AC101 Key Value Pairs
Summary
TLDRThis video explains how dictionaries in Python are used to map unique keys to values, offering a fast and efficient way to look up data by name. The concept is compared to how a traditional dictionary maps words to definitions. Internally, Python dictionaries use hash tables, where a hash function quickly locates the correct value associated with a key, avoiding time-consuming row-by-row searches. The video also addresses potential hash collisions and assures viewers that Python handles them efficiently. This powerful tool is crucial for anyone learning Python, enabling fast data retrieval and manipulation.
Takeaways
- π Dictionaries in Python allow fast lookups of values using a key, making them ideal for mapping information like country capitals.
- π Unlike lists and strings, which use numerical indices, dictionaries use named keys for accessing data.
- π In Python, the process of mapping involves associating a name (key) with a corresponding piece of information (value).
- π The key-value pairs in dictionaries are stored using a data structure called a hash table, which enables efficient lookups.
- π When searching for a value in a dictionary, Python uses a hash function to compute the location of the key in the table.
- π Hash functions convert a string (e.g., 'India') into a numerical hash value, allowing for quick access to the corresponding data.
- π The efficiency of hash tables comes from directly accessing the location of key-value pairs, instead of scanning through the entire table.
- π When adding a new key-value pair, Python uses the hash function to compute the location and store the new entry efficiently.
- π Hash collisions, where two keys produce the same hash value, can occur but are handled effectively by Python's internal mechanisms.
- π Pythonβs dictionary structure is designed for fast insertion and retrieval, making it a powerful tool in programming, especially for handling large datasets.
- π Understanding how dictionaries work behind the scenes, especially the role of hash tables and hash functions, can help demystify their use and increase their effectiveness in code.
Q & A
What is the main purpose of using a dictionary in Python?
-The main purpose of using a dictionary in Python is to store data in key-value pairs, allowing you to quickly look up information based on a unique key, rather than a numerical index.
What is meant by 'mapping' in programming?
-'Mapping' refers to the operation of associating a unique identifier (the key) with specific information (the value), which allows for fast retrieval of the value when the key is provided.
What is the role of the key in a Python dictionary?
-In a Python dictionary, the key is the unique identifier used to look up a corresponding value. The key must be immutable (e.g., strings, numbers, or tuples).
How does a hash table work in Python dictionaries?
-A hash table in Python dictionaries uses a hash function to compute a hash value for each key. This value determines the specific location in the table where the key-value pair is stored, allowing for fast lookups.
Why might the order of items in a Python dictionary be different from the order in which they were inserted?
-The order of items in a Python dictionary may differ because the dictionary stores data using a hash table. The hash function determines where each key-value pair is placed in memory, which may not correspond to the order of insertion.
What happens if two keys in a dictionary hash to the same location?
-If two keys hash to the same location, Python handles this collision by using techniques like chaining or open addressing, which ensures that both key-value pairs can be stored and retrieved efficiently.
How does Python ensure efficient lookups when using dictionaries?
-Python ensures efficient lookups in dictionaries by using a hash table and a hash function. The hash function computes a hash value for a key, allowing Python to directly access the memory location of the corresponding value, which is much faster than searching through a list.
What is an example of using a dictionary to map countries to their capitals in Python?
-An example would be: `countries = {'India': 'New Delhi', 'France': 'Paris'}`. To get the capital of India, you would use: `countries['India']`, which would return 'New Delhi'.
How can you add a new key-value pair to an existing Python dictionary?
-You can add a new key-value pair to a Python dictionary by using the assignment syntax: `dictionary[key] = value`. For example, `countries['Malta'] = 'Valletta'` adds Malta and its capital to the dictionary.
What makes hash functions efficient in terms of performance in Python dictionaries?
-Hash functions are efficient because they generate a unique numerical value for each key, allowing Python to directly access the memory location of the value associated with that key. This eliminates the need for a linear search and significantly speeds up data retrieval.
