Variables and Datatypes
Summary
TLDRThis lecture introduces essential concepts of variables and data types in Python, emphasizing the importance of variable naming conventions such as being short, descriptive, and avoiding conflicts with built-in functions. It covers data type identification, verification, and coercion, explaining how Python handles different data types like Boolean, integer, complex, float, and string. The lecture also distinguishes between statically and dynamically typed languages, highlighting Python's dynamic nature, and provides practical examples to demonstrate type checking and conversion.
Takeaways
- 📝 Variables are named using the assignment operator `=` and should be short, descriptive, and avoid clashing with built-in functions.
- 🔡 Variable names should be alphanumeric and can start with letters or underscores, but not numbers or special characters.
- 🔑 Avoid using single-character variable names; they are typically used in loops and functions.
- 🔠 Python variable names can be in different cases like camelCase, snake_case, and PascalCase, but not with hyphens.
- 🚫 Do not start or end variable names with an underscore, as it's against common naming conventions, even though the compiler allows it.
- 🔢 Multiple values can be assigned to variables in a single line, allowing for sequential assignment.
- 🔽 Python has basic data types: Boolean (bool), Integer (int), Complex (complex), Float (float), and String (str).
- 📊 Python is a dynamically typed language, meaning the data type of a variable is known at runtime, not at compile time.
- 🔍 The `type()` function is used to identify the data type of an object in Python.
- 🤖 To verify an object's data type, use the expression `type(variable) is data_type`, which returns a Boolean value.
- 🔄 Data types can be coerced using type conversion functions like `int()`, `float()`, and `str()`, but some coercions are not possible, such as converting a string of text to a number.
Q & A
What is the purpose of naming variables in programming?
-The purpose of naming variables is to convey information and intent, making the code more understandable and maintainable.
What are some common rules for naming variables in Python?
-Variable names should be short and descriptive, avoid clashing with built-in functions, and not start with a number. They can be alphanumeric and may include underscores, but should not begin or end with them.
Why should we avoid using one character variable names in Python?
-One character variable names are typically used in iterations, functions, and looping constructs. Using them for other purposes can lead to confusion and reduce code readability.
What are the different case types mentioned in the script for naming variables?
-The case types mentioned are camelCase (both lower and upper), snake_case, and PascalCase. These are commonly accepted conventions in Python for naming variables.
What is the difference between statically and dynamically typed languages in terms of variable declaration?
-In statically typed languages, the type of a variable is known at compile time and must be declared upfront, as seen in languages like C, C++, and Java. In contrast, dynamically typed languages like Python do not require upfront declaration, and the type is determined at runtime.
How can you determine the data type of a variable in Python?
-You can determine the data type of a variable in Python by using the `type()` function and passing the variable as an argument.
What is the purpose of the `is` keyword when checking the data type of a variable?
-The `is` keyword is used to verify if a variable is of a certain data type. It returns a Boolean value (True or False) indicating whether the variable belongs to the specified data type.
How can you convert the data type of a variable to a new type in Python?
-You can convert the data type of a variable by using the new data type as a function and passing the variable as an argument, e.g., `int(variable)` to convert to an integer.
What are the basic data types discussed in the script?
-The basic data types discussed are Boolean, Integer (int), Complex (complex), Float (float), and String (str).
Why is it important to know the difference between statically and dynamically typed languages when working with data types?
-Knowing the difference helps programmers understand when and how to declare variable types, which affects how they write and manage code, especially when working with different programming languages.
What is an example of a coercion that is not possible in Python?
-Converting a string that contains non-numeric characters to an integer or float is not possible in Python and will result in an error.
