The What, How, and Why of Void Pointers in C and C++?
Summary
TLDRIn this educational video, the concept of void pointers is demystified for beginner programmers. The host explains that void pointers, which lack type information, essentially point to 'nothing' or rather, no specific type. They serve as a flexible way to store any memory address without making assumptions about the data it points to. The video illustrates void pointers' functionality with examples, including using them with memory allocation functions like malloc and free, which return and accept void pointers respectively. The host also highlights the difference in handling void pointers between C and C++, where C++ requires an explicit cast due to its stricter type safety. The aim is to clarify the use of void pointers and to help viewers overcome the initial confusion they might face when encountering them in programming.
Takeaways
- 😀 The video is aimed at beginner programmers who are familiar with pointers but encounter confusion with void pointers.
- 🔍 The term 'void' in programming is first encountered in the context of functions that do not return any value.
- 🤔 A void pointer is a pointer that does not have type information, essentially a pointer to 'nothing' or 'no type'.
- 📌 Void pointers are used to store any type of address without making assumptions about the data stored at that address.
- 💡 The size of a void pointer is the same as any other pointer on a given system, as they all store addresses.
- 🚫 You cannot directly dereference a void pointer because it lacks type information, but you can cast it to a specific pointer type to dereference it.
- 🔧 Void pointers are useful in scenarios where you need to store generic memory addresses, such as with memory allocation functions like malloc.
- 🛠️ Functions like malloc and free use void pointers because they are concerned with the size of the memory block rather than the type of data it holds.
- 🔄 When using void pointers, it's important to cast them to the appropriate pointer type before dereferencing to avoid errors.
- ⚠️ There is a difference in how C and C++ handle assigning void pointers to other pointer types, with C being more lenient and C++ requiring an explicit cast.
Q & A
What is the main topic discussed in the video?
-The main topic discussed in the video is void pointers, their purpose, and how they are used in programming, particularly in C and C++.
What does the term 'void' signify in the context of a function?
-In the context of a function, 'void' signifies that the function does not have a result type and does not produce a value upon completion.
What is a void pointer according to the video?
-A void pointer is a pointer that does not have type information attached to it. It stores an address in memory without making any assumptions about what is stored at that location.
Why might someone want to use a void pointer?
-One might want to use a void pointer when they want to store generic memory addresses without caring about the type of data those addresses point to.
How does the size of a void pointer compare to other pointers?
-The size of a void pointer is the same as other pointers, as they all store an address in memory, which is typically the size of a memory address on the system (e.g., 8 bytes on the presenter's machine).
What is the issue with dereferencing a void pointer directly?
-The issue with dereferencing a void pointer directly is that the compiler does not have type information, so it cannot perform operations on the data at the address the void pointer is storing.
How can a void pointer be made to work like a regular pointer?
-A void pointer can be made to work like a regular pointer by casting it to a specific pointer type, which provides the necessary type information for the compiler to perform operations on the data.
What is the practical use of void pointers in memory allocation?
-Void pointers are used in memory allocation functions like malloc, because these functions return a generic pointer to a block of memory without caring about the type of data that will be stored in it.
How does the video demonstrate the use of void pointers with malloc and free?
-The video demonstrates assigning a void pointer to the result of malloc to dynamically allocate memory and then casting the void pointer to an int pointer before using it. It also shows passing a void pointer to the free function to deallocate memory.
What is the difference between how C and C++ handle assigning a void pointer to another pointer type?
-C allows assigning a void pointer to another pointer type without a cast, while C++ requires an explicit cast to acknowledge the change from one pointer type to another, reflecting C++'s stronger type safety.
Why is it important to understand the difference between C and C++ when using void pointers?
-Understanding the difference is important for writing portable code that works in both languages and for avoiding type-related bugs that can occur when pointers are not properly cast.
