Data Structures: Hash Tables
Summary
TLDRIn this video, Gayle Laakmann McDowell, author of 'Cracking the Coding Interview,' dives into the importance of hash tables, a fundamental data structure for coding interviews. She explains how hash tables allow for quick key-value lookups and introduces key concepts like hash functions, hash codes, and collision resolution using chaining. Gayle also covers the runtime performance of hash tables, emphasizing the average constant time for operations. While encouraging further exploration of advanced topics, she stresses the importance of mastering the basics for successful problem-solving in interviews and real-world applications.
Takeaways
- π Hash tables are crucial for coding interviews and real-world applications, offering efficient key-value lookups.
- π A hash table uses a hash function to map keys (like strings) to array indices for quick access.
- π A hash function converts a key into an integer, which is then mapped to an index in a smaller array.
- π Collisions occur when two keys map to the same index, and one common solution is chaining (using linked lists).
- π In chaining, when a collision happens, multiple key-value pairs are stored in a linked list at the same index.
- π When accessing a value, the hash function is used to find the correct index, and a linked list may be traversed to locate the key.
- π A hash table typically provides constant time complexity (O(1)) for insertions, deletions, and lookups in ideal conditions.
- π In cases of poor hash functions or high collision rates, the time complexity could degrade to linear time (O(n)).
- π Good hash functions distribute values evenly, ensuring efficient operations and reducing the chance of collisions.
- π The array in a hash table stores linked lists of key-value pairs, not the actual values directly, to handle collisions effectively.
- π Understanding hash tables is essential for coding interviews, and learning about collision resolution and resizing helps build a deeper knowledge.
Q & A
What is a hash table and why is it important?
-A hash table is a data structure that stores key-value pairs, allowing for fast lookups based on keys. It is important because it is one of the most efficient ways to store and retrieve data, making it a common choice in both interviews and real-life applications.
How does a hash table work at a high level?
-At a high level, a hash table uses a hash function to convert a key into an index in an array, where the corresponding value is stored. This allows for very fast lookups, as the index directly maps to the location of the value.
What is the role of the hash function in a hash table?
-The hash function takes a key (like a string) and converts it into an integer, which is then mapped to an index in the hash table's array. This enables the hash table to quickly locate the value associated with the given key.
Why is a hash code not directly used as the index in a hash table?
-The hash code is not directly used as the index because there are a finite number of possible array indices but an infinite number of potential hash codes. Therefore, the hash code is remapped to fit within the bounds of the array.
What happens when two keys result in the same hash code?
-When two different keys have the same hash code, it is called a collision. One way to resolve this is by using chaining, where each array index holds a linked list of key-value pairs, allowing multiple entries to map to the same index.
What is the chaining method used to resolve collisions in a hash table?
-Chaining involves storing multiple values that hash to the same index in a linked list. When a collision occurs, the new key-value pair is simply added to the linked list at the corresponding index.
How does a hash table perform lookups when there is a collision?
-When a collision occurs, the hash table must traverse the linked list at the index to find the correct key-value pair. This adds some overhead compared to an ideal case with no collisions.
What is the expected time complexity for operations in a hash table?
-In an ideal case with a good hash function, the time complexity for operations like insert, find, and delete is constant (O(1)). However, in the worst-case scenario, such as when many collisions occur, the time complexity can degrade to linear time (O(n)).
How can a hash table grow or resize when it reaches its capacity?
-While not discussed in detail in the video, hash tables often resize by allocating a new, larger array and rehashing the existing elements when the load factor (number of elements relative to the size of the array) exceeds a threshold. This allows the hash table to maintain its efficiency as it grows.
Why is it important to have a good hash function in a hash table?
-A good hash function helps to evenly distribute the keys across the array, minimizing collisions and ensuring that the hash table performs efficiently with constant-time operations. A poor hash function can lead to frequent collisions and degrade performance.
