Declaring & Initializing Pointers in C
Summary
TLDRThis presentation explains how to declare and initialize pointers in C programming. It covers the general syntax for pointer declaration, highlighting that a pointer doesn't have its own data type but points to the address of another object. Examples include pointers to integers, characters, and floats. The video also demonstrates how to initialize pointers using the address-of operator (&) by assigning the memory address of a variable to a pointer. Finally, it emphasizes that both declaration and initialization can be combined into a single statement for simplicity.
Takeaways
- π The general syntax for declaring a pointer in C involves specifying the data type, followed by an asterisk (*), and then the pointer variable name.
- π The data type in a pointer declaration refers to the type of value that the pointer will point to, not the pointer itself.
- β‘οΈ A pointer variable doesn't have its own data type; it stores the address of another object, so the type is defined by the object it points to.
- π’ For example, 'int *ptr' means that 'ptr' is a pointer to an integer value, and similarly, 'char *ptr' means it's a pointer to a character.
- βοΈ Declaring a pointer alone is not enough; it must be initialized before use, typically with the address of a variable.
- π The address of a variable can be assigned to a pointer using the address-of operator ('&'), e.g., 'ptr = &x;' where 'x' is an integer variable.
- π§ In the example, if 'x' contains the value 5 and its address is 1000, assigning '&x' to 'ptr' allows the pointer to reference this memory location.
- π‘ Both the variable and the pointer occupy memory, and each has its own base address (e.g., 'x' at 1000 and 'ptr' at 2000).
- π Multiple pointer operations can be combined into a single statement, both for declaration and initialization, making the code more concise.
- π― Assigning the address during the declaration itself helps avoid writing redundant lines of code, e.g., 'int *ptr = &x;' instead of separate steps.
Q & A
What is the general syntax for declaring a pointer variable in C?
-The general syntax for declaring a pointer variable involves specifying the data type, followed by an asterisk (*), and then the name of the pointer. For example, `int *ptr` declares a pointer `ptr` that points to an integer.
Why doesn't a pointer have its own data type?
-A pointer does not have its own data type because it always contains the address of another object. The data type specified for the pointer refers to the type of value that the pointer will point to, not the pointer itself.
What does the statement `int *ptr` mean?
-The statement `int *ptr` means that `ptr` is a pointer variable that will point to an integer value.
How do you initialize a pointer variable in C?
-A pointer is initialized by assigning it the address of another variable using the address-of operator (`&`). For example, if `x` is a variable, the pointer can be initialized as `ptr = &x`, which assigns the address of `x` to the pointer `ptr`.
What is the role of the address-of operator (&) in pointer initialization?
-The address-of operator (`&`) is used to obtain the memory address of a variable. This address is then assigned to the pointer during initialization, allowing the pointer to reference the memory location of the variable.
How can the declaration and initialization of a pointer be combined into one statement?
-The declaration and initialization of a pointer can be combined into one statement by declaring the pointer and assigning it the address of a variable simultaneously. For example, `int *ptr = &x;` declares the pointer `ptr` and initializes it with the address of the variable `x` in a single line.
What does the expression `ptr = &x` do?
-The expression `ptr = &x` assigns the address of the variable `x` to the pointer `ptr`. This means that `ptr` now points to the memory location where `x` is stored.
Can the base address of a variable and a pointer be different?
-Yes, the base address of a variable and a pointer will typically be different because they occupy different memory locations. For instance, in the example, the variable `x` might have a base address of 1000, while the pointer `ptr` could have a base address of 2000.
Why is it important to initialize a pointer before using it?
-It is important to initialize a pointer before using it because an uninitialized pointer may contain a garbage value, which could lead to the pointer referencing an invalid or unknown memory location, potentially causing undefined behavior or a program crash.
What does it mean when a pointer points to a specific memory location?
-When a pointer points to a specific memory location, it means that the pointer holds the address of a variable stored in that memory location. The pointer can then be used to access or modify the value stored at that address.
