GCSE Computer Science Python #1 - Variables, Constants and Assignments
Summary
TLDRThis tutorial explores the fundamentals of Python programming, focusing on variables, constants, and naming conventions. It explains how variables store data types like strings, integers, floats, and booleans, with clear examples. The video highlights the importance of naming variables logically for readability and long-term maintainability, introducing styles like camelCase and snake_case. Additionally, it discusses the distinction between variables and constants, particularly how Python handles constants through uppercase naming conventions. The video encourages hands-on learning by experimenting with different data types and naming practices to solidify understanding of Python programming basics.
Takeaways
- 😀 Variables are memory locations or named addresses that hold values, such as booleans, strings, or integers in Python.
- 😀 A string can be assigned to a variable using single or double quotes, and Python identifies its type as 'str'.
- 😀 Integers in Python represent whole numbers without quotes, whereas strings with quotes are treated as text.
- 😀 Floating-point numbers (floats) use decimal points to store values and are identified as 'float' in Python.
- 😀 Boolean variables in Python can store either 'True' or 'False' values, with case sensitivity being crucial.
- 😀 Variable names should be clear, descriptive, and consistent to ensure readability and maintainability of code.
- 😀 The camel case convention is widely used for variable names, starting with a lowercase letter and capitalizing subsequent words.
- 😀 Alternative naming conventions, such as snake case (e.g., customer_first_name), are also used for readability.
- 😀 Python does not support constants in the traditional sense, but constants can be simulated using uppercase variable names.
- 😀 While Python allows numbers in variable names, they cannot begin with a number, and special characters like '@' are not allowed.
- 😀 Constants (e.g., charger cost) are typically written in uppercase and placed at the top of the code for clarity and consistency.
Q & A
What is a variable in Python?
-A variable in Python is a memory location or a named address that holds a value, which can be changed during program execution. Different types of values like booleans, strings, and integers can be assigned to variables.
How can you identify the type of a variable in Python?
-You can identify the type of a variable in Python using the `type()` function. For example, `type(customer)` would return the type of the variable 'customer', such as 'str' for string.
Can you assign a string to a variable in Python using both single and double quotes?
-Yes, you can assign a string to a variable in Python using either single quotes or double quotes. For example, both `customer = 'Reval'` and `customer = "Reval"` are valid assignments.
What is the difference between an integer and a float in Python?
-An integer in Python is a whole number, while a float is a number that includes a decimal point. For example, `250` is an integer, while `10.99` is a float.
What are booleans in Python and how are they used?
-Booleans in Python represent two possible values: `True` or `False`. They are often used in conditional statements to determine the flow of the program. It is case-sensitive, meaning 'True' and 'False' must be capitalized.
Why is consistent naming of variables important in programming?
-Consistent naming of variables is important because it helps programmers understand and maintain the code more easily, especially when working on large projects. Clear and consistent variable names make the code more readable and reduce confusion.
What is camel case and why is it used in Python variable naming?
-Camel case is a variable naming convention where the first word starts with a lowercase letter, and subsequent words start with uppercase letters. It is used to improve readability, especially when dealing with multi-word variable names like 'customerFirstName'.
Can you use numbers in Python variable names?
-Yes, you can use numbers in variable names in Python, but you cannot start a variable name with a number. For example, `customer1` is valid, but `1customer` would result in an error.
What are constants in Python, and how are they different from variables?
-In Python, constants are values that do not change throughout the program, like the cost of a phone or charger. Although Python doesn't support constants explicitly, developers use uppercase letters to signify constants (e.g., `PHONE_COST = 250`).
What is the purpose of using uppercase for constants in Python?
-Uppercase letters are used to identify constants in Python, helping to distinguish them from variables. This is a convention used by Python developers, even though Python does not have a specific constant data type.
