Object-oriented Programming in 7 minutes | Mosh
Summary
TLDRThis video script delves into the four fundamental concepts of object-oriented programming (OOP): encapsulation, abstraction, inheritance, and polymorphism. It contrasts OOP with procedural programming, illustrating how OOP organizes related variables and functions into objects, reducing complexity and interdependence. The script uses relatable examples, such as a car and a DVD player, to explain these concepts, emphasizing the benefits of OOP in code reusability, simplicity, and minimizing the impact of changes. It concludes by highlighting the advantages of OOP in creating more manageable and scalable code.
Takeaways
- 📚 Object-oriented programming (OOP) introduces four core concepts: encapsulation, abstraction, inheritance, and polymorphism to address issues with procedural programming.
- 🔍 Encapsulation groups related variables (properties) and functions (methods) into a single unit called an object, simplifying code management and reducing interdependencies.
- 🔑 Abstraction hides the complex internal workings of an object, exposing only necessary operations to the user, which simplifies the interface and minimizes the impact of internal changes.
- 📝 Inheritance allows new objects to inherit properties and methods from existing ones, reducing redundancy and promoting code reusability.
- 🎨 Polymorphism enables objects to take on many forms, allowing the same method to behave differently across different object types without the need for complex conditional statements.
- 🚗 The script uses a car as an example of an object with properties like make, model, and color, and methods like start, stop, and move to illustrate encapsulation.
- 💾 The local storage object in web browsers is given as a real-world example of an object with properties like length and methods like setItem and removeItem.
- 🛠 Procedural programming is criticized for leading to 'spaghetti code' due to its interdependent functions and variables, making it difficult to maintain and modify.
- 🔄 The transition from procedural to OOP is exemplified by moving from a function with multiple parameters to an object with properties and a method, reducing parameter count and complexity.
- 🔍🔑 Abstraction is further explained through the DVD player analogy, where users interact with simple controls without needing to understand the complex internal components.
- 📈 The benefits of OOP include reduced complexity, easier maintenance, and the ability to isolate changes, making software development more efficient and scalable.
- 🎓 The script concludes with an invitation to learn more about OOP through a linked course, emphasizing continuous education for mastering programming concepts.
Q & A
What are the four core concepts of object-oriented programming?
-The four core concepts of object-oriented programming are encapsulation, abstraction, inheritance, and polymorphism.
Why did object-oriented programming emerge as a solution to the problems of procedural programming?
-Object-oriented programming emerged to address issues like code duplication, interdependence between functions, and the complexity of maintaining large codebases, which were common in procedural programming.
What is encapsulation in the context of object-oriented programming?
-Encapsulation is the concept of bundling related variables and functions into a single unit called an object, where variables are referred to as properties and functions as methods.
Can you provide an example of encapsulation from the script?
-An example of encapsulation in the script is the 'car' object, which has properties like 'make', 'model', and 'color', and methods like 'start', 'stop', and 'move'.
What is the purpose of abstraction in object-oriented programming?
-Abstraction in object-oriented programming is used to hide the complex internal workings of an object from the outside world, simplifying the interface and reducing the impact of changes on the code.
How does the script illustrate the concept of abstraction?
-The script uses the example of a DVD player, where the user interacts with simple buttons without needing to understand the complex logic board inside, to illustrate the concept of abstraction.
What is inheritance in object-oriented programming, and how does it help in reducing redundancy?
-Inheritance is a mechanism that allows new objects to inherit properties and methods from existing objects, eliminating the need to redefine common attributes and behaviors, thus reducing redundancy in code.
Can you explain polymorphism using the script's HTML elements example?
-Polymorphism allows different objects to respond to the same method call in different ways. In the script's example, all HTML elements have a 'render' method, but each element's 'render' method behaves differently based on the object's type.
How does object-oriented programming simplify the process of code maintenance and reuse?
-Object-oriented programming simplifies code maintenance and reuse by encapsulating related variables and functions into objects, abstracting complex details, inheriting common behaviors, and using polymorphism to handle different object types with the same method calls.
What is the benefit of having fewer parameters in a method according to the script?
-Having fewer parameters in a method simplifies the function's usage and makes it easier to maintain, as stated by Uncle Bob in the script.
What does the script suggest about the relationship between object-oriented programming and the reduction of code complexity?
-The script suggests that object-oriented programming reduces code complexity by grouping related variables and functions, hiding details through abstraction, eliminating redundancy via inheritance, and simplifying conditional logic with polymorphism.