Outlines
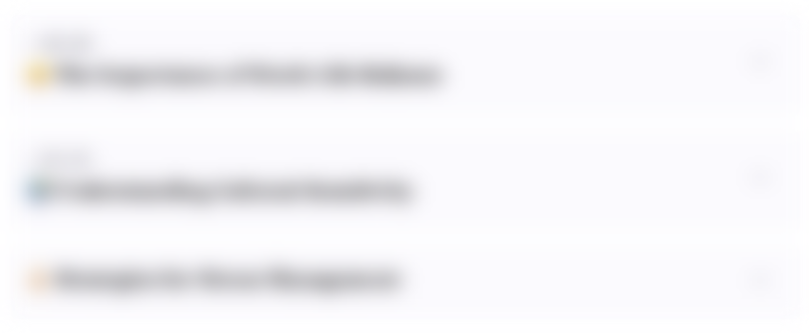
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
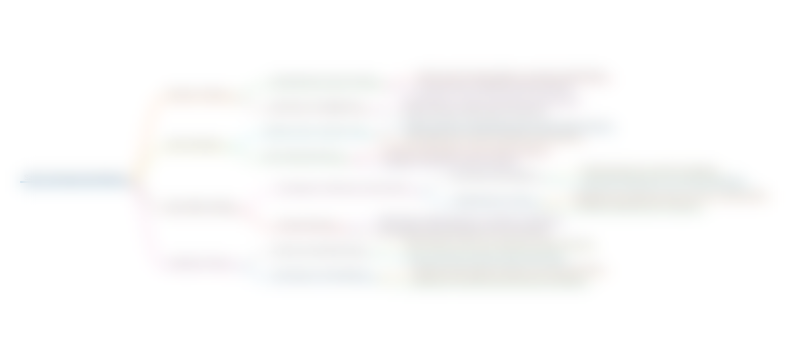
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
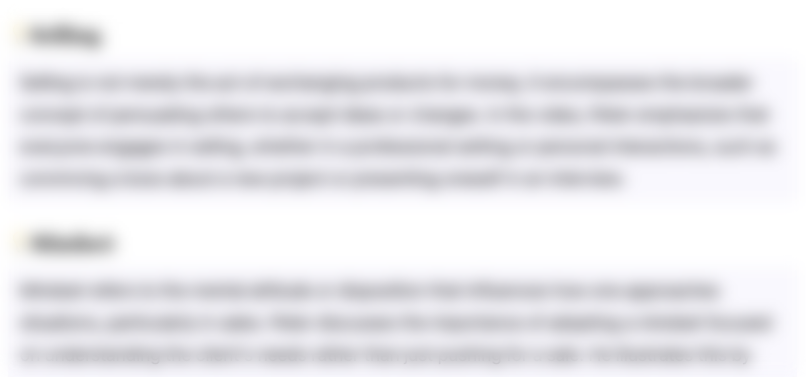
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
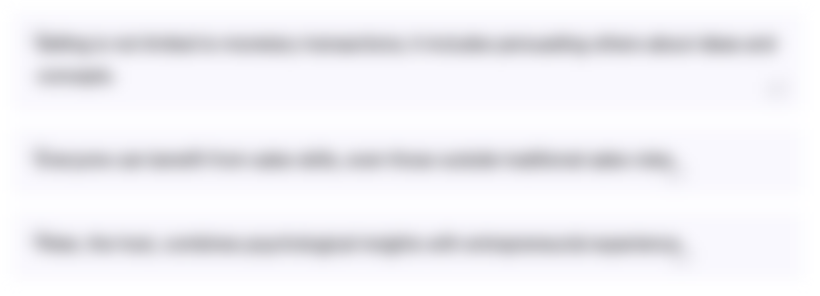
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
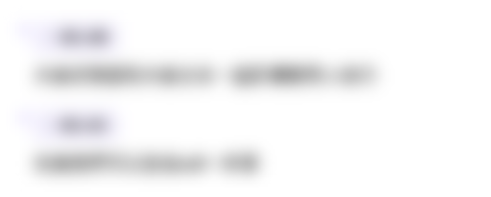
此内容仅限付费用户访问。 请升级后访问。
立即升级5.0 / 5 (0 votes)