C++ programming, Void function, get input by reference, call function by value and by reference
Summary
TLDRIn this instructional video, Professor Liu introduces the concept of void functions in C++ programming. Void functions do not return a value but can modify variables by passing them by reference, making them useful for handling multiple inputs. The lesson covers key programming concepts, including function prototypes, reference passing, and separating logic into functions for cleaner, more modular code. Using a practical example of calculating the area and perimeter of a rectangle, the video emphasizes how to structure programs efficiently, implement loops for repeated tasks, and organize input/output tasks within void functions, enhancing code clarity and maintainability.
Takeaways
- 😀 Void functions do not return a value; they are used for actions that don't require returning data to the calling function.
- 😀 The header of a void function starts with the keyword 'void', and the function body does not include the 'return' keyword.
- 😀 To call a void function, you simply use the function's name along with the necessary arguments, if applicable.
- 😀 Void functions are particularly useful when you need to modify variables directly via passing by reference, allowing changes to be reflected outside the function.
- 😀 Passing variables by reference (using &) enables a function to modify the original variable's value, unlike passing by value which only modifies the local copy.
- 😀 Void functions can pass multiple values back by reference, while value-returning functions can only return one value.
- 😀 In C++, functions that need multiple outputs should use void functions with parameters passed by reference to avoid creating many separate return functions.
- 😀 When writing a program with void functions, it is essential to keep the main function as a dispatcher, calling other functions without containing direct logic.
- 😀 A typical example of void function usage includes getting input from users and calculating results based on that input, such as calculating the area and perimeter of a rectangle.
- 😀 The example program uses a void function to get input (length and width) and calculate the area and perimeter, with values passed by reference to update the original variables.
- 😀 The main function should be focused on organizing and calling functions, and not on performing actual computations, making the code easier to maintain and reuse.
Q & A
What is a void function in C++?
-A void function in C++ is a function that does not return any value. The return type of the function is specified as 'void'. It is used when the function’s purpose is to perform an action rather than compute and return a value.
How does a void function pass information to the calling function?
-A void function can pass information to the calling function by reference using reference parameters (denoted by '&' or 'n%' in C++). This allows the void function to modify the values of variables in the caller's scope directly.
Why is passing by reference preferred in some cases over passing by value in void functions?
-Passing by reference is preferred when multiple values need to be returned or modified by the function. Unlike passing by value, where only one value can be returned, passing by reference allows a function to modify several variables directly, which is more efficient and avoids the need for multiple return statements.
What is the advantage of using a void function to read input values?
-The advantage of using a void function to read input is that it can modify the caller’s variables directly by passing them by reference. This allows the function to gather multiple inputs without returning values, making the code cleaner and more efficient.
What is meant by 'aliasing' in the context of void functions?
-Aliasing refers to the concept where two variables share the same memory address, effectively becoming 'twins' of each other. When a reference is passed in a void function, the variable in the caller’s scope and the parameter in the function share the same memory address, so any changes made to one reflect on the other.
In the example given, why is the 'n%' symbol used when passing parameters to a void function?
-'n%' (or '&' symbol) is used to pass parameters by reference, meaning that the actual memory addresses of the variables are passed to the function. This allows the function to modify the original variables in the caller’s scope, rather than working with copies.
How can void functions be used to calculate area and perimeter in the rectangle example?
-In the rectangle example, a void function is used to get input from the user for the rectangle’s length and width. Then, another void function calculates the area and perimeter and passes the results back to the main function using reference parameters. The main function then displays the results.
What does the concept of 'function prototypes' refer to in C++?
-A function prototype in C++ is a declaration of the function that specifies its return type, name, and parameters but does not contain the function's implementation. It is used to inform the compiler about the function’s signature before it is defined in the code, allowing for proper function calls.
What role does the 'main' function play in a well-structured C++ program with void functions?
-In a well-structured C++ program, the 'main' function acts as a dispatcher. It should contain minimal logic, typically just variable declarations and function calls. The bulk of the program’s logic, such as calculations and input/output, should be handled by separate functions to promote modularity and clarity.
How does using loops improve the structure of the program demonstrated in the transcript?
-Using loops allows the program to repeatedly execute tasks, such as asking for user input and calculating results, without needing to duplicate code. This makes the program more efficient and scalable, as the loop can handle multiple iterations of the process, as demonstrated in the rectangle example.