Outlines
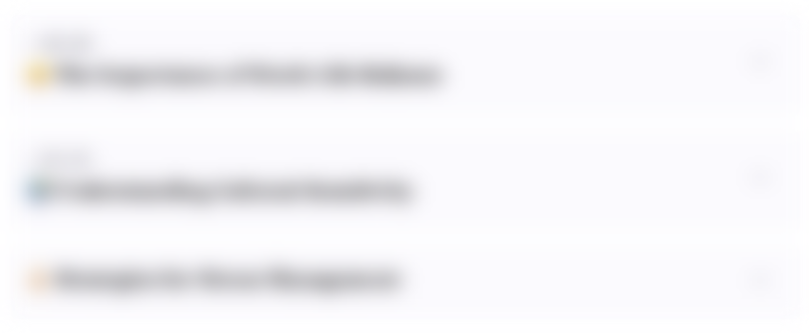
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
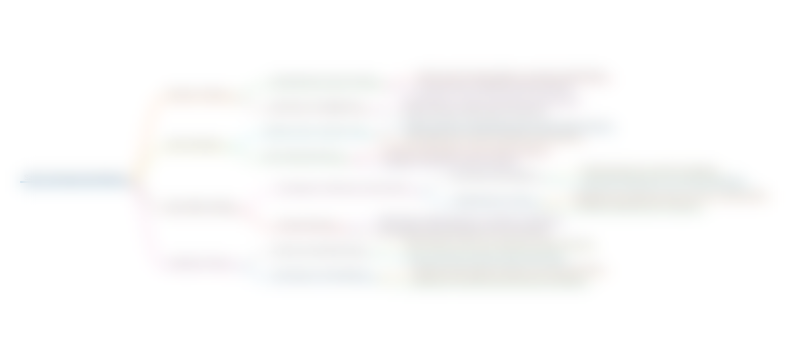
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
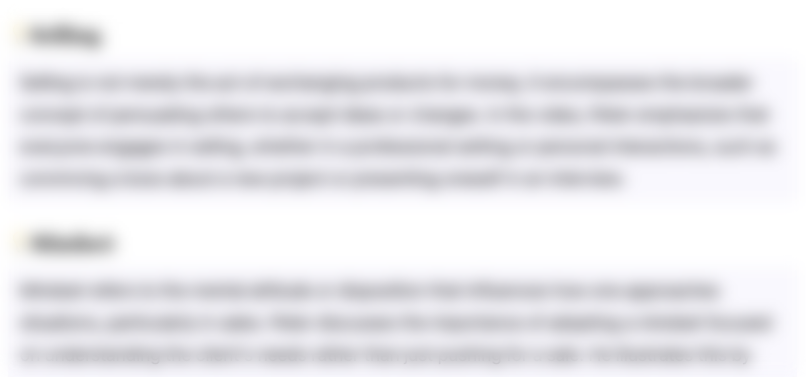
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
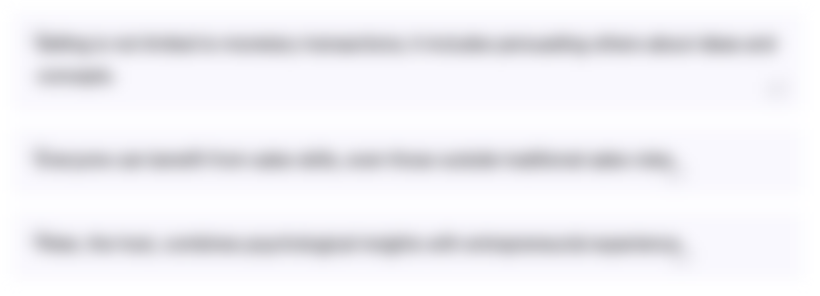