Outlines
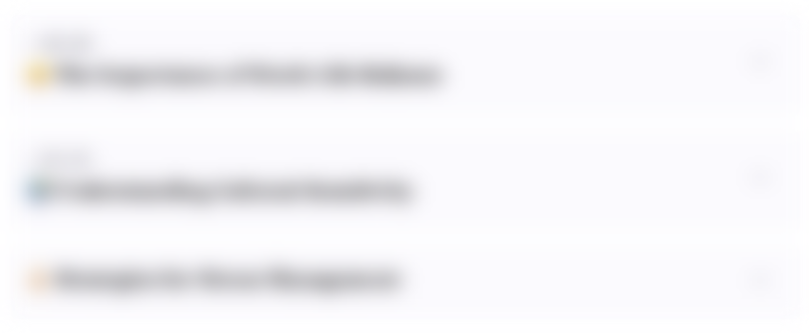
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
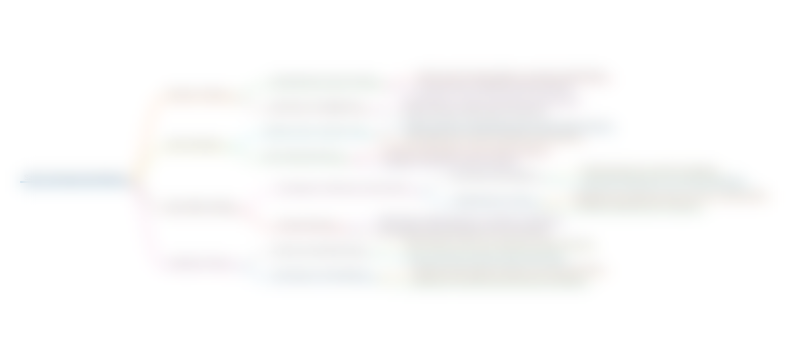
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
