caesar - CS50 Walkthroughs 2019
Summary
TLDRThe script outlines a Caesar cipher program that encrypts text by shifting letters using a provided key. It explains the process of taking command-line arguments for the key, ensuring its validity, and converting it to an integer. The program prompts for plain text, then shifts each alphabetic character by the key while preserving case and non-alphabetic characters. ASCII values and modulo operations are utilized to achieve the wrap-around effect for the alphabet. The script provides a step-by-step guide on implementing the cipher in C, including handling strings, character checks, and encryption logic.
Takeaways
- 🔑 The task is to create a program that encrypts text by shifting each letter by a specified key.
- 🖥️ The program will prompt the user for a key, receive plaintext, and then produce the corresponding ciphertext.
- 🔠 The encryption shifts letters forward in the alphabet by the key value, wrapping around if necessary (e.g., 'Z' shifted by 2 becomes 'B').
- 🔄 The program must preserve the case of each letter and leave non-alphabetic characters unchanged.
- 🧮 The key is provided as a command-line argument, which must be validated to ensure it is numeric and that only one argument is given.
- 🔍 If the user inputs invalid arguments, the program should display a usage message guiding the correct input format.
- 🔡 Alphabetic characters are identified using functions like isalpha, isupper, and islower, and are then shifted based on their ASCII values.
- 🔁 The formula to wrap around the alphabet after shifting involves using the modulo operation (mod 26) to ensure continuity.
- 📏 The program calculates the alphabetical index for each character, applies the shift, and then converts it back to the correct case.
- 🚀 The final step involves processing the entire plaintext string character by character, applying the shift, and then printing the resulting ciphertext.
Q & A
What is the main task of the Caesar program described in the script?
-The main task of the Caesar program is to encipher or encrypt text by shifting all the letters in the text by a certain key amount, creating a ciphertext.
How does the Caesar cipher work with the alphabet?
-The Caesar cipher works by shifting each letter in the plaintext by a fixed number of positions (the key) in the alphabet. If the shift goes beyond 'Z', it wraps around to the beginning of the alphabet.
What is the significance of preserving case when using the Caesar cipher?
-Preserving case is important because it ensures that uppercase letters remain uppercase and lowercase letters remain lowercase in the ciphertext, maintaining the original style of the plaintext.
How does the script handle non-alphabetic characters in the plaintext?
-Non-alphabetic characters such as spaces, numbers, or punctuation marks are not changed during the encryption process; they remain the same in the ciphertext.
What is the purpose of the command line argument in the Caesar program?
-The command line argument is used to provide the key for the Caesar cipher, which determines the number of positions each letter in the plaintext will be shifted.
How can the program ensure that only a single, numeric command line argument is provided?
-The program checks the number of arguments (argc) and verifies that the argument contains only digit characters. It uses functions like 'isalpha' to check for non-numeric characters.
What is the role of the 'argv' array in the main function of a C program?
-The 'argv' array holds the command line arguments provided by the user when running the program. Each element of the array represents a separate argument.
How does the script explain the conversion of a string to an integer in C?
-The script suggests using a function like 'atoi' (declared in stdlib.h) to convert a string containing a number into an actual integer value.
What functions are mentioned in the script to check if a character is alphabetic, uppercase, or lowercase?
-The script mentions 'isalpha', 'isupper', and 'islower' as functions to check if a character is alphabetic, uppercase, or lowercase, respectively.
How can the ASCII values of characters be manipulated to implement the Caesar cipher?
-By treating characters as their corresponding ASCII values, you can perform arithmetic operations to shift the characters. Using modulo 26 ensures the wrap-around effect when shifting past 'Z'.
What is the formula used to convert plaintext into ciphertext in the Caesar cipher?
-The formula to convert plaintext into ciphertext is to take the ASCII value of the character, add the key, and then take the result modulo 26, which ensures the wrap-around within the alphabet.
How can you determine the length of the plaintext string in C?
-The 'strlen' function can be used to determine the length of a string in C, which is useful for iterating through all characters in the plaintext for encryption.