Outlines
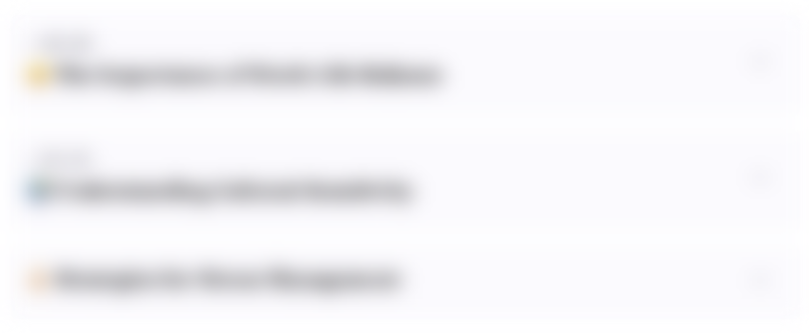
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
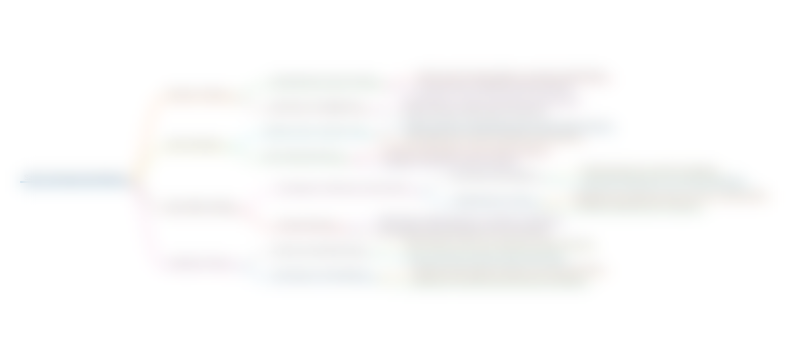
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
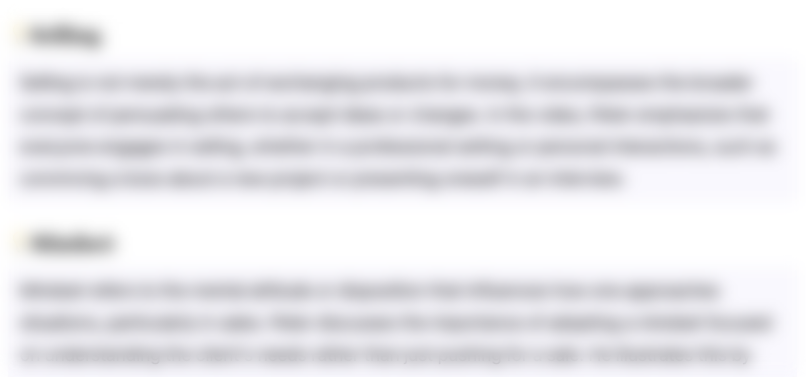
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
