains that a void pointer, akin to an empty glass that can be filled with any liquid, is a generic pointer without an associated data type. The script teaches how to declare and use void pointers, emphasizing the need for type casting when dereferencing to access values of different data types. The practical demonstration shows using a single void pointer to access variables of various types, highlighting its utility in simplifying code and handling dynamic memory allocation.
Takeaways
- 😀 The video discusses special pointers in C programming, focusing on void pointers.
- 🔍 A void pointer in C is a generic pointer that has no associated data type, similar to an empty glass that can be filled with any liquid.
- 📚 The term 'void' generally means 'empty' or 'nothing', and a void pointer is used to point to any data type.
- 💡 Declaring a void pointer involves using the 'void' keyword in the pointer declaration, such as `void *vp;`.
- 🚫 A void pointer cannot be dereferenced directly; it must be typecast to a specific data type before being used.
- 🔄 The flexibility of a void pointer allows it to be converted into any other pointer type, making it useful for various programming scenarios.
- 💻 The video demonstrates how to use a void pointer in a program, showing how it can store the address of different data types like int, float, and char.
- 🔑 Typecasting a void pointer is essential for accessing the value it points to, as the compiler needs to know the data type to correctly interpret the memory.
- 📈 The practical use of void pointers is highlighted in the video, showing how they can be used to access multiple data types with a single pointer.
- 🔍 The video also mentions that void pointers are used in dynamic memory allocation functions like malloc and calloc, which return void pointers to the allocated memory.
Q & A
What is a void pointer in C?
-A void pointer in C is a pointer that has no associated data type. It is essentially a generic pointer that can point to any data type. It is declared using the 'void' keyword and can be typecast to any other pointer type.
Why are void pointers useful in programming?
-Void pointers are useful because they can be used as a generic pointer to any data type. This allows a single pointer to be used to access different data types, which can be convenient in situations like dynamic memory allocation where the data type is not known at compile time.
How do you declare a void pointer in C?
-A void pointer is declared in C using the 'void' keyword followed by an asterisk and the pointer name. For example, `void *vp;` declares a void pointer named 'vp'.
Can you directly dereference a void pointer?
-No, you cannot directly dereference a void pointer. Before dereferencing, you must typecast the void pointer to the appropriate data type pointer (e.g., `int *`, `float *`, `char *`) to ensure the compiler knows how many bytes to access.
What is the significance of the term 'void' in the context of a void pointer?
-The term 'void' in the context of a void pointer signifies that the pointer does not have an associated data type. It can be thought of as an 'empty' or 'generic' pointer that can be filled with any type of data.
How does a void pointer differ from other pointers like integer pointer, float pointer, or character pointer?
-A void pointer differs from other pointers in that it does not have a specific data type associated with it. Other pointers, such as integer pointers (`int *`), float pointers (`float *`), or character pointers (`char *`), are associated with specific data types and can only point to variables of those types.
What is the purpose of typecasting a void pointer?
-Typecasting a void pointer is necessary to specify the data type of the variable it points to. This is required before dereferencing the pointer so that the compiler knows how many bytes to access and can correctly interpret the data.
Can a void pointer store the address of any variable?
-Yes, a void pointer can store the address of any variable, regardless of its data type. However, before accessing the data at that address, the void pointer must be typecast to the appropriate pointer type corresponding to the data type of the variable.
What are some common uses of void pointers in C?
-Void pointers are commonly used in situations where the data type is not known until runtime, such as in dynamic memory allocation with functions like `malloc` and `calloc`. They are also used in generic functions or APIs where the function needs to handle data of various types.
How does the concept of a void pointer relate to dynamic memory allocation in C?
-In C, functions like `malloc` and `calloc` return a void pointer. This void pointer is then typecast to the appropriate pointer type by the programmer to store and access dynamically allocated memory. This allows for flexible memory management where the data type can be specified at runtime.