Outlines
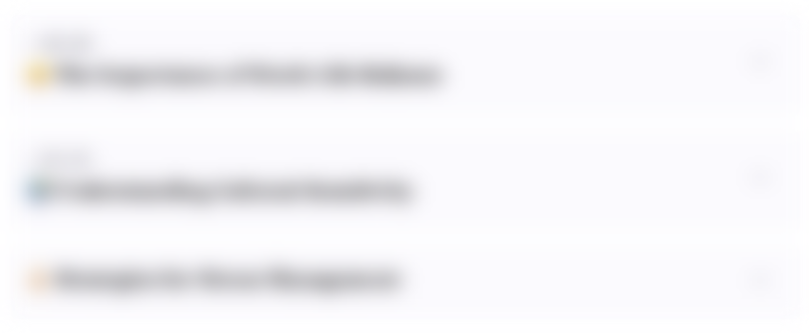
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
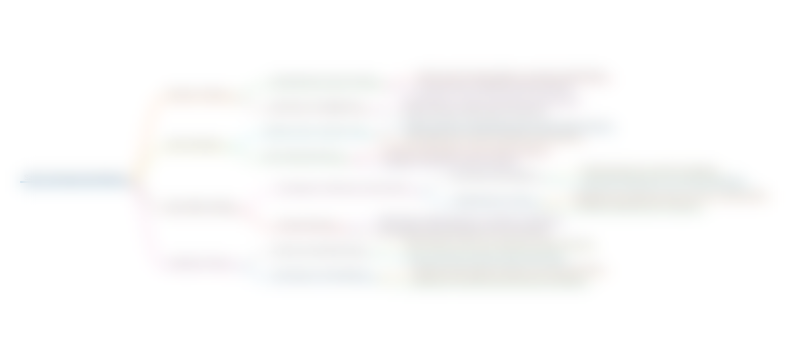
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
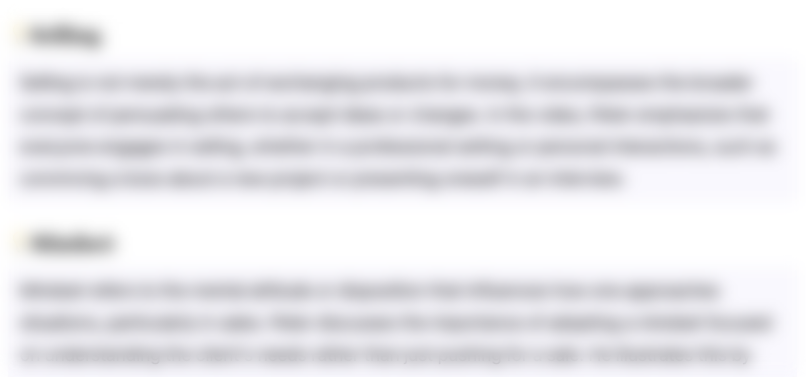
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
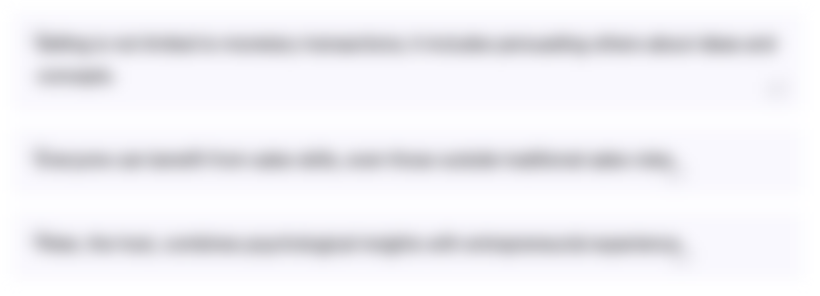
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
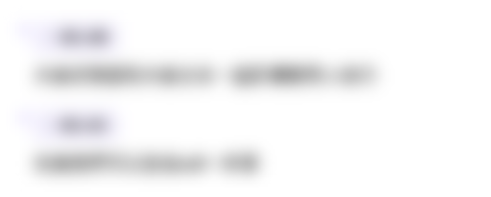
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)