Dictionaries | Godot GDScript Tutorial | Ep 12
Summary
TLDRThis episode of the GD Script Fundamentals tutorial series delves into the concept and usage of dictionaries in Godot's GD Script. Dictionaries are associative containers that store values referenced by unique keys, often used as key-value stores. The tutorial explains how to create, access, and manipulate dictionaries, including adding and removing key-value pairs. It also covers how to compare dictionaries using the hash method and clear them using specific methods. The script provides examples of declaring dictionaries with various data types as keys and values, and demonstrates how to work with nested dictionaries. The episode concludes with a GitHub link for further exploration and practice.
Takeaways
- ๐ A dictionary in GDScript is an associative container that stores values referenced by unique keys, also known as a key-value store.
- ๐ Creating a dictionary involves using the 'var' keyword, a variable name, and curly braces with key-value pairs separated by colons.
- ๐ฏ Keys can be of any literal value, with strings being the most common, but integers and booleans can also be used.
- ๐ To declare an empty dictionary, simply use curly braces with nothing inside.
- ๐ Accessing a value in a dictionary is done by using the variable name followed by square brackets containing the key.
- ๐ Adding a key-value pair to an existing dictionary can be done using dot notation or square brackets for keys.
- ๐ Complex data types, such as arrays or even other dictionaries, can be used as values within a dictionary.
- โ ๏ธ When using strings as keys, it's important to note that they are case-sensitive.
- ๐ To compare two dictionaries, use the 'hash' method instead of direct comparison with '==' as it converts the dictionary into a set of integers for comparison.
- ๐๏ธ The 'clear' method can be used to remove all key-value pairs from a dictionary, while the 'erase' method is used to remove a specific key.
- ๐ The tutorial provides an example GD file demonstrating the creation, assignment, and manipulation of dictionaries, including nested dictionaries and array values.
Q & A
What is a dictionary in the context of GDScript?
-A dictionary in GDScript is an associative container that stores values referenced by unique keys, also known as a key-value store.
How do you create a dictionary in GDScript?
-You create a dictionary in GDScript by using the 'var' keyword, followed by a variable name and an equal sign, then enclosing key-value pairs within curly braces.
What can be used as keys in a GDScript dictionary?
-Keys in a GDScript dictionary can be any literal value, with string values being the most common, but integers and booleans can also be used.
How do you declare an empty dictionary in GDScript?
-You declare an empty dictionary in GDScript by simply using curly braces with nothing inside: {}.
Can you use complex data types as values in a GDScript dictionary?
-Yes, you can use complex data types such as arrays or even other dictionaries as values in a GDScript dictionary.
How do you access a value in a dictionary using its key?
-To access a value, you type out the variable name of the dictionary followed by square brackets containing the key, using double quotes for string keys and the integer itself for integer keys.
What is the difference between using dot notation and square brackets when adding a key-value pair to an existing dictionary in GDScript?
-Dot notation automatically converts the key to a string, while square brackets allow you to use integer or string values as keys with more control.
Why can't you use direct comparison with the double equal sign for dictionaries in GDScript?
-Direct comparison with the double equal sign for dictionaries will always return false, even if the key-value pairs are the same, because you need to compare the hashes of the dictionaries instead.
How do you compare two dictionaries for equality in GDScript?
-To compare two dictionaries for equality, you should use the 'hash' method on both dictionaries and compare the resulting hash values.
What methods can be used to clear or remove a key from a dictionary in GDScript?
-The 'clear' method can be used to remove all key-value pairs from a dictionary, and the 'erase' method can be used to remove a specific key by specifying its value.
Can you assign one dictionary to another in GDScript?
-Yes, you can assign one dictionary to another by using the variable of one dictionary as the value for a key in the other dictionary.