Outlines
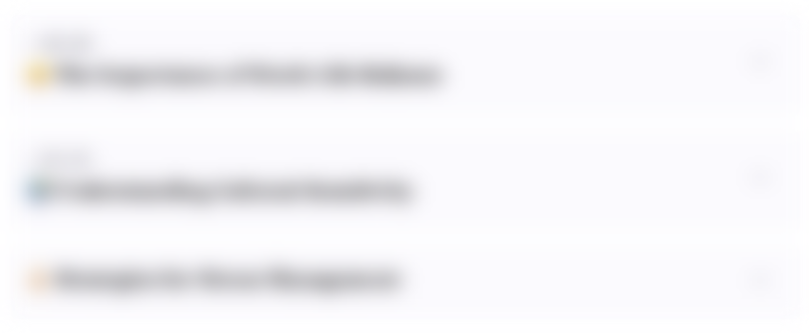
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
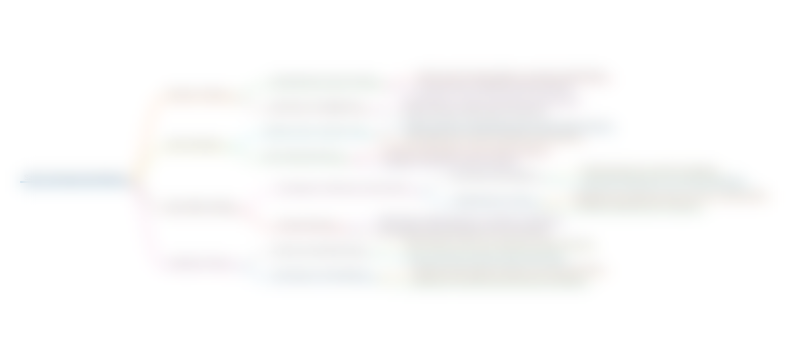
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
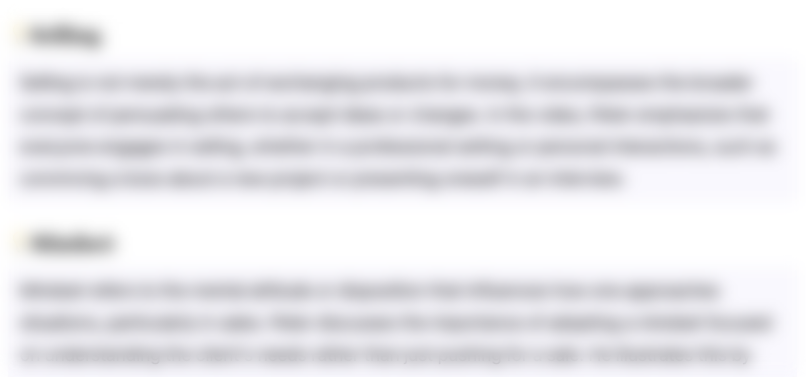
