SHA256 and Symmetric Encryption Examples
Summary
TLDRThis video tutorial covers two main questions. The first involves using Python's hash library to find an English word with a specific SHA-256 hash value. It guides viewers to use a provided dictionary file, strip newline characters, and loop through words to match the hash. The second question tackles decrypting cipher text generated by a symmetric encryption algorithm. The video explains the decryption process, which involves XORing each block of the cipher text with the key, and emphasizes the importance of key length for security. Additionally, it suggests an exhaustive search method to find both the key and the encrypted word from a given ciphertext.
Takeaways
- 🔐 **Hashlib Usage**: The video discusses using Python's hashlib library to find an English word with a specific SHA-256 hash digest.
- 📄 **Dictionary Utilization**: A 'dictionary.txt' file is provided, containing a list of meaningful English words to be used for hash matching.
- 🔍 **Word Processing**: The script explains how to process each word in the dictionary by removing newline characters.
- 🔢 **Hash Calculation**: It details the process of calculating the SHA-256 hash of each word and converting it to a hexadecimal digest.
- 🔎 **Target Matching**: The goal is to find a word whose hash matches a given target hash value.
- 🔄 **Decryption Function**: The video explains how to write a Python function to decrypt a cipher text generated by a symmetric encryption algorithm.
- 🔑 **Key Manipulation**: It describes how the encryption algorithm uses an 8-bit key to create a 32-bit extended key by repeating the key four times.
- 🔡 **Block Conversion**: The script mentions converting blocks of text into 32-bit integers for encryption and decryption processes.
- 🔐 **XOR Operation**: The exclusive OR (XOR) operation is central to both the encryption and decryption processes.
- 🔍 **Exhaustive Search**: For the security attack example, the script suggests an exhaustive search through all possible keys (0-255) to find the correct decryption.
Q & A
What is the task described in the first part of the video?
-The task is to use Python's hash library to find an English word whose SHA-256 hash digest matches a given decimal value.
What hint is provided for finding the word in the first task?
-The hint is to use the 'hashlib.sha256()' function in Python to calculate the hash digest of words from a provided dictionary.
How is the dictionary of words accessed according to the video?
-The dictionary is accessed by reading a file named 'dictionary.txt' which contains a list of meaningful English words.
What preprocessing is done to each word read from the dictionary?
-Each word is stripped of newline characters, particularly by removing the last character which is a newline.
What is the formula used to calculate the hash digest of a word?
-The formula used is 'hashlib.sha256(word.encode('ascii')).hexdigest()' which calculates the SHA-256 hash digest of the word in ASCII encoding.
How is the matching word identified in the first task?
-A loop is used to iterate over each word, calculate its hash digest, and compare it to the target hash digest to find a match.
What is the second task discussed in the video?
-The second task is to write a Python function for decrypting a cipher text generated by a specified symmetric encryption algorithm.
How is the encryption algorithm described in the video?
-The algorithm breaks the message into four-character blocks, converts each block to a 32-bit integer, and then XORs it with an extended key to produce the cipher text.
What is the decryption process as described in the video?
-Decryption involves XORing the cipher text with the same key used for encryption to retrieve the original message.
What is the significance of the exclusive OR (XOR) operation in the encryption and decryption process?
-The XOR operation is used because it is reversible; applying it twice with the same key returns the original value, which is essential for both encryption and decryption.
What is the approach to find the key and word if only the cipher text is known?
-An exhaustive search is performed over all possible keys (0 to 255) to decrypt the cipher text and match the result with a list of English words to find the original word and key.