Outlines
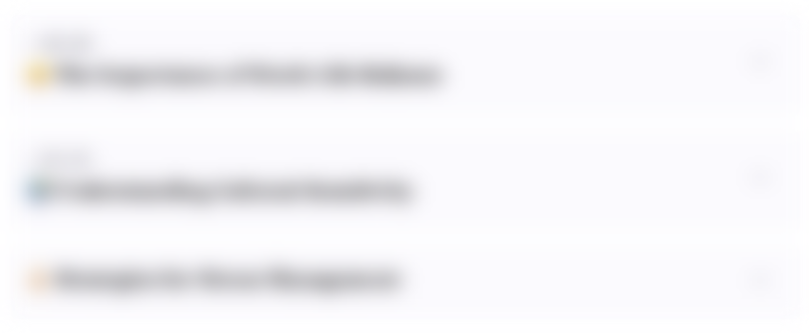
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
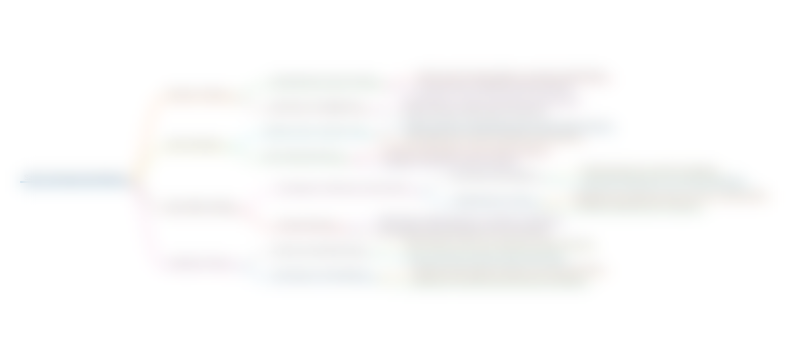
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