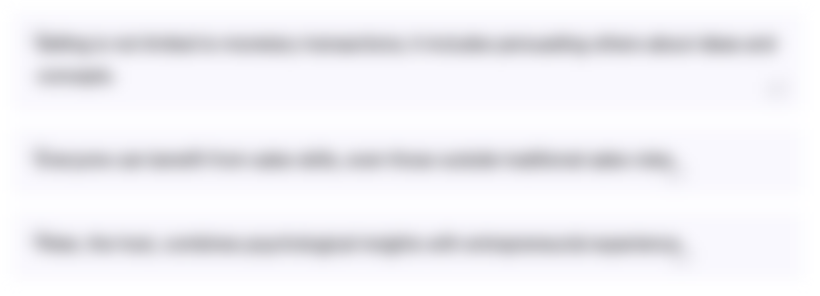
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
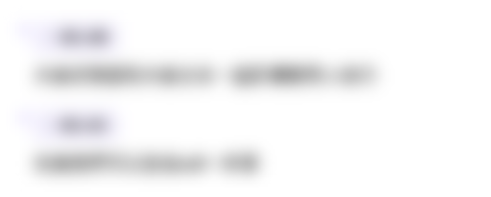
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

Algorithms: Binary Search
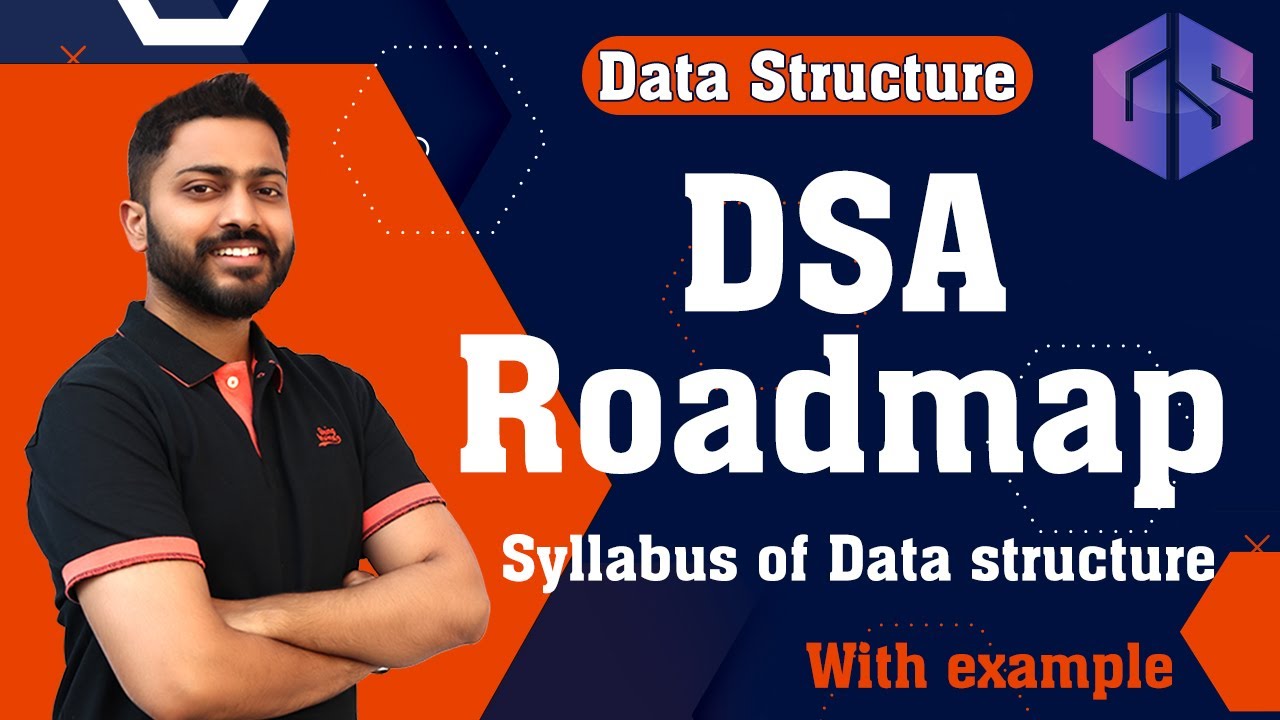