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
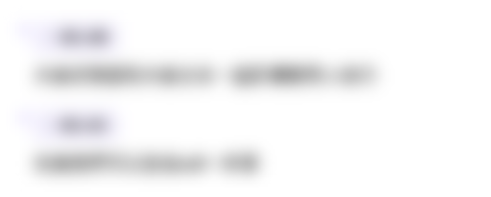
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
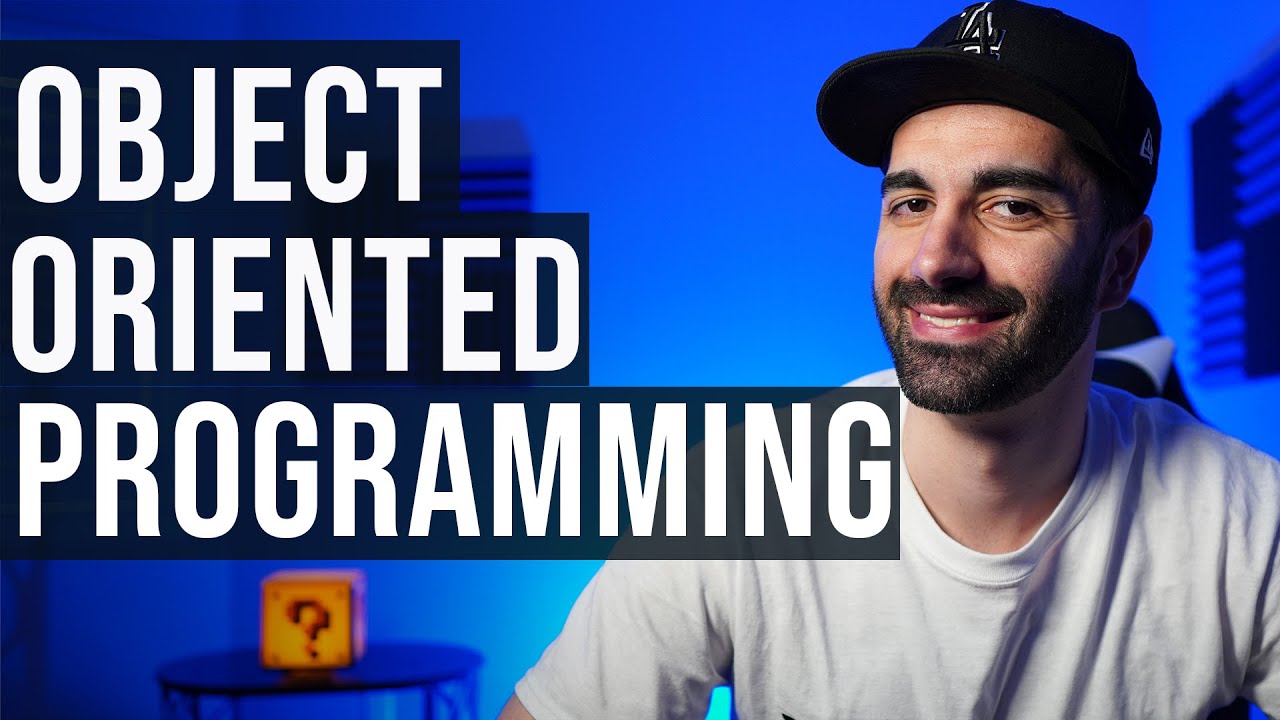
Object Oriented Programming - The Four Pillars of OOP
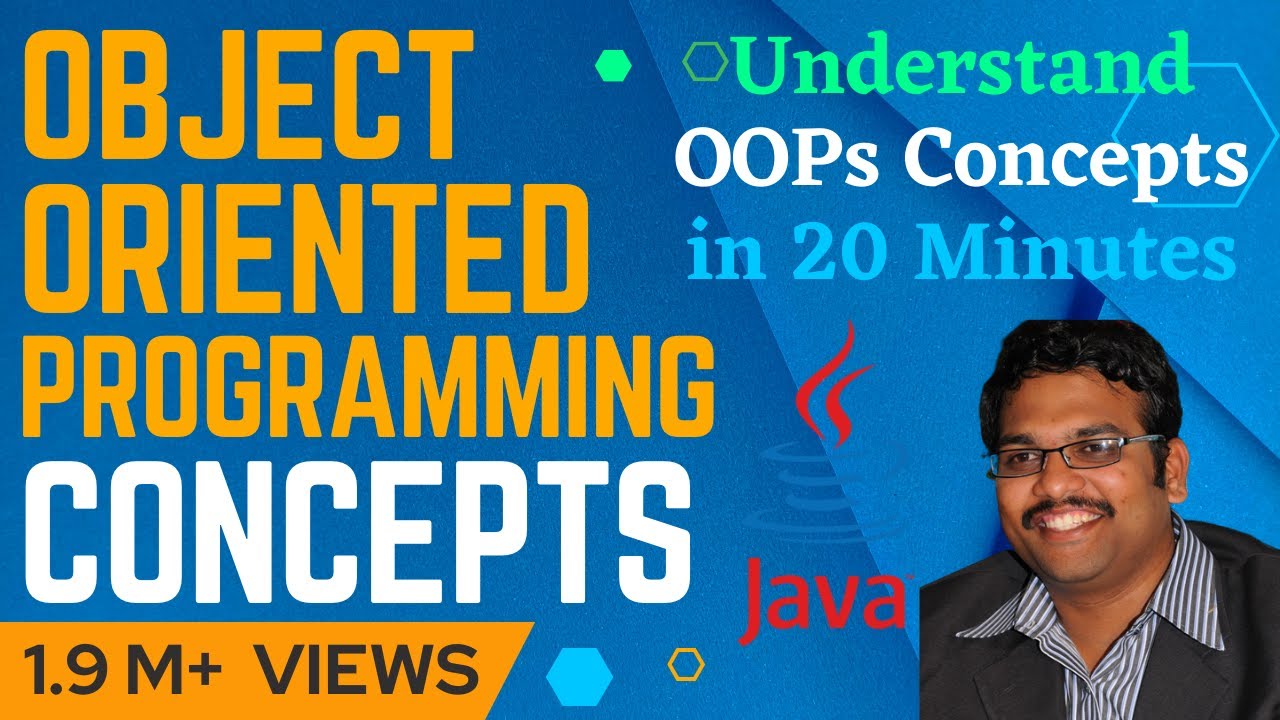
OOPS CONCEPTS - JAVA PROGRAMMING

Fundamental Concepts of Object Oriented Programming
