Dictionary
Summary
TLDRThis lecture introduces Python dictionaries as a key-value pair data structure, using hash table concepts. It explains how to create, access, modify, and manipulate dictionaries with examples, including adding and updating key-value pairs, as well as removing elements using 'del' and 'clear' methods. The summary highlights the mutability of dictionaries and their practical applications in Python programming.
Takeaways
- 📚 A dictionary in Python is a data structure that maps keys to values, forming key-value pairs.
- 🔑 Dictionaries are enclosed by curly braces and use colons to separate keys and values, with keys on the left and values on the right.
- 🚀 To create a dictionary, assign values to keys using the format: `dictionary_name = {key1: value1, key2: value2, ...}`.
- 🖨 Printing a dictionary displays all key-value pairs contained within it.
- 🔍 Accessing a specific value in a dictionary is done by referencing its key, using the format: `dictionary_name[key]`.
- 🔑 The `keys()` method of a dictionary returns a list of all keys, and the `values()` method returns a list of all values.
- 🔄 The `items()` method provides a list of key-value pairs in the form of tuples.
- 🛠 Dictionaries are mutable, meaning you can add, modify, or remove key-value pairs.
- 📝 To add a new key-value pair, assign a value to a new key within the dictionary, or use the `update()` method.
- 🔄 To modify an existing key's value, assign a new value to the key within the dictionary.
- 🗑 To remove a key-value pair, use the `del` statement followed by the dictionary name and the key to be removed.
- 🧹 The `clear()` method removes all key-value pairs from a dictionary, resulting in an empty dictionary.
Q & A
What is a dictionary in Python?
-A dictionary in Python is a data structure that works as a hash table. It maps keys to values and is composed of key-value pairs enclosed by curly braces.
How are keys and values represented in a Python dictionary?
-In a Python dictionary, keys are placed to the left of the colon, and values are placed to the right. Keys are immutable types, usually numbers or strings.
Can you provide an example of creating a dictionary in Python?
-Yes, an example of creating a dictionary is `Fuel_type = {'Petrol': 1, 'Diesel': 2, 'CNG': 3}`. Here, 'Petrol', 'Diesel', and 'CNG' are keys, and 1, 2, and 3 are their corresponding values.
How do you print a dictionary in Python?
-You can print a dictionary in Python by using the `print` function followed by the dictionary name, e.g., `print(Fuel_type)`.
How can you access the value of a specific key in a dictionary?
-To access the value of a specific key, you use the key name within square brackets after the dictionary name, like `Fuel_type['Petrol']` which would return the value 1.
What is the method to access all keys of a dictionary?
-You can access all keys of a dictionary by using the `keys()` method, like `Fuel_type.keys()`, which returns a view of the dictionary's keys.
How do you access all values in a dictionary?
-To access all values, you use the `values()` method, such as `Fuel_type.values()`, which returns a view of all the values in the dictionary.
Can you retrieve both keys and values at the same time from a dictionary?
-Yes, you can retrieve both keys and values simultaneously using the `items()` method, e.g., `Fuel_type.items()`, which returns an iterable view of the dictionary's key-value pairs.
Is it possible to add a new key-value pair to an existing dictionary?
-Yes, you can add a new key-value pair to an existing dictionary by directly assigning a value to a new key within the dictionary, like `Fuel_type['Electric'] = 4`.
What is the built-in method to update a dictionary with multiple new key-value pairs?
-The `update()` method is the built-in function used to add multiple key-value pairs to a dictionary, e.g., `Fuel_type.update({'Electric': 4})`.
How can you modify the value of an existing key in a dictionary?
-To modify the value of an existing key, you assign a new value to that key within the dictionary, like `Fuel_type['CNG'] = 5`.
What is the purpose of the `del` statement when working with dictionaries?
-The `del` statement is used to remove a key-value pair from a dictionary, e.g., `del Fuel_type['Petrol']` which removes the 'Petrol' key and its associated value from the dictionary.
How do you clear all key-value pairs from a dictionary?
-You can clear all key-value pairs from a dictionary by using the `clear()` method, like `Fuel_type.clear()`, which empties the dictionary.
What is the significance of dictionaries being mutable in Python?