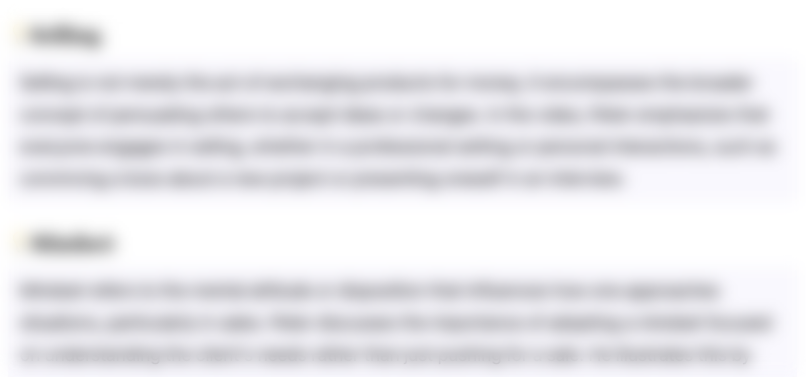
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
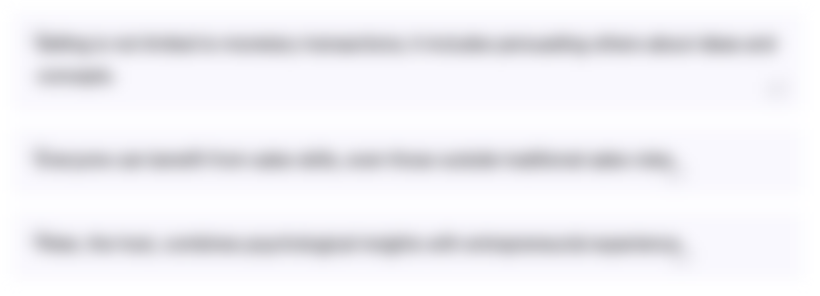
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
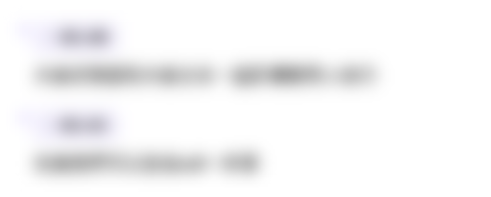