Outlines
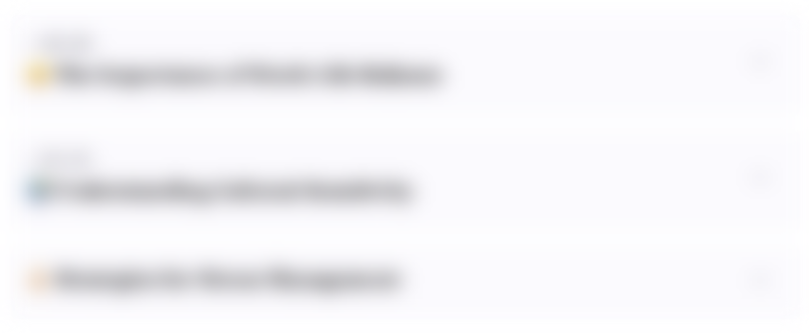
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
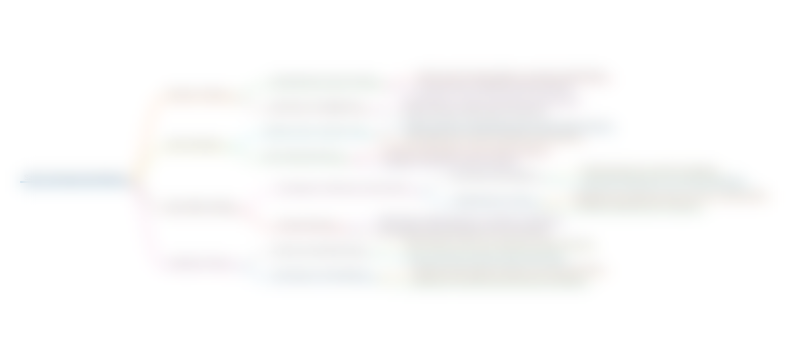
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
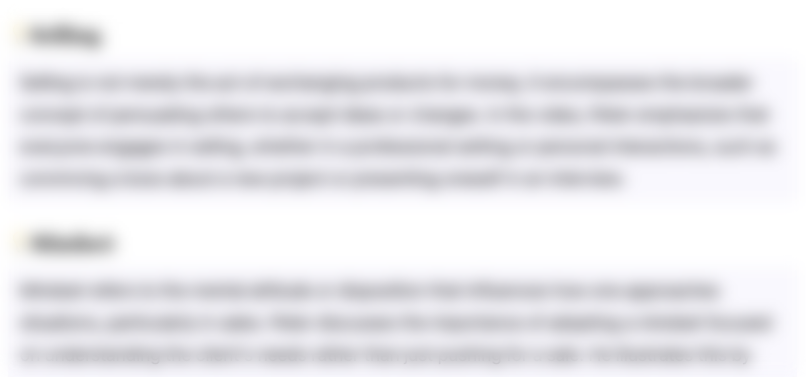
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
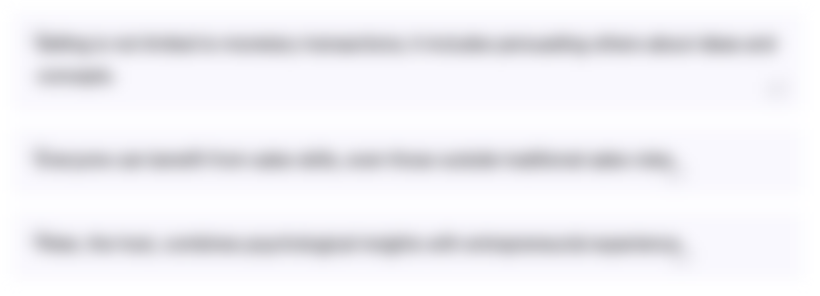
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
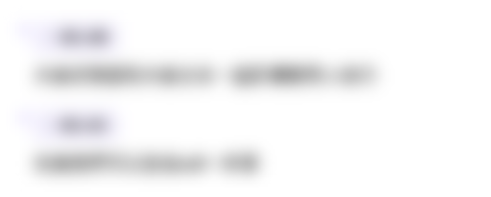
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)