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
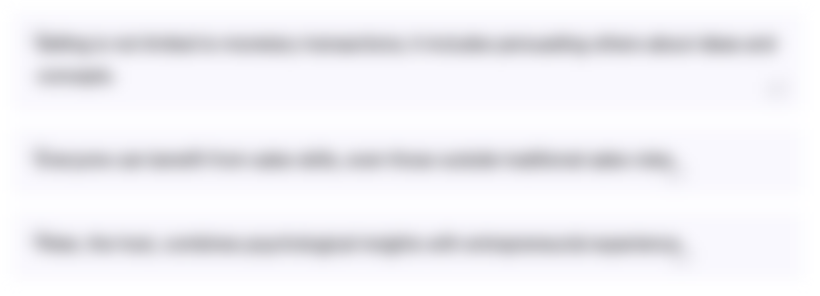
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
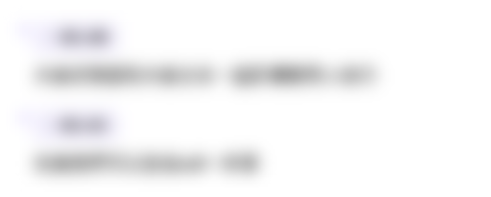
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
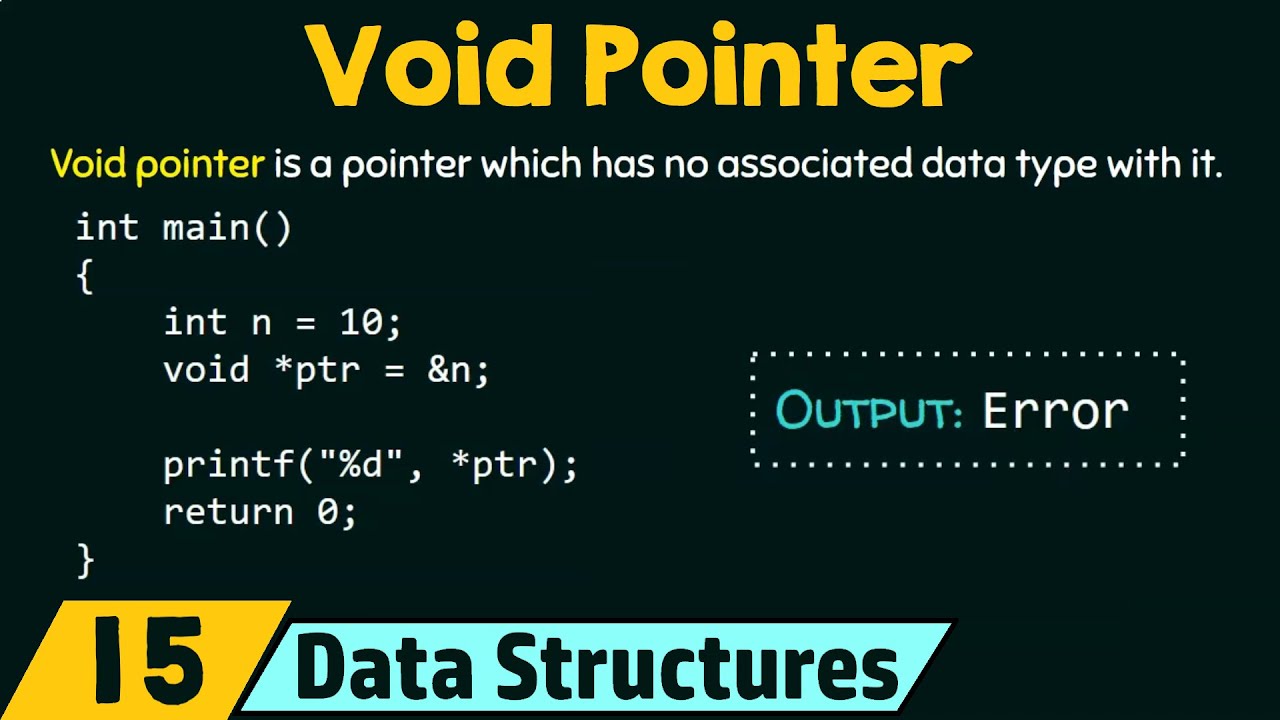
Understanding the Void Pointers

C_80 Void Pointer in C | Detailed explanation with program
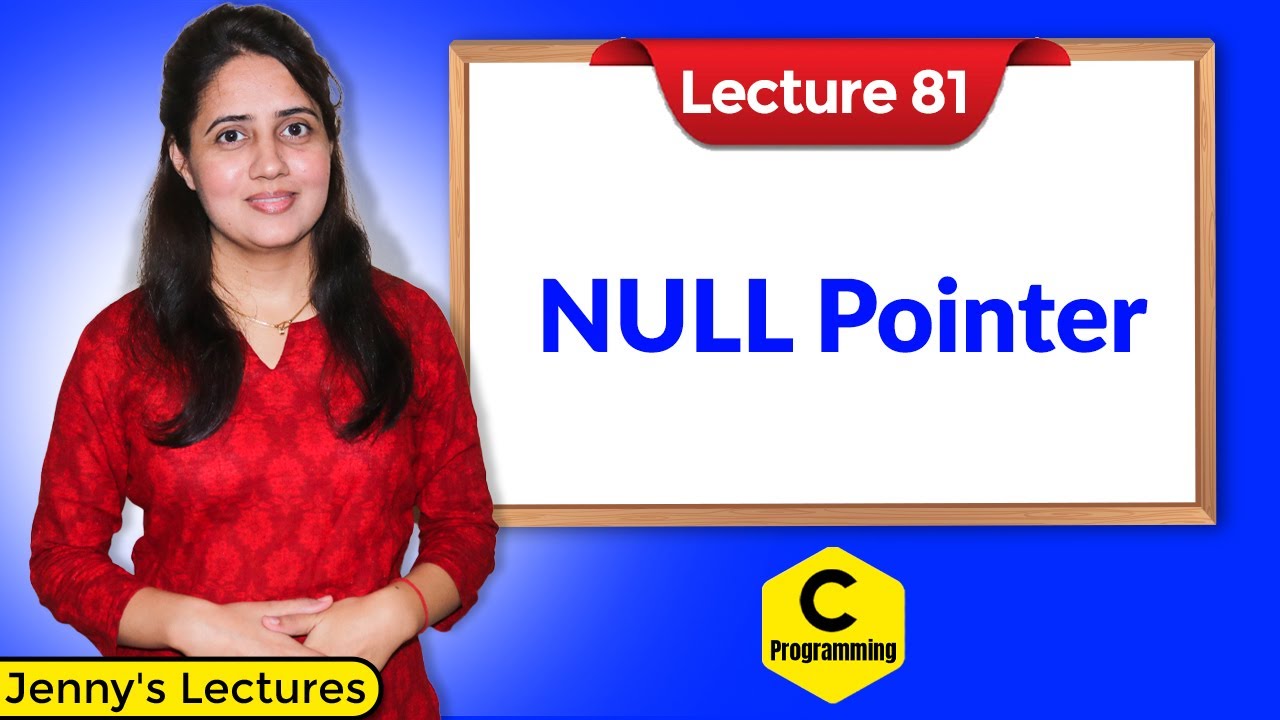
C_81 Null Pointer in C | C Programming Tutorials

DAY 30 | COMPUTER SCIENCE | II PUC | POINTERS | L1