Outlines
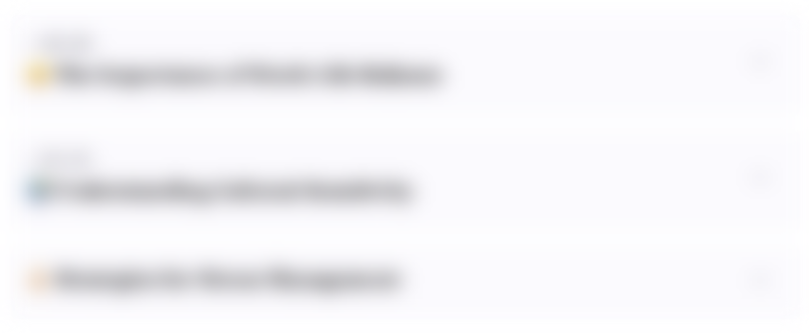
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
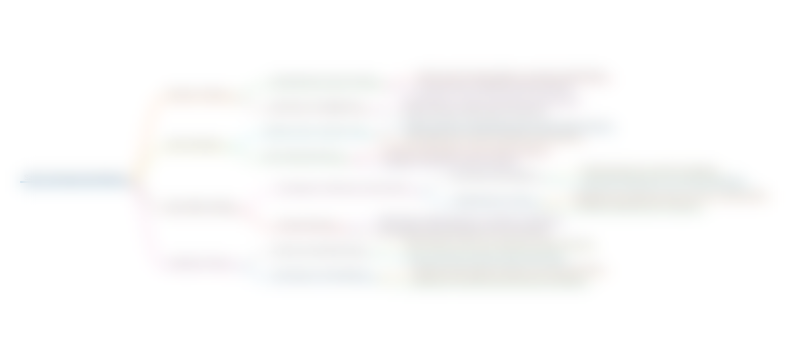
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
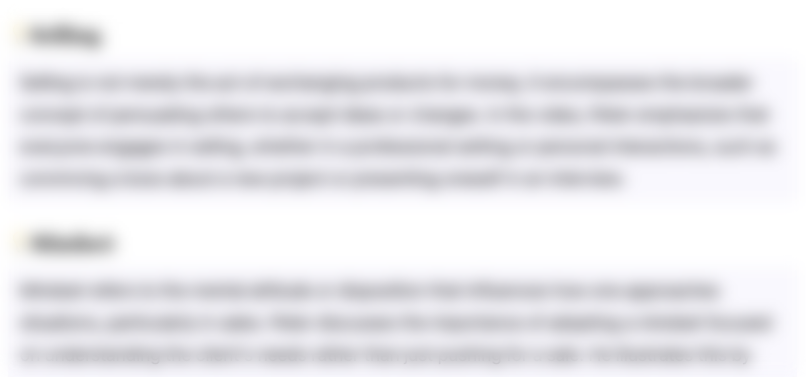
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
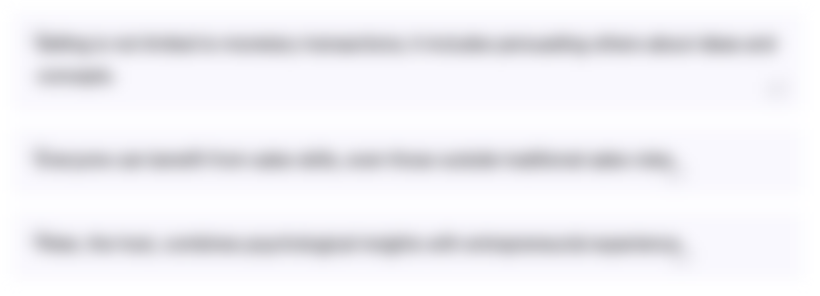
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
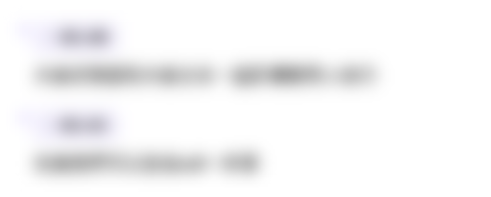
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

