Python Variables and Data Types
Summary
TLDRThis Python tutorial covers the basics of variables, data types, and their applications. It introduces variables as essential building blocks, explaining their components: symbolic names, assignment operators, and values. The lesson delves into numeric data types, including integers and floats, with practical examples like calculating the probability of COVID-19 infection in British Columbia. It then explores strings, demonstrating how to manipulate and concatenate them using various methods. The tutorial also touches on boolean values and their role in conditional statements, setting the stage for future discussions on if statements and loops. The video promises more on advanced data types and variable naming conventions in the next installment.
Takeaways
- ๐ Variables in Python are fundamental building blocks used to store data for easy access in code.
- ๐ข Variables can hold different data types, including integers, floats, strings, and booleans.
- ๐ Python automatically converts integers to floats when performing division, resulting in decimal values.
- ๐ Strings are sequences of characters enclosed in quotation marks and can include letters, numbers, and symbols.
- ๐ The `type()` function can be used to check the data type of a variable in Python.
- ๐งฎ Basic mathematical operations like addition, subtraction, multiplication, division, and exponentiation can be performed on numeric data types.
- ๐ String concatenation can be done using the `+` operator or within print statements using commas.
- ๐ The `format` method offers a more organized way to create formatted strings with variables.
- ๐ Strings can be repeated using the multiplication operator (`*`) to repeat a string a specified number of times.
- ๐ Boolean values (`True` or `False`) are used in conditional statements and are integral to control flow in Python.
- ๐ Advanced data types, which can contain more than one value, will be covered in later lessons.