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
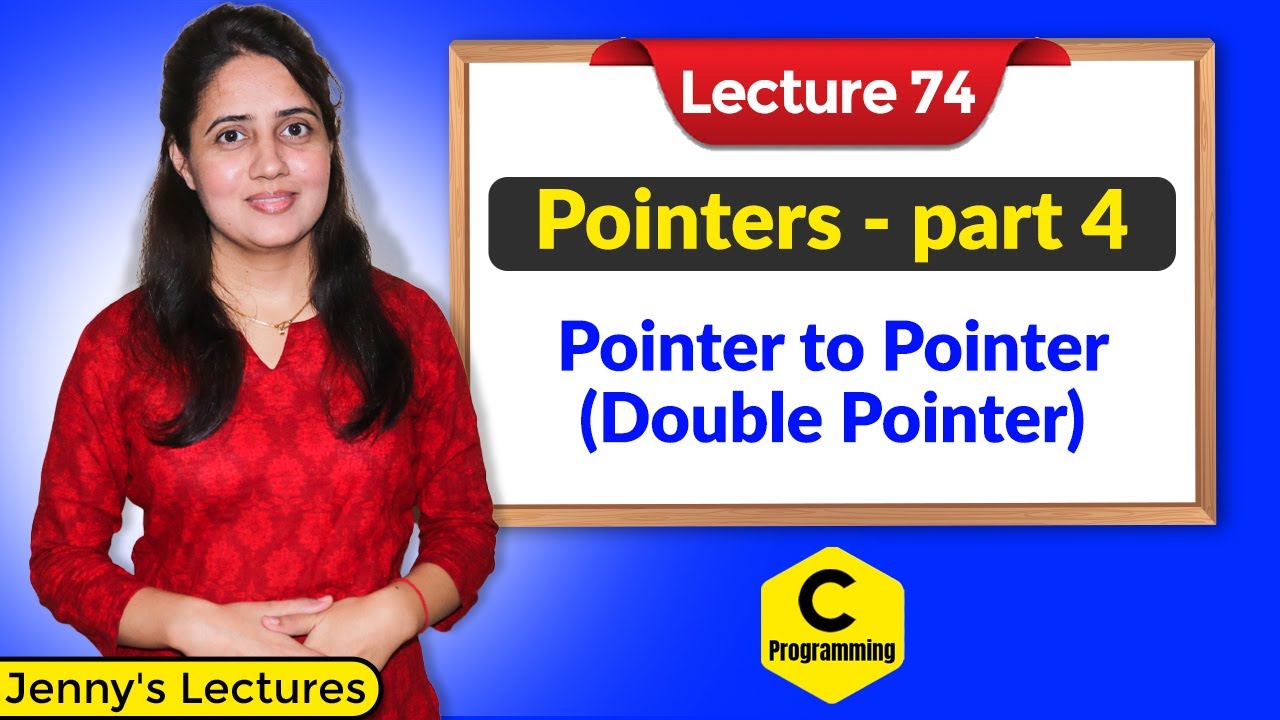
C_74 Pointers in C- part 4 | Pointer to Pointer (Double Pointer)
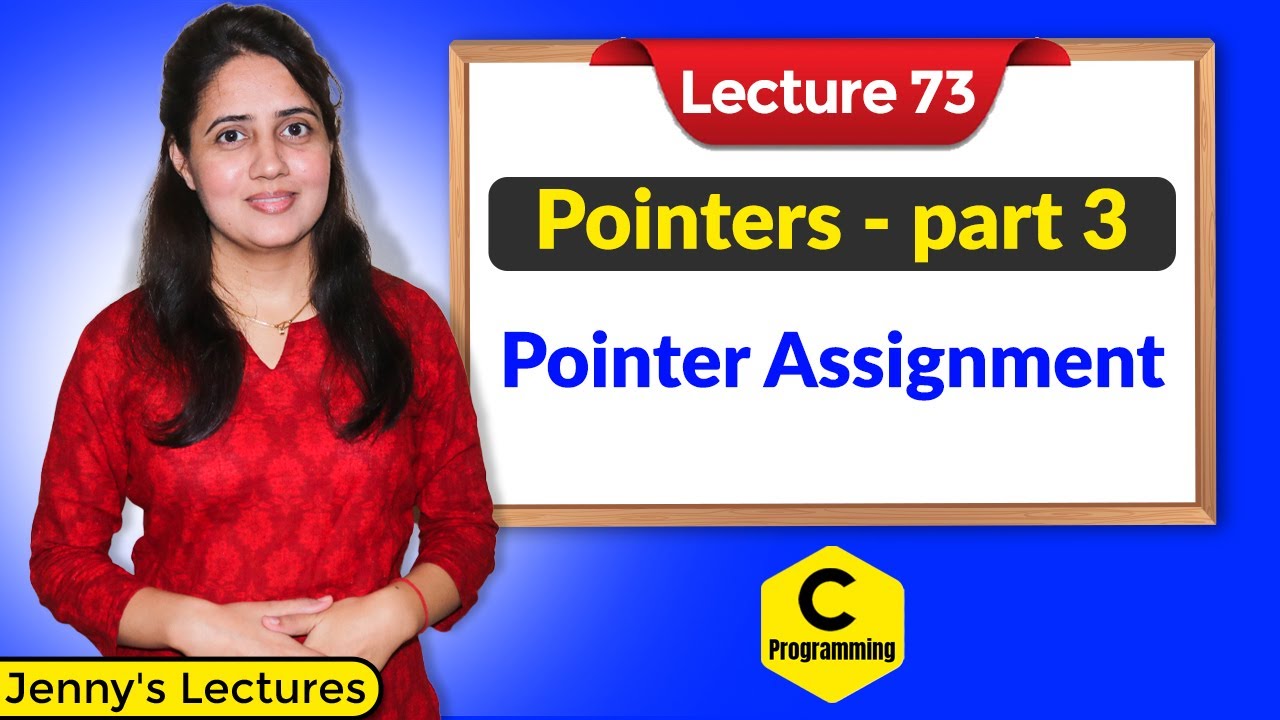