Outlines
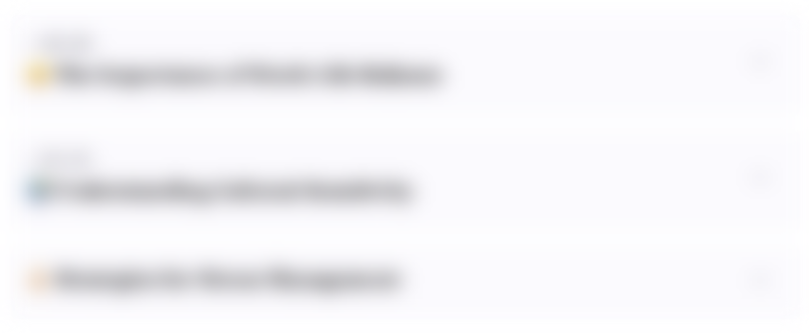
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
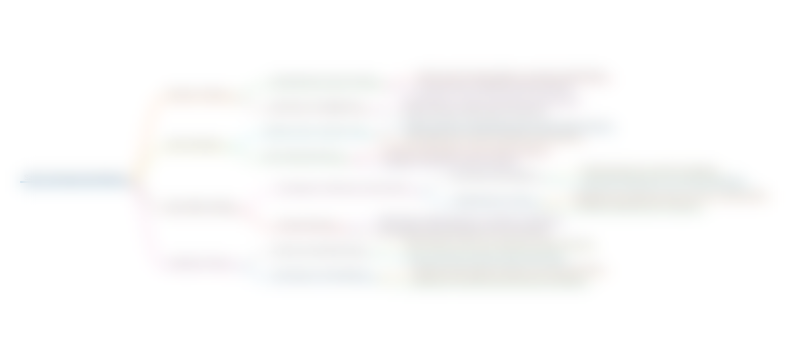
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
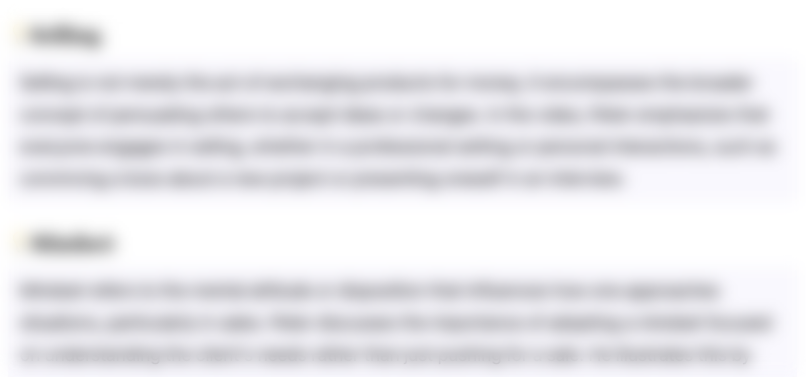
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
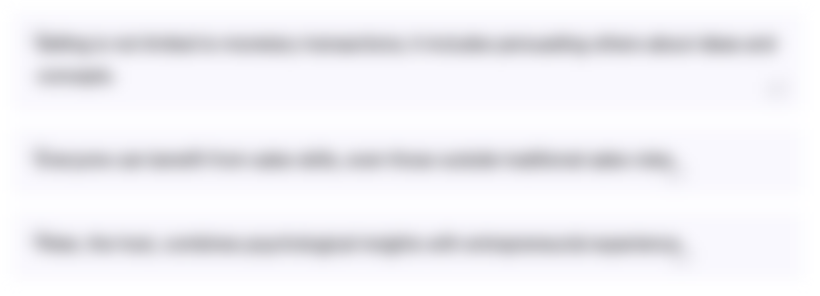
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
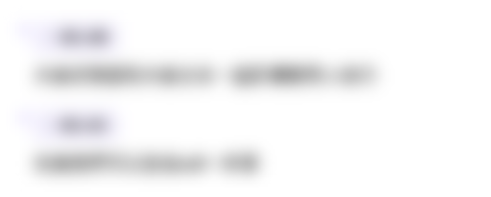
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)