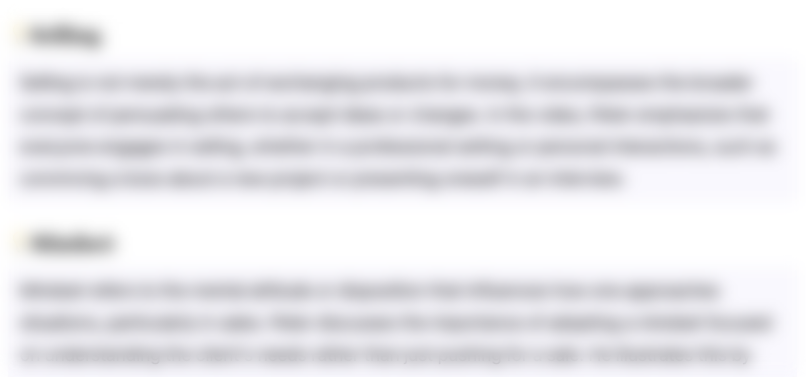
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
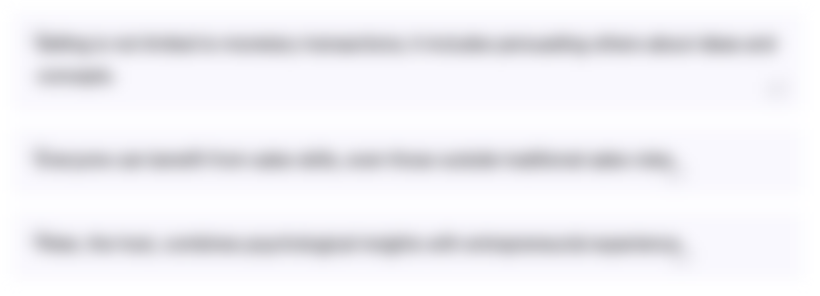
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
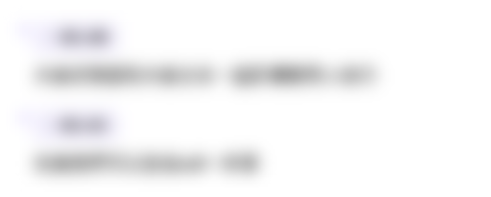