-The mutability of dictionaries in Python means that you can change their content after creation, allowing for the addition, modification, or removal of key-value pairs.
Outlines
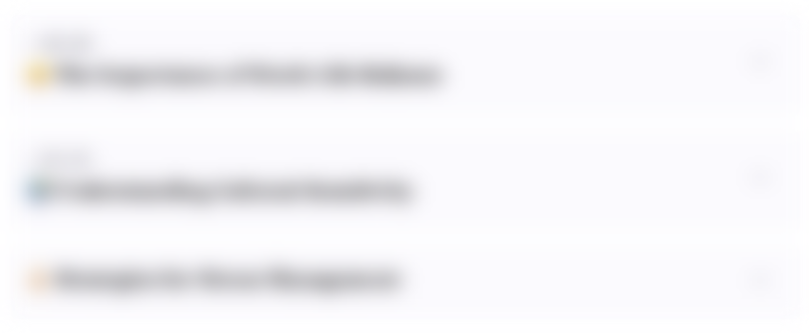
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
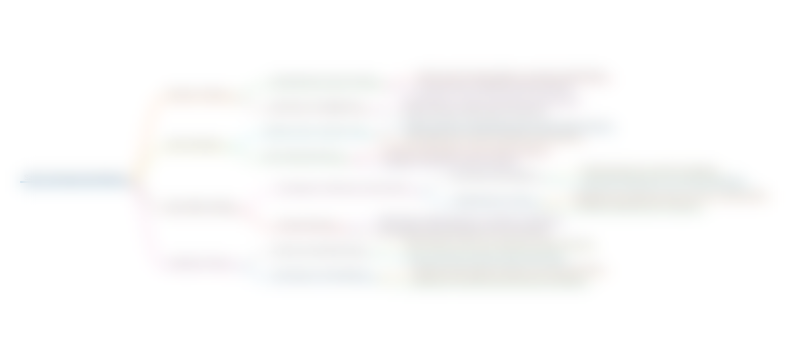
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
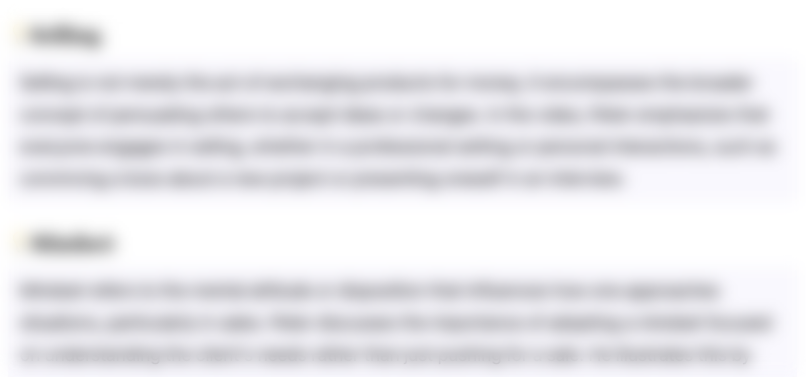
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
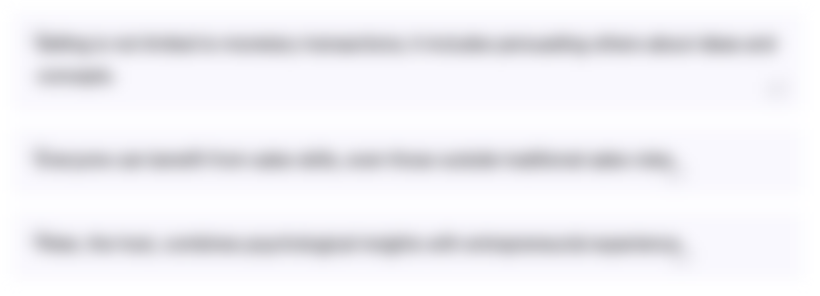
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
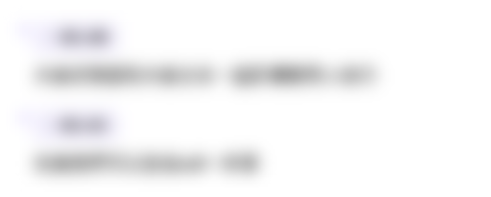
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)