Outlines
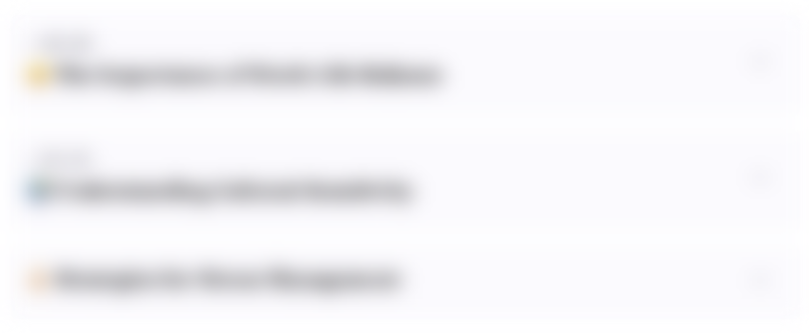
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
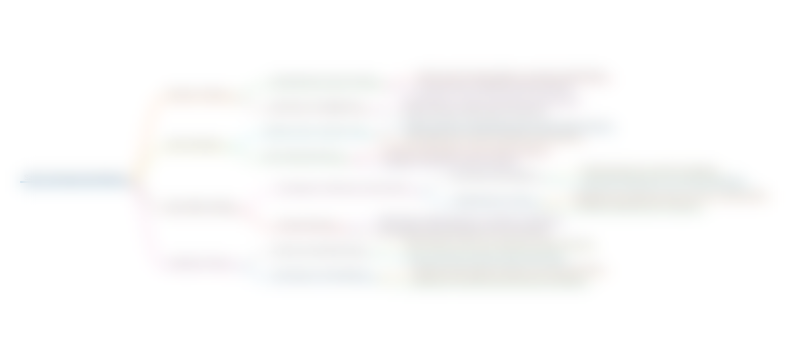
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
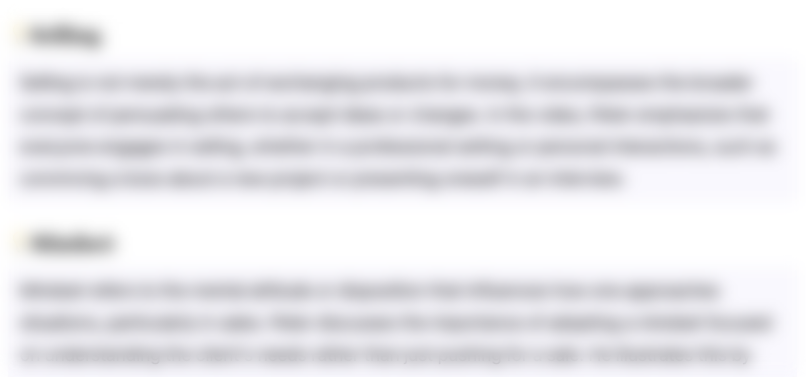