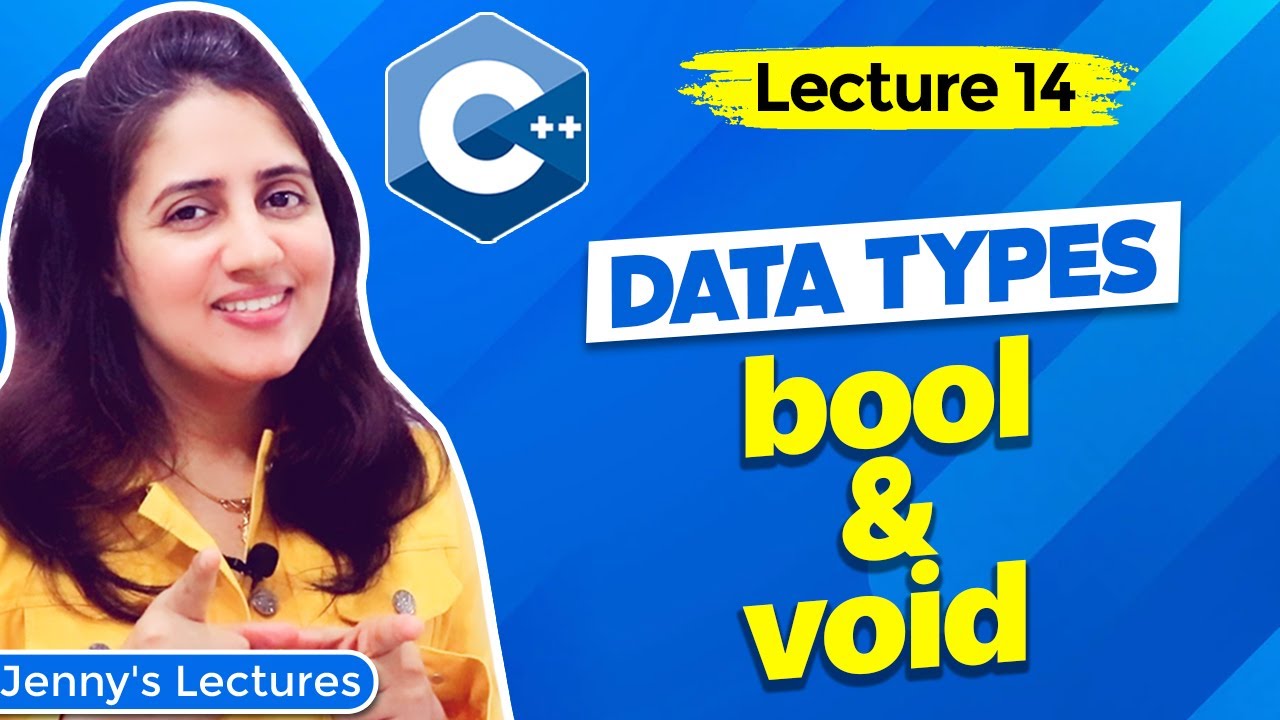
Lec14: bool and void Data Types in C++ | C++ Tutorials for beginners
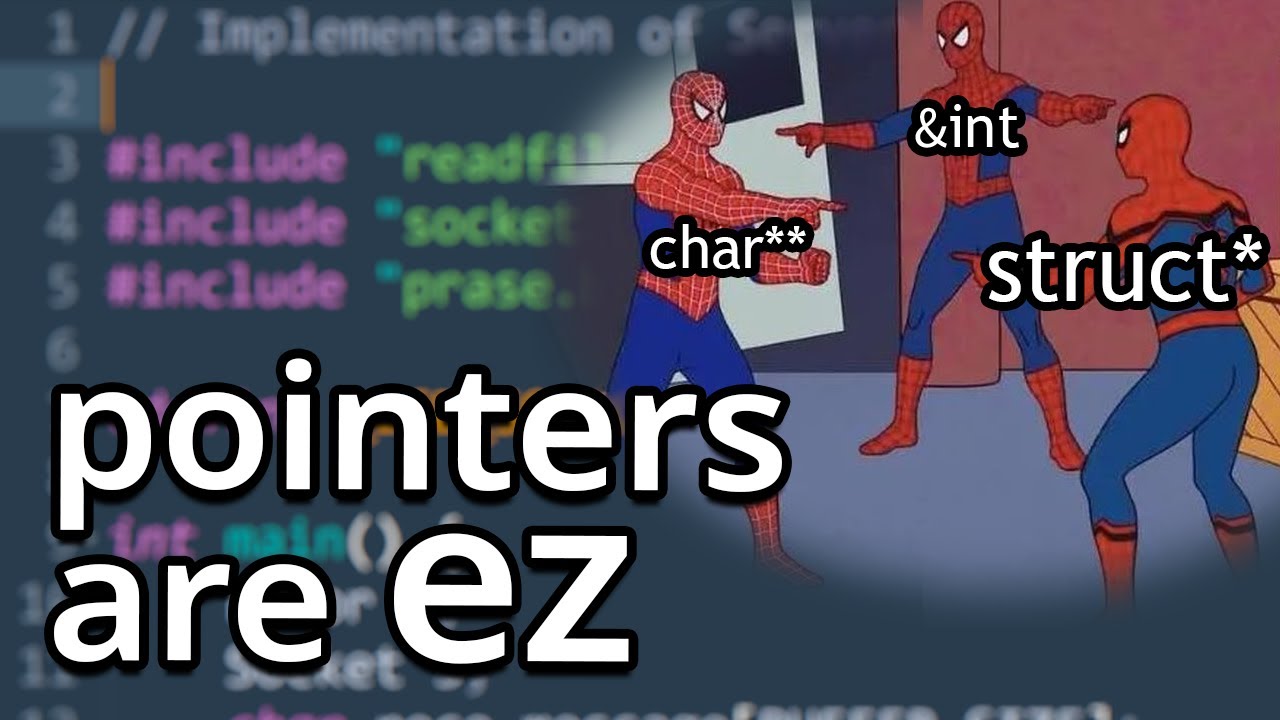
you will never ask about pointers again after watching this video
5.0 / 5 (0 votes)