Roadmap π£οΈ of DSA | Syllabus of Data structure | Data Structure for Beginners

What is a HashTable Data Structure - Introduction to Hash Tables , Part 0
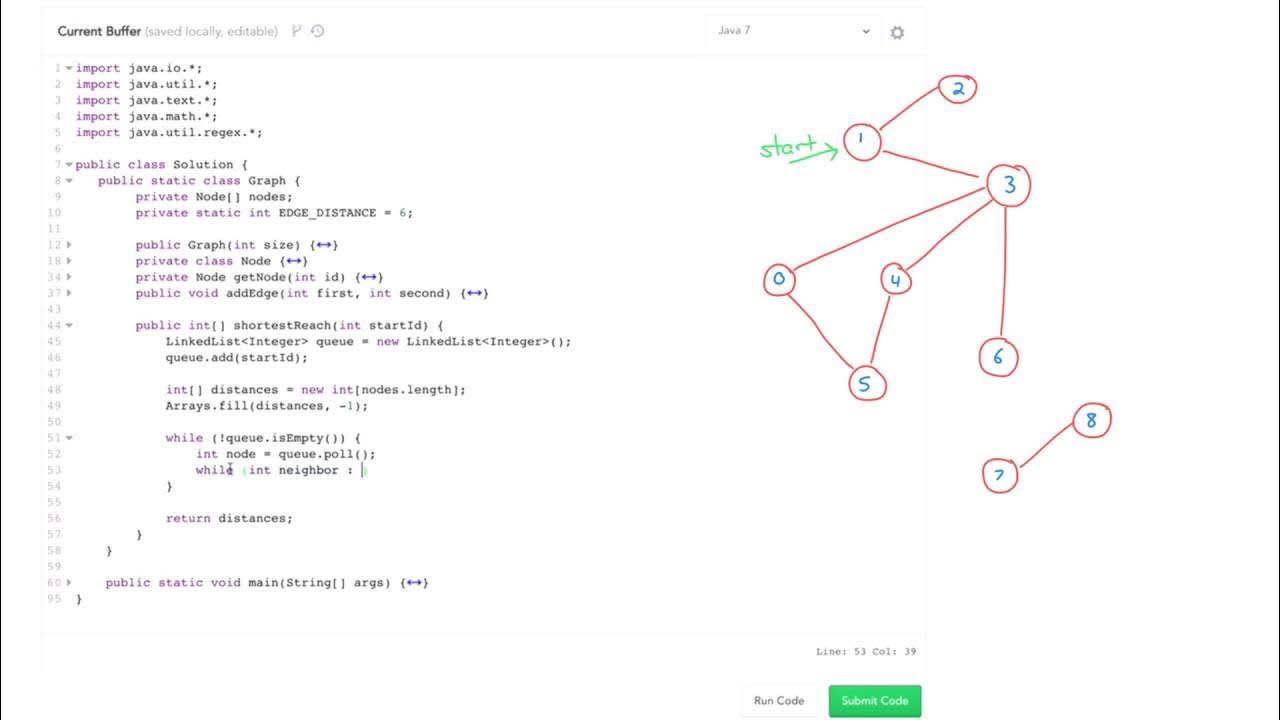
Algorithms: Solve 'Shortest Reach' Using BFS
