COS 333: Chapter 12, Part 2
Summary
TLDRThis lecture delves into object-oriented programming (OOP) languages, focusing on C++, Java, C#, and Ruby. It explores the design issues and characteristics of each language, including C++'s efficiency and hybrid nature, Java's object-oriented purity and memory management, C#'s class and struct distinctions, and Ruby's dynamic and flexible OOP approach. The lecture also covers access controls, inheritance, polymorphism, and the unique features of each language, providing a comprehensive understanding of OOP in different programming environments.
Takeaways
- 📘 C++ is a hybrid language that supports both imperative and object-oriented programming paradigms, inheriting much of its syntax from C.
- 🔒 C++ uses access controls like public, private, and protected to manage the visibility of class members, with specific rules for inheritance to maintain encapsulation.
- 🔄 C++ supports multiple inheritance, which allows a class to inherit from more than one parent class, but this can lead to complexities in member access.
- 🚀 C++ is designed with efficiency in mind, offering fast execution speeds, especially when compared to languages like Smalltalk.
- 🔄 Java is more object-oriented than C++ and requires all data to be in the form of objects, with wrapper classes for primitive data types.
- 🗑️ Java manages memory through a garbage collector, relieving the programmer from manual memory deallocation, unlike C++ which requires manual management.
- 🔗 Java supports single inheritance and interfaces to simulate multiple inheritance without the complexities associated with true multiple inheritance.
- 📝 C# differs from Java in that it includes structs in addition to classes, with structs being stack-allocated and not supporting inheritance or self-referencing.
- 📜 Ruby is a pure object-oriented language where everything is an object, and it shares similarities with Smalltalk in terms of message passing and dynamic nature.
- 🔄 Ruby allows for dynamic changes to class and method definitions during runtime, offering flexibility but potentially complicating maintenance and predictability.
- 🛑 Ruby does not support abstract classes or direct multiple inheritance but uses modules to provide a form of multiple inheritance through mixing functions into classes.
Q & A
What programming language is considered a hybrid language and why?
-C++ is considered a hybrid language because it has a mixed type system, inheriting an imperative type system from C and adding an object-oriented class system, allowing for both procedural and object-oriented programming to be used interchangeably within the same program.
What are the three types of access controls in C++ for class members?
-The three types of access controls in C++ for class members are public, private, and protected. Public members are accessible to subclasses and client code, private members are only visible within the class and to friends, and protected members are visible to the class, friends, and subclasses but not to client code.
How does private inheritance in C++ differ from public inheritance?
-Private inheritance in C++ makes the public and protected members of the parent class private in the derived class, effectively disallowing the derived class from being a subtype of the parent class. Public inheritance, on the other hand, does not change the access controls of the inherited members, allowing the derived class to maintain the same behavior as the parent class.
What is the default message binding in C++ and why is it the default?
-The default message binding in C++ is static message binding. It is the default because it is much faster than dynamic binding, which aligns with C++'s design focus on efficiency.
What is the difference between a class and a struct in C#?
-In C#, a class is a reference type that can be allocated on the heap and supports inheritance, while a struct is a value type that is stack-allocated and does not support inheritance. Structs are less powerful than classes and cannot reference themselves, making them unsuitable for creating complex data structures like linked lists or trees.
Why is Java's approach to message binding simpler than C++'s?
-Java's approach to message binding is simpler because almost all message binding in Java is dynamic by default, requiring no special keywords for virtual methods as in C++. Static binding in Java only occurs with final, private, or static methods, which are the only cases where methods cannot be overridden.
What is the purpose of interfaces in Java and how do they differ from C++'s approach to multiple inheritance?
-Interfaces in Java provide a way to simulate multiple inheritance by allowing classes to implement multiple interfaces. Unlike C++, which supports true multiple inheritance, Java interfaces only provide method declarations and named constants, without the ability to implement methods or have instance variables, thus avoiding the complexity and potential issues of multiple inheritance.
How does Ruby's approach to object-oriented programming differ from the other languages discussed?
-Ruby's approach differs significantly as it is a more pure object-oriented language where everything is an object, similar to Smalltalk. It supports dynamic message binding with typeless object references, and allows for changes in class and method definitions at runtime, providing a flexible but less static approach compared to languages like C++, Java, and C#.
What is the concept of 're-exportation' in C++ and why is it used?
-Re-exportation in C++ is the process of making a member that is private due to private inheritance visible as if it had been inherited publicly. It is used to overcome the limitation of private inheritance, which changes the access of inherited members to private, by using the scope resolution operator to re-export the member with its original access level.
Why is dynamic message binding not required for methods marked as final in Java?
