C_71 Pointers in C - part 1| Introduction to pointers in C | C Programming Tutorials
Summary
TLDRThis lecture introduces pointers in C programming, covering their definition, need, declaration, and initialization. It clarifies common misconceptions, emphasizing pointers as simple yet special variables that store addresses of other variables. The lecture explains pointer declaration syntax, initialization using the address-of operator, and memory allocation. It also highlights the importance of data types in pointer assignments and the risks of uninitialized pointers. Practical examples and exercises are provided to reinforce understanding. The lecture sets the stage for deeper exploration of pointers, including dereferencing and complex pointer-related programs in subsequent lessons.
Takeaways
- 📌 Pointers are special variables that contain the address of other variables.
- 📌 Pointers are not inherently difficult; they become simple once you understand the basics.
- 📌 Variables in C have a name, value, and address, which are fundamental to understanding pointers.
- 📌 The general syntax to declare a pointer in C is: `data type *pointer_name`.
- 📌 Pointers can only store addresses of variables of the same data type they are declared to point to.
- 📌 Declaring a pointer and initializing it can be done in a single step or separately.
- 📌 Uninitialized pointers can point to unknown locations and are risky to use.
- 📌 The data type in the pointer declaration specifies the type of variable whose address the pointer will store.
- 📌 Proper pointer initialization is crucial to avoid errors and ensure correct program output.
- 📌 Using the dereferencing operator (*) allows access to the value stored at the address the pointer is pointing to.
Q & A
What is a pointer in C?
-A pointer in C is a special variable that contains the address of another variable.
Why are pointers considered special variables?
-Pointers are considered special variables because they store the address of another variable rather than a direct value like integers or floats.
What are the three main components associated with a variable in C?
-The three main components associated with a variable in C are the variable's name, its value, and its address.
How do you declare a pointer in C?
-A pointer in C is declared using the syntax: data_type *pointer_name. For example, int *p declares a pointer to an integer.
What does the data type in a pointer declaration indicate?
-The data type in a pointer declaration indicates the type of variable whose address the pointer will store. For example, int *p means that the pointer p will store the address of an integer variable.
How do you initialize a pointer in C?
-A pointer is initialized by assigning it the address of a variable using the address-of operator (&). For example, if int a = 10, then int *p = &a initializes the pointer p with the address of the variable a.
What is the difference between declaring and initializing a pointer?
-Declaring a pointer allocates memory for it and specifies its type, whereas initializing a pointer assigns it the address of an appropriate variable.
What is the base address of a variable, and why is it important for pointers?
-The base address of a variable is the address of its first byte in memory. It is important for pointers because pointers store this base address to refer to the variable.
How do you use a pointer to access the value of the variable it points to?
-You use the dereferencing operator (*) to access the value of the variable a pointer points to. For example, if int *p = &a, then *p gives the value of a.
Why must you be careful with uninitialized pointers?
-Uninitialized pointers can point to any memory location, leading to undefined behavior or program crashes. It is essential to initialize pointers before using them.
Outlines
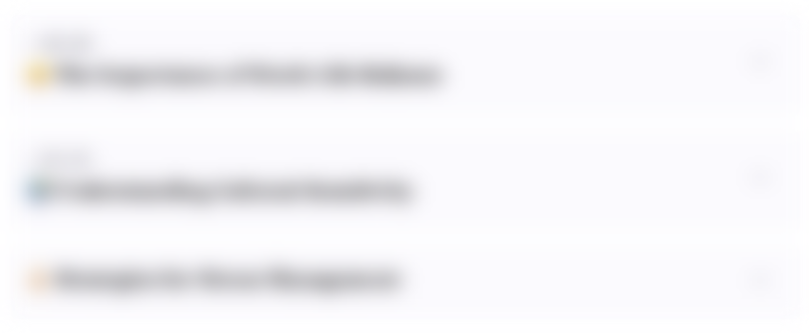
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
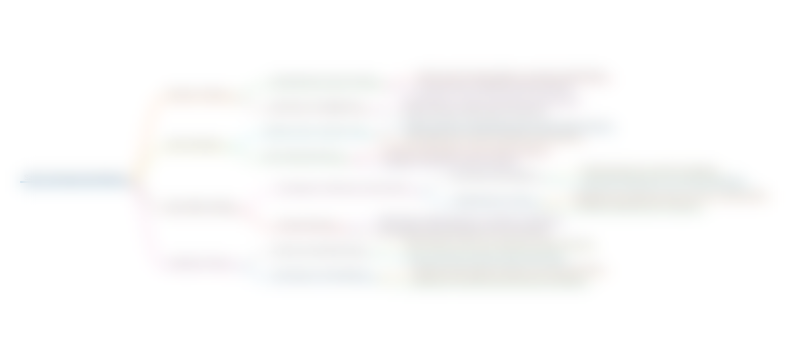
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
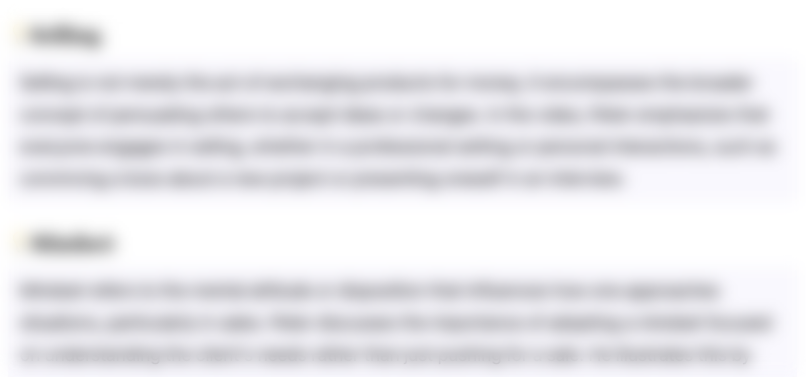
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
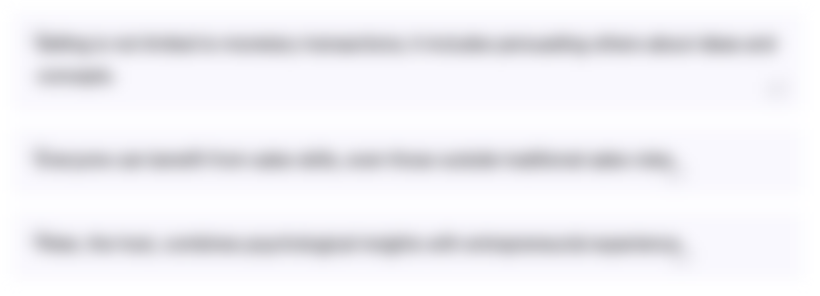
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
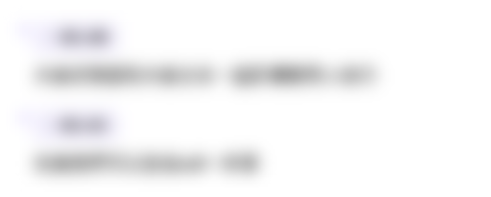
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)