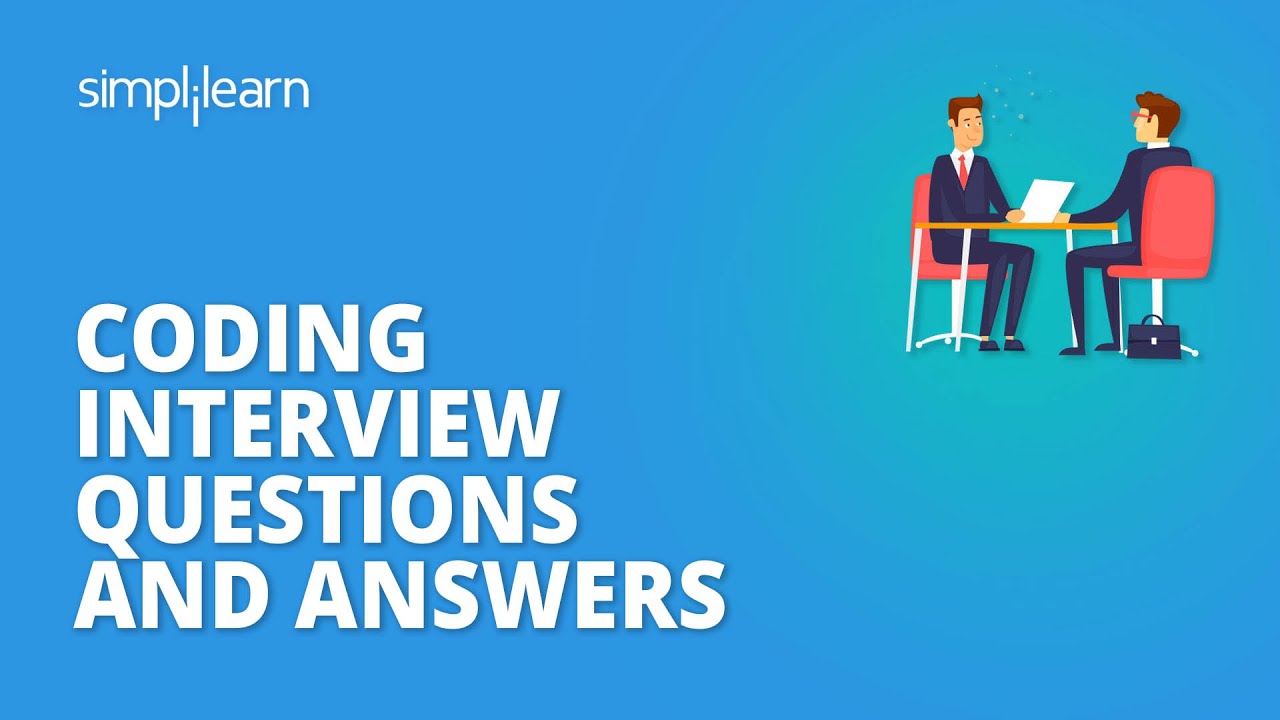
Coding Interview Questions And Answers | Programming Interview Questions And Answers | Simplilearn
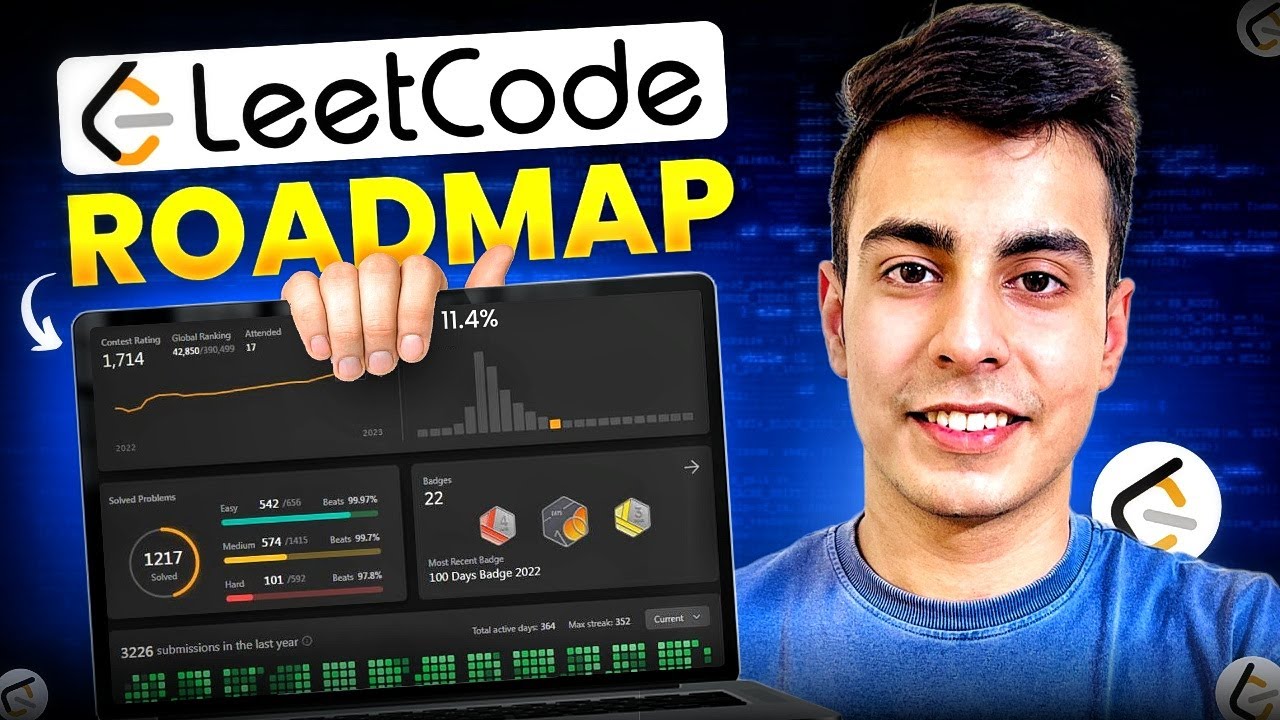
How I Used LeetCode & Cracked 5+ Job Offers | Full Strategy | Atlassian, Juspay, BNY Mellon
5.0 / 5 (0 votes)