COS 333: Chapter 15, Part 3
Summary
TLDRThis lecture concludes the chapter on functional programming languages, focusing on Scheme and its list operations, numeric predicate functions, and control flow. It delves into recursive function implementations, tail recursion, and the efficiency benefits of converting non-tail recursive functions. The power of first-class functions is demonstrated through 'map' and 'eval', and a brief comparison with Common Lisp is provided. The lecture also touches on real-world applications of functional languages, particularly in AI, and contrasts functional with imperative programming, highlighting their respective advantages.
Takeaways
- ๐ The lecture series concludes with a discussion on functional programming languages, focusing on Scheme and its capabilities for list operations and recursion.
- ๐ The script delves into numeric predicate functions, control flow, and list data structure functions in Scheme, emphasizing the language's pure functional nature without loop constructs.
- ๐ An in-depth look at the 'equal?' and 'equal-simp' functions illustrates how to recursively compare elements in lists, highlighting base cases and recursive cases in Scheme.
- ๐ง The 'append' function is explored, demonstrating how to concatenate lists in Scheme using recursion and the 'cons' function, rather than appending atom by atom.
- ๐ Tail recursion in Scheme is mandatory for the compiler to optimize recursive calls into iterative constructs, improving efficiency and reducing memory usage.
- ๐ ๏ธ The 'apply-to-all' functional form showcases the power of first-class functions in Scheme, allowing functions to be passed as parameters and returned as results.
- ๐ค Common Lisp is contrasted with Scheme, presenting a more complex language with additional features like iterative control structures and a rich set of data structures.
- ๐ข Applications of Lisp and Scheme are discussed, including their historical use in AI, knowledge representation, machine learning, and natural language processing.
- ๐ซ Scheme's role in education is highlighted, being used to teach introductory programming concepts in universities and colleges.
- ๐ก A comparison between functional and imperative programming languages is made, noting the simplicity and mathematical nature of functional languages versus the efficiency and complexity of imperative ones.
- ๐ The script provides guidance for students preparing for tests and exams, emphasizing the importance of understanding the provided code examples and practical exercises.
Q & A
What is the main focus of the third and final lecture on chapter 15?
-The lecture concludes the discussion on functional programming languages, specifically focusing on Scheme functions, tail recursion, first-class functions, and a brief overview of Common Lisp and real-world applications of functional programming languages.
What are predicate functions in Scheme?
-Predicate functions in Scheme are functions that evaluate to a boolean result, typically used for testing certain conditions or properties of data.
How does Scheme handle control flow within its functions?
-Scheme handles control flow through the use of recursion, as it is a pure functional programming language and does not have traditional loop structures.
What is the purpose of the 'equal simp' function in Scheme?
-The 'equal simp' function is used to compare two simple lists (lists containing only atoms, no nested sublists) and returns true if the lists are equal in terms of their elements, and false otherwise.
What is tail recursion and why is it important in Scheme?
-Tail recursion is a form of recursion where the recursive call is the last operation performed in a function. It is important in Scheme because the language definition requires all tail recursive functions to be automatically converted to iterative structures, making them more efficient in terms of speed and memory usage.
How can a non-tail recursive function be converted into a tail recursive function in Scheme?
-A non-tail recursive function can be converted into a tail recursive function by introducing an additional parameter, often called an accumulator or a 'partial' result, which carries the ongoing result through each recursive call, allowing the recursive call to be the last operation.
What is the significance of first-class functions in Scheme?
-First-class functions in Scheme mean that functions can be treated like any other data type. They can be passed as arguments to other functions, returned as results, and assigned to variables. This feature is demonstrated through functions like 'map car' and 'adder', which utilize functional forms.
What is Common Lisp and how does it differ from Scheme?
-Common Lisp is a dialect of the Lisp programming language that combines features from many popular Lisp dialects in the early 1980s. It is a large and complex language with a rich set of data structures and powerful I/O capabilities, unlike Scheme, which is designed to be simple and easy to understand.
What are some real-world applications of functional programming languages?
-Functional programming languages have been used in various fields such as artificial intelligence for knowledge representation, machine learning, and natural language processing. They have also been used in end-user software systems like the Emacs text editor and the Maxima mathematics system.
What are the advantages of functional programming languages over imperative programming languages in terms of concurrency?
-Functional programming languages naturally divide programs into functions, which can be executed concurrently or in a distributed fashion without the programmer having to explicitly manage mutual exclusion locks or other concurrency control mechanisms.