C_73 Pointers in C- part 3 | Pointer Assignment
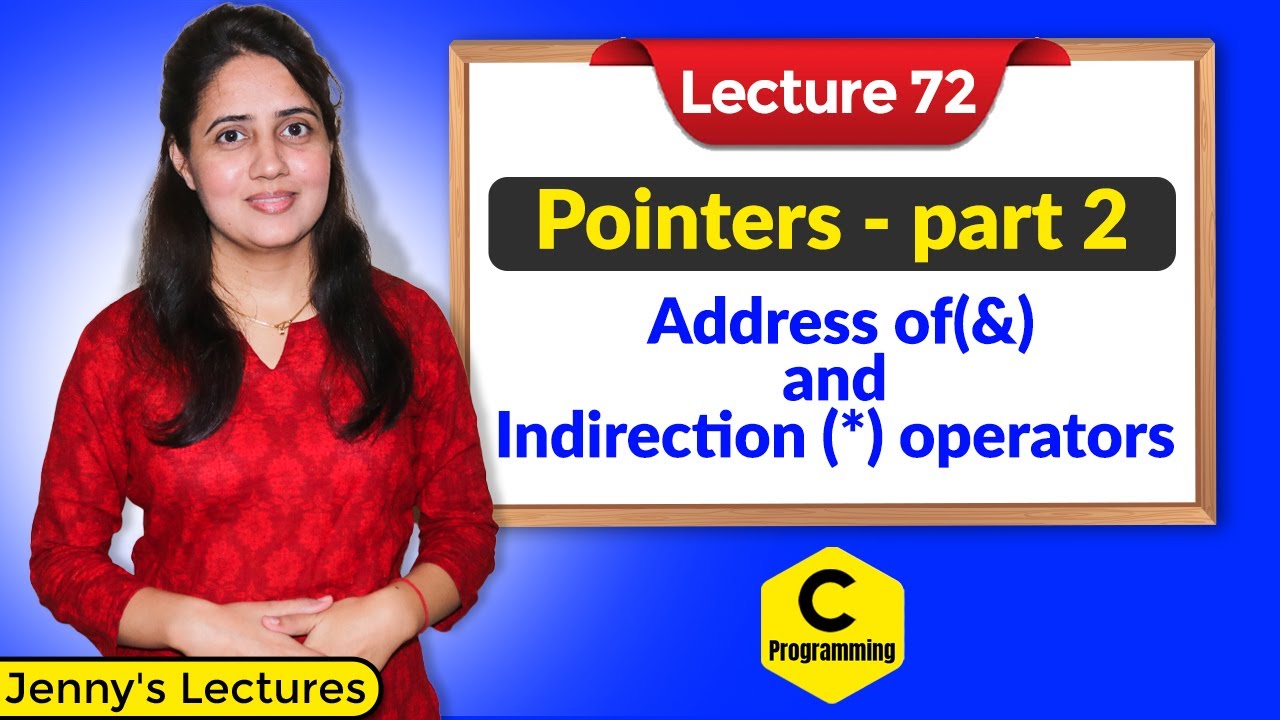
C_72 Pointers in C- part 2 |Address of(&) and Indirection (*) operator in Pointers I C Programming