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频
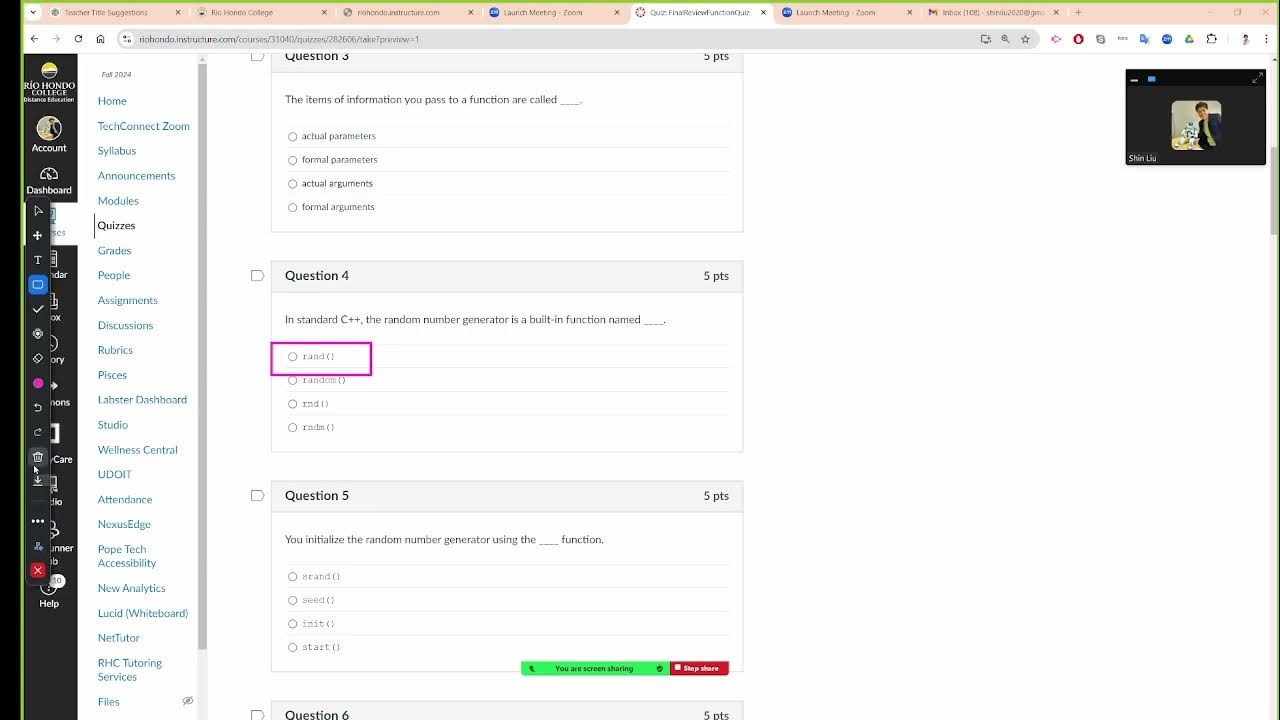
C++ Final Review Function Quiz 10 questions
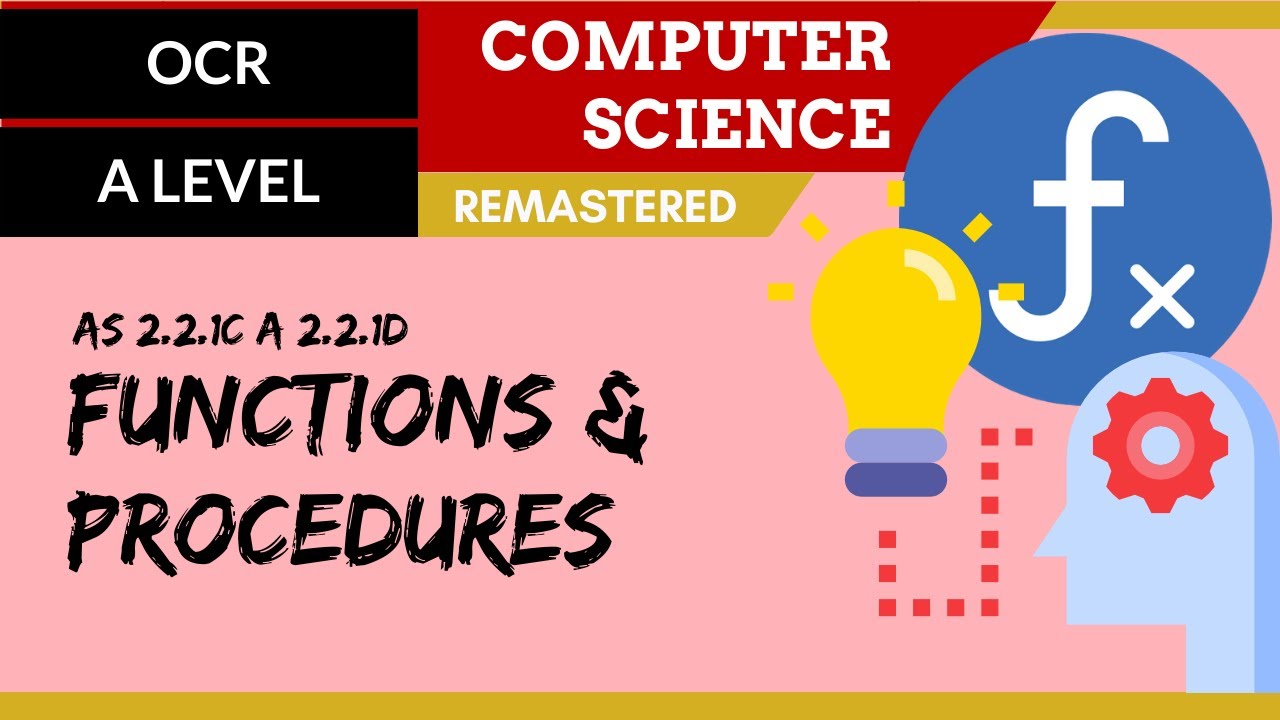
136. OCR A Level (H046-H446) SLR23 - 2.2 Functions & procedures