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
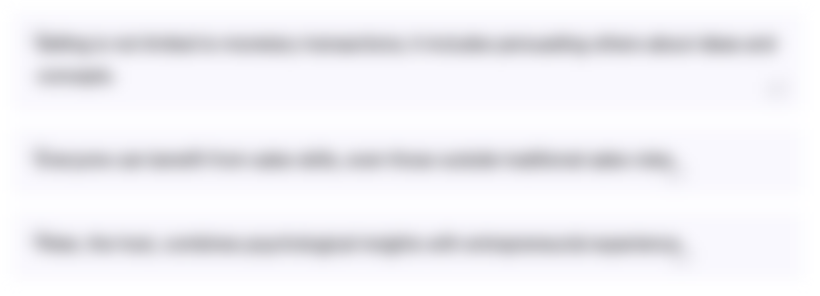
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
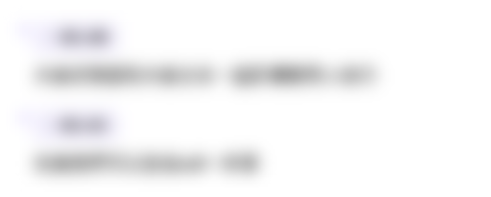
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
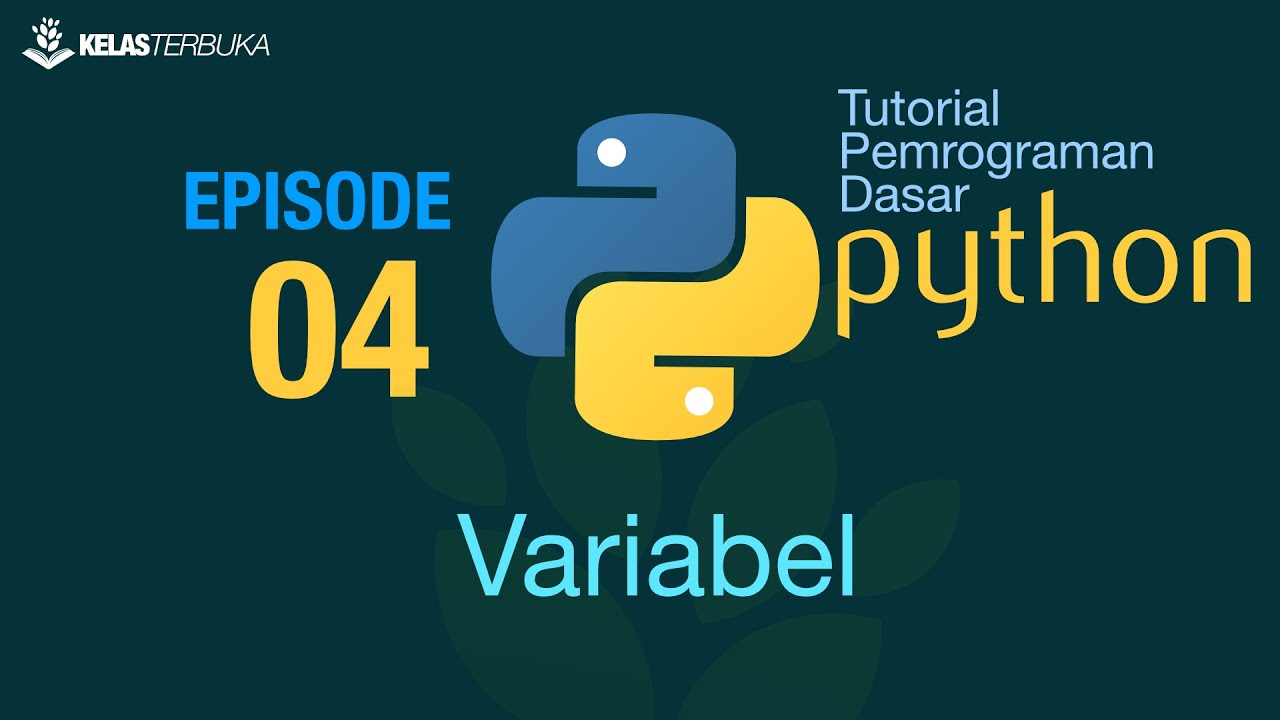
Belajar Python [Dasar] - 04 - Mengenal Variabel
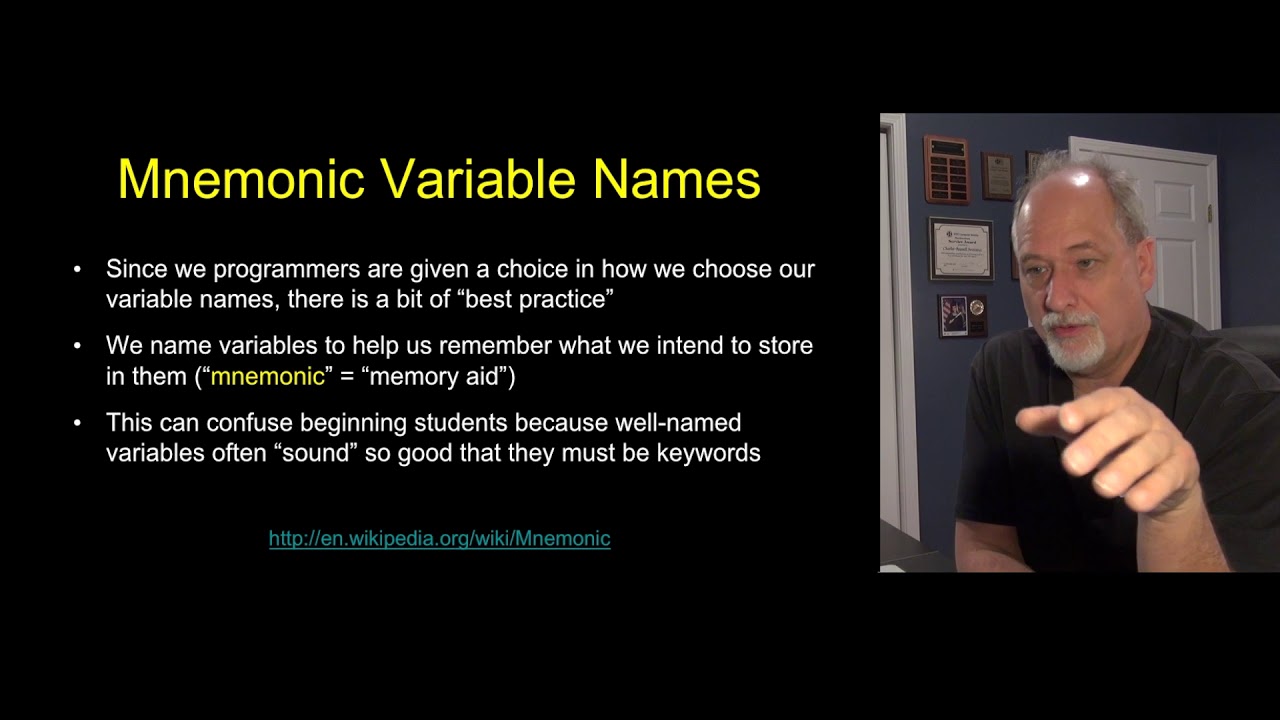
02 - Expressions A - Python for Everybody Course
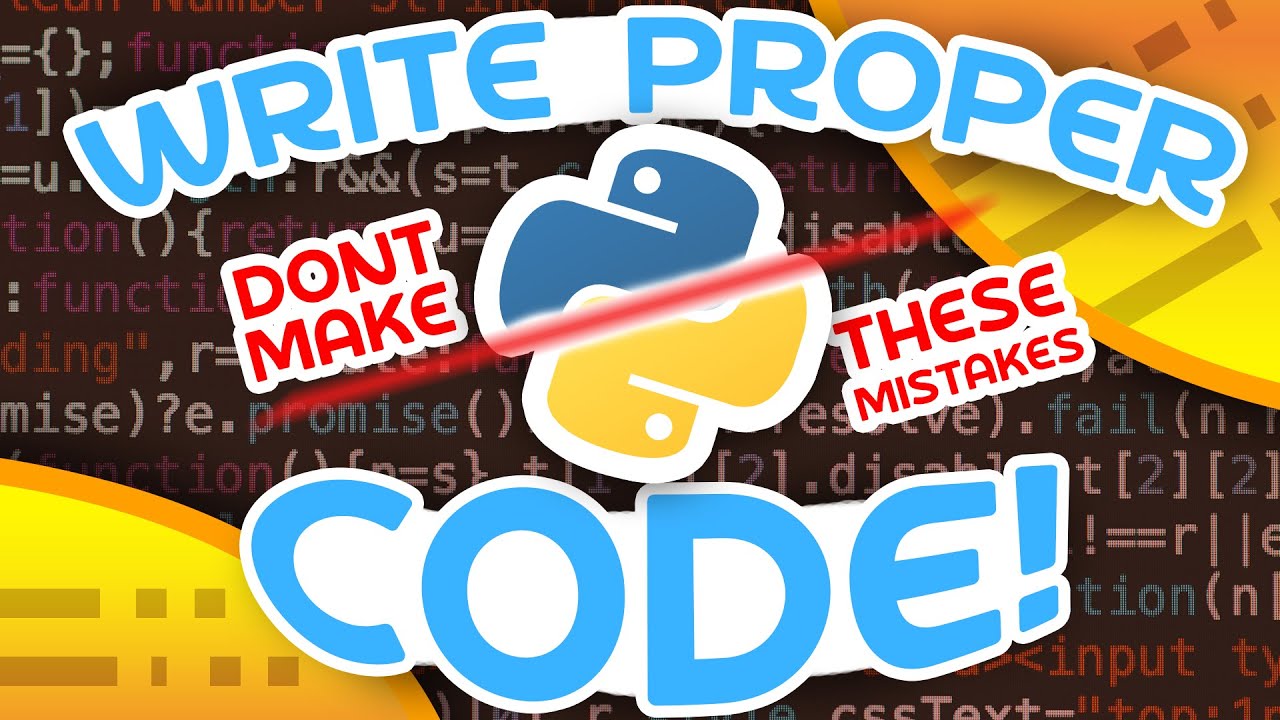
Write Python Code Properly!
