What is a HashTable Data Structure - Introduction to Hash Tables , Part 0
Summary
TLDRIn this tutorial, Paul introduces hash tables, a data structure used to store information in key-value pairs. He explains how a hash table maps keys to array indices using a hash function, which ensures fast lookups. Paul discusses how collisions are handled using chaining, where linked lists are formed at the same array index. The video aims to simplify the concept of hash tables, demonstrating their efficiency in searching for values by hashing keys to specific indices. Paul also plans to provide coding examples for implementing hash tables in C++ in future videos.
Takeaways
- 😀 A hash table is a data structure used to store information in key-value pairs.
- 😀 The key in a hash table could be something like a name, and the value could be a related piece of information, like a phone number.
- 😀 Hash tables use an array structure where each index holds a key-value pair, and the key is mapped to a specific index using a hash function.
- 😀 A hash function evaluates a key and returns an index, helping to determine where the value should be stored in the array.
- 😀 The hash function ensures that for the same key, the same index is returned every time.
- 😀 Collisions can occur when two keys hash to the same index, meaning multiple values are stored at the same location.
- 😀 One way to handle collisions is by chaining, which involves creating a linked list at each index to store multiple values.
- 😀 When searching for a value, the hash function directs you to the correct index, where you can traverse the linked list if needed.
- 😀 Chaining reduces the need to search the entire hash table by efficiently organizing values at specific indexes.
- 😀 A well-implemented hash table provides fast access to data, even when dealing with a large number of entries or collisions.
- 😀 In future tutorials, the speaker will demonstrate how to code a hash table in C++, focusing on implementing the chaining technique.
Q & A
What is a hash table?
-A hash table is a data structure used to store information in key-value pairs. Each key is associated with a specific value, and the data is stored in an array-like structure with indices derived from a hash function.
How does a hash table store data?
-Data is stored in a hash table by mapping the key to an index using a hash function. Each key-value pair is placed in the array at the index provided by the hash function.
What are the components of a hash table?
-The two main components of a hash table are the 'key' and the 'value'. The key is a unique identifier, while the value is the data associated with that key.
What is the role of the hash function in a hash table?
-The hash function takes a key as input and returns an index where the corresponding value will be stored in the hash table's array. It ensures that the same key always maps to the same index.
What happens if two keys map to the same index in a hash table?
-When two keys map to the same index, it creates a collision. One common method for handling collisions is 'chaining', where a linked list of key-value pairs is stored at the same index.
What is chaining in the context of hash tables?
-Chaining is a collision resolution technique where, instead of replacing the value at a given index, additional key-value pairs are linked together in a list at that index.
Why do we need to handle collisions in hash tables?
-Collisions occur when multiple keys hash to the same index. Handling collisions is crucial to maintain the integrity and efficiency of the hash table, ensuring that all data is stored and accessible.
How does a hash table search for a value?
-To search for a value in a hash table, the key is passed to the hash function, which calculates the index. The value is then retrieved from the corresponding index, and if there are collisions, the linked list at that index is searched.
What are the advantages of using a hash table?
-Hash tables offer efficient data retrieval, as the hash function directly provides the index where the value is stored, minimizing the need for searching through large datasets.
What might be a downside of using chaining to handle collisions?
-A downside of chaining is that it can lead to longer linked lists at certain indices if many collisions occur, which can reduce the overall efficiency of the hash table as search times increase.
Outlines
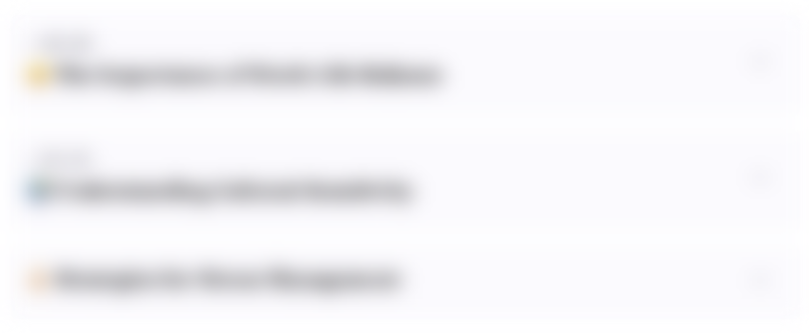
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
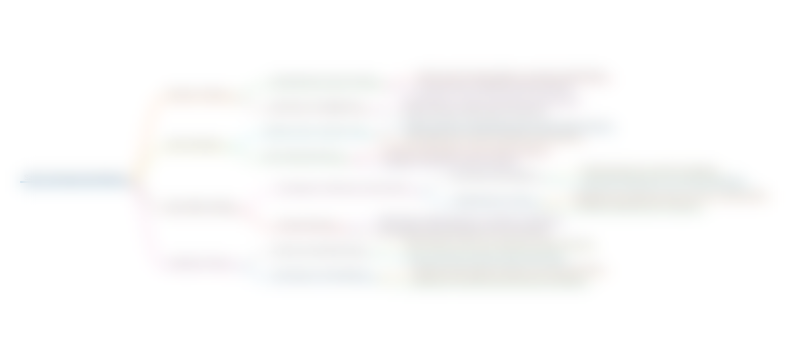
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
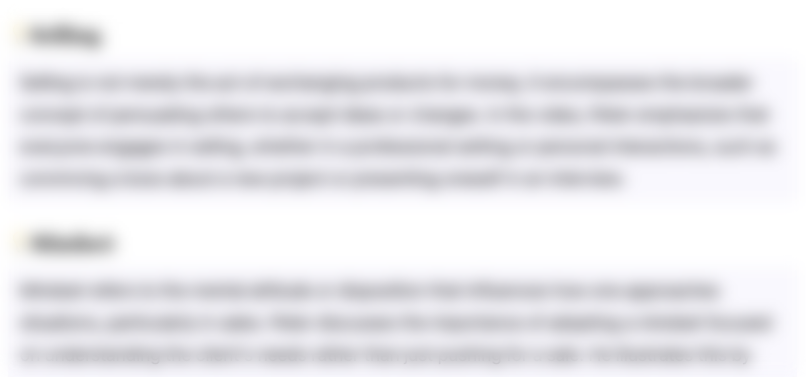
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
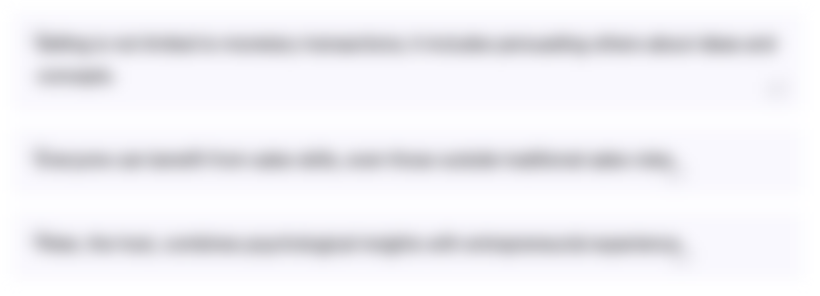
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
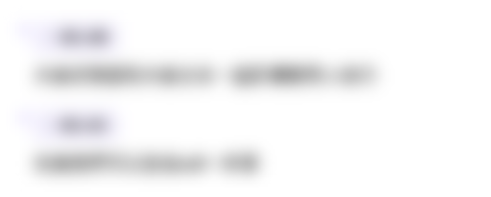
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)