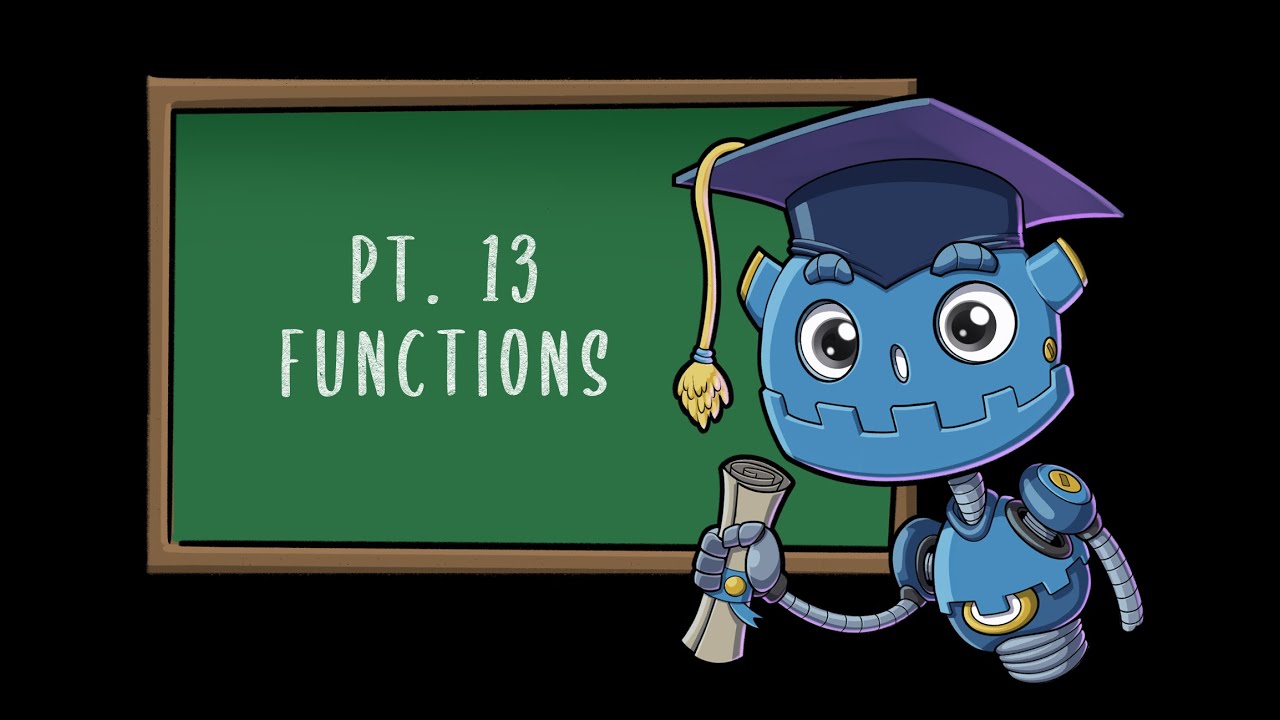
Functions | Godot GDScript Tutorial | Ep 13
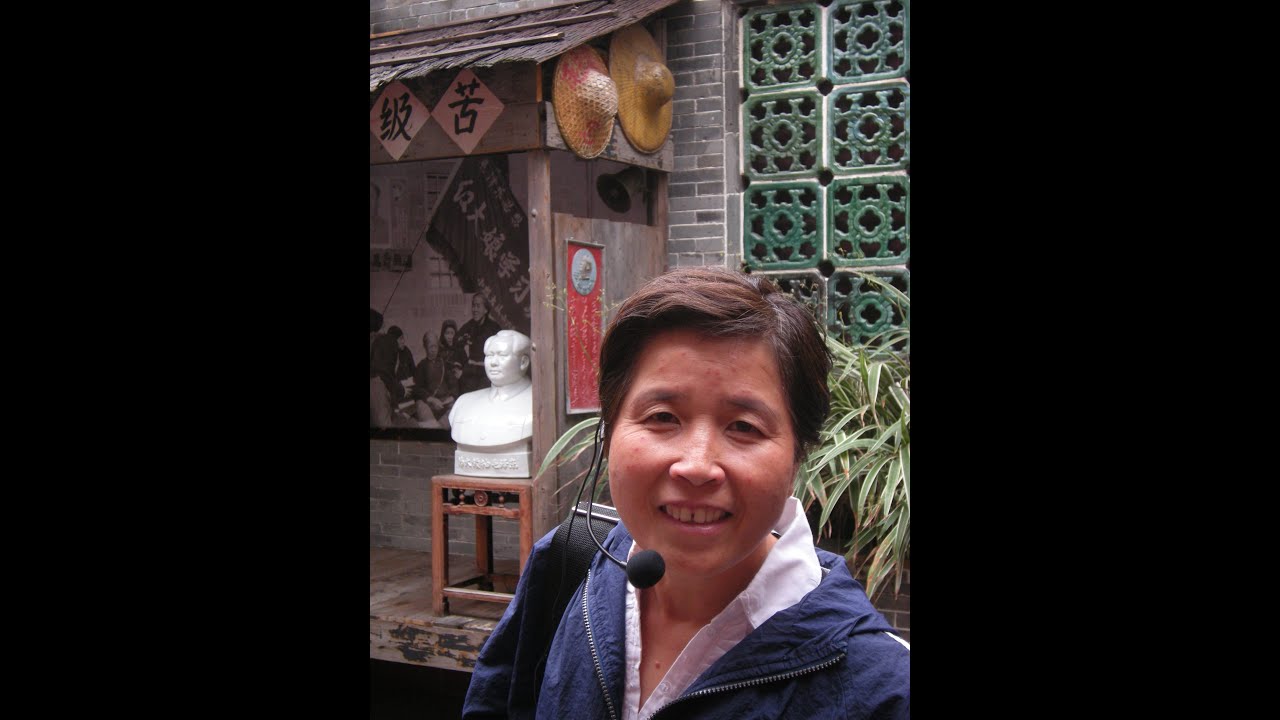
C++ programming to calculate area and perimeter, with functions.