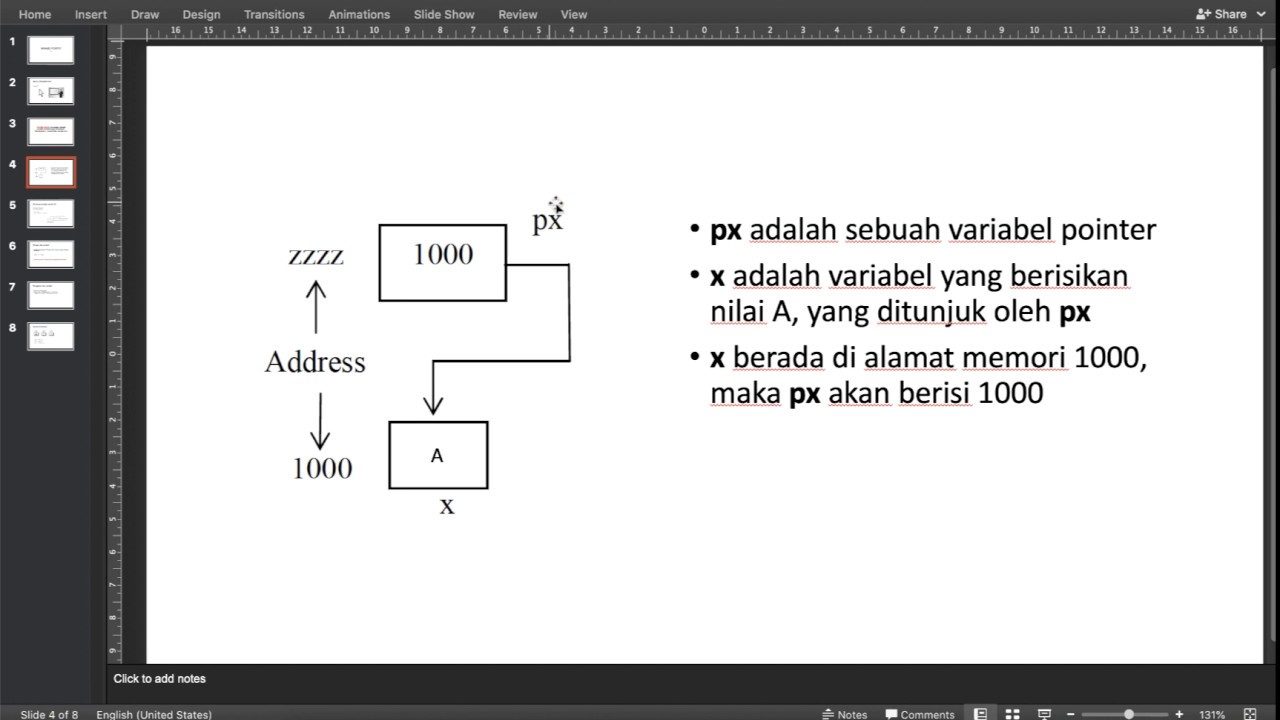
Variabel Pointer
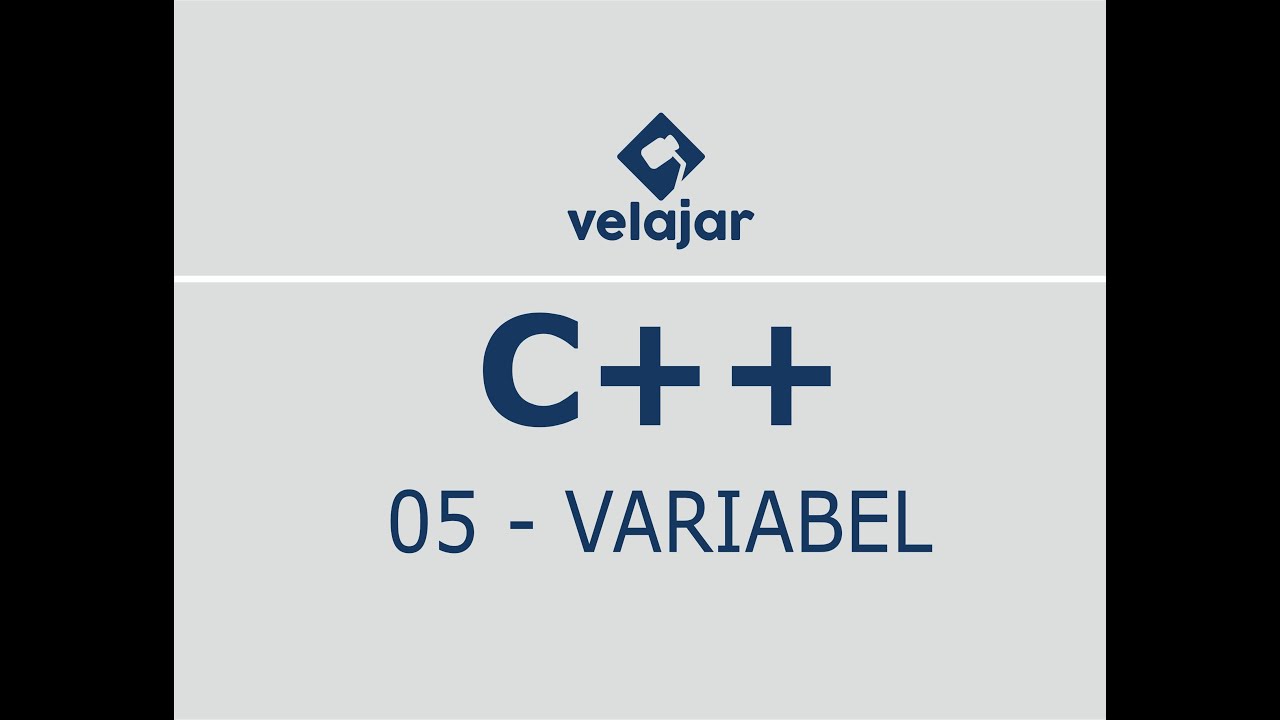
Variabel #5 | C++ | Bahasa Indonesia

C_80 Void Pointer in C | Detailed explanation with program
5.0 / 5 (0 votes)