Q & A
What are the three elements that make up a variable in Python?
-A variable in Python consists of a symbolic name, an assignment operator (usually the equality sign), and the value that is being stored.
How many basic data types are there in Python?
-There are eight basic data types in Python.
What is an integer in Python and what does it represent?
-An integer in Python represents whole numbers, which can be positive or negative.
How do you represent floating-point numbers in Python?
-Floating-point numbers, or floats, in Python represent decimals and can also be positive or negative.
What is the purpose of the 'type' function in Python?
-The 'type' function in Python is used to check the data type of a variable when you are unsure of it.
What happens to the data type when you divide two integers in Python?
-When you divide two integers in Python, the result is a float, even if the division results in a whole number.
What is a string in Python?
-A string in Python is a set of characters enclosed in quotation marks, which can include letters, numbers, punctuation, and spaces.
How can you concatenate strings in Python?
-You can concatenate strings in Python using the plus operator, the comma operator within print statements, or the format method.
What are the two arithmetic operations that can be performed on strings?
-The two arithmetic operations that can be performed on strings are concatenation using the plus operator and multiplication using the asterisk operator.
What is a boolean data type in Python and what values can it take?
-A boolean data type in Python can only take two values: 'True' or 'False', represented with a capital 'T' and 'F' respectively.
How do you check for equality in Python?
-To check for equality in Python, you use the double equality sign (==) instead of the single equality sign, which is used for assignment.
Outlines
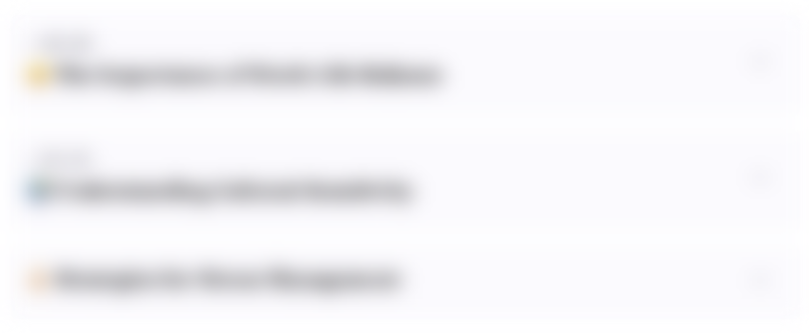