-Dynamic message binding is not required for final methods in Java because final methods cannot be overridden in derived classes. Since there can't be an overridden method to bind to, static binding is used instead, which is more efficient as it doesn't require the overhead of determining the method to call at runtime.
How does Ruby's handling of access control for data and methods differ from C++?
-In Ruby, all data within a class is private by default and can only be accessed by methods within that class, similar to C++. However, Ruby checks method access at runtime, unlike C++ where access can usually be checked at compile time. This difference is due to Ruby's dynamic nature and the fact that all variables are typeless object references.
Outlines
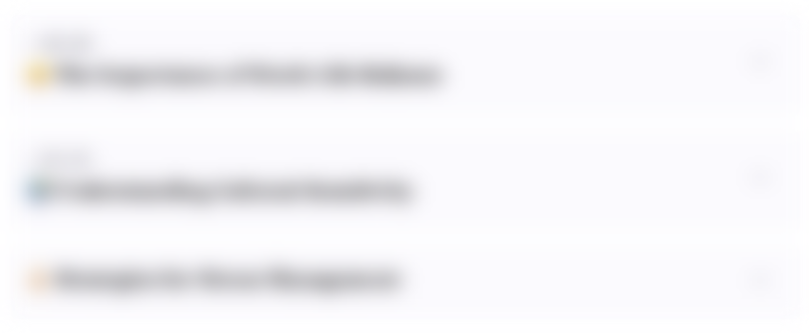
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
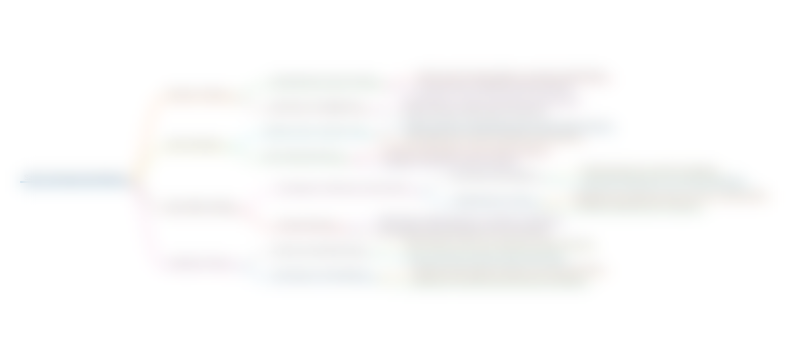
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
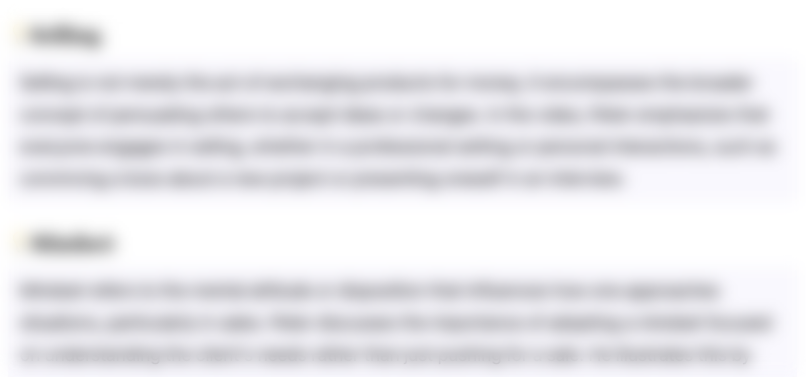
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
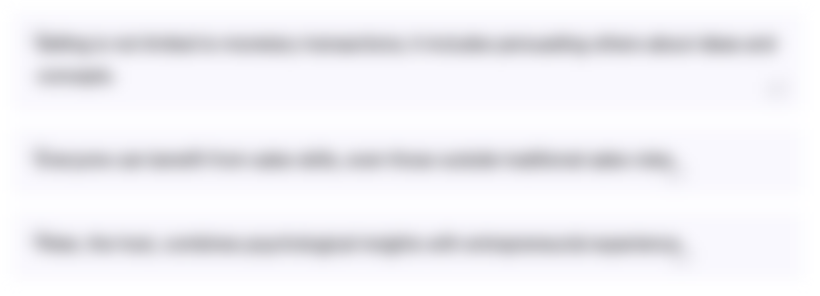
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
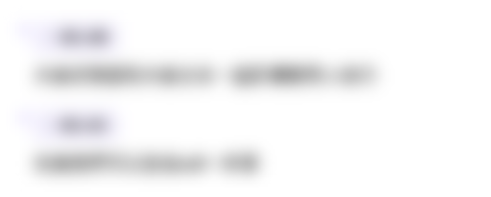
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)