Cara Bertanya APAKAH untuk WAKTU LAMPAU | Yes - No Question Simple Past Tense Bahasa Inggris
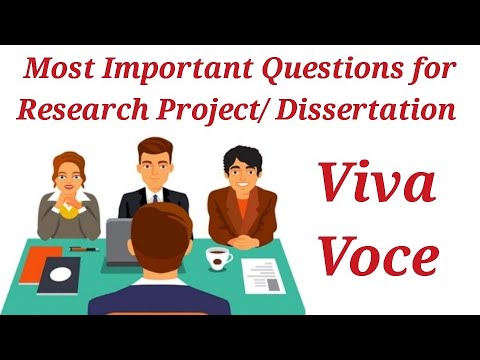
Most Important Viva Questions for Research Project, Dissertation | MA, MSc, PhD, MPhil - Psychology

Paragraphs (Part II) - Topic Sentences
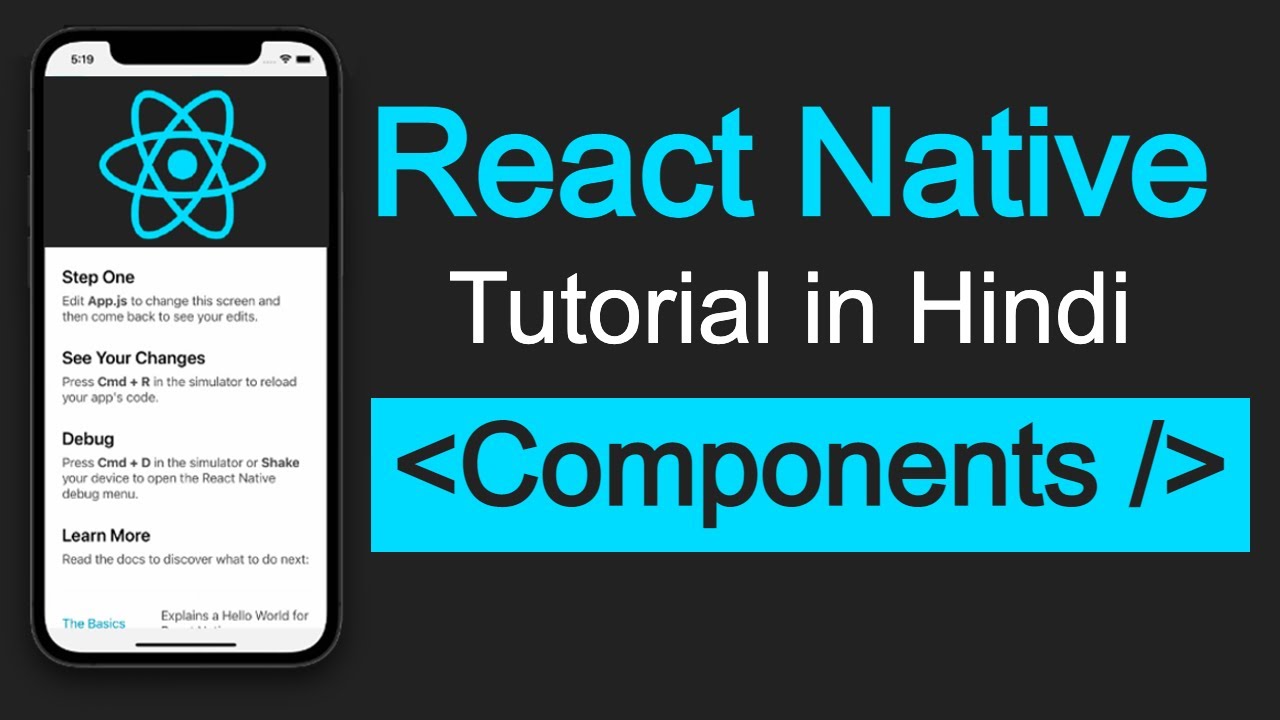
React Native tutorial in Hindi #7 What is component
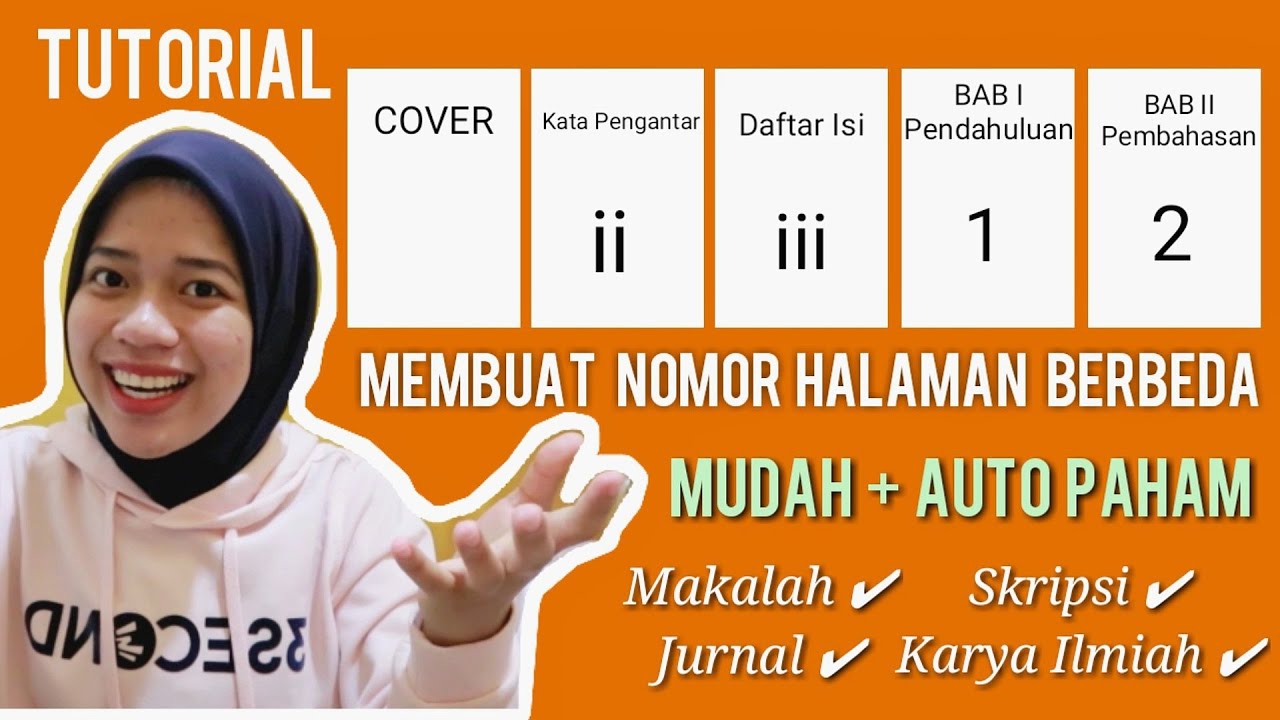
CARA MUDAH MEMBUAT NOMOR HALAMAN DI MICROSOFT WORD | TUTORIAL

Cara Menjumlahkan Nilai Raport di Excel Nilai Akhir, Ranking dan Nilai Grade
5.0 / 5 (0 votes)