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
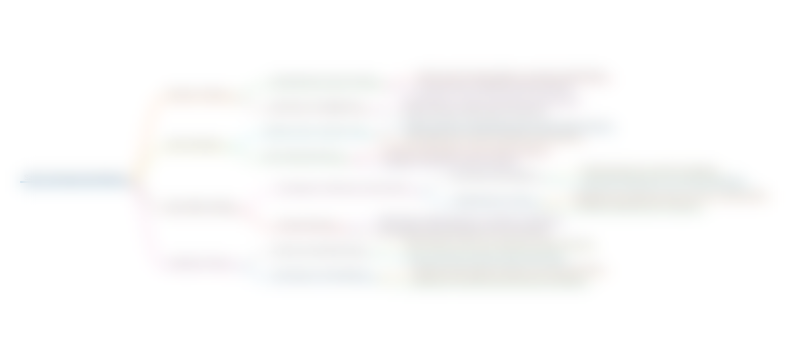
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
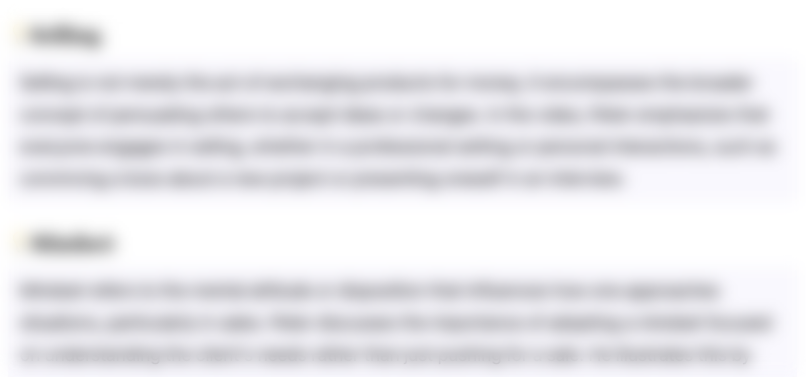
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
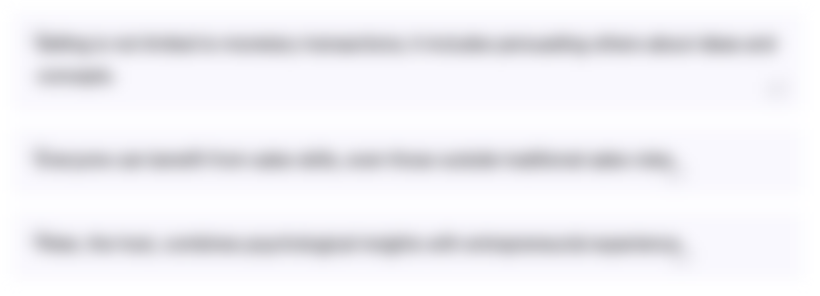
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
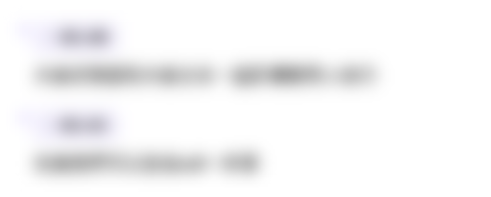
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
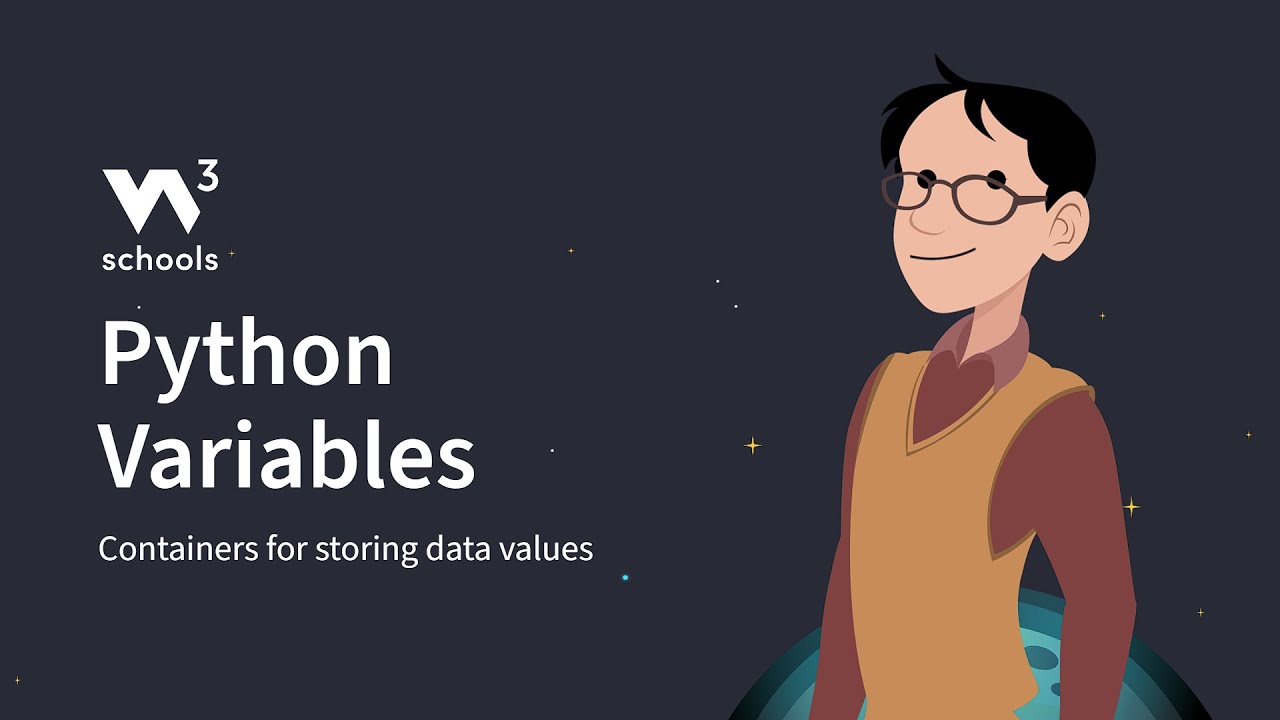
Python - Variables - W3Schools.com
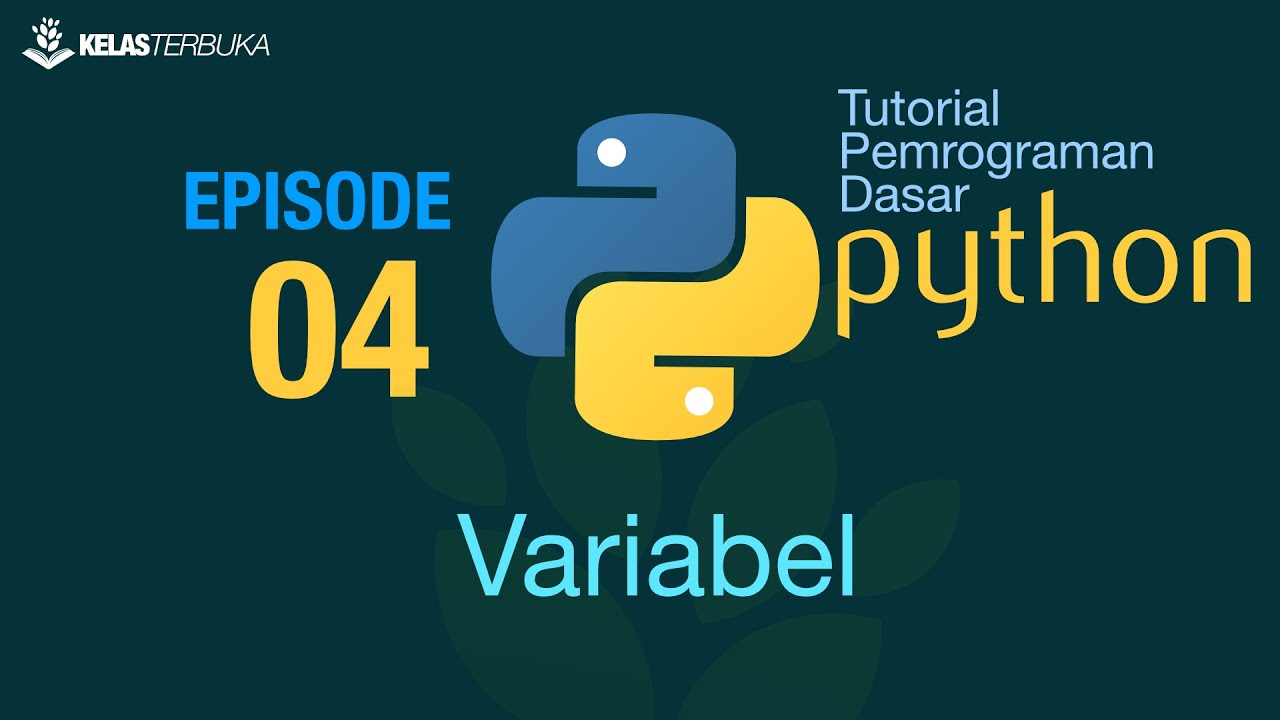
Belajar Python [Dasar] - 04 - Mengenal Variabel
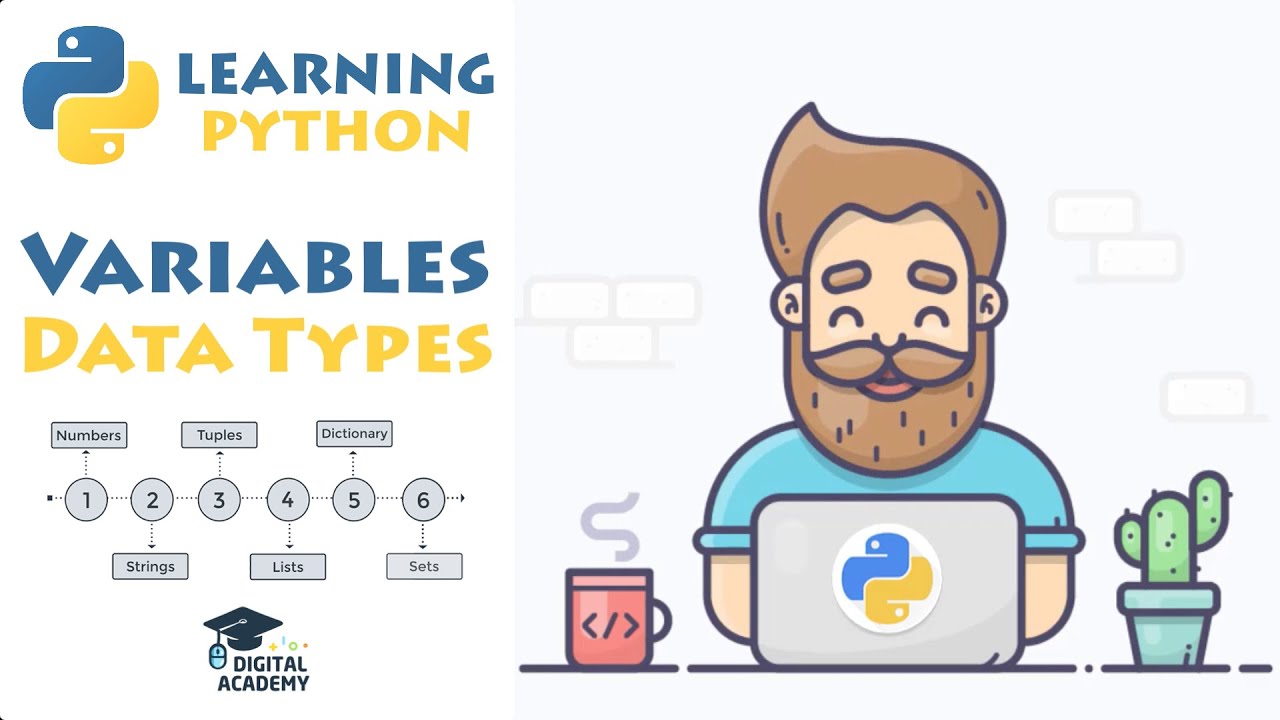
DATA TYPES in Python (Numbers, Strings, Lists, Dictionary, Tuples, Sets) - Python for Beginners
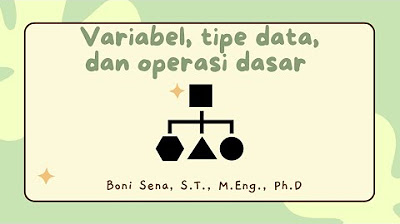
Variabel, tipe data dan operasi dasar
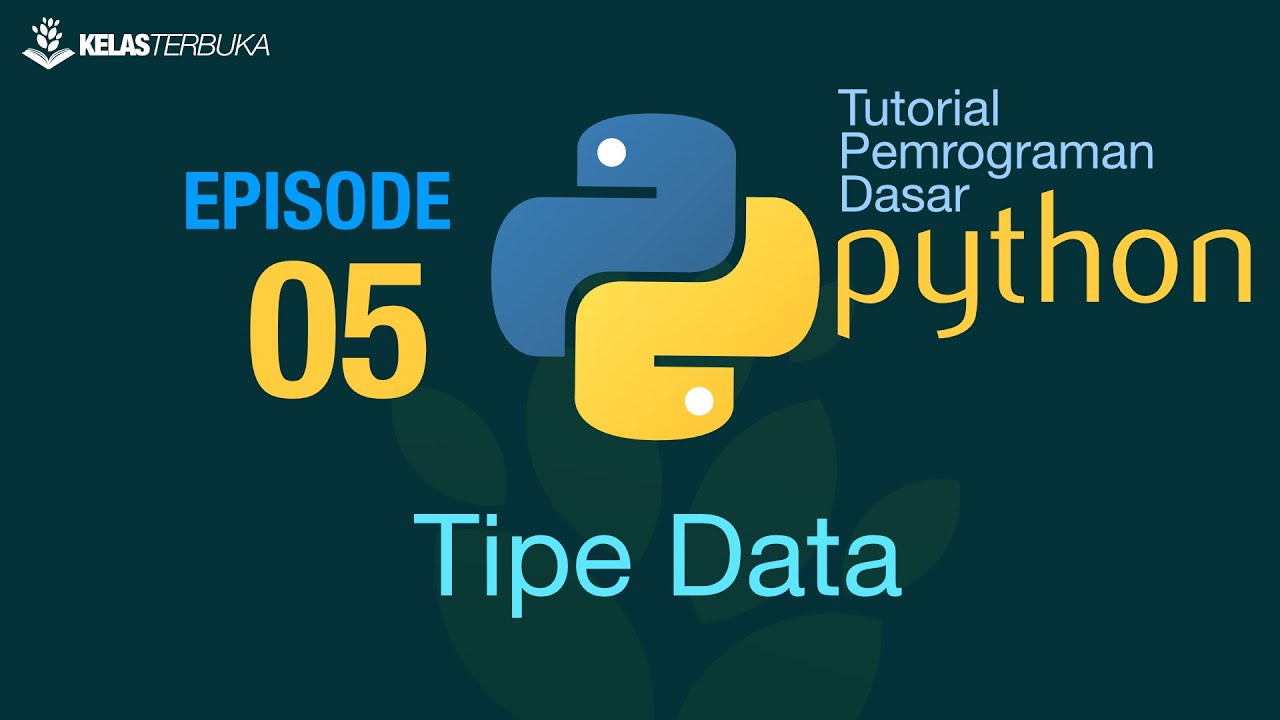
Belajar Python [Dasar] - 05 - Tipe Data
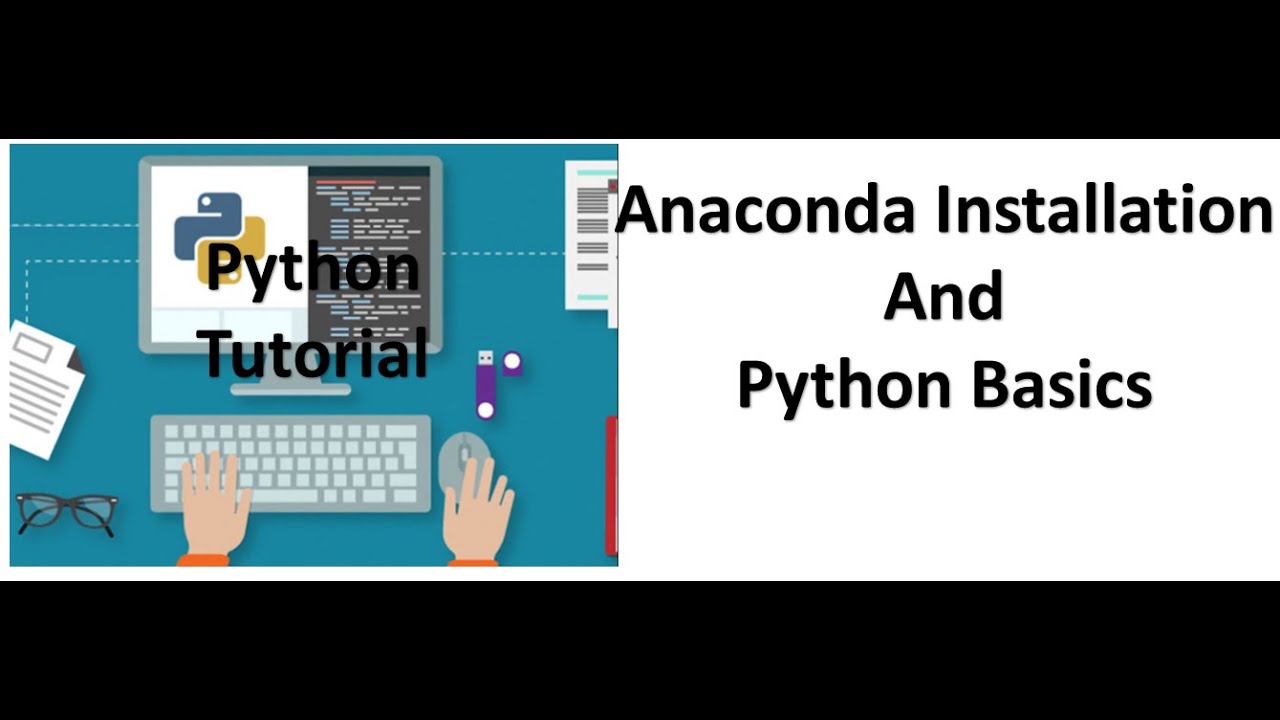
Tutorial 1- Anaconda Installation and Python Basics
5.0 / 5 (0 votes)