What type of questions can be expected in tests and exams related to functional programming languages?
-Tests and exams may include theory questions, questions asking for the result of provided Scheme code, analysis and correction of Scheme code, and implementation of Scheme functions with a complexity level similar to the examples covered in lectures.
Outlines
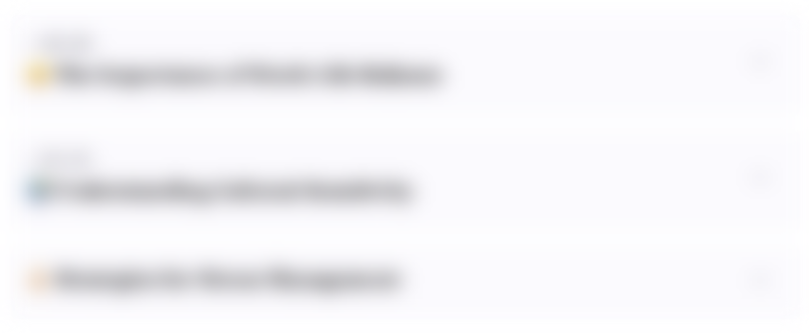
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
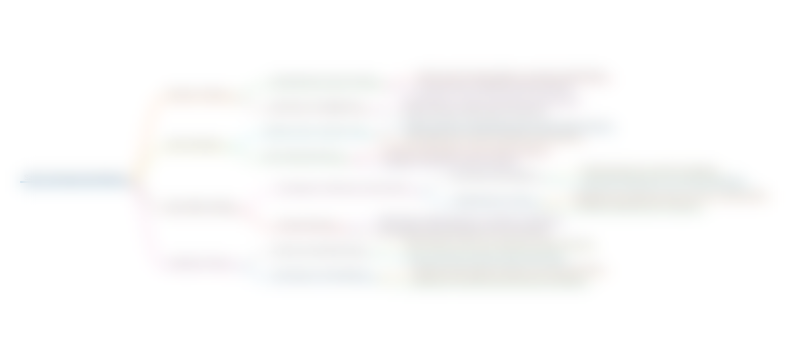
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
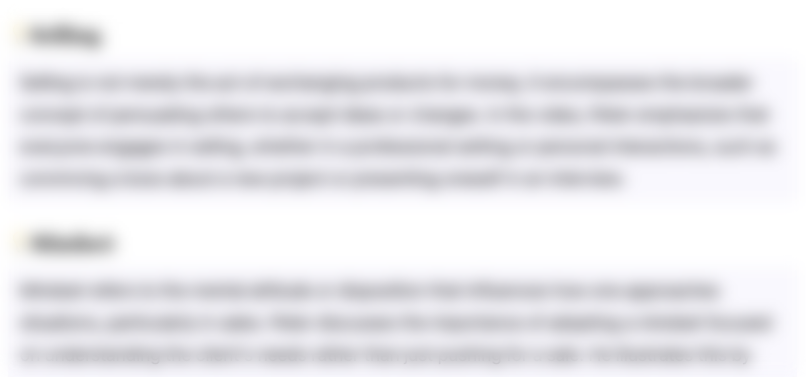
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
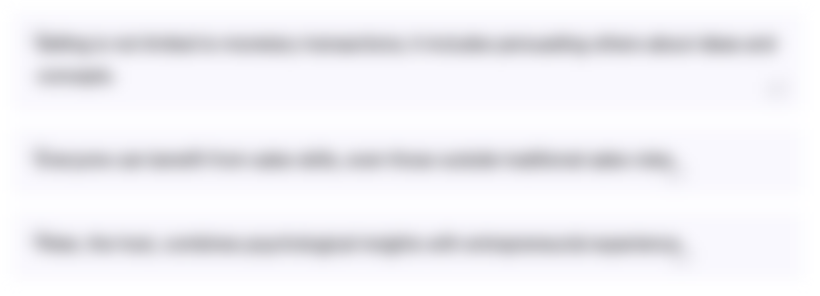
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
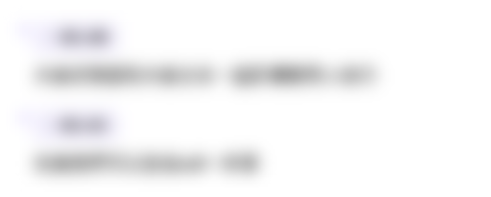
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)