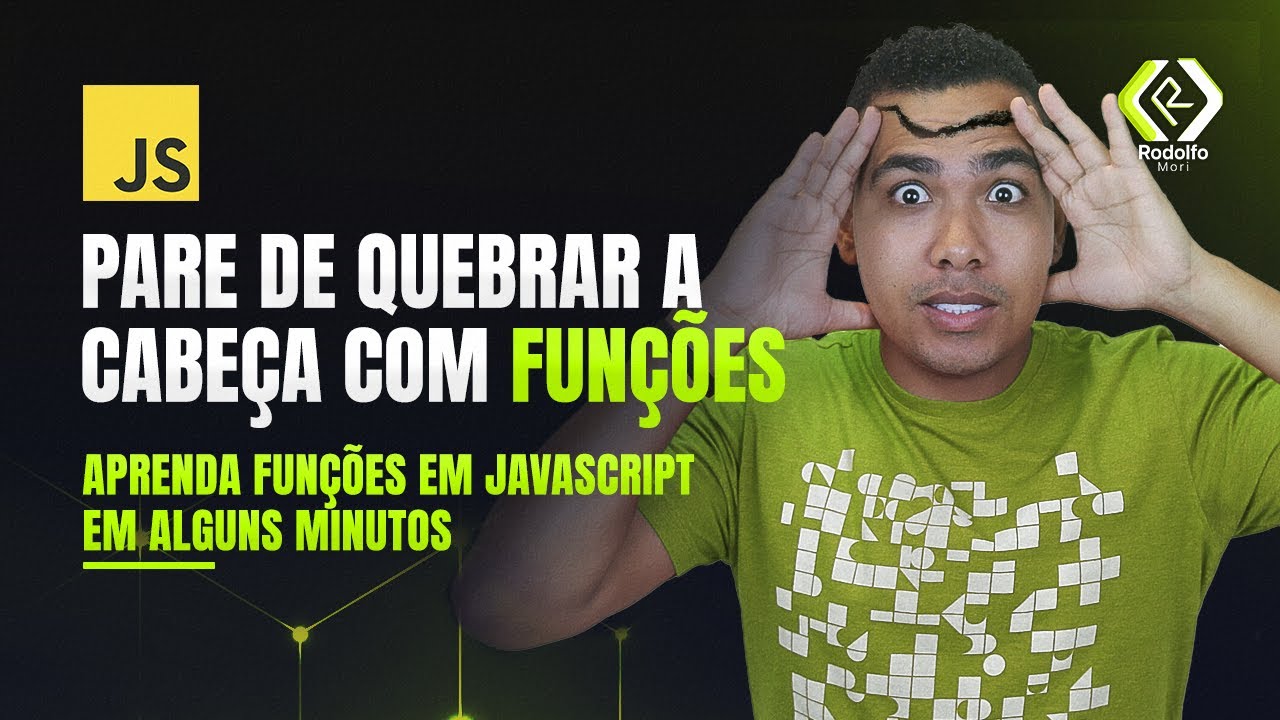
🔴APRENDA FUNÇÕES EM JAVASCRIPT EM ALGUNS MINUTOS
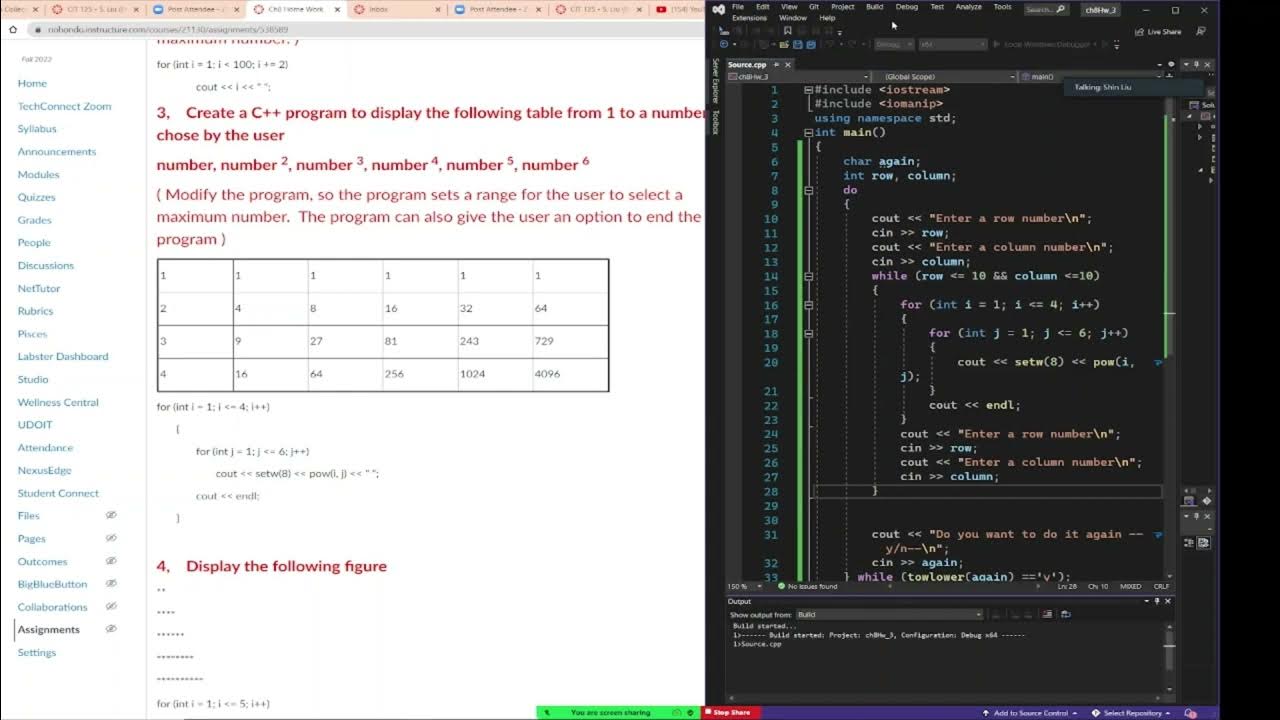
C++ programming, for loop to create a exponential table, do while loop to continue
5.0 / 5 (0 votes)