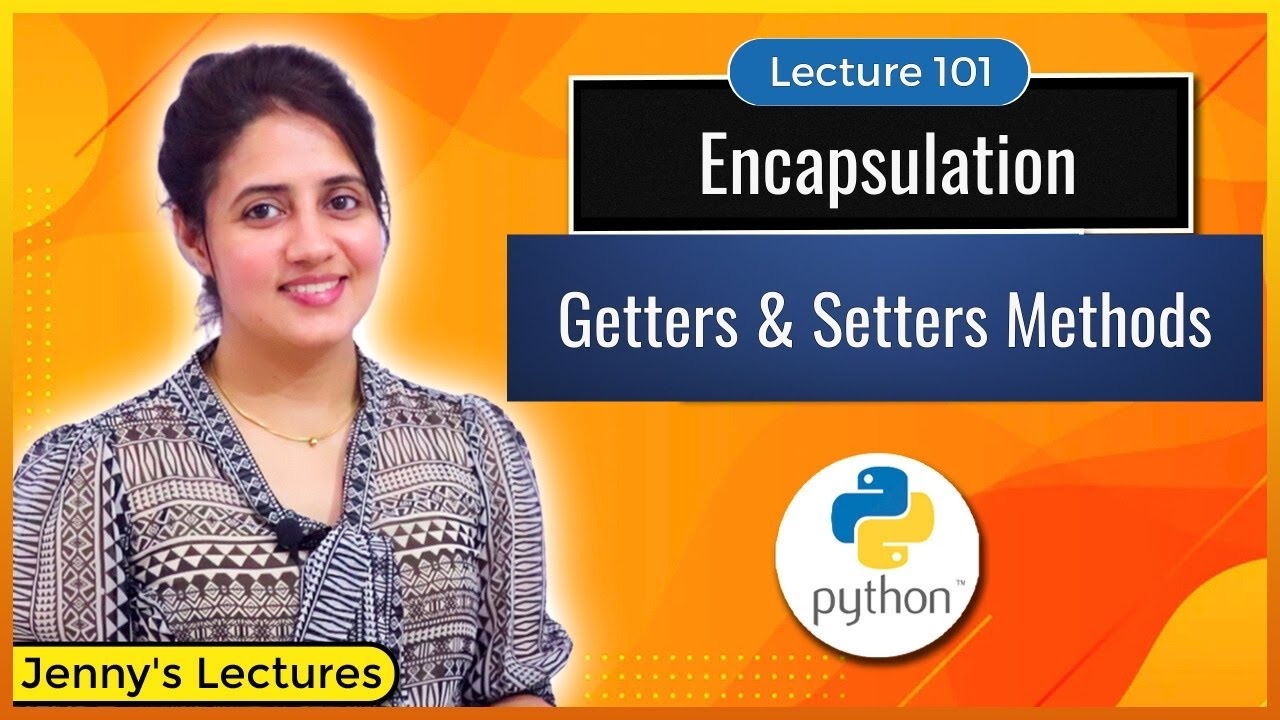
Encapsulation in Python | Getters & Setters methods | Python Tutorials for Beginners #lec101

Overview of Object-oriented programming in C# | C# object-oriented programming | C# oops

Object Oriented Programming Using Python [With Examples] - Part 2 | The Knowledge Academy
5.0 / 5 (0 votes)