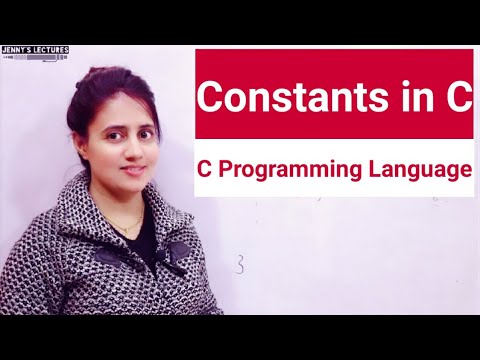
C_07 Constants in C | Types of Constants | Programming in C
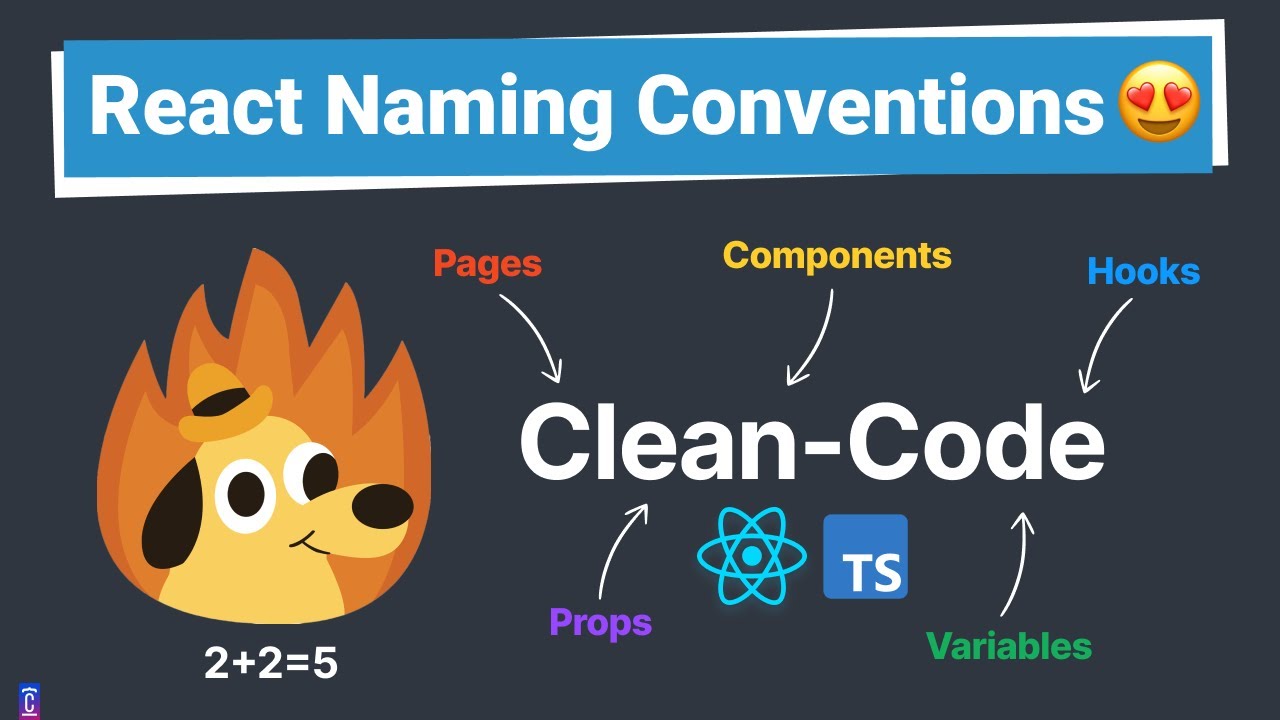
React Naming Conventions You should follow as a Junior Developer - clean-code

Variables & Data Types In C: C Tutorial In Hindi #6
5.0 / 5 (0 votes)