Outlines
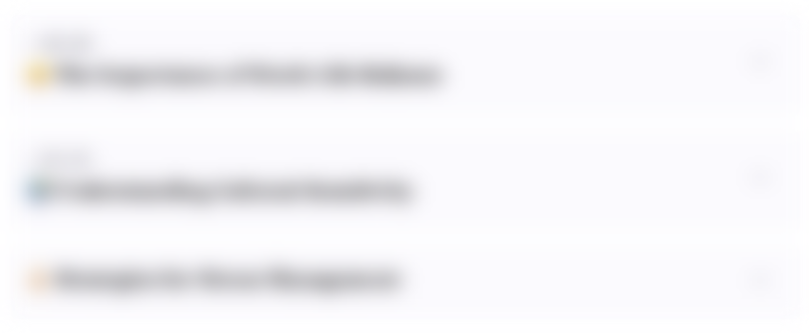
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
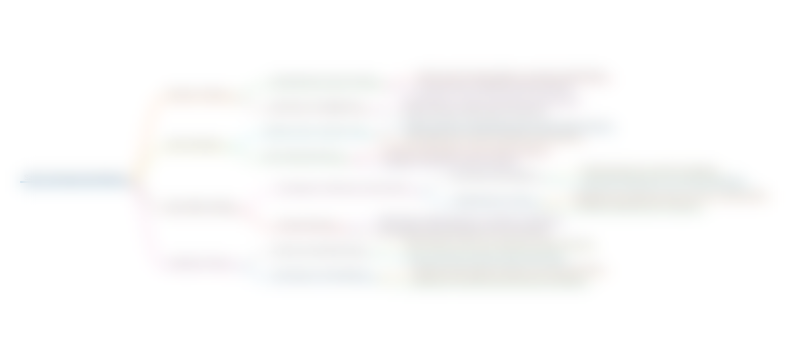
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
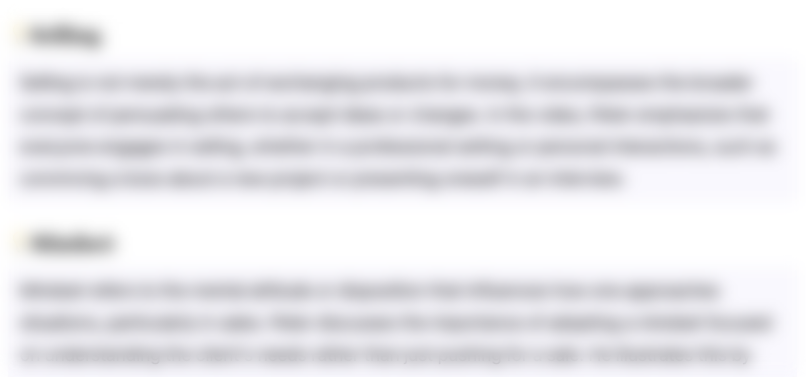
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
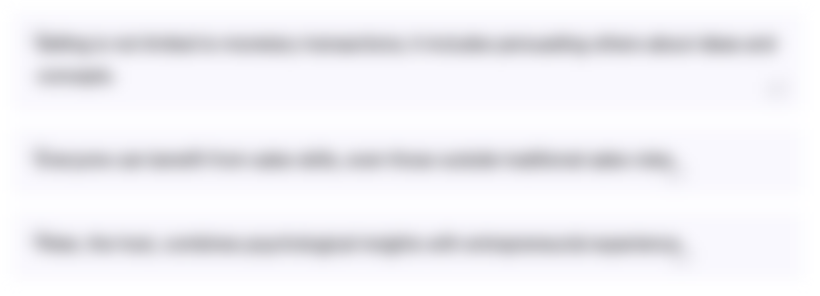
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
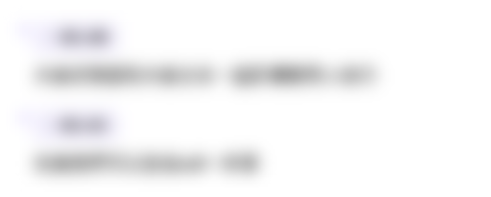
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآن5.0 / 5 (0 votes)